Qt实现FTP下载
2017-01-18 15:28
253 查看
简单实现ftp下载上传功能,因为Qt5不再支持QFtp类,需要的话得重现编译.所以遗憾未实现一个目录列表.只能在根目录上传下载.
效果如下:
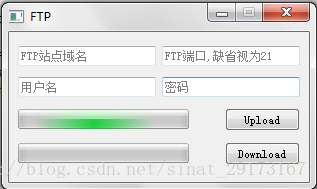
效果如下:
/*---------------ftpmanager.h---------------------*/ #ifndef FTPMANAGER_H #define FTPMANAGER_H #include <QUrl> #include <QFile> #include <QNetworkReply> #include <QNetworkAccessManager> class FtpManager : public QObject { Q_OBJECT public: explicit FtpManager(QObject *parent = 0); // 设置地址和端口 void setHostPort(const QString &host, int port = 21) { m_pUrl.setHost(host); m_pUrl.setPort(port); } // 设置登录 FTP 服务器的用户名和密码 void setUserInfo(const QString &userName, const QString &password); // 上传文件 void put(const QString &fileName, const QString &path); // 下载文件 void get(const QString &path, const QString &fileName); signals: void error(QNetworkReply::NetworkError); // 上传进度 void uploadProgress(qint64 bytesSent, qint64 bytesTotal); // 下载进度 void downloadProgress(qint64 bytesReceived, qint64 bytesTotal); private slots: // 下载过程中写文件 void finished(); private: QUrl m_pUrl; QFile m_file; QNetworkAccessManager m_manager; }; #endif // FTPMANAGER_H /*---------------ftpmanager.h---------------------*/
/*---------------submitdlg.h---------------------*/ #ifndef SUBMITDLG_H #define SUBMITDLG_H #include <QString> #include <QLineEdit> #include <QPushButton> #include <QDialog> class submitDlg : public QDialog { Q_OBJECT public: submitDlg(); QString fileName; private: QLineEdit *getFileName; QPushButton *okButton; private slots: void submitFile(); }; #endif // SUBMITDLG_H /*---------------submitdlg.h---------------------*/
/*---------------mainwindow.h---------------------*/ #ifndef MAINWINDOW_H #define MAINWINDOW_H #include "ftpmanager.h" #include "submitdlg.h" #include <QMainWindow> #include <QWidget> #include <QPushButton> #include <QProgressBar> #include <QLineEdit> #include <QCloseEvent> class MainWindow : public QMainWindow { Q_OBJECT public: MainWindow(QWidget *parent = 0); ~MainWindow(); void closeEvent(QCloseEvent *event); FtpManager m_ftp; private slots: void upload_clicked(); void download_clicked(); void uploadProgress(qint64 bytesSent, qint64 bytesTotal); void downloadProgress(qint64 bytesReceived, qint64 bytesTotal); void error(QNetworkReply::NetworkError error); private: QWidget *widget; QLineEdit *file; QPushButton *pUploadButton; QPushButton *pDownloadButton; QLineEdit *ip; QLineEdit *port; QLineEdit *user; QLineEdit *passwd; QProgressBar *m_pUploadBar; QProgressBar *m_pDownloadBar; void init(bool md); QString getFileName(QString filename); }; #endif // MAINWINDOW_H /*---------------mainwindow.h---------------------*/
/*---------------ftpmanager.cpp---------------------*/ #include <QFileInfo> #include "ftpmanager.h" FtpManager::FtpManager(QObject *parent) : QObject(parent) { // 设置协议 m_pUrl.setScheme("ftp"); } // 设置地址和端口 // inline // 设置登录 FTP 服务器的用户名和密码 void FtpManager::setUserInfo(const QString &userName, const QString &password) { m_pUrl.setUserName(userName); m_pUrl.setPassword(password); } // 上传文件 void FtpManager::put(const QString &fileName, const QString &path) { QFile file(fileName); file.open(QIODevice::ReadOnly); QByteArray data = file.readAll(); m_pUrl.setPath(path); QNetworkReply *pReply = m_manager.put(QNetworkRequest(m_pUrl), data); connect(pReply, SIGNAL(uploadProgress(qint64, qint64)), this, SIGNAL(uploadProgress(qint64, qint64))); connect(pReply, SIGNAL(error(QNetworkReply::NetworkError)), this, SIGNAL(error(QNetworkReply::NetworkError))); } // 