Java数组实现可以动态增长的队列
2016-09-28 20:05
417 查看
队列是一种先进先出的数据结构,由队尾进入,由队头删除,类似于现实中的排队。用Java数组实现队列,用first和last分别指向队头和队尾,用storage数组存储元素,但是需要注意的是不同于堆栈,数组中的元素不一定全是队列中的元素,因为如果是一般的数组,first在最前面,last在最后,可能会出现first和last都在数组最后指向一个元素(整个队列只有一个元素),不能增加元素,但是前面的空间没法利用的情况。所以这里采用环形数组实现。另外考虑到队列容量需求会动态增加这里采用了动态数组实现队列扩容。关于数组容量增加请参考:数组容量动态增加。注意,删除队头元素,不是真正的删除,而是将元素排除在first和last索引之外,只有数组容量不够时,删除的元素才会被新元素覆盖。
代码如下:
运行程序如下:
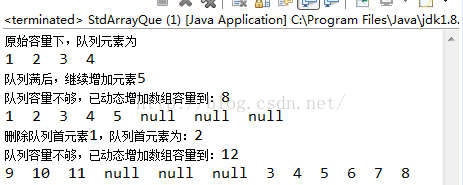
参考来源:数据结构与算法 Adam Drozdek
代码如下:
interface StdQueue<e> { public boolean isEmpty(); public boolean isFull(); public void enQueue(E el); public Object deQueue(); public void printAll(); void enLarge(); } class StdArrayQue<e> implements StdQueue<e> { int first,last; Object[] storage; int inicap=4,step=4;//队列初始容量和增加时的步长 public StdArrayQue() { first=last=-1; storage=new Object[inicap]; } public boolean isEmpty() { return first==-1; } @Override public void enQueue(E el) { if(isFull()) { enLarge(); System.out.println("队列容量不够,已动态增加数组容量到:"+storage.length); } if(last==storage.length-1||first==-1) { storage[0]=el; last=0; if(first==-1) first=0; } else storage[++last]=el; } @Override public boolean isFull() { return (first==0&&last==storage.length-1)||(first==last+1); } @Override public Object deQueue() { // TODO Auto-generated method stub Object temp=storage[first]; if(first==last) first=last=-1; else if(first==storage.length-1) first=0; else first++; return temp; } @Override public void printAll() { for(Object i:storage) System.out.print(i+" "); System.out.println(); } @Override public void enLarge() { Object[] a=new Object[storage.length+step]; if(first==0&&last==storage.length-1) System.arraycopy(storage, 0, a, 0, storage.length); else { System.arraycopy(storage, 0, a, 0, last+1); System.arraycopy(storage, first, a, a.length-storage.length+first, storage.length-first); } storage=a; } public static void main(String[] args) { StdArrayQue<integer> se=new StdArrayQue<>(); se.enQueue(1); se.enQueue(2); se.enQueue(3); se.enQueue(4); System.out.println("原始容量下,队列元素为"); se.printAll(); System.out.println("队列满后,继续增加元素5"); se.enQueue(5); se.printAll(); se.deQueue(); System.out.println("删除队列首元素1,队列首元素为:"+se.storage[se.first]); se.deQueue(); se.enQueue(6); se.enQueue(7); se.enQueue(8); se.enQueue(9); se.enQueue(10); se.enQueue(11); se.printAll(); } } </string>
运行程序如下:
参考来源:数据结构与算法 Adam Drozdek
相关文章推荐
- Java之Vector向量类实现自动动态增长的对象数组-类似动态数组
- 算法(第四版)笔记<一>-------动态队列的数组实现(Java语言)
- 队列--基于动态循环数组实现(Java)
- 算法(第四版)学习笔记之java实现可以动态调整数组大小的栈
- Java数组反射实现动态的判断一个对象书否是数组,并且对数组进行拆包输出 。。
- 动态数组实现循环队列
- java反射实现动态扩建数组
- Java数组实现循环队列
- java使用数组和链表实现队列示例
- Java实现队列二:通过数组方式实现
- 使用数组实现栈和循环队列(JAVA语言)
- 用java实现单位的跟踪 , 稍加升级就可以实现两个单位的动态跟随
- Java数组实现循环队列
- java实现队列(数组方式)
- java实现动态智能数组,将旧数组copy到新数组
- 用Java数组实现队列
- java 基于数组实现的队列
- 实现AMF3与Java之间数组的传递(动态创建数组)...
- Java核心技术很优美的代码3 实现数组的动态分配
- C语言队列动态数组实现