C#基础-异常处理
2016-12-04 22:37
176 查看
异常是指程序本身没错,而在运行中发生的错误:
--- 用户输入错误引发
--- 数据库连接不上
---其它因为运行环境导致的错误
常见异常:
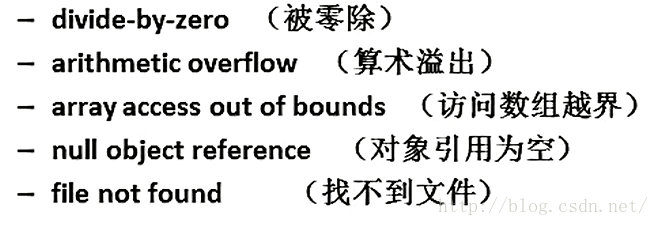
捕获异常:
当代码段有可能发生异常的时候,我们应该把该代码段放置在 try 中。
捕获到异常后的处理方法放置在 catch 中。
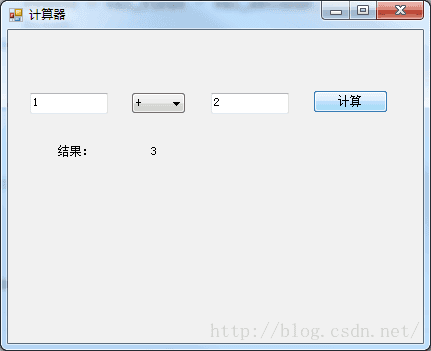
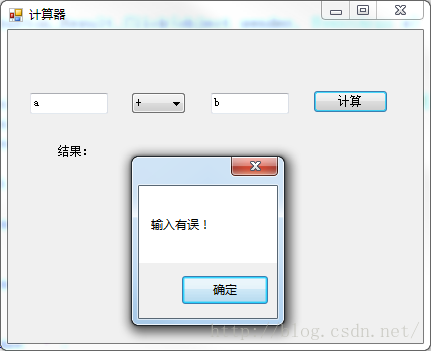
多个 catch 块
--- 为每个可能的 Exception 定制解决方法
异常捕获的顺序:
必须正确排列捕获异常的 catch 子句
--- 范围小的 Exception 放在前面的 catch 子句
--- 即如果 Exception 之间有继承关系,把子类放在前面的 catch 子句中,把父类放在后面的 catch 子句中
在进行完 catch 子句后,程序将继续执行
。。。除非在 catch 子句中有return 、 throw 、或者 exit
Finally 关键字:
使用 try - catch - finally 来确保一些收尾工作
finally 段语句总在 try or catch 之后执行
catch (Exception ex)//最大类
{
MessageBox.Show(ex.Message);
return;
}
finally
{
this.Close();
}
Throw 关键字:
类中可以抛出异常
不返回错误代码,不输出错误信息,
抛出特定的类型。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 异常
{
public partial class Form_Main : Form
{
public Form_Main()
{
InitializeComponent();
cb_oper.SelectedIndex = 0;//初始选择为 + 运算
//comboBox 不能修改 在 DropDownStyle 属性设置为 DropDownList.
//Simple 简单的下拉列表框(始终显示列表)
//DropDown 可以编辑,与有下拉列表。默认.
//DropDownList 只有下拉列表,不能编辑。
}
private void button_Result_Click(object sender, EventArgs e)
{
try
{
int val_first = Convert.ToInt32(tb_first.Text);//若检验数据出错 跳转到catch
int val_seconde = int.Parse(tb_seconde.Text);
int result = 0;
switch (cb_oper.Text)
{
case "+":
result = val_first + val_seconde;
break;
case "-":
result = val_first - val_seconde;
break;
case "*":
result = val_first * val_seconde;
break;
case "/":
result = val_first / val_seconde;
break;
default:
result = 0;
break;
}
lb_result.Text = result.ToString();
if (result > 10)
{
throw new ApplicationException("大于10 抛出异常");
}
}
//catch
//{
// MessageBox.Show("输入有误!");
// return;//返回程序出错之前
//}
catch (FormatException ex)
{
MessageBox.Show(ex.Message);
return;
}
catch (OverflowException ex)
{
MessageBox.Show(ex.Message);
return;
}
catch (DivideByZeroException ex)
{
MessageBox.Show(ex.Message);
return;
}
catch (Exception ex)//最大类
{
MessageBox.Show(ex.Message);
return;
}
finally
{
this.Close();
}
}
}
}
--- 用户输入错误引发
--- 数据库连接不上
---其它因为运行环境导致的错误
常见异常:
捕获异常:
当代码段有可能发生异常的时候,我们应该把该代码段放置在 try 中。
捕获到异常后的处理方法放置在 catch 中。
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace 异常 { public partial class Form_Main : Form { public Form_Main() { InitializeComponent(); cb_oper.SelectedIndex = 0;//初始选择为 + 运算 //comboBox 不能修改 在 DropDownStyle 属性设置为 DropDownList. //Simple 简单的下拉列表框(始终显示列表) //DropDown 可以编辑,与有下拉列表。默认. //DropDownList 只有下拉列表,不能编辑。 } private void button_Result_Click(object sender, EventArgs e) { try { int val_first = Convert.ToInt32(tb_first.Text);//若检验数据出错 跳转到catch int val_seconde = int.Parse(tb_seconde.Text); int result = 0; switch (cb_oper.Text) { case "+": result = val_first + val_seconde; break; case "-": result = val_first - val_seconde; break; case "*": result = val_first * val_seconde; break; case "/": result = val_first / val_seconde; break; default: result = 0; break; } lb_result.Text = result.ToString(); } catch { MessageBox.Show("输入有误!"); return;//返回程序出错之前 } } } }
多个 catch 块
--- 为每个可能的 Exception 定制解决方法
异常捕获的顺序:
必须正确排列捕获异常的 catch 子句
--- 范围小的 Exception 放在前面的 catch 子句
--- 即如果 Exception 之间有继承关系,把子类放在前面的 catch 子句中,把父类放在后面的 catch 子句中