下载文件 void FtpManager::get(const QString &path, const QString &fileName) { QFileInfo info; info.setFile(fileName); m_file.setFileName(fileName); m_file.open(QIODevice::WriteOnly | QIODevice::Append); m_pUrl.setPath(path); QNetworkReply *pReply = m_manager.get(QNetworkRequest(m_pUrl)); connect(pReply, SIGNAL(finished()), this, SLOT(finished())); connect(pReply, SIGNAL(downloadProgress(qint64, qint64)), this, SIGNAL(downloadProgress(qint64, qint64))); connect(pReply, SIGNAL(error(QNetworkReply::NetworkError)), this, SIGNAL(error(QNetworkReply::NetworkError))); } // 下载过程中写文件 void FtpManager::finished() { QNetworkReply *pReply = qobject_cast<QNetworkReply *>(sender()); switch (pReply->error()) { case QNetworkReply::NoError : { m_file.write(pReply->readAll()); m_file.flush(); } break; default: break; } m_file.close(); pReply->deleteLater(); } /*---------------ftpmanager.cpp---------------------*/
/*---------------submitdlg.cpp---------------------*/ #include "submitdlg.h" #include <QGridLayout> submitDlg::submitDlg() { getFileName = new QLineEdit(); getFileName->setPlaceholderText(tr("输入要下载的文件名")); okButton = new QPushButton(tr("确定"),this); QGridLayout *layout = new QGridLayout(this); layout->addWidget(getFileName, 0, 0); layout->addWidget(okButton, 0, 1); this->setLayout(layout); this->setWindowTitle(tr("请求下载文件名")); connect(okButton, SIGNAL(clicked()), this, SLOT(submitFile())); } void submitDlg::submitFile() { fileName = getFileName->text(); this->hide(); } /*---------------submitdlg.cpp---------------------*/
/*---------------mainwindow.cpp---------------------*/ #include "mainwindow.h" #include <QHBoxLayout> #include <QMessageBox> #include <QFileDialog> MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent) { widget = new QWidget(); this->setFixedSize(300,150); this->setCentralWidget(widget); this->setWindowTitle(tr("FTP")); ip = new QLineEdit(); ip->setPlaceholderText(tr("FTP站点域名")); port = new QLineEdit(); port->setPlaceholderText(tr("FTP端口,缺省视为21")); user = new QLineEdit; user->setPlaceholderText(tr("用户名")); passwd = new QLineEdit; passwd->setPlaceholderText(tr("密码")); passwd->setEchoMode(QLineEdit::Password); pUploadButton= new QPushButton; pUploadButton->setText("Upload"); pDownloadButton = new QPushButton; pDownloadButton->setText("Download"); m_pUploadBar = new QProgressBar(this); m_pDownloadBar = new QProgressBar(this); QHBoxLayout *hLayout0 = new QHBoxLayout(); hLayout0->addWidget(ip); hLayout0->addWidget(port); QHBoxLayout *hLayout1 = new QHBoxLayout(); hLayout1->addWidget(user); hLayout1->addWidget(passwd); QHBoxLayout *hLayout2 = new QHBoxLayout(); hLayout2->addWidget(m_pUploadBar); hLayout2->addWidget(pUploadButton); QHBoxLayout *hLayout3 = new QHBoxLayout(); hLayout3->addWidget(m_pDownloadBar); hLayout3->addWidget(pDownloadButton); QVBoxLayout *vLayout = new QVBoxLayout(widget); vLayout->addLayout(hLayout0); vLayout->addLayout(hLayout1); vLayout->addLayout(hLayout2); vLayout->addLayout(hLayout3); widget->setLayout(vLayout); connect(pUploadButton, SIGNAL(clicked(bool)), this, SLOT(upload_clicked())); connect(pDownloadButton, SIGNAL(clicked(bool)), this, SLOT(download_clicked())); } void MainWindow::upload_clicked() { init(true); QString