java连接MySQL版本的电话本管理系统
2016-08-27 11:28
573 查看
最近老师讲到了JDBC,以电话簿为例,系统的整理一下
要求:
1.创建数据库test中,创建一张名为telephone的表
2.telephone包含的字段有ID(主键自增)、Name、Sex、Age、Telephone、QQ、Address
3.使用程序完成增删改查的功能。
4.具体实现方式仿照8月26日课堂案例。
5.要求:使用PreparedStatement来完成
(1)打开Navicat连接MySQL在test数据库下新建如下图的表:
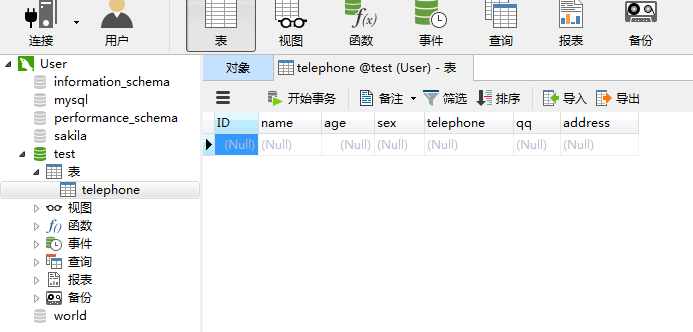
(2)建好工程后记得导入mySQL-connector的jar包
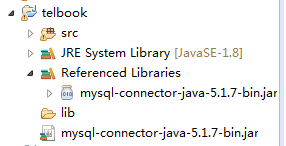
程序实现后控制台如图所示:
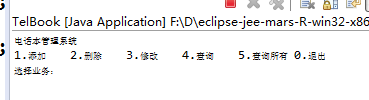
(3)建一个telephonebook的类,封装好
(4)新建一个TelManager类,里面存放各种方法
JDBC编程步骤为:
装载驱动程序
建立连接
操作语句
释放资源
JDBC访问数据库的流程:
加载驱动程序Driver;
通过DriverManager类获得表示数据库连接的Connection类(连接接口)对象;
通过Connection对象绑定要执行的语句,生成Statement类(语句对象接口)对象;
执行SQL语句,接收执行结果集ResultSet;
可选的对结果集ResultSet类对象的处理;
必要的关闭ResultSet、Statement和Connection
电话本的添加方法
这里使用PreparedStatement,先来讲一下与Statement的区别:
PreparedStatement是Statement的子接口,它的实例对象可以通过调用Connection.preparedStatement(sql)方法获得,相对于Statement对象而言:PreperedStatement可以避免SQL注入的问题。
Statement会使数据库频繁编译SQL,可能造成数据库缓冲区溢出。PreparedStatement 可对SQL进行预编译,从而提高数据库的执行效率
并且PreperedStatement对于sql中的参数,允许使用占位符的形式进行替换,简化sql语句的编写。
删除方法:
首先根据ID查询,查询返回的是电话本对象,如果对象为null则不存在,否则执行删除方法
修改: 在主方法中,也是要先根据ID查询再修改
查询全部:
需要遍历所有数据,所以要建一个arraylist数组,将数据存入数组,然后再逐个遍历输出
关闭ResultSet、Statement和Connection方法
(5)建一个TelBook类,作为程序运行的入口
结果如下:通过在控制台的增删改查来操作数据库的数据
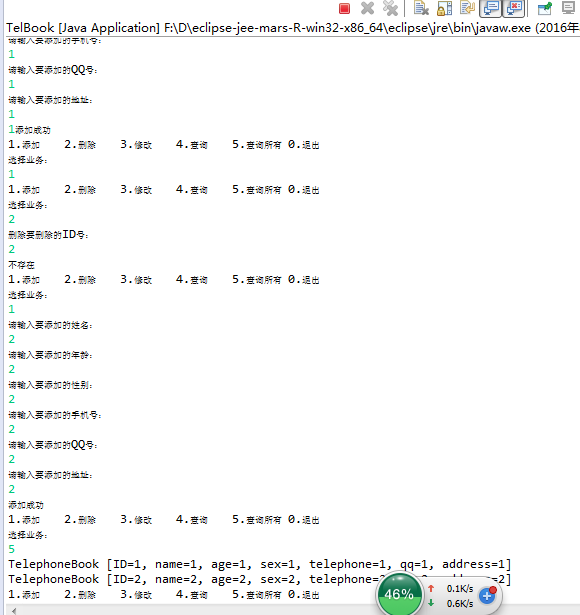
要求:
1.创建数据库test中,创建一张名为telephone的表
2.telephone包含的字段有ID(主键自增)、Name、Sex、Age、Telephone、QQ、Address
3.使用程序完成增删改查的功能。
4.具体实现方式仿照8月26日课堂案例。
5.要求:使用PreparedStatement来完成
(1)打开Navicat连接MySQL在test数据库下新建如下图的表:
(2)建好工程后记得导入mySQL-connector的jar包
程序实现后控制台如图所示:
(3)建一个telephonebook的类,封装好
public class TelephoneBook { private int ID; private String name; private int age; private String sex; private String telephone; private String qq; private String address; public int getID() { return ID; } public void setID(int iD) { ID = iD; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getSex() { return sex; } public void setSex(String sex) { this.sex = sex; } public String getTelephone() { return telephone; } public void setTelephone(String telephone) { this.telephone = telephone; } public String getQq() { return qq; } public void setQq(String qq) { this.qq = qq; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } @Override public String toString() { return "TelephoneBook [ID=" + ID + ", name=" + name + ", age=" + age + ", sex=" + sex + ", telephone=" + telephone + ", qq=" + qq + ", address=" + address + "]"; } public TelephoneBook(int iD, String name, int age, String sex, String telephone, String qq, String address) { super(); ID = iD; this.name = name; this.age = age; this.sex = sex; this.telephone = telephone; this.qq = qq; this.address = address; } public TelephoneBook() { super(); } }
(4)新建一个TelManager类,里面存放各种方法
JDBC编程步骤为:
装载驱动程序
建立连接
操作语句
释放资源
JDBC访问数据库的流程:
加载驱动程序Driver;
通过DriverManager类获得表示数据库连接的Connection类(连接接口)对象;
通过Connection对象绑定要执行的语句,生成Statement类(语句对象接口)对象;
执行SQL语句,接收执行结果集ResultSet;
可选的对结果集ResultSet类对象的处理;
必要的关闭ResultSet、Statement和Connection
//获取Connection的方法 public static Connection getConnection(){ String driver="com.mysql.jdbc.Driver";//mysql的Driver String url="jdbc:mysql://localhost:3306/test";//数据库的位置 String user="root"; String password="123456"; Connection conn=null;//获取Connection try { Class.forName(driver);//加载驱动 conn=DriverManager.getConnection(url,user,password); } catch (ClassNotFoundException e) { e.printStackTrace(); } catch (SQLException e) { e.printStackTrace(); } return conn;//返回连接 }
