Java IO流知识汇总
2016-10-24 00:00
501 查看
摘要: Io流分为输出流和输入流,又分为字符流和字节流两大类,关于javaIO相关流的知识做了汇总,基础不扎实的同学可以做参考
java.io.File类的使用:
File类用构造器创建其对象,此对象可以对应文件,也可以对应文件目录。
File对象与平台无关;File中的方法仅涉及到如何创建、删除、重命名等操作,涉及到文件内容就要用到流了。
常用方法:
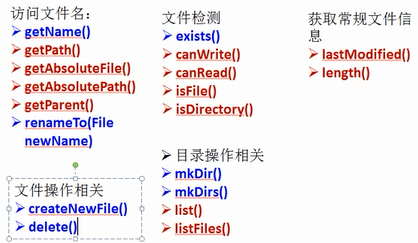
File类的操作比较简单,自己去看Api,renameTo():
file1.renameTo(file2):是将file1重命名为file2,要求file1必须存在,file2必须不存在,才可成功。(重命名原理是删除了原来的文件重新建了一个文件)
File类的方法既能操作文件,又能操作目录;
创建删除文件及目录示例:
mkdir和mkdirs区别在于:如果File路径参数多级均不存在,就用mkdirs,可以创建多个目录,mkdir只能创建一个目录,要求上级目录存在的情况下才返回true,mkdirs上级目录不存在则一并创建。
list()方法返回的是字符串数组,相当于获取相应目录下的所有文件目录,listFiles返回的是File数组,可以对文件及目录进行进一步操作。
Io原理常用流的分类:
原理:
输入:读取外部数据(磁盘、光盘等存储设备的数据)到程序(内存)中。
输出:将程序(内存)数据输出到磁盘、光盘等存储设备中。
关系:
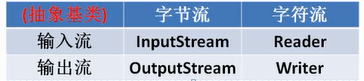
(了解节点流和处理流的概念!如buffered*,节点流直接作用于文件)
文件流:FileInputStream/FileOutputStream/FileReader/FileWriter(从角色角度看是四个最基本的节点流;从处理单位不同的角度看前两个是字节流,后两个是字符流;从数据流向角度看一三是输入流,二四是输出流;每种流都可以拿到三个角度去看)
FileInputStream程序实例(具体注意见注释):
FileOutputStream程序实例(具体注意见注释):
FileInputStream和FileOutputStream综合程序实例(文件复制,复制任何格式的文件):
FileReader程序实例(具体注意见注释):
使用FileReader和FileWriter实现文本复制:(FileReader、FileWriter字符流只能操作文本文件,非文本文件(视频、音频、图片)等其它格式的文件还是要用字节流)
缓冲流:BufferedInputStream/BufferedOutputStream/BufferedReader/BufferedWriter(处理流的一种,实际开发过程中都用这四种,因为用这种处理流包装字节流和字符流,效率大大增加,测试一个20kb的文件,用节点流使用17ms,使用buffered耗时4ms,并且文件越大优势越明显,节点流的read方法是阻塞式的,bufferes是非阻塞式的)使用bufferedoutputsteam和bufferedWriter最后要加上flush()方法,下面提供一个使用处理流的文件复制的方法,具体见注释:
缓冲字符流使用实例:(在读取和写入字符的时候,除了支持读写字符,流还提供了读写一行字符串,readLine和write(String)具体见注释):
转换流:InputStreamReader/OutputStreamWriter(用于字节流和字符流之间的转换,比如读取文本文件使用字符流效率比较高,如果读取到的是字节流,就可以做转换,使用频率并不是很高。解码:字符数组->字符串;编码:字符串->字符数组)
程序实例:
标准输入输出流:(了解)
打印流:PrintStream/PrintWriter()
数据流:DataInputStream/DataOutputStream

数据流使用实例:

基本数据类型用数据流传输,引用数据类型就用对象流来进行传输:
对象流(涉及序列化、反序列化):ObjectInputStream/ObjectOutputStream:
用于存储和读取对象的处理流,强大之处就是可以把Java中的对象写入到数据源中,也可以把对象从数据源中还原回来。
序列化(Serialize):用ObjectOutputStream类将一个java对象写入IO流中;
反序列化(Deserilize):用ObjectInputStream类从IO流中恢复该Java对象。
程序实例:
serialVersionUID用来表明类的不同版本间的兼容性,如果类没有显示定义这个静态变量,它的值是java运行时环境根据类的内部细节自动生成的,若类的源代码做了修改,serialVersionUID可能发生改变,所以建议显示声明。
随机存取文件流:RandomAccessFile类支持“随机访问”的方式,程序可以直接跳到文件的任意地方来读写文件;也可向已存在的文件后追加内容;支持只访问文件的部分内容。
具体详细用法见程序实例:
具体分类:

:
java.io.File类的使用:
File类用构造器创建其对象,此对象可以对应文件,也可以对应文件目录。
File对象与平台无关;File中的方法仅涉及到如何创建、删除、重命名等操作,涉及到文件内容就要用到流了。
常用方法:
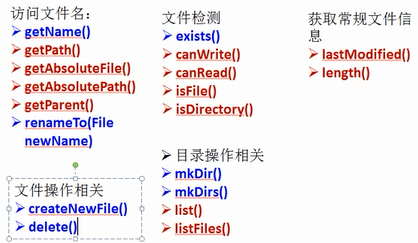
File类的操作比较简单,自己去看Api,renameTo():
file1.renameTo(file2):是将file1重命名为file2,要求file1必须存在,file2必须不存在,才可成功。(重命名原理是删除了原来的文件重新建了一个文件)
File类的方法既能操作文件,又能操作目录;
创建删除文件及目录示例:
public void createFile() throws IOException{ File file1 = new File("D:\\io\\hello.txt"); System.out.println(file1.delete()); if(!file1.exists()){ boolean b = file1.createNewFile(); System.out.println(b); } File file2 = new File("D:\\io\\io2"); System.out.println(file2.delete()); if(!file2.exists()){ boolean b = file2.mkdir(); System.out.println(b); } }
mkdir和mkdirs区别在于:如果File路径参数多级均不存在,就用mkdirs,可以创建多个目录,mkdir只能创建一个目录,要求上级目录存在的情况下才返回true,mkdirs上级目录不存在则一并创建。
list()方法返回的是字符串数组,相当于获取相应目录下的所有文件目录,listFiles返回的是File数组,可以对文件及目录进行进一步操作。
Io原理常用流的分类:
原理:
输入:读取外部数据(磁盘、光盘等存储设备的数据)到程序(内存)中。