filepath; QString dev; filepath = QFileDialog::getOpenFileName(this, tr("选择上传文件"), "C:\\Users\\Administrator\\Desktop"); dev = getFileName(filepath); QMessageBox::information(this,"", "准备上传文件: " + filepath); m_ftp.put(filepath, dev); connect(&m_ftp, SIGNAL(error(QNetworkReply::NetworkError)), this, SLOT(error(QNetworkReply::NetworkError))); connect(&m_ftp, SIGNAL(uploadProgress(qint64, qint64)), this, SLOT(uploadProgress(qint64, qint64))); } void MainWindow::download_clicked() { init(true); QString filepath; QString submitFile; submitDlg dlg; dlg.exec(); submitFile = dlg.fileName; filepath = QFileDialog::getSaveFileName(this, tr("保存文件位置"), "C:\\Users\\Administrator\\Desktop"); m_ftp.get(submitFile, filepath); connect(&m_ftp, SIGNAL(error(QNetworkReply::NetworkError)), this, SLOT(error(QNetworkReply::NetworkError))); connect(&m_ftp, SIGNAL(downloadProgress(qint64, qint64)), this, SLOT(downloadProgress(qint64, qint64))); } void MainWindow::init(bool mode) { if(mode == true) { m_ftp.setHostPort(ip->text(),port->text().toInt()); m_ftp.setUserInfo(user->text(), passwd->text()); ip->setEnabled(false); port->setEnabled(false); user->setEnabled(false); passwd->setEnabled(false); pUploadButton->setEnabled(false); pDownloadButton->setEnabled(false); } if(mode == false) { ip->setEnabled(true); port->setEnabled(true); user->setEnabled(true); passwd->setEnabled(true); pUploadButton->setEnabled(true); pDownloadButton->setEnabled(true); } } QString MainWindow::getFileName(QString filename) { char *tmpPath = (char *)filename.toStdString().data(); for(int i = filename.length(); i > 0; --i) { if(tmpPath[i-1] == '/') { tmpPath += i; break; } } filename.sprintf("%s",tmpPath); return filename; } // 更新上传进度 void MainWindow::uploadProgress(qint64 bytesSent, qint64 bytesTotal) { m_pUploadBar->setMaximum(bytesTotal); m_pUploadBar->setValue(bytesSent); init(false); } // 更新下载进度 void MainWindow::downloadProgress(qint64 bytesReceived, qint64 bytesTotal) { m_pDownloadBar->setMaximum(bytesTotal); m_pDownloadBar->setValue(bytesReceived); init(false); } // 错误处理 void MainWindow::error(QNetworkReply::NetworkError error) { switch (error) { case QNetworkReply::HostNotFoundError : qDebug() << QString::fromLocal8Bit("主机没有找到"); break; // 其他错误处理 default: break; } } MainWindow::~MainWindow() { } void MainWindow::closeEvent(QCloseEvent *event) { QMessageBox::StandardButton button = QMessageBox::question(this, tr("退出程序"),QString(tr("是否退出")), QMessageBox::Yes|QMessageBox::No,QMessageBox::No); if(button == QMessageBox::Yes) { event->accept(); } else { event->ignore(); } } /*---------------mainwindow.cpp---------------------*/
/*---------------main.cpp---------------------*/ #include "mainwindow.h" #include <QApplication> int main(int argc, char *argv[]) { QApplication a(argc, argv); MainWindow w; w.show(); return a.exec(); } /*---------------main.cpp---------------------*/
相关文章推荐
- QT学习 第一章:基本对话框
- 使用Shiboken为C++和Qt库创建Python绑定
- 配置ftp服务
- 用vsftp快速搭建ftp服务器
- CentOS7安装配置vsftp搭建FTP
- Qt 5.6更新至RC版,最终版本近在咫尺
- linux中 qt5 的环境搭建
- 实现FTP整站上传的批处理代码
- 批处理向FTP上传具有指定属性的文件(增量备份)
- 使用 iisftpdr.vbs 创建 FTP 虚拟目录的方法
- Delphi解析FTP地址的方法
- IIS里FTP多用户设置方法,终于不用Serv-U了
- FTP 服务器关于权限的问题
- IIS FTP PASV模式下更改端口范围的方法
- FTP 常用命令 使用说明
- 通过批处理修改FTP账号和密码
- FTP下载服务器 TYPSoft FTP Server V1.11 简体中文版 下载
- FTP 分类账户设置经验谈
- php实现curl模拟ftp上传的方法
- 批处理bat下载FTP服务器上某个目录下的文件