iOS 自定义下拉选项框 —— HERO博客
2017-01-07 15:31
323 查看
之前两篇简述了UISearchbar的属性、方法,以及UISearchbar的简单使用。本篇在此基础上简单封装了一个下拉选项框。
先看一下效果图:
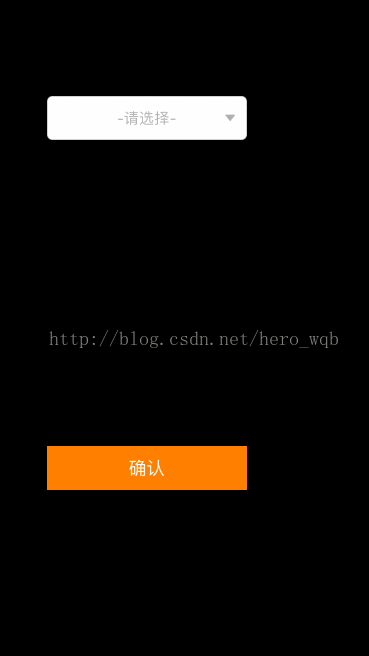
封装的HWOptionButton属性:
@property (nonatomic,strong)NSArray
*array; //需要显示的选项数组
@property (nonatomic,copy,readonly)NSString
*title; //选择的标题
@property (nonatomic,assign,readonly)NSInteger
row; //选择的行数
@property (nonatomic,assign)BOOL
showPlaceholder; //是否显示提示文字,默认为显示
@property (nonatomic,assign)BOOL
showSearchBar; //是否显示搜索条,默认为不显示
下面贴上代码:
ViewController:
HWOptionButton:
写博客是希望大家共同交流成长,博主水平有限难免有偏颇不足之处,欢迎批评指正。
先看一下效果图:
封装的HWOptionButton属性:
@property (nonatomic,strong)NSArray
*array; //需要显示的选项数组
@property (nonatomic,copy,readonly)NSString
*title; //选择的标题
@property (nonatomic,assign,readonly)NSInteger
row; //选择的行数
@property (nonatomic,assign)BOOL
showPlaceholder; //是否显示提示文字,默认为显示
@property (nonatomic,assign)BOOL
showSearchBar; //是否显示搜索条,默认为不显示
下面贴上代码:
ViewController:
#import <UIKit/UIKit.h> @interface ViewController : UIViewController @end /*** ---------------分割线--------------- ***/ #import "ViewController.h" #import "HWOptionButton.h" @interface ViewController ()<HWOptionButtonDelegate> @property (nonatomic, weak) HWOptionButton *optionButton; @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; self.view.backgroundColor = [UIColor blackColor]; [self creatControl]; } - (void)creatControl { HWOptionButton *optionBtn = [[HWOptionButton alloc] initWithFrame:CGRectMake(50, 100, 200, 44)]; optionBtn.array = @[@"mac", @"kobe", @"tracy", @"allen", @"ios", @"android", @"swift", @"object", @"activity"]; optionBtn.delegate = self; optionBtn.showSearchBar = YES; // optionBtn.showPlaceholder = NO; [self.view addSubview:optionBtn]; self.optionButton = optionBtn; UIButton *sureBtn = [[UIButton alloc] initWithFrame:CGRectMake(50, 450, 200, 44)]; [sureBtn setTitle:@"确认" forState:UIControlStateNormal]; sureBtn.backgroundColor = [UIColor orangeColor]; [sureBtn addTarget:self action:@selector(sureBtnOnClick) forControlEvents:UIControlEventTouchUpInside]; [self.view addSubview:sureBtn]; } - (void)sureBtnOnClick { if ([_optionButton.title isEqualToString:@"-请选择-"]) { NSLog(@"未选择"); return; } NSLog(@"选择了第%ld行,标题为%@", _optionButton.row, _optionButton.title); } #pragma mark - HWOptionButtonDelegate - (void)didSelectOptionInHWOptionButton:(HWOptionButton *)optionButton { //do something... } @end
HWOptionButton:
#import <UIKit/UIKit.h> @class HWOptionButton; @protocol HWOptionButtonDelegate <NSObject> //确认选项后,如有其它特殊操作,用此代理事件 - (void)didSelectOptionInHWOptionButton:(HWOptionButton *)optionButton; @end @interface HWOptionButton : UIView @property (nonatomic, strong) NSArray *array; @property (nonatomic, copy, readonly) NSString *title; @property (nonatomic, assign, readonly) NSInteger row; @property (nonatomic, assign) BOOL showPlaceholder; //default is YES. @property (nonatomic, assign) BOOL showSearchBar; //default is NO. @property (nonatomic, weak) id<HWOptionButtonDelegate> delegate; @end /*** ---------------分割线--------------- ***/ #import "HWOptionButton.h" #define KMainW [UIScreen mainScreen].bounds.size.width #define KMainH [UIScreen mainScreen].bounds.size.height #define KMarginYWhenMoving 20.0f #define KRowHeight 44.0f #define KMaxShowLine 6 #define KFont [UIFont systemFontOfSize:15.0f] #define KBackColor [UIColor whiteColor] @interface HWOptionButton ()<UITableViewDelegate, UITableViewDataSource, UISearchBarDelegate> @property (nonatomic, strong) NSArray *searchArray; @property (nonatomic, strong) UIWindow *cover; @property (nonatomic, strong) UITableView *tableView; @property (nonatomic, strong) UISearchBar *searchBar; @property (nonatomic, weak) UIView *view; @property (nonatomic, weak) UIButton *button; @property (nonatomic, copy, readwrite) NSString *title; @property (nonatomic, assign, readwrite) NSInteger row; @end @implementation HWOptionButton static NSString *KOptionButtonCell = @"KOptionButtonCell"; - (instancetype)initWithFrame:(CGRect)frame { if (self = [super initWithFrame:frame]) { [self setup]; } return self; } - (instancetype)initWithCoder:(NSCoder *)coder { if (self = [super initWithCoder:coder]) { [self setup]; } return self; } - (void)setup { self.title = @"-请选择-"; UIButton *button = [[UIButton alloc] initWithFrame:self.bounds]; [button setTitleColor:[UIColor colorWithRed:180/255.0 green:180/255.0 blue:180/255.0 alpha:1.0f] forState:UIControlStateNormal]; [button setTitleColor:[UIColor blackColor] forState:UIControlStateSelected]; [button setTitle:_title forState:UIControlStateNormal]; button.titleLabel.font = KFont; [button setBackgroundImage:[UIImage imageNamed:@"optionBtn_nor"] forState:UIControlStateNormal]; [button addTarget:self action:@selector(buttonAction:) forControlEvents:UIControlEventTouchUpInside]; [self addSubview:button]; self.button = button; //搜索框 _searchBar = [[UISearchBar alloc] init]; _searchBar.barTintColor = KBackColor; _searchBar.layer.borderWidth = 1.0f; _searchBar.layer.borderColor = [[UIColor blackColor] CGColor]; _searchBar.delegate = self; _searchBar.keyboardType = UIKeyboardTypeASCIICapable; //选项视图 _tableView = [[UITableView alloc] init]; _tableView.rowHeight = KRowHeight; _tableView.separatorStyle = UITableViewCellSeparatorStyleNone; _tableView.layer.borderWidth = 1.0f; _tableView.layer.borderColor = [[UIColor blackColor] CGColor]; _tableView.dataSource = self; _tableView.delegate = self; self.showPlaceholder = YES; self.showSearchBar = NO; } - (void)buttonAction:(UIButton *)button { [self creatControl]; [self endEditing]; } - (void)creatControl { //遮盖window _cover = [[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]]; _cover.windowLevel = UIWindowLevelAlert; _cover.hidden = NO; //window视图 UIView *view = [[UIView alloc] initWithFrame:[[UIScreen mainScreen] bounds]]; [_cover addSubview:view]; self.view = view; //遮盖视图 UIView *backview = [[UIView alloc] initWithFrame:[[UIScreen mainScreen] bounds]]; backview.backgroundColor = [UIColor colorWithRed:(0)/255.0 green:(0)/255.0 blue:(0)/255.0 alpha:0.0f]; [backview addGestureRecognizer:[[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(Tap:)]]; [self.view addSubview:backview]; //坐标转换 CGRect frame = [self.superview convertRect:self.frame toView:self.view]; //显示选项按钮 UIButton *button = [[UIButton alloc] initWithFrame:CGRectMake(frame.origin.x, frame.origin.y, self.frame.size.width, self.frame.size c809 .height)]; button.titleLabel.font = KFont; [button setTitle:_button.titleLabel.text forState:UIControlStateNormal]; [button setTitleColor:[UIColor blackColor] forState:UIControlStateNormal]; [button addTarget:self action:@selector(btnOnClick) forControlEvents:UIControlEventTouchUpInside]; [button setBackgroundImage:[UIImage imageNamed:@"optionBtn_sel"] forState:UIControlStateNormal]; [self.view addSubview:button]; //搜索框 if (_showSearchBar) { _searchBar.frame = CGRectMake(frame.origin.x, CGRectGetMaxY(frame), frame.size.width, KRowHeight); [self.view addSubview:_searchBar]; } //设置tableviewFrame NSInteger rowCount = _showSearchBar ? KMaxShowLine - 1 : KMaxShowLine; CGFloat tabelViewY = _showSearchBar ? CGRectGetMaxY(_searchBar.frame) : CGRectGetMaxY(frame); if (_array.count <= rowCount) { _tableView.frame = CGRectMake(frame.origin.x, tabelViewY, frame.size.width, _array.count * KRowHeight); }else { _tableView.frame = CGRectMake(frame.origin.x, tabelViewY, frame.size.width, rowCount * KRowHeight); } [self.view addSubview:_tableView]; } - (void)endEditing { [[[self findViewController] view] endEditing:YES]; } - (UIViewController *)findViewController { id target = self; while (target) { target = ((UIResponder *)target).nextResponder; if ([target isKindOfClass:[UIViewController class]]) { break; } } return target; } - (void)setArray:(NSArray *)array { _array = array; self.searchArray = [_array copy]; [self setInfo]; } - (void)setShowPlaceholder:(BOOL)showPlaceholder { _showPlaceholder = showPlaceholder; [self setInfo]; } - (void)setInfo { if (!