使用Struts2+Hibernate开发学生信息管理功能
2016-12-03 19:41
429 查看
运行结果:
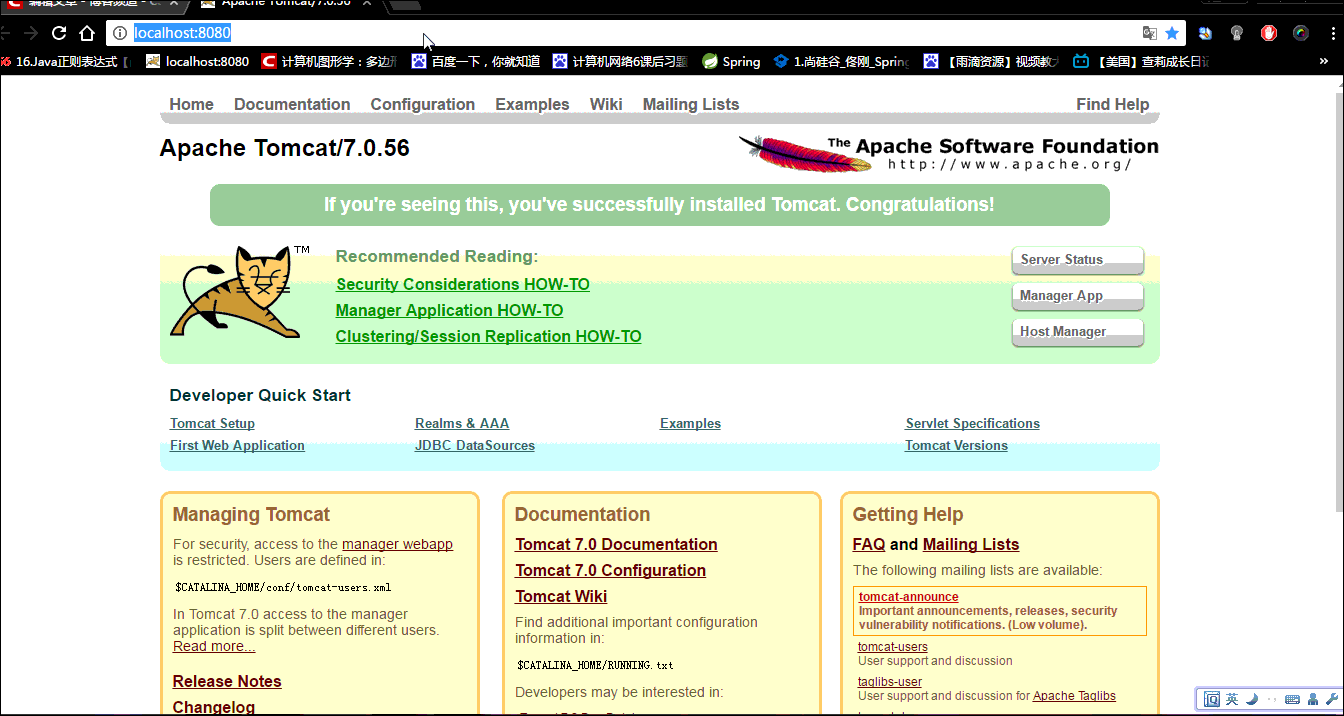
总共两个表,用户表Users和学生信息表Students。
项目内容:
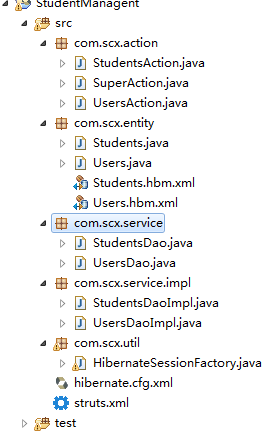
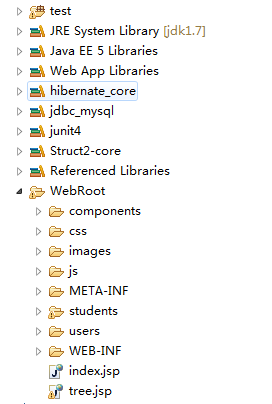
其中com.scx.action包中包含
所有action动作的父类SuperAction
学生信息动作类StudentsAction
用户动作类UsersAction
com.scx.entity包中包含
学生实体类Students
用户实体类Users
Students对象关系映射文件Students.hbm.xml
Users对象关系映射文件Users.hbm.xml
com.scx.service包中包含
学生业务逻辑接口StudentsDao
用户业务逻辑接口UsersDao
com.scx.service.impl包中包含
用户业务逻辑接口StudentsDao对应的实现类StudentsDaoImpl
用户业务逻辑接口UsersDao对应的实现类UsersDaoImpl
com.scx.util包中包含
HibernateSessionFactory类,一个单例模式返回SessionFactory对象
test文件夹 主要是junit测试内容
代码展示:
Students实体类
用户业务逻辑接口
在添加学生时 为学生获取一个最新的学号。
所有action的父类SuperAction 类,为了获得常用的内置对象,采用耦合IOC方式注入属性。继承ActionSupport 并实现ServletRequestAware,ServletResponseAware,ServletContextAware 。获取相应的request,response,session,这样的话,每个继承SuperAction 的类都能够使用request,response,session
UsersAction类,继承SuperAction并实现ModelDriven<Users>
StudentsAction类,继承SuperAction并实现ModelDriven<Students>
struct.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.1//EN" "http://struts.apache.org/dtds/struts-2.1.dtd">
<struts>
<package name="default" namespace="/" extends="struts-default">
</package>
<package name="users" namespace="/users" extends="default">
<action name="*_*" class="com.scx.action.{1}Action" method="{2}">
<result name="login_success">/users/Users_login_success.jsp</result>
<result name="login_failure">/users/Users_login.jsp</result>
<result name="logout_success">/users/Users_login.jsp</result>
<result name="input">/users/Users_login.jsp</result>
</action>
</package>
<package name="students" namespace="/students" extends="default">
<action name="*_*" class="com.scx.action.{1}Action" method="{2}">
<result name="query_success">/students/Students_query_success.jsp</result>
<result name="delete_success" type="chain">Students_query</result>
<result name="add_success" >/students/Students_add_success.jsp</result>
<result name="student_modify" >/students/Students_modify.jsp</result>
<result name="update_success" >/students/Students_modify_success.jsp</result>
</action>
</package>
</struts>
struct.xml.Flow
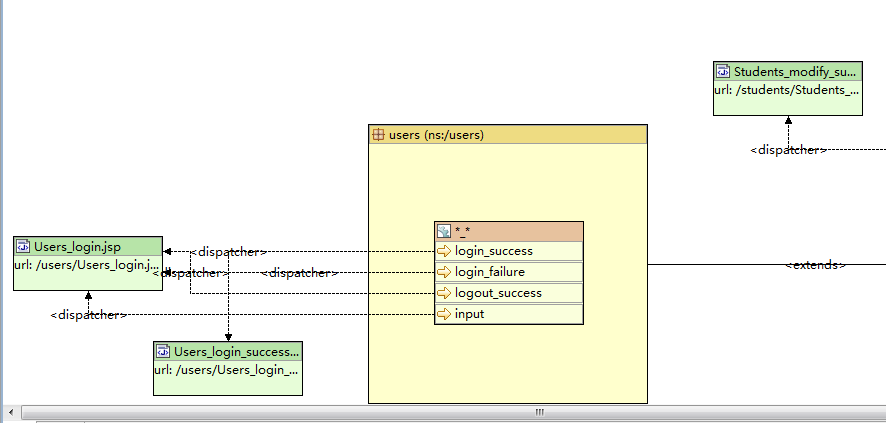
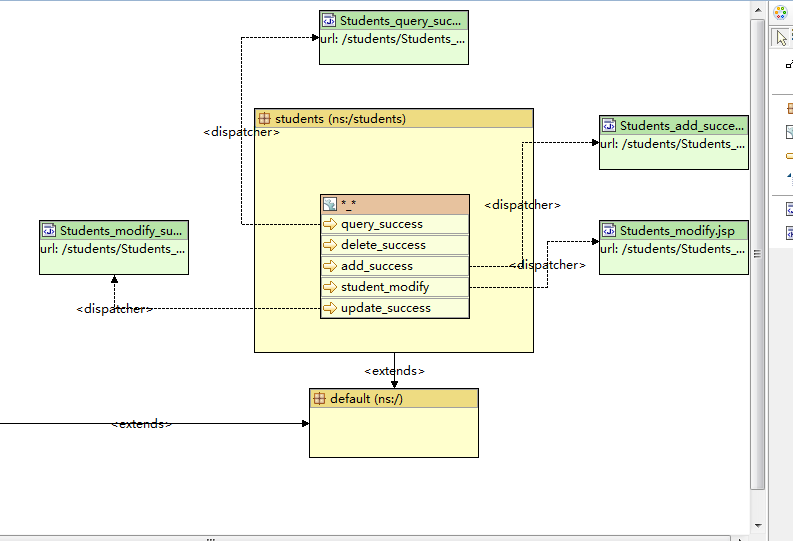
jsp代码就不发了。
jsp素材点击下载
所有代码点击下载
总共两个表,用户表Users和学生信息表Students。
项目内容:
其中com.scx.action包中包含
所有action动作的父类SuperAction
学生信息动作类StudentsAction
用户动作类UsersAction
com.scx.entity包中包含
学生实体类Students
用户实体类Users
Students对象关系映射文件Students.hbm.xml
Users对象关系映射文件Users.hbm.xml
com.scx.service包中包含
学生业务逻辑接口StudentsDao
用户业务逻辑接口UsersDao
com.scx.service.impl包中包含
用户业务逻辑接口StudentsDao对应的实现类StudentsDaoImpl
用户业务逻辑接口UsersDao对应的实现类UsersDaoImpl
com.scx.util包中包含
HibernateSessionFactory类,一个单例模式返回SessionFactory对象
test文件夹 主要是junit测试内容
代码展示:
Students实体类