输出:将程序(内存)数据输出到磁盘、光盘等存储设备中。
关系:
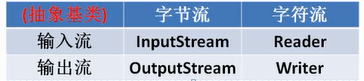
(了解节点流和处理流的概念!如buffered*,节点流直接作用于文件)
文件流:FileInputStream/FileOutputStream/FileReader/FileWriter(从角色角度看是四个最基本的节点流;从处理单位不同的角度看前两个是字节流,后两个是字符流;从数据流向角度看一三是输入流,二四是输出流;每种流都可以拿到三个角度去看)
FileInputStream程序实例(具体注意见注释):
public void testFileInputOutputStream(){ //2、创建一个FileInputStream类的对象 FileInputStream fis = null; try { //1、创建一个File类的对象 File file = new File("hello.txt"); fis = new FileInputStream(file); //3、调用FileInputStream类的方法,实现File文件的读取。 /* int b; while((b = fis.read()) != -1){//这是一个字节一个字节的读,效率低,开发时定义数据一次读多个 System.out.print((char)b); }*/ byte[] b = new byte[5];//读取到的数据要写入的数组 int len;//每次读入到byte中的字节的长度 while((len=fis.read(b)) != -1){ /* for(int i=0;i<len;i++){ System.out.print((char)b[i]); }*/ //也可以不用循环,改进如下“ String str = new String(b, 0, len);//遍历字符数组的时候考虑此构造方法,比循环效率高…… System.out.print(str); } } catch (IOException e) { e.printStackTrace(); }finally{ //4、关闭输入流 if(fis != null){ try { fis.close(); } catch (IOException e) { e.printStackTrace(); } } } }
FileOutputStream程序实例(具体注意见注释):
public void testFileOutputStream(){ //1、创建一个File对象,表明要写入的文件位置 //输出的物理文件可以不存在,执行过程中,不存在则自动创建,若存在会将原有的文件覆盖 File file = new File("hello2.txt"); FileOutputStream fos = null; try { //2、创建一个FileOutputStream的对象,将File的对象做为形参传捡来 fos = new FileOutputStream(file); //3、写入操作 fos.write(new String("I Love you!").getBytes()); } catch (Exception e) { e.printStackTrace(); }finally{ //4、关闭输出流 if(fos != null){ try { fos.close(); } catch (IOException e) { e .printStackTrace(); } } } }
FileInputStream和FileOutputStream综合程序实例(文件复制,复制任何格式的文件):
public void testFileInputOutputStream(){//使用字节流实现复制功能 File file = new File("hello.txt"); FileInputStream fis = null; FileOutputStream fos = null; try { fis = new FileInputStream(file); fos = new FileOutputStream("aa.txt"); byte[] b = new byte[5]; int len; while((len=fis.read(b)) != -1){ //String string = new String(b, 0, len); //fos.write(string.getBytes()); fos.write(b, 0, len);; } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ try { if(fos != null) fos.close(); } catch (IOException e) { e.printStackTrace(); } try { if(fis != null) fis.close(); } catch (IOException e) { e.printStackTrace(); } } }
FileReader程序实例(具体注意见注释):
public void testFileReader(){ File file = new File("readerwriter.txt"); FileReader reder = null; try { reder = new FileReader(file); char[] c = new char[24]; int len; while((len = reder.read(c)) != -1){ String string = new String(c, 0, len); System.out.print(string); } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ if(reder != null){ try { reder.close(); } catch (Exception e2) { e2.printStackTrace(); } } } }
使用FileReader和FileWriter实现文本复制:(FileReader、FileWriter字符流只能操作文本文件,非文本文件(视频、音频、图片)等其它格式的文件还是要用字节流)
public void testFileReaderWriter(){//使用字符流实现复制功能 FileReader fr = null; FileWriter fw = null; try { File file = new File("readerwriter.txt"); fr = new FileReader(file); fw = new FileWriter("writer.txt"); char[] c = new char[24]; int len; while((len = fr.read(c)) != -1){ fw.write(c, 0, len); } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ if(fw != null){ try { fw.close(); } catch (Exception e2) { e2.printStackTrace(); }finally{ if(fr != null){ try { fr.close(); } catch (Exception e3) { e3.printStackTrace(); } } } } } }
