学习笔记之Spring入门HelloWorld
2016-12-08 16:49
393 查看
Spring 是一个开源框架.
Spring 为简化企业级应用开发而生. 使用 Spring 可以使简单的 JavaBean 实现以前只有 EJB 才能实现的功能.
Spring 是一个 IOC(DI) 和 AOP 容器框架.
具体描述 Spring:
轻量级:Spring 是非侵入性的 - 基于 Spring 开发的应用中的对象可以不依赖于 Spring 的 API
依赖注入(DI --- dependency injection、IOC)
面向切面编程(AOP --- aspect oriented programming)
容器: Spring 是一个容器, 因为它包含并且管理应用对象的生命周期
框架: Spring 实现了使用简单的组件配置组合成一个复杂的应用. 在 Spring 中可以使用 XML 和 Java 注解组合这些对象
一站式:在 IOC 和 AOP 的基础上可以整合各种企业应用的开源框架和优秀的第三方类库 (实际上 Spring 自身也提供了展现层的 SpringMVC 和 持久层的 Spring JDBC)
Spring模块:
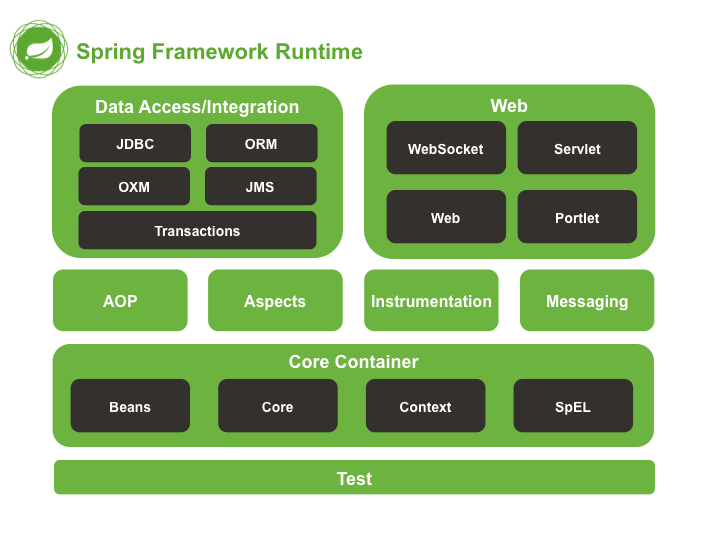
搭建Spring开发环境
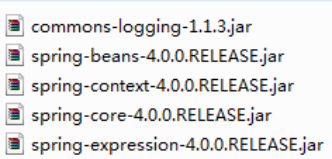
Spring 的配置文件: 一个典型的 Spring 项目需要创建一个或多个 Bean 配置文件, 这些配置文件用于在 Spring IOC 容器里配置 Bean. Bean 的配置文件可以放在 classpath 下, 也可以放在其它目录下.
HelloWolrd类
配置文件applicationContext.xml:
测试类:
运行结果:
HelloWorld's constructor...
setUser:Spring
Hello: Spring
扩展:
如果是这样
运行结果:
HelloWorld's constructor...
setUser:Spring
我们可以得出这样一个结论:创建 Spring 的 IOC 容器 时,完成了对 Bean 的初始化。
Spring 为简化企业级应用开发而生. 使用 Spring 可以使简单的 JavaBean 实现以前只有 EJB 才能实现的功能.
Spring 是一个 IOC(DI) 和 AOP 容器框架.
具体描述 Spring:
轻量级:Spring 是非侵入性的 - 基于 Spring 开发的应用中的对象可以不依赖于 Spring 的 API
依赖注入(DI --- dependency injection、IOC)
面向切面编程(AOP --- aspect oriented programming)
容器: Spring 是一个容器, 因为它包含并且管理应用对象的生命周期
框架: Spring 实现了使用简单的组件配置组合成一个复杂的应用. 在 Spring 中可以使用 XML 和 Java 注解组合这些对象
一站式:在 IOC 和 AOP 的基础上可以整合各种企业应用的开源框架和优秀的第三方类库 (实际上 Spring 自身也提供了展现层的 SpringMVC 和 持久层的 Spring JDBC)
Spring模块:
搭建Spring开发环境
Spring 的配置文件: 一个典型的 Spring 项目需要创建一个或多个 Bean 配置文件, 这些配置文件用于在 Spring IOC 容器里配置 Bean. Bean 的配置文件可以放在 classpath 下, 也可以放在其它目录下.
HelloWolrd类
package com.atguigu.spring.helloworld; public class HelloWorld { private String name; public HelloWorld() { System.out.println("HelloWorld's constructor..."); } public void setName(String name) { System.out.println("setUser:" + name); this.name = name; } public void hello(){ System.out.println("Hello: " + name); } }
配置文件applicationContext.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="helloWorld" class="com.atguigu.spring.helloworld.HelloWorld"> <property name="name" value="Spring"></property> </bean> </beans>
测试类:
package com.atguigu.spring.helloworld; import org.springframework.context.support.ClassPathXmlApplicationContext; public class Main { public static void main(String[] args) { // HelloWorld helloWorld = new HelloWorld(); // helloWorld.setName("Spring"); // helloWorld.hello(); //1. 创建 Spring 的 IOC 容器 ClassPathXmlApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml"); //2. 从 IOC 容器中获取 bean 的实例 HelloWorld helloWorld = (HelloWorld) ctx.getBean("helloWorld"); //3. 使用 hello 方法 helloWorld.hello(); } }
运行结果:
HelloWorld's constructor...
setUser:Spring
Hello: Spring
扩展:
如果是这样
package com.atguigu.spring.helloworld; import org.springframework.context.support.ClassPathXmlApplicationContext; public class Main { public static void main(String[] args) { //1. 创建 Spring 的 IOC 容器 ClassPathXmlApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml"); //2. 从 IOC 容器中获取 bean 的实例 // HelloWorld helloWorld = (HelloWorld) ctx.getBean("helloWorld"); //3. 使用 hello 方法 // helloWorld.hello(); } }
运行结果:
HelloWorld's constructor...
setUser:Spring
我们可以得出这样一个结论:创建 Spring 的 IOC 容器 时,完成了对 Bean 的初始化。
相关文章推荐
- Spring4 学习笔记(1)-入门及 HelloWorld -(供自己学习)
- 1. 笔记JAVA框架学习——Spring入门环境搭建及helloworld
- Spring4 学习笔记(1)-入门及 HelloWorld
- Spring学习笔记1--简介及入门例子
- Spring学习笔记 AOP的HelloWorld
- spring入门篇-学习笔记
- Spring入门-学习笔记
- Spring学习笔记:第二章 Spring中IoC的入门实例
- 【原】Spring.NET 学习笔记1 入门
- Spring学习笔记:2-Spring中IoC的入门实例
- 【Spring】Spring学习笔记-01-入门级实例
- java 从零开始,学习笔记之基础入门<Struts2_Spring_整合>(四十一)
- Spring学习笔记-springMVC入门Demo
- 别人的spring学习入门笔记
- Spring学习笔记----01. 入门知识,IoC/DI
- Spring笔记之一 -- 简单入门讲解HelloWorld
- ejb 学习笔记--HelloWorld入门实例
- spring学习笔记 aop入门
- mybatis-spring 学习笔记之简介与入门
- Spring学习笔记:Spring中IoC的入门实例