package com.scx.entity; import java.util.Date; //学生实体类 public class Students { private String sid;//学号 private String sname;//姓名 private String gender;//性别 private Date birthday;//出生日期 private String address;//地址 public Students() { } public Students(String sid, String sname, String gender, Date birthday, String address) { this.sid = sid; this.sname = sname; this.gender = gender; this.birthday = birthday; this.address = address; } public String getSid() { return sid; } public void setSid(String sid) { this.sid = sid; } public String getSname() { return sname; } public void setSname(String sname) { this.sname = sname; } public String getGender() { return gender; } public void setGender(String gender) { this.gender = gender; } public Date getBirthday() { return birthday; } public void setBirthday(Date birthday) { this.birthday = birthday; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } }Users实体类
package com.scx.entity; public class Users { private int uid;//主键id private String username;//用户名 private String password;//密码 public Users(int uid, String username, String password) { this.uid = uid; this.username = username; this.password = password; } public Users() { } public int getUid() { return uid; } public void setUid(int uid) { this.uid = uid; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } }Students.hbm.xml 这里要注意 学生主键的生成方式为assigned
<?xml version="1.0" encoding="utf-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <!-- Mapping file autogenerated by MyEclipse Persistence Tools --> <hibernate-mapping> <class name="com.scx.entity.Students" table="students" catalog="studentmanagent"> <id name="sid" type="java.lang.String"> <column name="SID" /> <generator class="assigned" /> </id> <property name="sname" type="java.lang.String"> <column name="SNAME" length="20" /> </property> <property name="gender" type="java.lang.String"> <column name="GENDER" length="2" /> </property> <property name="birthday" type="date"> <column name="BIRTHDAY" /> </property> <property name="address" type="string"> <column name="ADDRESS" length="50"></column> </property> </class> </hibernate-mapping>Users.hbm.xml
<?xml version="1.0" encoding="utf-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <!-- Mapping file autogenerated by MyEclipse Persistence Tools --> <hibernate-mapping> <class name="com.scx.entity.Users" table="USERS" catalog="studentmanagent"> <id name="uid" type="java.lang.Integer"> <column name="UID" /> <generator class="native" /> </id> <property name="username" type="string"> <column name="USERNAME" length="30"></column> </property> <property name="password" type="string"> <column name="PASSWORD" length="30"></column> </property> </class> </hibernate-mapping>hibernate.cfg.xml