缓冲流:BufferedInputStream/BufferedOutputStream/BufferedReader/BufferedWriter(处理流的一种,实际开发过程中都用这四种,因为用这种处理流包装字节流和字符流,效率大大增加,测试一个20kb的文件,用节点流使用17ms,使用buffered耗时4ms,并且文件越大优势越明显,节点流的read方法是阻塞式的,bufferes是非阻塞式的)使用bufferedoutputsteam和bufferedWriter最后要加上flush()方法,下面提供一个使用处理流的文件复制的方法,具体见注释:
public void testBuffered() {//使用缓冲字节流实现文件复制的方法 long start = System.currentTimeMillis(); BufferedInputStream bis = null; BufferedOutputStream bos = null; try { //1、实现读入、写出的文件 File file = new File("wife.jpg"); File file1 = new File("shax.jpg"); //2、想创建相应的节点流 FileInputStream fis = new FileInputStream(file); FileOutputStream fos = new FileOutputStream(file1); //3、将创建的节点流的对象做为形参传递给缓冲流的构造器中 bis = new BufferedInputStream(fis); bos = new BufferedOutputStream(fos); //4、文件复制具体操作 byte[] b = new byte[24]; int len; while((len = bis.read(b)) != -1){ bos.write(b, 0, len); bos.flush();//最后一次读可能数组没满,把最后一点自己刷新进数组 } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ if(bos != null){//使用缓冲流关流只需要关闭缓冲流,相关的字节流或字符流会自动关闭 try { bos.close(); } catch (Exception e2) { e2.printStackTrace(); } } if(bis != null){ try { bis.close(); } catch (Exception e2) { e2.printStackTrace(); } } } long end = System.currentTimeMillis(); System.err.println(end - start); }
缓冲字符流使用实例:(在读取和写入字符的时候,除了支持读写字符,流还提供了读写一行字符串,readLine和write(String)具体见注释):
public void testFileReaderWriter(){//使用字符流实现复制功能 BufferedReader reader = null; BufferedWriter writer = null; try { File file = new File("readerwriter.txt"); File file1 = new File("writer21.txt"); FileReader fr = new FileReader(file); FileWriter fw = new FileWriter(file1); reader = new BufferedReader(fr); writer = new BufferedWriter(fw); String str; while((str = reader.readLine()) != null){ writer.write(str); writer.newLine();//或是writer.write(str+"\n");因为程序读取不会自动换行导致数据写到一行 writer.flush(); } /*char[] c = new char[500]; int len; while((len = reader.read(c)) != -1){ writer.write(c, 0, len); }*///另外提供了读取行 } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ if(writer != null){ try { writer.close(); } catch (Exception e2) { e2.printStackTrace(); }finally{ if(reader != null){ try { reader.close(); } catch (Exception e3) { e3.printStackTrace(); } } } } } }
转换流:InputStreamReader/OutputStreamWriter(用于字节流和字符流之间的转换,比如读取文本文件使用字符流效率比较高,如果读取到的是字节流,就可以做转换,使用频率并不是很高。解码:字符数组->字符串;编码:字符串->字符数组)
程序实例:
/** 如何实现字节流和字符流之间的转换 * 转换流:InputStreamReader OutputStreamReader * 解码:字符数组->字符串 * 编码:字符串->字符数组 */ public void testSwitchStream(){ //解码 BufferedReader br = null; BufferedWriter bw = null; try { File file = new File("writer.txt"); FileInputStream fis = new FileInputStream(file); InputStreamReader isr = new InputStreamReader(fis,"UTF-8"); br = new BufferedReader(isr); //编码 File file1 = new File("switch.txt"); FileOutputStream fos = new FileOutputStream(file1); OutputStreamWriter osw = new OutputStreamWriter(fos, "UTF-8"); bw = new BufferedWriter(osw); String str; while((str = br.readLine()) != null){ bw.write(str); bw.newLine(); bw.flush(); } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ if(bw != null){ try { bw.close(); } catch (Exception e2) { e2.printStackTrace(); } } if(br != null){ try { br.close(); } catch (Exception e2) { e2.printStackTrace(); } } } }
