微信小程序记账应用实例课程(三)服务端实现账目CRUD
2016-10-24 15:13
756 查看
[2016-10-24]
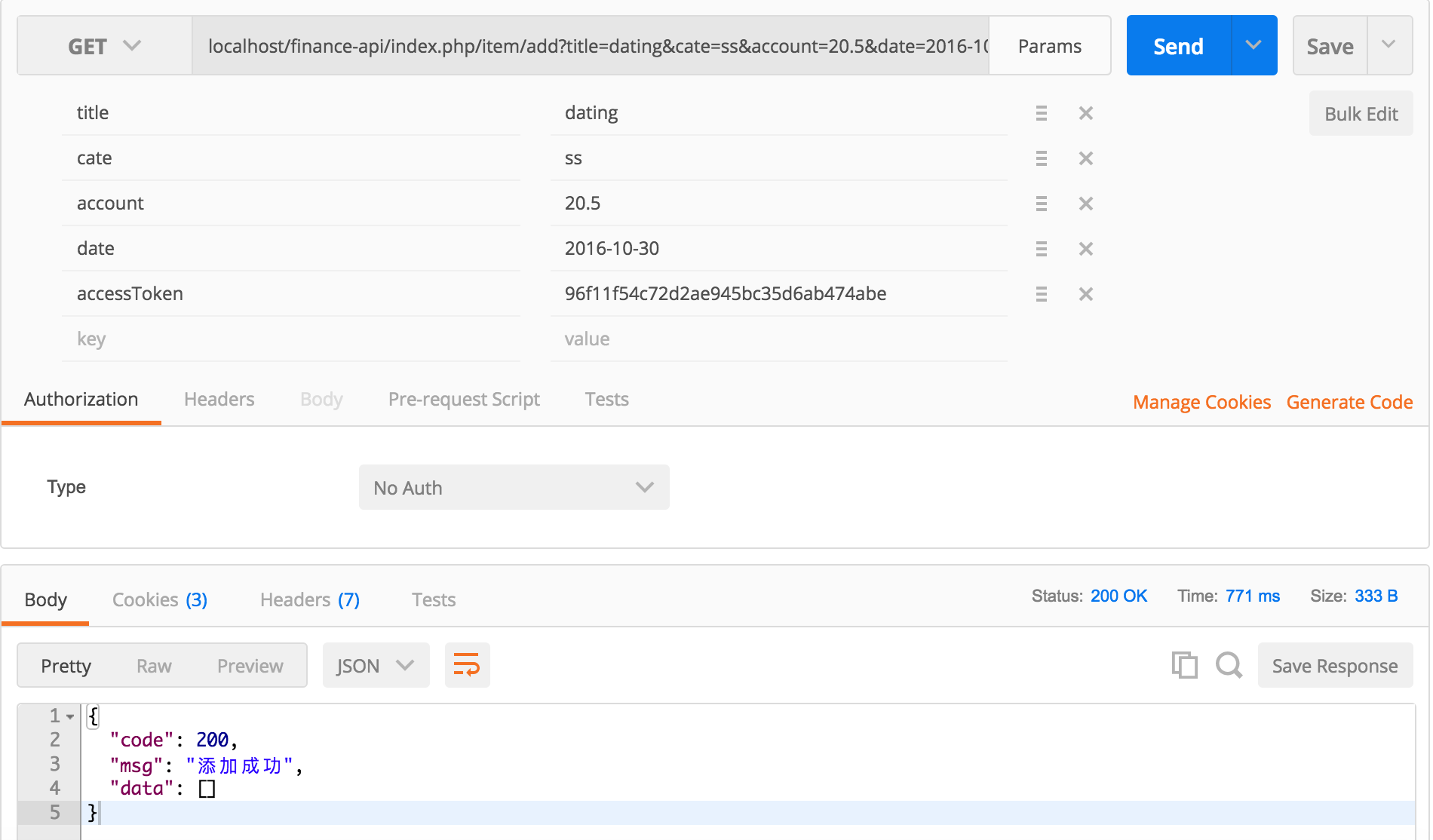
实现json输出函数,$data每次必传,msg,code可以缺省
继承之
起一个add方法,输出一个标准json
2.对accessToken进行认证
声明一个auth的私有方法,在构造函数__construct()中调用,如果认证通过返回true,使得程序继续执行;失败则输出json错误提示信息,不再执行,退出程序。
3.控制器add方法写入数据库
4.依样画葫芦,完成CRUD的其他操作,注意访问权限,不可跨用户操作
附:
参数:openid
返回uid nickname accessToken
入参:title,cate,account,date
返回是否成功
返回
id,title,cate,account,date
入参:id,title,cate,account,date
入参:除accessToken无
源码下载:关注下方的公众号->回复数字1009
对小程序开发有趣的朋友关注公众号: huangxiujie85,QQ群: 575136499,微信: small_application,陆续还将推出更多作品。
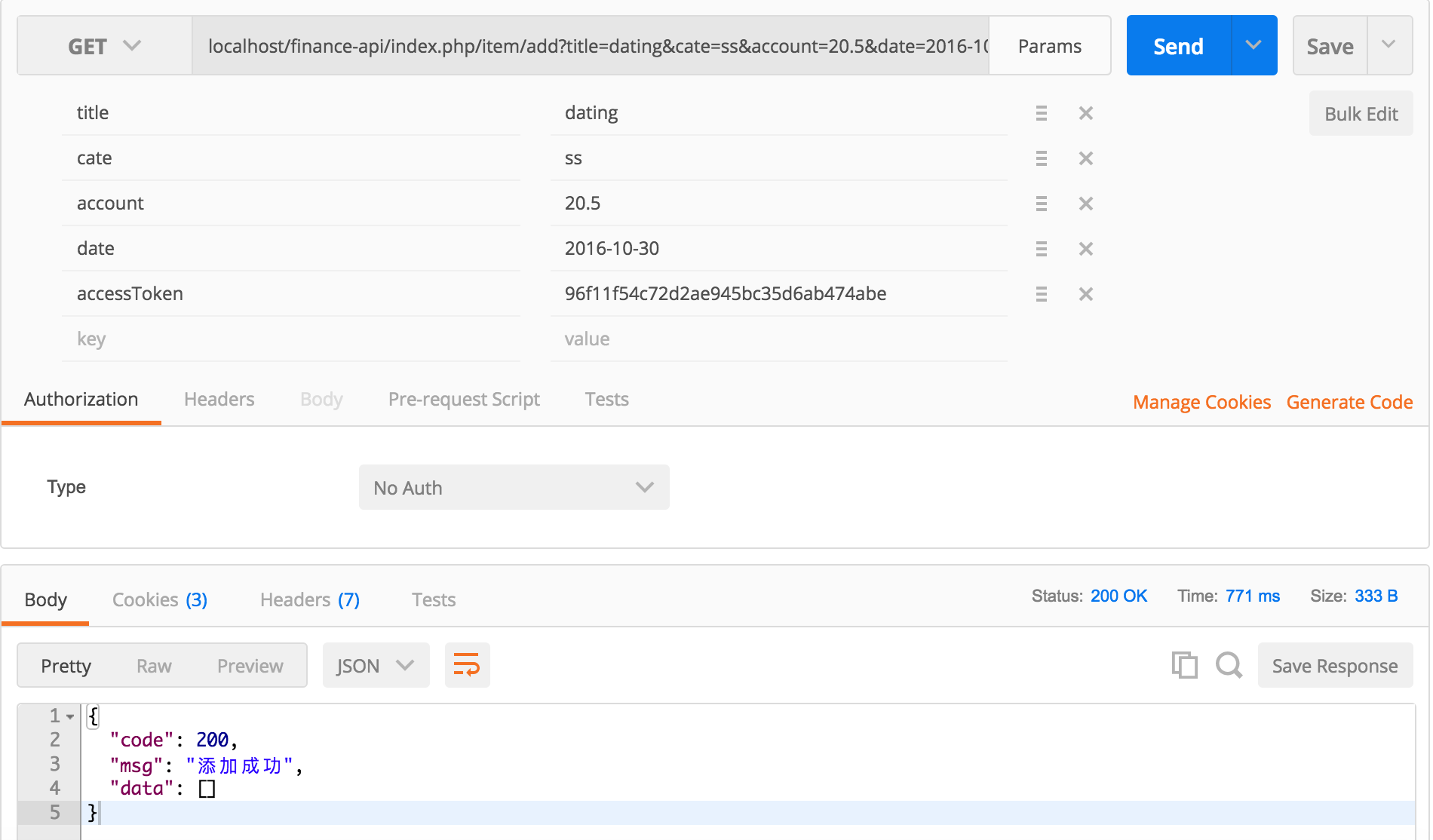
目标:凭借accessToken来添加一条账目
对应接口item/add创建基类
完成json默认输出格式实现json输出函数,$data每次必传,msg,code可以缺省
<?php header("Content-type: application/json"); defined('BASEPATH') OR exit('No direct script access allowed'); class BaseController extends CI_Controller { protected function json_output($data, $msg = '加载成功', $code = 200){ echo json_encode(array('code' => $code, 'msg' => $msg,'data' => $data)); } }
调用示例
引入BaseController.php继承之
起一个add方法,输出一个标准json
<?php require_once 'BaseController.php'; class Item extends BaseController { public function add() { $this->json_output(array(), '成功', 200); } }
账目保存数据库
1.建表CREATE TABLE `item` ( `id` int(11) NOT NULL AUTO_INCREMENT, `title` varchar(32) DEFAULT NULL, `cate` varchar(32) DEFAULT NULL, `account` decimal(10,1) DEFAULT NULL, `date` date DEFAULT NULL, `uid` int(11) DEFAULT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=utf8;
2.对accessToken进行认证