<?xml version='1.0' encoding='UTF-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <!-- Generated by MyEclipse Hibernate Tools. --> <hibernate-configuration> <session-factory> <property name="dialect"> org.hibernate.dialect.MySQLDialect </property> <property name="connection.url"> jdbc:mysql://localhost:3306/susu </property> <property name="connection.username">root</property> <property name="connection.password">123</property> <property name="connection.driver_class"> com.mysql.jdbc.Driver </property> <property name="myeclipse.connection.profile">susu</property> <property name="hbm2ddl.auto">update</property> <property name="show_sql">true</property> <property name="hibernate.current_session_context_class">thread</property> <mapping resource="com/scx/entity/Students.hbm.xml" /> <mapping resource="com/scx/entity/Users.hbm.xml" /> </session-factory> </hibernate-configuration>
用户业务逻辑接口
package com.scx.service; import com.scx.entity.Users; //用户业务逻辑接口 public interface UsersDao { //用户登录操作 public boolean usersLogin(Users user); }学生业务逻辑接口
package com.scx.service; import java.util.List; import com.scx.entity.Students; //学生业务逻辑接口 public interface StudentsDao { //查询所有学生信息 public List<Students> queryAllStudents(); //根据学号查询学生信息 public Students queryStudentsBySid(String sid); //根据学号删除学生 public boolean deleteStudentsBySid(String sid); //添加学生 public boolean addStudents(Students stu); //更新学生信息 public boolean updateStudent(Students stu); }用户业务逻辑接口实现类
package com.scx.service.impl; import java.util.List; import org.hibernate.Query; import org.hibernate.Session; import org.hibernate.Transaction; import com.scx.entity.Students; import com.scx.entity.Users; import com.scx.service.UsersDao; import com.scx.util.HibernateSessionFactory; public class UsersDaoImpl implements UsersDao { //用户登录操作 @SuppressWarnings("unchecked") public boolean usersLogin(Users user) { Transaction transaction = null; String hql = ""; try { Session session = HibernateSessionFactory.getSessionFactory() .getCurrentSession(); transaction = session.beginTransaction(); hql = "from Users where username = ? and password = ?"; Query query = session.createQuery(hql); query.setParameter(0, user.getUsername()); query.setParameter(1, user.getPassword()); List<Students> list = query.list(); transaction.commit(); if (list.size() > 0 && list != null) { return true; } } catch (Exception e) { e.printStackTrace(); return false; } finally { if (transaction != null) { transaction = null; } } return false; } }学生业务逻辑接口实现类 除了接口内的方法外 还有一个私有的方法getNewSid
在添加学生时 为学生获取一个最新的学号。
package com.scx.service.impl; import java.util.ArrayList; import java.util.List; import org.hibernate.Query; import org.hibernate.Session; import org.hibernate.Transaction; import com.scx.entity.Students; import com.scx.service.StudentsDao; import com.scx.util.HibernateSessionFactory; public class StudentsDaoImpl implements StudentsDao{ //查询所有的学生信息 @SuppressWarnings("unchecked") public List<Students> queryAllStudents() { List<Students> students=new ArrayList<Students>(); Transaction tc=null; String hql=""; try{ Session session=HibernateSessionFactory.getSessionFactory().getCurrentSession(); tc=session.beginTransaction(); hql=" from Students"; Query query=session.createQuery(hql); students=query.list(); tc.commit(); if(students.size()>0&&students!