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace 异常 { public partial class Form_Main : Form { public Form_Main() { InitializeComponent(); cb_oper.SelectedIndex = 0;//初始选择为 + 运算 //comboBox 不能修改 在 DropDownStyle 属性设置为 DropDownList. //Simple 简单的下拉列表框(始终显示列表) //DropDown 可以编辑,与有下拉列表。默认. //DropDownList 只有下拉列表,不能编辑。 } private void button_Result_Click(object sender, EventArgs e) { try { int val_first = Convert.ToInt32(tb_first.Text);//若检验数据出错 跳转到catch int val_seconde = int.Parse(tb_seconde.Text); int result = 0; switch (cb_oper.Text) { case "+": result = val_first + val_seconde; break; case "-": result = val_first - val_seconde; break; case "*": result = val_first * val_seconde; break; case "/": result = val_first / val_seconde; break; default: result = 0; break; } lb_result.Text = result.ToString(); } //catch //{ // MessageBox.Show("输入有误!"); // return;//返回程序出错之前 //} catch (FormatException ex) { MessageBox.Show(ex.Message); return; } catch (OverflowException ex) { MessageBox.Show(ex.Message); return; } catch (DivideByZeroException ex) { MessageBox.Show(ex.Message); return; } catch (Exception ex)//最大类 { MessageBox.Show(ex.Message); return; } } } }
在进行完 catch 子句后,程序将继续执行
。。。除非在 catch 子句中有return 、 throw 、或者 exit
Finally 关键字:
使用 try - catch - finally 来确保一些收尾工作
finally 段语句总在 try or catch 之后执行
catch (Exception ex)//最大类
{
MessageBox.Show(ex.Message);
return;
}
finally
{
this.Close();
}
Throw 关键字:
类中可以抛出异常
不返回错误代码,不输出错误信息,
抛出特定的类型。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 异常
{
public partial class Form_Main : Form
{
public Form_Main()
{
InitializeComponent();
cb_oper.SelectedIndex = 0;//初始选择为 + 运算
//comboBox 不能修改 在 DropDownStyle 属性设置为 DropDownList.
//Simple 简单的下拉列表框(始终显示列表)
//DropDown 可以编辑,与有下拉列表。默认.
//DropDownList 只有下拉列表,不能编辑。
}
private void button_Result_Click(object sender, EventArgs e)
{
try
{
int val_first = Convert.ToInt32(tb_first.Text);//若检验数据出错 跳转到catch
int val_seconde = int.Parse(tb_seconde.Text);
int result = 0;
switch (cb_oper.Text)
{
case "+":
result = val_first + val_seconde;
break;
case "-":
result = val_first - val_seconde;
break;
case "*":
result = val_first * val_seconde;
break;
case "/":
result = val_first / val_seconde;
break;
default:
result = 0;
break;
}
lb_result.Text = result.ToString();
if (result > 10)
{
throw new ApplicationException("大于10 抛出异常");
}
}
//catch
//{
// MessageBox.Show("输入有误!");
// return;//返回程序出错之前
//}
catch (FormatException ex)
{
MessageBox.Show(ex.Message);
return;
}
catch (OverflowException ex)
{
MessageBox.Show(ex.Message);
return;
}
catch (DivideByZeroException ex)
{
MessageBox.Show(ex.Message);
return;
}
catch (Exception ex)//最大类
{
MessageBox.Show(ex.Message);
return;
}
finally
{
this.Close();
}
}
}
}
相关文章推荐
- c#基础语言编程-异常处理
- C#基础总结——异常处理
- c#基础-异常处理
- 【C#】基础知识—程序调试与异常处理机制
- 异常处理基础(C#参考)
- c#基础之异常处理
- C#语言学习--基础部分(六) --异常处理
- (C#基础)异常处理
- [基础]c#.net基本函数列表
- 数据结构基础 - 链表的创建(C和C#代码)
- 在C#中使用异步Socket编程实现TCP网络服务的C/S的通讯构架(一)----基础类库部分
- 给豆浆出的c#基础题目
- ASP.NET(C#)学习基础
- 异常处理机制(JAVA&C#)
- [c#基础] windows窗体锁定
- 在C#中使用异步Socket编程实现TCP网络服务的C/S的通讯构架(一)----基础类库部分
- <<展现C#>> 第二章 NGWS Runtime 技术基础(修订)
- 在C#中使用异步Socket编程实现TCP网络服务的C/S的通讯构架(一)----基础类库部分
- 企业信息化系统基础——AD:使用C#批量创建帐号
- c#网络编程之------------Socket编程基础