java类的加载以及初始化顺序
2017-03-07 10:18
260 查看
类的加载和初始化的了解对于我们对编程的理解有很大帮助,最近在看类的记载方面的问题。从网上查阅了若干文章,现总结如下:
单独一个类的场景下,初始化顺序为依次为 静态数据,继承的基类的构造函数,成员变量,被调用的构造函数。
其中静态数据只会初始化一次。
输出:

稍微修改一下代码,添加两个基类,让Son继承Father, Father继承Grandpa。
继承的情况就比较复杂了。由于继承了基类,还将往上回溯,递归地调用基类的无参构造方法。
在我们的例子中,在初始化静态数据后,会先往上追溯,调用Father的默认构造方法,此时再往上追溯到Grandpa的默认构造方法。
注:如果在子类的构造方法中,显式地调用了父类的带参构造方法,那么JVM将调用指定的构造方法而非默认构造方法。
我们继续修改代码,让其最终呈现如下:
public class App2 {
public static void main(String[] args) {
Son son = new Son();
}
}
class Grandpa {
public Grandpa() {
System.out.println("this is grandpa.");
}
public Grandpa(int age) {
System.out.println("grandpa is " + age + " years old.");
}
private Height height = new Height(1.5f);
public static Gender gender = new Gender(true, "grandpa");
}
class Father extends Grandpa {
public Father() {
System.out.println("this is father.");
}
public Father(int age) {
System.out.println("father is " + age + " years old.");
}
private Height height = new Height(1.6f);
public static Gender gender = new Gender(true, "father");
}
class Son extends Father {
public Son() {
super(50);
System.out.println("this is son.");
}
public Son(int age) {
System.out.println("son is " + age + " years old.");
}
private Height height = new Height(1.8f);
public static Gender gender = new Gender(true, "son");
}
class Height {
public Height(float height) {
System.out.println("initializing height " + height + " meters.");
}
}
class Gender {
public Gender(boolean isMale) {
if (isMale) {
System.out.println("this is a male.");
} else {
System.out.println("this is a female.");
}
}
public Gender(boolean isMale, String identify) {
if (isMale) {
System.out.println(identify + " is a male.");
} else {
System.out.println(identify + " is a female.");
}
}
}
最后输出会是什么呢?
参考下面另一个案例的分析。链接:http://bbs.csdn.net/topics/310164953

在我们的示例中,加载顺序应该是这样的:
Grandpa 静态数据
Father 静态数据
Son 静态数据
Grandpa 成员变量
Grandpa 构造方法
Father 成员变量
Father 构造方法
Son 成员变量
Son 构造方法
所以输出如下:
1. 没有继承的情况
单独一个类的场景下,初始化顺序为依次为 静态数据,继承的基类的构造函数,成员变量,被调用的构造函数。其中静态数据只会初始化一次。
public class App2 { public static void main(String[] args) { Son son = new Son(); } } class Son { public Son() { System.out.println("this is son."); } public Son(int age) { System.out.println("son is " + age + " years old."); } private Height height = new Height(1.8f); public static Gender gender = new Gender(true); } class Height { public Height(float height) { System.out.println("initializing height " + height + " meters."); } } class Gender { public Gender(boolean isMale) { if (isMale) { System.out.println("this is a male."); } else { System.out.println("this is a female."); } } }
输出:

2. 继承的情况
稍微修改一下代码,添加两个基类,让Son继承Father, Father继承Grandpa。继承的情况就比较复杂了。由于继承了基类,还将往上回溯,递归地调用基类的无参构造方法。
在我们的例子中,在初始化静态数据后,会先往上追溯,调用Father的默认构造方法,此时再往上追溯到Grandpa的默认构造方法。
注:如果在子类的构造方法中,显式地调用了父类的带参构造方法,那么JVM将调用指定的构造方法而非默认构造方法。
我们继续修改代码,让其最终呈现如下:
public class App2 {
public static void main(String[] args) {
Son son = new Son();
}
}
class Grandpa {
public Grandpa() {
System.out.println("this is grandpa.");
}
public Grandpa(int age) {
System.out.println("grandpa is " + age + " years old.");
}
private Height height = new Height(1.5f);
public static Gender gender = new Gender(true, "grandpa");
}
class Father extends Grandpa {
public Father() {
System.out.println("this is father.");
}
public Father(int age) {
System.out.println("father is " + age + " years old.");
}
private Height height = new Height(1.6f);
public static Gender gender = new Gender(true, "father");
}
class Son extends Father {
public Son() {
super(50);
System.out.println("this is son.");
}
public Son(int age) {
System.out.println("son is " + age + " years old.");
}
private Height height = new Height(1.8f);
public static Gender gender = new Gender(true, "son");
}
class Height {
public Height(float height) {
System.out.println("initializing height " + height + " meters.");
}
}
class Gender {
public Gender(boolean isMale) {
if (isMale) {
System.out.println("this is a male.");
} else {
System.out.println("this is a female.");
}
}
public Gender(boolean isMale, String identify) {
if (isMale) {
System.out.println(identify + " is a male.");
} else {
System.out.println(identify + " is a female.");
}
}
}
最后输出会是什么呢?
参考下面另一个案例的分析。链接:http://bbs.csdn.net/topics/310164953

在我们的示例中,加载顺序应该是这样的:
Grandpa 静态数据
Father 静态数据
Son 静态数据
Grandpa 成员变量
Grandpa 构造方法
Father 成员变量
Father 构造方法
Son 成员变量
Son 构造方法
所以输出如下:
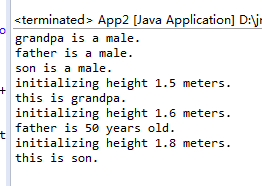
相关文章推荐
- java web的初始化加载顺序,以及servlet的运行过程
- java类的加载以及初始化顺序
- java类的加载以及初始化顺序
- java类的加载以及初始化顺序
- java类的加载以及初始化顺序 .
- java类的加载以及初始化顺序
- java类的加载以及初始化顺序
- java程序在执行过程中,类,对象以及它们成员加载、初始化的顺序如下:
- Java中的Package.Import.Class以及和C++的比较(二)--类成员初始化及顺序
- Java杂谈3——类加载机制与初始化顺序
- 简易Java(03):Java类何时以及如何加载并初始化?
- Java学习总结6——类加载1(类初始化顺序)
- java中类/对象的初始化顺序以及静态代码块的使用
- 关于java文件加载和初始化顺序问题分析
- Java 继承加载顺序 以及 多态中变量和方法
- Java中初始化对象的顺序,静态代码块的用法以及Static的用法详解
- java 初始化顺序以及由此可能引发的构造方法的潜在问题。
- Java中初始化对象的顺序,静态代码块的用法以及Static的用法详解
- java 构造函数 成员函数初始化顺序 以及多态的构造函数的调用顺序
- java 静态初始化,动态初始化,以及构造器执行的顺序