=null){ return students; }else{ return null; } }catch(Exception e){ e.printStackTrace(); tc.commit(); return null; }finally{ if(tc!=null){ tc=null; } } } //根据学号查询学生信息 public Students queryStudentsBySid(String sid) { Students stu=null; Transaction tc=null; try{ Session session=HibernateSessionFactory.getSessionFactory().getCurrentSession(); tc=session.beginTransaction(); stu=(Students) session.get(Students.class, sid); tc.commit(); return stu; }catch(Exception e){ e.printStackTrace(); tc.commit(); return null; }finally{ if(tc!=null){ tc=null; } } } //根据学号删除学生 public boolean deleteStudentsBySid(String sid) { Transaction tc=null; Session session=null; try{ session=HibernateSessionFactory.getSessionFactory().getCurrentSession(); tc=session.beginTransaction(); Students stu=(Students) session.get(Students.class, sid); session.delete(stu); tc.commit(); return true; }catch(Exception e){ e.printStackTrace(); tc.commit(); return false; }finally{ if(tc!=null){ tc=null; } } } //添加学生 public boolean addStudents(Students stu) { Transaction tc=null;; try{ stu.setSid(getNewSid()); Session sessin=HibernateSessionFactory.getSessionFactory().getCurrentSession(); tc=sessin.beginTransaction(); sessin.save(stu); tc.commit(); return true; }catch(Exception e){ tc.commit(); e.printStackTrace(); return false; }finally{ if(tc!=null){ tc=null; } } } //获取新增学生的学号 private String getNewSid(){ String sid=null; Transaction tc=null; String hql=""; try{ //首先从数据库中读取最大的学号 然后拼装学号 Session session=HibernateSessionFactory.getSessionFactory().getCurrentSession(); tc=session.beginTransaction(); hql="select max(sid) from Students"; Query query=session.createQuery(hql); String temp=(String) query.uniqueResult(); tc.commit(); if(temp==null||"".equals(temp.trim())){ sid="s00000001"; }else{ int x=Integer.parseInt(temp.substring(1)); System.out.println(x); temp=String.valueOf(++x); //拼装学号为8为 不够前面补0 while(temp.length()<8){ temp="0"+temp; } sid="s"+temp; } return sid; }catch(Exception e){ tc.commit(); e.printStackTrace(); return sid; }finally{ if(tc!=null){ tc=null; } } } //更新学生信息 public boolean updateStudent(Students stu) { Transaction tc=null; try{ Session session=HibernateSessionFactory.getSessionFactory().getCurrentSession(); tc=session.beginTransaction(); session.update(stu); tc.commit(); return true; }catch (Exception e) { e.printStackTrace(); tc.commit(); return false; } } }
所有action的父类SuperAction 类,为了获得常用的内置对象,采用耦合IOC方式注入属性。继承ActionSupport 并实现ServletRequestAware,ServletResponseAware,ServletContextAware 。获取相应的request,response,session,这样的话,每个继承SuperAction 的类都能够使用request,response,session
