温故而知新(2)——C#变量(枚举,结构,数组)
2017-02-13 16:27
369 查看
复杂的变量类型
1.枚举类型
枚举类型的取值范围是用户提供的值的有限集合。 如定义一个包含了周一到周日的week类型。
枚举类型的默认基本类型为int,可根据需求自己更改。 (基本类型为:byte,sbyte,short,ushor,int,uint,long,ulong)

如果要把string类型转换为枚举类型,则用Enum.Parse()方法即可:

2.结构
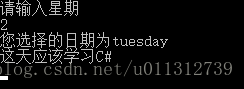
区别:结构可以存储不同的数据类型,而枚举不行。
3.数组
数组是一个变量的索引列表,存储在数组类型的变量中。
数组有一个基本类型,该数组中的所有成员都是这种类型。通常我们以下述2种方式声明数组:
注:[]中的索引值必须为数组中元素的真实个数,否则程序会报错。数组索引从0开始,不是从1!
使用for和foreach都可以循环遍历数组,区别在于,使用for可以修改元素的值,而foreach不可以。
当然,以上介绍的仅仅是最简单的一维数组,还有多维数组(矩形数组)和数组的数组(锯齿数组)。
多维数组的初始化:
本文就变量存储的角度,简要总结了枚举类型,结构,数组在存储变量方面的一些知识要点,在今后的学习和工作中会根据碰到的实际需求继续深入的学习,学到老,活到老。
1.枚举类型
枚举类型的取值范围是用户提供的值的有限集合。 如定义一个包含了周一到周日的week类型。
enum week:byte { monday=1, tuesday=2, wednesday=3, thursday=4, friday=5, saturday=6, sunday=7 }
枚举类型的默认基本类型为int,可根据需求自己更改。 (基本类型为:byte,sbyte,short,ushor,int,uint,long,ulong)
static void Main(string[] args) { week a = week.monday; Console.WriteLine(a.ToString()); Console.WriteLine(Convert.ToInt32(a)); Console.ReadKey(); }
如果要把string类型转换为枚举类型,则用Enum.Parse()方法即可:
static void Main(string[] args) { week a = new week { }; string b = "2"; a = (week)Enum.Parse(typeof(week), b); Console.WriteLine(a.ToString()); Console.WriteLine(Convert.ToInt32(a)); Console.ReadKey(); }
2.结构
enum week:byte { monday=1, tuesday=2, wednesday=3, thursday=4, friday=5, saturday=6, sunday=7 } struct day { public week w; public string events; } class Program { static void Main(string[] args) { day myday; int myweek = 0; do { Console.WriteLine("请输入星期"); myweek = Convert.ToInt32(Console.ReadLine()); } while (myweek < 1 || myweek > 7); string incident = "学习C#"; myday.events = incident; myday.w = (week)myweek; Console.WriteLine("您选择的日期为"+ myday.w); Console.WriteLine("这天应该" + myday.events); Console.ReadKey(); } }
区别:结构可以存储不同的数据类型,而枚举不行。
3.数组
数组是一个变量的索引列表,存储在数组类型的变量中。
数组有一个基本类型,该数组中的所有成员都是这种类型。通常我们以下述2种方式声明数组:
class Program { static void Main(string[] args) { string[] student = {"张三","李四","钱五","王六","周七" }; string[] teacher = new string[2]; teacher[0] = "张老师"; teacher[1] = "宋老师"; } }
注:[]中的索引值必须为数组中元素的真实个数,否则程序会报错。数组索引从0开始,不是从1!
使用for和foreach都可以循环遍历数组,区别在于,使用for可以修改元素的值,而foreach不可以。
当然,以上介绍的仅仅是最简单的一维数组,还有多维数组(矩形数组)和数组的数组(锯齿数组)。
多维数组的初始化:
//二维数组命名 int[,] height={ {1,2 }, { 1,2}, { 2,4} }; int[,] weight = new int[2,2]; weight[0,0] = 1; //三维数组命名 int[,,] a= new int[2,3,3]; int[,,] b = { { { 1, 2 }, { 2, 3 } }, { {2,3 }, {3,4 } } };
本文就变量存储的角度,简要总结了枚举类型,结构,数组在存储变量方面的一些知识要点,在今后的学习和工作中会根据碰到的实际需求继续深入的学习,学到老,活到老。
相关文章推荐
- c#第5章 变量的更多内容 隐式和显式转换、枚举、结构、数组、
- C#变量(枚举,结构,数组)
- C#入门--其他变量(枚举、结构、数组)
- C# 代码示例_结构/数组/枚举...
- C#数组 枚举 结构
- 复杂的变量类型:枚举,结构,数组
- C#学习笔记6——C#中枚举、结构、数组
- C#中的数组,结构,枚举
- C#枚举 枚举和int以及string类型之间的转换 结构 重载 全局变量
- C#学习笔记12:枚举、结构、数组基础学习
- C#枚举类型、枚举类型与其他类型转换、结构类型、数组类型
- 复杂的变量类型——枚举、结构、数组
- C#基础-结构、枚举和数组(Day4)
- 复杂的变量类型---枚举,结构,数组
- 【1】C#基础:数据类型、运算、随机、枚举、结构、数组、列表、文件和文件流、序列化、MD5
- C#学习第四天 常量、枚举、结构、数组
- C#枚举、结构、数组、排序
- c#学习笔记--数组、枚举、结构、值类型和引用类型
- c#之旅--第四天(结构,枚举,数组)
- C# 枚举、结构和数组