Java23种设计模式案例:适配器模式(adapter)
2017-03-05 00:00
671 查看
属于结构型模式,其主要作用是将一个类的接口转换成客户希望的另外一个接口。适配器模式使得原本由于接口不兼容而不能一起工作的那些类可以一起工作。比如你手机只有2.5mm接口(貌似就Nokia干的出来),但你只能买到3.5mm的,这时就需要买个适配器了。适配器模式宗旨:保留现有类所提供的服务,对调用方提供接口,以满足调用方的期望。
(2)你想创建一个可以复用的类,该类可以与其他不相关的类或不可预见的类(即那些接口可能不一定兼容的类)协同工作。
(3)你想使用一些已经存在的子类,但是不可能对每一个都进行子类化以匹配它们的接口。对象适配器可以适配它的父类接口——仅适用于对象Adapter。
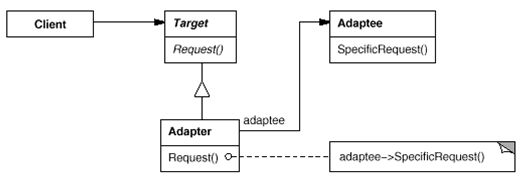
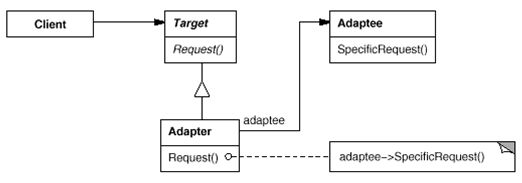
类适配器模式:当希望将一个类转换成满足另一个新接口的类时,可以使用类的适配器模式,创建一个新类,继承原有的类,实现新的接口即可。
对象适配器模式:当希望将一个对象转换成满足另一个新接口的对象时,可以创建一个Wrapper类,持有原类的一个实例,在Wrapper类的方法中,调用实例的方法就行。
1、试用场景
(1)你想使用一个已经存在的类,而它的接口不符合你的需求。(2)你想创建一个可以复用的类,该类可以与其他不相关的类或不可预见的类(即那些接口可能不一定兼容的类)协同工作。
(3)你想使用一些已经存在的子类,但是不可能对每一个都进行子类化以匹配它们的接口。对象适配器可以适配它的父类接口——仅适用于对象Adapter。
2、代码实例
(1)关系图
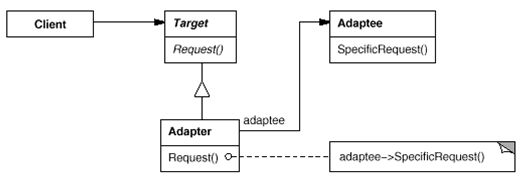
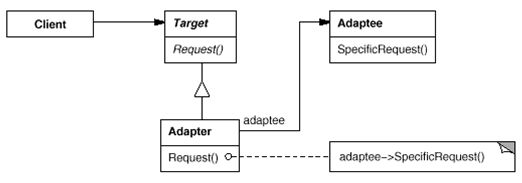
(2)代码实现
接口的适配器模式:当不希望实现一个接口中所有的方法时,可以创建一个抽象类Wrapper,实现所有方法,我们写别的类的时候,继承抽象类即可。package com.design.mode.adapter; /** * A interface */ public interface Shape { public void Draw(); public void Border(); }
public abstract class AbstractShape implements Shape { public void Draw(){} public void Border(){} }
public class Shape1 extends AbstractShape { @Override public void Draw() { System.err.println("drawer-==============="); } }
public class Shape2 extends AbstractShape { @Override public void Border() { System.err.println("border==="); } }
public class TestAbstractShape { public static void main(String[] args) { Shape source1 = new Shape1(); Shape source2 = new Shape2(); source1.Draw(); source1.Border(); source2.Draw(); source2.Border(); } }
类适配器模式:当希望将一个类转换成满足另一个新接口的类时,可以使用类的适配器模式,创建一个新类,继承原有的类,实现新的接口即可。
public class Text { private String content; public Text() { } public void SetContent(String str) { content = str; } public String GetContent() { return content; } }
public class TextShapeClass extends Text implements Shape { public TextShapeClass() { } public void Draw() { System.out.println("Draw a shap ! Impelement Shape interface !"); } public void Border() { System.out.println("Set the border of the shap ! Impelement Shape interface !"); } public static void main(String[] args) { TextShapeClass myTextShapeClass = new TextShapeClass(); myTextShapeClass.Draw(); myTextShapeClass.Border(); myTextShapeClass.SetContent("A test text !"); System.out.println("The content in Text Shape is :" + myTextShapeClass.GetContent()); } }
对象适配器模式:当希望将一个对象转换成满足另一个新接口的对象时,可以创建一个Wrapper类,持有原类的一个实例,在Wrapper类的方法中,调用实例的方法就行。
public class TextShapeObject implements Shape { private Text txt; public TextShapeObject(Text t) { txt = t; } public void Draw() { System.out.println("Draw a shap ! Impelement Shape interface !"); } public void Border() { System.out.println("Set the border of the shap ! Impelement Shape interface !"); } public void SetContent(String str) { txt.SetContent(str); } public String GetContent() { return txt.GetContent(); } public static void main(String[] args) { Text myText = new Text(); TextShapeObject myTextShapeObject = new TextShapeObject(myText); myTextShapeObject.Draw(); myTextShapeObject.Border(); myTextShapeObject.SetContent("A test text !"); System.out.println("The content in Text Shape is :" + myTextShapeObject.GetContent()); } }
相关文章推荐
- java23种常用设计模式之适配器模式(Adapter)
- Java开发中的23种设计模式之六:适配器模式(Adapter)
- Java23种设计模式之适配器模式(Adapter Pattern)
- java23种设计模式之适配器模式(Adapter)
- java23种设计模式--适配器模式(Adapter)
- Java设计模式 - Adapter(适配器模式)
- java设计模式——适配器模式(Adapter)
- Java设计模式之适配器模式(Adapter Pattern)
- 【JAVA】设计模式之适配器模式(Adapter模式)的使用分析
- Java设计模式 结构模式-适配器模式(Adapter)
- java设计模式(7):适配器模式(Adapter)
- JAVA设计模式(06):结构型-适配器模式(Adapter)
- Java经典23种设计模式之结构型模式(三)------附代理模式、适配器模式、外观模式区别
- Java设计模式(9)适配器模式(Adapter模式)
- JAVA设计模式之 适配器模式【Adapter Pattern】
- Java23种设计模式--Adapter(适配器)
- Java设计模式二十:适配器模式(Adapter)
- java_23种设计模式之适配器模式
- 设计模式(六)----- 适配器模式(Adapter)----(JAVA版)
- 23种经典设计模式的java实现_2_适配器模式