_showPlaceholder && _array.count > 0) { [_button setSelected:YES]; _title = _array[0]; [_button setTitle:_title forState:UIControlStateNormal]; } [_tableView reloadData]; } - (void)btnOnClick { [self dismissOptionAlert]; } - (void)Tap:(UITapGestureRecognizer *)recognizer { [self dismissOptionAlert]; } - (void)dismissOptionAlert { [_searchBar resignFirstResponder]; if (self.view.frame.origin.y == 0) { [self removeCover]; }else { [self searchBarTextDidEndEditing:_searchBar]; } } - (void)removeCover { [_searchBar resignFirstResponder]; _cover.hidden = YES; _cover = nil; } #pragma mark - UISearchBarDelegate - (void)searchBar:(UISearchBar *)searchBar textDidChange:(NSString *)searchText { if (searchText.length > 0) { NSPredicate *predicate = [NSPredicate predicateWithFormat:@"SELF CONTAINS[c] %@", searchText]; _searchArray = [[_array filteredArrayUsingPredicate:predicate] copy]; }else { _searchArray = [_array copy]; } [_tableView reloadData]; } - (BOOL)searchBarShouldBeginEditing:(UISearchBar *)searchBar { UIView *view = self.superview; while (view.superview) { view = view.superview; } CGFloat Y = KMarginYWhenMoving - [self.superview convertRect:self.frame toView:self.view].origin.y; [UIView animateWithDuration:0.22f animations:^{ view.frame = CGRectMake(0, Y, KMainW, KMainH); self.view.frame = CGRectMake(0, Y, KMainW, KMainH); }]; return YES; } - (void)searchBarTextDidEndEditing:(UISearchBar *)searchBar { UIView *view = self.superview; while (view.superview) { view = view.superview; } [UIView animateWithDuration:0.22f animations:^{ view.frame = CGRectMake(0, 0, KMainW, KMainH); self.view.frame = CGRectMake(0, 0, KMainW, KMainH); }completion:^(BOOL finished) { [self removeCover]; }]; } #pragma mark - tableViewDelegate - (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section { return _searchArray.count; } - (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath { UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:KOptionButtonCell]; if (!cell) { cell = [[UITableViewCell alloc] initWithStyle:UITableViewCellStyleValue1 reuseIdentifier:KOptionButtonCell]; } cell.textLabel.text = _searchArray[indexPath.row]; cell.backgroundColor = [UIColor whiteColor]; cell.textLabel.font = KFont; return cell; } - (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath { _row = indexPath.row; [_button setSelected:YES]; self.title = _searchArray[_row]; [self.button setTitle:self.title forState:UIControlStateNormal]; [self dismissOptionAlert]; if (_delegate && [_delegate respondsToSelector:@selector(didSelectOptionInHWOptionButton:)]) { [_delegate didSelectOptionInHWOptionButton:self]; } } @end
写博客是希望大家共同交流成长,博主水平有限难免有偏颇不足之处,欢迎批评指正。
相关文章推荐
- iOS 自定义UIPickerView地区选择器视图 —— HERO博客
- iOS 自定义水平滚动条、进度条 —— HERO博客
- iOS 自定义UIPickerView天数选择器视图 —— HERO博客
- iOS 自定义键盘 —— HERO博客
- iOS 自定义UIDatePicker日期选择器视图 —— HERO博客
- iOS 封装下拉、上拉刷新控件 —— HERO博客
- iOS 自定义日历 —— HERO博客
- iOS 自定义UIButton点击动画特效 —— HERO博客
- iOS 自定义滚动条,可展示交互 —— HERO博客
- iOS 绘图机制简介,Quartz 2D绘图用CGContextRef绘制音频波形图 —— HERO博客
- iOS Touch ID指纹识别技术简介 —— HERO博客
- iOS Quartz 2D绘图用CGContextRef绘制三角形 —— HERO博客
- iOS MVC设计模式与MVVM设计模式简介 —— HERO博客
- iOS AFN监听网络,封装网络请求 —— HERO博客
- iOS MD5加密 —— HERO博客
- ios自定义下拉列表
- 自定义控件之------仿ios下拉回弹效果
- IOS博客项目搭建-06-自定义TabBarButton-03
- iOS UIView类扩展,直接访问属性 —— HERO博客
- iOS 十六进制颜色字符串转为UIColor —— HERO博客