package com.scx.action; import javax.servlet.ServletContext; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.http.HttpSession; import org.apache.struts2.interceptor.ServletRequestAware; import org.apache.struts2.interceptor.ServletResponseAware; import org.apache.struts2.util.ServletContextAware; import com.opensymphony.xwork2.ActionSupport; //所有action动作的父类 public class SuperAction extends ActionSupport implements ServletRequestAware, ServletResponseAware, ServletContextAware { /** * */ private static final long serialVersionUID = 1L; protected HttpServletRequest request;// 请求对象 protected HttpServletResponse response;// 响应对象 protected HttpSession session;// 会话对象 protected ServletContext context;//全局对象 public void setServletContext(ServletContext context) { this.context = context; } public void setServletResponse(HttpServletResponse response) { this.response = response; } public void setServletRequest(HttpServletRequest request) { this.request = request; this.session = this.request.getSession(); } }
UsersAction类,继承SuperAction并实现ModelDriven<Users>
package com.scx.action; import org.apache.struts2.interceptor.validation.SkipValidation; import com.opensymphony.xwork2.ModelDriven; import com.scx.entity.Users; import com.scx.service.UsersDao; import com.scx.service.impl.UsersDaoImpl; /** * @author scx * 用户动作类 */ public class UsersAction extends SuperAction implements ModelDriven<Users>{ /** * */ private static final long serialVersionUID = 1L; private Users user=new Users(); //用户登录动作 public String login(){ UsersDao dao=new UsersDaoImpl(); if(dao.usersLogin(user)){ session.setAttribute("loginUserName", user.getUsername()); return "login_success"; }else{ return "login_failure"; } } //用户退出动作 跳过验证 @SkipValidation public String logout(){ if(session.getAttribute("loginUserName")!=null){ session.removeAttribute("loginUserName"); } return "logout_success"; } public Users getModel() { return user; } @Override public void validate() { if(user.getUsername()==null||"".equals(user.getUsername().trim())){ this.addFieldError("usernameError", "请输入帐号"); } if(user.getPassword().length()<6){ this.addFieldError("passwordError", "密码不少于6位"); } } }
StudentsAction类,继承SuperAction并实现ModelDriven<Students>
package com.scx.action; import java.util.List; import com.opensymphony.xwork2.ModelDriven; import com.scx.entity.Students; import com.scx.service.StudentsDao; import com.scx.service.impl.StudentsDaoImpl; /** * @author scx * 学生信息动作类 */ public class StudentsAction extends SuperAction implements ModelDriven<Students>{ /** * */ private static final long serialVersionUID = 1L; private Students student=new Students(); //查询所有学生 public String query(){ StudentsDao sdao=new StudentsDaoImpl(); List<Students> students=sdao.queryAllStudents(); if(students!