标准输入输出流:(了解)
打印流:PrintStream/PrintWriter()
数据流:DataInputStream/DataOutputStream

数据流使用实例:
package com.review; import java.io.DataInputStream; import java.io.DataOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import org.junit.Test; public class DataStream { @Test public void testDataOutputStream() { DataOutputStream dos = null; try { dos = new DataOutputStream(new FileOutputStream(new File("data.txt"))); dos.writeUTF("你是不是傻,dhdh傻叉!"); dos.writeBoolean(true); dos.writeLong(34567890); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } finally { if (dos != null) { try { dos.close(); } catch (Exception e2) { e2.printStackTrace(); } } } } @Test public void testInputStram(){ DataInputStream dis = null; try { dis = new DataInputStream(new FileInputStream(new File("data.txt"))); /*byte[] b = new byte[4];//这样读读出来是乱码,通过下面方式读才没问题,相当于暗文 int len; while((len=dis.read(b)) != -1){ System.out.print(new String(b, 0, len)); }*/ System.out.println(dis.readUTF()); System.out.println(dis.readBoolean()); System.out.println(dis.readLong()); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ if(dis != null){ try { dis.close(); } catch (Exception e2) { e2.printStackTrace(); } } } } }

基本数据类型用数据流传输,引用数据类型就用对象流来进行传输:
对象流(涉及序列化、反序列化):ObjectInputStream/ObjectOutputStream:
用于存储和读取对象的处理流,强大之处就是可以把Java中的对象写入到数据源中,也可以把对象从数据源中还原回来。
序列化(Serialize):用ObjectOutputStream类将一个java对象写入IO流中;
反序列化(Deserilize):用ObjectInputStream类从IO流中恢复该Java对象。
程序实例:
package com.qimeng; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.io.Serializable; import org.junit.Test; public class TestObjectInputOutputStream { @Test //反序列化过程,将硬盘中的文件通过ObjectInputStream转换位相应的对象 public void testObjectInputStream(){ ObjectInputStream ois = null; try { ois = new ObjectInputStream(new FileInputStream("person.txt")); Person p1 = (Person)ois.readObject(); System.out.println(p1); Person p2 = (Person)ois.readObject(); System.out.println(p2); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (ClassNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ if(ois != null){ try { ois.close(); } catch (IOException e) { e.printStackTrace(); } } } } @Test //对象的序列化过程,将内存中的对象通过ObjectOutputStream转换为二进制流,存在硬盘中 public void testObjectOutputStream(){ Person p1 = new Person("小米", 23); Person p2 = new Person("红米", 21); ObjectOutputStream oos = null; try { oos = new ObjectOutputStream(new FileOutputStream("person.txt")); oos.writeObject(p1); oos.flush(); oos.writeObject(p2); oos.flush(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } finally{ if(oos != null){ try { oos.close(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } } } /** * 要实现序列化的类 * 1、要求此类是可序列化的,所以必须实现Serializable或Extenalizable让此类可序列化 * 2、要求类的属性同样要实现Serializable接口。Serializable * 3、提供一个版本号 * 4、这两个对象流不能序列化static和transient修饰的成员变量 */ class Person implements Serializable{ private static final long serialVersionUID = 1807922889872508207L;//建议显示标注 String name; Integer age; public Person(String name, Integer age) { this.name = name; this.age = age; } @Override public String toString() { return "Person [name=" + name + ", age=" + age + "]"; } }