声明一个auth的私有方法,在构造函数__construct()中调用,如果认证通过返回true,使得程序继续执行;失败则输出json错误提示信息,不再执行,退出程序。
// 缓存uid protected $uid; /** * 构造函数,子类如Item控制器会自动调用它 */ function __construct() { parent::__construct(); $this->auth(); } /** * 认证,拿到accessToken,表明用户是已授权微信登录的用户,该accessToken缓存在小程序侧 */ private function auth(){ $accessToken = $this->input->get('accessToken'); // 查询数据库,是否有此用户 $query = $this->db->query("select * from user where accessToken = '$accessToken'"); if ($query->num_rows() > 0) { $this->uid = $query->first_row()->uid; return true; } $this->json_output(array(), '认证失败', 401); // 如果没有查询到,直接结束程序,不必走正常控制器方法如item/add的json输出 exit; }
3.控制器add方法写入数据库
// 添加账目 public function add() { // 取到数组 $data = $this->input->get(); // 移除accessToken,因不被添加到数据表 unset($data['accessToken']); // 添加uid $data['uid'] = $this->uid; // 写入数据库 if ($this->db->insert('item', $data)) { // 返回结果 return $this->json_output(array(), '添加成功', 200); } }
4.依样画葫芦,完成CRUD的其他操作,注意访问权限,不可跨用户操作
// 删除账目 public function del(){ // 获取id $id = $this->input-> 4000 ;get('id'); // 数据库中删除。需依据id与uid,保证资源的合法性,只删除自己的账目 $this->db->delete('item', array('id'=>$id, 'uid'=>$this->uid)); if ($this->db->affected_rows() > 0) { $this->json_output(array(), '删除成功', 200); } else { $this->json_output(array(), '权限不足', 400); } } // 读取账目 public function view(){ //获取id $id = $this->input->get('id'); //查询数据库 $query = $this->db->get_where('item', array('id' => $id, 'uid' => $this->uid)); if ($query->num_rows() > 0) { // 输出账目 $this->json_output($query->first_row(), '加载成功', 200); } else { $this->json_output(array(), '权限不足', 400); } } // 修改账目 public function