=null&&students.size()>0){ session.setAttribute("students_list", students); }else{ session.setAttribute("students_list", null); } return "query_success"; } //删除学生 public String delete(){ StudentsDao sdao=new StudentsDaoImpl(); String sid=request.getParameter("sid"); sdao.deleteStudentsBySid(sid); return "delete_success"; } //添加学生 public String add(){ StudentsDao sdao=new StudentsDaoImpl(); sdao.addStudents(student); return "add_success"; } //获得要修改的学生的信息 public String modify(){ StudentsDao sdao=new StudentsDaoImpl(); Students stu=sdao.queryStudentsBySid(request.getParameter("sid")); session.setAttribute("modify_students", stu); return "student_modify"; } //修改学生信息 public String update(){ StudentsDao sdao=new StudentsDaoImpl(); sdao.updateStudent(student); return "update_success"; } public Students getModel() { return student; } }
struct.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.1//EN" "http://struts.apache.org/dtds/struts-2.1.dtd">
<struts>
<package name="default" namespace="/" extends="struts-default">
</package>
<package name="users" namespace="/users" extends="default">
<action name="*_*" class="com.scx.action.{1}Action" method="{2}">
<result name="login_success">/users/Users_login_success.jsp</result>
<result name="login_failure">/users/Users_login.jsp</result>
<result name="logout_success">/users/Users_login.jsp</result>
<result name="input">/users/Users_login.jsp</result>
</action>
</package>
<package name="students" namespace="/students" extends="default">
<action name="*_*" class="com.scx.action.{1}Action" method="{2}">
<result name="query_success">/students/Students_query_success.jsp</result>
<result name="delete_success" type="chain">Students_query</result>
<result name="add_success" >/students/Students_add_success.jsp</result>
<result name="student_modify" >/students/Students_modify.jsp</result>
<result name="update_success" >/students/Students_modify_success.jsp</result>
</action>
</package>
</struts>
struct.xml.Flow
jsp代码就不发了。
jsp素材点击下载
所有代码点击下载
相关文章推荐
- 慕课网 使用Struts2+Hibernate开发学生信息管理功能
- 慕课网_使用Struts2+Hibernate开发学生信息管理系统
- 使用Struts2+Hibernate开发学生信息管理系统
- 使用Struts+Hibernate开发学生信息管理系统
- 【Struts2+Hibernate4】开发学生信息管理功能(三)
- 【Struts2+Hibernate4】开发学生信息管理功能(一)
- [置顶] Struts2+Hibernate4开发学生信息管理功能--(二)Struts2和Hibernate整合
- 【Struts2+Hibernate4】开发学生信息管理功能(二)
- 慕课网 项目实战 使用struts2和hibernate开发学生信息管理系统
- 完成一个学生管理程序,使用学号作为键添加5个学生对象,并可以将全部信息保存在文件中,可以实现对学生信息的学号查找,输出全部学生信息的功能。
- [置顶] Struts2+Hibernate4开发学生信息管理功能--(四)学生信息管理模块
- 完成一个学生管理程序,使用学号作为键添加5个学生对象,并可以将全部信息保存在文件中,可以实现对学生信息的学号查找,输出全部学生信息的功能。
- 完成一个学生管理程序,使用学号作为键添加5个学生对象,并可以将全部信息保存在文件中,可以实现对学生信息的学号查找,输出全部学生信息的功能。
- 完成一个学生管理程序,使用学号作为键添加5个学生对象,并可以将全部信息保存在文件中,可以实现对学生信息的学号查找,输出全部学生信息的功能。
- 完成一个学生管理程序,使用学号作为键添加5个学生对象,并可以将全部信息保存在文件中,可以实现对学生信息的学号查找,输出全部学生信息的功能。
- [置顶] Struts2+Hibernate4开发学生信息管理功能--(三)用户登录模块
- 完成一个学生管理程序,使用学号作为键添加5个学生对象,并可以将全部信息保存在文件中,可以实现对学生信息的学号查找,输出全部学生信息的功能。
- node.js学习笔记——学生信息管理的实现(把功能模块化)使用官方提供的http模块实现
- 实践hibernate的应用——struts2+hibernate的简单学生信息管理
- 基于Struts2+Hibernate的学生信息管理系统实例