serialVersionUID用来表明类的不同版本间的兼容性,如果类没有显示定义这个静态变量,它的值是java运行时环境根据类的内部细节自动生成的,若类的源代码做了修改,serialVersionUID可能发生改变,所以建议显示声明。
随机存取文件流:RandomAccessFile类支持“随机访问”的方式,程序可以直接跳到文件的任意地方来读写文件;也可向已存在的文件后追加内容;支持只访问文件的部分内容。
具体详细用法见程序实例:
package com.qimeng; import java.io.File; import java.io.FileNotFoundException; import java.io.IOException; import java.io.RandomAccessFile; import org.junit.Test; /** * RandomAccessFile:支持随机访问 * 1、既可以充当输入流,也可以充当输出流 * 2、支持从任意位置读取、写入(插入) */ public class TestRandomAccessFile { @Test //相较于test3,更通用 public void test4(){ RandomAccessFile raf = null; try { raf = new RandomAccessFile(new File("hello.txt"), "rw"); raf.seek(4);//定位到d的指针 byte[] b = new byte[10]; int len; StringBuffer sb = new StringBuffer(); while((len = raf.read(b)) != -1){ sb.append(new String(b, 0, len)); } raf.seek(4); raf.write("xy".getBytes()); raf.write(sb.toString().getBytes()); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ if(raf != null){ try { raf.close(); } catch (IOException e) { e.printStackTrace(); } } } } @Test //实现插入的效果,向字符串abcdef123456的d后面插入“xy” public void test3(){ RandomAccessFile raf = null; try { raf = new RandomAccessFile(new File("hello.txt"), "rw"); raf.seek(4);//定位到d的指针 String str = raf.readLine();//表示读取了d后面的内容。此时指针在6后面,可以用getFilePointer查看 /*long f = raf.getFilePointer(); System.out.println(f);*/ raf.seek(4);//把指针调回去 raf.write("xy".getBytes()); raf.write(str.getBytes()); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ if(raf != null){ try { raf.close(); } catch (IOException e) { e.printStackTrace(); } } } } @Test //向指定位置写入,实际上是覆盖指定位置的字符 public void test2(){ RandomAccessFile raf = null;; try { raf = new RandomAccessFile(new File("hello.txt"), "rw"); raf.seek(3);//从0开始,3代表从第四个字符后面开始写 raf.write("xy".getBytes()); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ if(raf != null){ try { raf.close(); } catch (Exception e2) { e2.printStackTrace(); } } } } //进行文件的读写 @Test public void test1(){ RandomAccessFile raf1 = null; RandomAccessFile raf2 = null; try { raf1 = new RandomAccessFile(new File("hello.txt"), "r"); raf2 = new RandomAccessFile(new File("hello1.txt"), "rw"); byte[] b = new byte[5]; int len; while((len = raf1.read(b)) != -1){ raf2.write(b, 0, len); } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ try { raf2.close(); } catch (IOException e) { e.printStackTrace(); } try { raf1.close(); } catch (IOException e) { e.printStackTrace(); } } } }
具体分类:

:
相关文章推荐
- java提高篇(一) java知识汇总-------io流知识汇总(io学习流程)
- java提高篇(一)拓展篇 java知识汇总---IO流的使用规律总结(含代码示例)浅显易懂
- Java基础知识部分汇总
- 转:Java基础知识部分汇总
- 再学java基础(6)关于 java IO 知识汇总。
- java知识:增强for循环应用汇总
- 黑马程序员_java初级基础知识汇总(常量和变量、语句、函数、数组)
- Java面试题目汇总/英文Java面试题(核心知识)
- 黑马程序员Java基础知识学习部分汇总
- Java中的IO流知识总结
- VB.Net C# 和Java知识汇总(一)
- Java基础知识——IO流(一)
- Java基础知识部分汇总
- Java中的IO流知识总结(转)
- Java中的IO流知识总结(转)
- Serializable java序列化知识汇总
- Java基础知识部分汇总
- Java中的IO流知识总结 【转】
- JAVA基础知识汇总
- Java小知识汇总