update() { // 获取提交进来的参数 $data = $this->input->get(); // 移除accessToken,因不被添加到数据表 unset($data['accessToken']); // 先判断资源所属 $query = $this->db->get_where('item', array('id' => $data['id'], 'uid' => $this->uid)); if ($query->num_rows() > 0) { // 更新数据库 if ($this->db->update('item', $data, array('id'=>$data['id']))) { $this->json_output(array(), '修改成功', 200); } } else { $this->json_output(array(), '权限不足', 400); } } // 账目列表 public function all(){ // 查询该用户下的所有账目 $query = $this->db->get_where('item', array('uid'=>$this->uid)); $this->json_output($query->result(), '加载成功', 200); }
附:
接口规范:
1.用户登录
user/login参数:openid
返回uid nickname accessToken
2.添加一条账目,accessToken均需要入参,下同
item/add入参:title,cate,account,date
返回是否成功
3.读取一条账目
入参:id返回
id,title,cate,account,date
4.修改一条账目
item/edit入参:id,title,cate,account,date
5.删除一条账目
入参:id6.读取自己的账目
item/all入参:除accessToken无
源码下载:关注下方的公众号->回复数字1009
对小程序开发有趣的朋友关注公众号: huangxiujie85,QQ群: 575136499,微信: small_application,陆续还将推出更多作品。
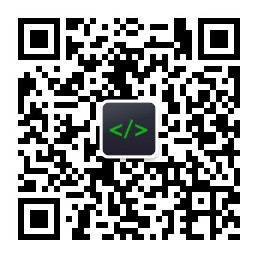
相关文章推荐
- 微信小程序记账应用实例课程(三)服务端实现账目CRUD
- 微信小程序记账应用实例课程(完结)——对接服务端账目CRUD
- 微信小程序 实例应用(记账)详解
- 微信小程序开发记账应用实战服务端之用户注册与登录-基于Yii2描述
- 微信小程序开发记账应用实战服务端之用户注册与登录基于ThinkPHP5描述
- 微信小程序(应用号)实战课程之记账应用开发
- 微信小程序(应用号)实战课程之记账应用开发(续)
- 微信小程序记账应用实例教程(续二)
- 如何实现微信小程序与.net core应用服务端的无状态身份验证
- 微信小程序开发记账应用实战服务端之用户注册与登录基于Codeigniter3描述
- 教你如何实现微信小程序与.net core应用服务端的无状态身份验证
- 微信小程序(应用号)开发新闻客户端的实战课程
- 微信小程序 摇一摇抽奖简单实例实现代码
- 微信小程序 实现列表刷新的实例详解
- 微信小程序(应用号)开发新闻客户端实例
- 微信小程序(应用号)开发新闻客户端实例
- 微信小程序 scroll-view组件实现列表页实例代码
- 微信小程序 实现tabs选项卡效果实例代码
- 微信小程序(应用号)简单实例应用及实例详解