文件AES加密、解密
2016-12-07 14:00
423 查看
对文件加密、解密。支持各种格式的文件
先看效果
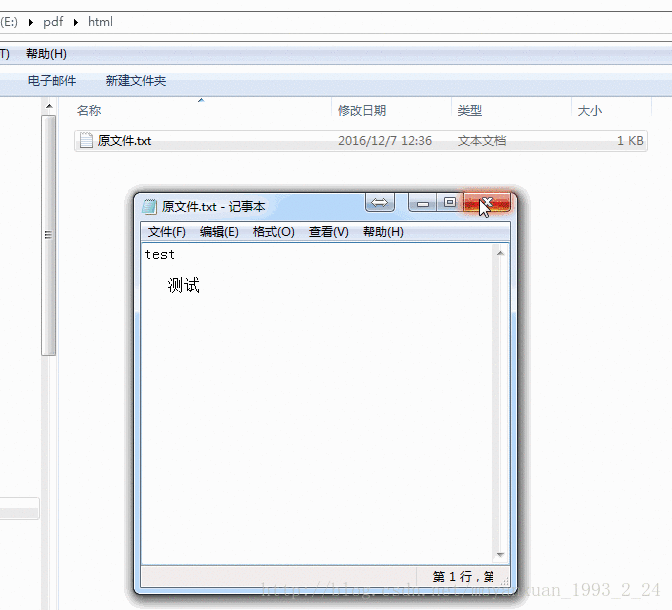
**可以看到加密之后内容为一串类似于乱码之类的
解密之后又恢复为原内容**
直接上代码:
注意:如果出现以下问题
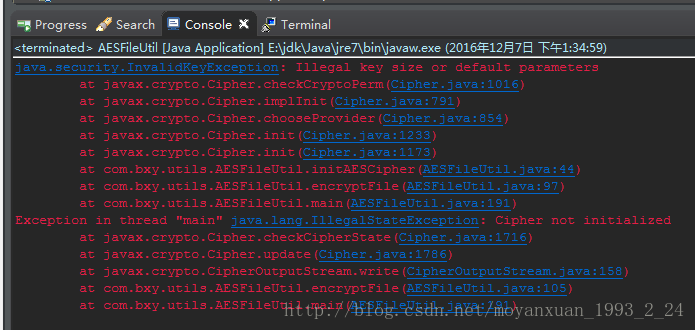
原因: Oracle在其官方网站上提供了无政策限制权限文件(Unlimited Strength Jurisdiction Policy Files),我们只需要将其部署在JRE环境中,就可以解决限制问题。
解决办法:
jdk6无政策限制权限文件下载
jdk7无政策限制权限文件下载
jdk8无政策限制权限文件下载
把下载好的文件解压出来,放到 java\jdk\jre\lib\security下
一个文件是:local_policy.jar
另一个是:US_export_policy.jar
OK!
先看效果
**可以看到加密之后内容为一串类似于乱码之类的
解密之后又恢复为原内容**
直接上代码:
import java.io.BufferedInputStream; import java.io.BufferedOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.UnsupportedEncodingException; import java.security.InvalidKeyException; import java.security.NoSuchAlgorithmException; import java.security.Security; import java.util.Arrays; import javax.crypto.Cipher; import javax.crypto.CipherInputStream; import javax.crypto.CipherOutputStream; import javax.crypto.NoSuchPaddingException; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; /** * @author ZSL * @since 2016年12月7日上午10:18:27 * @desc [文件加密] */ public class AESFileUtil { private static final String key = "password"; /** * init AES Cipher * @param passsword * @param cipherMode * @return */ public static Cipher initAESCipher(String passsword, int cipherMode) { Cipher cipher = null; try { SecretKey key = getKey(passsword); cipher = Cipher.getInstance("AES/ECB/PKCS7Padding"); cipher.init(cipherMode, key); } catch (NoSuchAlgorithmException e) { e.printStackTrace(); //To change body of catch statement use File | Settings | File Templates. } catch (NoSuchPaddingException e) { e.printStackTrace(); //To change body of catch statement use File | Settings | File Templates. } catch (InvalidKeyException e) { e.printStackTrace(); //To change body of catch statement use File | Settings | File Templates. } return cipher; } private static SecretKey getKey(String password) { int keyLength = 256; byte[] keyBytes = new byte[keyLength / 8]; SecretKeySpec key = null; try { Arrays.fill(keyBytes, (byte) 0x0); Security.addProvider(new org.bouncycastle.jce.provider.BouncyCastleProvider()); byte[] passwordBytes = password.getBytes("UTF-8"); int length = passwordBytes.length < keyBytes.length ? passwordBytes.length : keyBytes.length; System.arraycopy(passwordBytes, 0, keyBytes, 0, length); key = new SecretKeySpec(keyBytes, "AES"); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } return key; } /** * AES 加密 * @param encryptPath * @param decryptPath * @param sKey * @return */ public static boolean encryptFile(String encryptPath, String decryptPath, String sKey){ File encryptFile = null; File decryptfile = null; CipherOutputStream cipherOutputStream = null; BufferedInputStream bufferedInputStream = null; try { encryptFile = new File(encryptPath); if(!encryptFile.exists()) { throw new NullPointerException("Encrypt file is empty"); } decryptfile = new File(decryptPath); if(decryptfile.exists()) { decryptfile.delete(); } decryptfile.createNewFile(); Cipher cipher = initAESCipher(sKey, Cipher.ENCRYPT_MODE); cipherOutputStream = new CipherOutputStream(new FileOutputStream(decryptfile), cipher); bufferedInputStream = new BufferedInputStream(new FileInputStream(encryptFile)); byte[] buffer = new byte[1024]; int bufferLength; while ((bufferLength = bufferedInputStream.read(buffer)) != -1) { cipherOutputStream.write(buffer, 0, bufferLength); } bufferedInputStream.close(); cipherOutputStream.close(); // delFile(encryptPath); } catch (IOException e) { delFile(decryptfile.getAbsolutePath()); e.printStackTrace(); //To change body of catch statement use File | Settings | File Templates. return false; } return true; } /** * AES 解密 * @param encryptPath * @param decryptPath * @param mKey * @return */ public static boolean decryptFile(String encryptPath, String decryptPath, String mKey){ File encryptFile = null; File decryptFile = null; BufferedOutputStream outputStream = null; CipherInputStream inputStream = null; try { encryptFile = new File(encryptPath); if(!encryptFile.exists()) { throw new NullPointerException("Decrypt file is empty"); } decryptFile = new File(decryptPath); if(decryptFile.exists()) { decryptFile.delete(); } decryptFile.createNewFile(); Cipher cipher = initAESCipher(mKey, Cipher.DECRYPT_MODE); outputStream = new BufferedOutputStream(new FileOutputStream(decryptFile)); inputStream = new CipherInputStream(new FileInputStream(encryptFile), cipher); int bufferLength; byte[] buffer = new byte[1024]; while ((bufferLength = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, bufferLength); } inputStream.close(); outputStream.close(); // delFile(encryptPath); } catch (IOException e) { delFile(decryptFile.getAbsolutePath()); e.printStackTrace(); //To change body of catch statement use File | Settings | File Templates. return false; } return true; } /** * delete File * @param pathFile * @return */ public static boolean delFile(String pathFile) { boolean flag = false; if(pathFile == null && pathFile.length() <= 0) { throw new NullPointerException("文件不能为空"); }else { File file = new File(pathFile); // 路径为文件且不为空则进行删除 if (file.isFile() && file.exists()) { file.delete(); flag = true; } } return flag; } public static void main(String[] args) { boolean flag = AESFileUtil.encryptFile ("E:/pdf/html/原文件.txt", "E:/pdf/html/加密后.txt", key); System.out.println(flag); flag = AESFileUtil.decryptFile ( "E:/pdf/html/加密后.txt","E:/pdf/html/解密后.txt", key); System.out.println(flag); } }
注意:如果出现以下问题
原因: Oracle在其官方网站上提供了无政策限制权限文件(Unlimited Strength Jurisdiction Policy Files),我们只需要将其部署在JRE环境中,就可以解决限制问题。
解决办法:
jdk6无政策限制权限文件下载
jdk7无政策限制权限文件下载
jdk8无政策限制权限文件下载
把下载好的文件解压出来,放到 java\jdk\jre\lib\security下
一个文件是:local_policy.jar
另一个是:US_export_policy.jar
OK!
相关文章推荐
- 对称加密算法AES------使用AES算法对文件进行加密/解密的操作(JAVA)
- AES加密,解密 C# .net 解密支持大文件
- 在iOS中使用AES进行媒体文件的加密与解密
- android使用AES加密和解密文件实例代码
- 使用对称加密aes对文件进行zip加密解密
- android开发 文件数据的AES-128方式加密解密
- Unity 3D 文件加密下 AES的加密解密使用
- base64编码和aes加密和解密配置文件
- Android AES 文件加密解密
- Android AES 文件加密解密
- java delphi aes 加密与解密文件兼容算法
- 用AES来加密和解密文件
- aes加密解密文件,以及计算文件的效验值,附带字符串加密解密
- (原创)android使用AES加密和解密文件
- Android AES 文件加密解密
- Android 加密/解密音频文件(AES)
- java_AES加密解密文件以及字符串
- Unity3D 使用AES方式加密与解密文件
- [转]C# 使用 256 位 AES 加密和解密文件
- Android端可用的AES加密/解密,已直接封装为文件加密