电话本的添加方法
这里使用PreparedStatement,先来讲一下与Statement的区别:
PreparedStatement是Statement的子接口,它的实例对象可以通过调用Connection.preparedStatement(sql)方法获得,相对于Statement对象而言:PreperedStatement可以避免SQL注入的问题。
Statement会使数据库频繁编译SQL,可能造成数据库缓冲区溢出。PreparedStatement 可对SQL进行预编译,从而提高数据库的执行效率
并且PreperedStatement对于sql中的参数,允许使用占位符的形式进行替换,简化sql语句的编写。
//向数据库中插入信息,参数为电话本对象 public static int add(TelephoneBook tel ){ int row=0; String sql="insert into telephone(name,age,sex,telephone,qq,address) values (?,?,?,?,?,?)"; //SQl的添加语句 ?为占位符 数据库中id是自增的 Connection conn=null;// 获取连接 PreparedStatement ps=null;//// 创建PreparedStatement对象 try { conn = getConnection(); ps = (PreparedStatement) conn.prepareStatement(sql); ps.setString(1,tel.getName());//给sql语句中的占位符?赋值 ps.setInt(2,tel.getAge()); ps.setString(3, tel.getSex()); ps.setString(4,tel.getTelephone()); ps.setString(5,tel.getQq()); ps.setString(6,tel.getAddress()); row=ps.executeUpdate();// 执行sql语句 } catch (SQLException e) { e.printStackTrace(); } finally{ closePrepareStatement(ps);//关闭方法,见下面 closeConnection(conn); } return row; }
删除方法:
首先根据ID查询,查询返回的是电话本对象,如果对象为null则不存在,否则执行删除方法
public static TelephoneBook selectById(int id){ String sql="select *from telephone where id=?";//查询sql语句 ResultSet rs=null;//结果集对象, Connection conn=null; PreparedStatement ps=null; TelephoneBook t=null;//电话本对象用于接收查询的对象,返回 try { conn=getConnection(); ps=(PreparedStatement) conn.prepareStatement(sql); ps.setInt(1, id); rs=ps.executeQuery();//执行查询,返回的类型为ResultSet,如果数据库中有数据,rs.next()返回true while(rs.next()){ t=new TelephoneBook(); t.setID(rs.getInt(1)); t.setName(rs.getString(2)); t.setAge(rs.getInt(3)); t.setSex(rs.getString(4)); t.setTelephone(rs.getString(5)); t.setQq(rs.getString(6)); t.setAddress(rs.getString(7)); } } catch (SQLException e) { e.printStackTrace(); } finally{ closeResultSet(rs);//关闭方法,见最下面 closePrepareStatement(ps); closeConnection(conn); } return t; } //根据ID删除 public static int delete(int id){ String sql="delete from telephone where id=?";//删除sql语句 Connection conn=null; int row=0; PreparedStatement ps=null; try { conn=getConnection(); ps=(PreparedStatement) conn.prepareStatement(sql); ps.setInt(1,id); row=ps.executeUpdate();//执行sql语句,返回值为操作的行数 } catch (SQLException e) { e.printStackTrace(); } finally{ closePrepareStatement(ps); closeConnection(conn); } return row; }
修改: 在主方法中,也是要先根据ID查询再修改
//修改数据,所以传入的参数应该是电话本对象 public static int update(TelephoneBook tel){ int row=0; String sql="update telephone set name=?,age=?,sex=?,telephone=?,qq=?,address=? where id=?"; Connection conn=null; PreparedStatement ps=null; try { conn = getConnection(); ps = (PreparedStatement) conn.prepareStatement(sql); ps.setString(1,tel.getName()); ps.setInt(2,tel.getAge()); ps.setString(3, tel.getSex()); ps.setString(4,tel.getTelephone()); ps.setString(5, tel.getQq()); ps.setString(6, tel.getAddress()); ps.setInt(7,tel.getID()); row=ps.executeUpdate(); } catch (SQLException e) { e.printStackTrace(); } finally{ closePrepareStatement(ps); closeConnection(conn); } return row; }
查询全部:
需要遍历所有数据,所以要建一个arraylist数组,将数据存入数组,然后再逐个遍历输出
public static List<TelephoneBook> selectAll(){ List<TelephoneBook> list=new ArrayList<TelephoneBook>();//建一个arraylist数组 String sql="select *from telephone"; Connection conn=null; PreparedStatement ps=null; ResultSet rs=null; try { conn = getConnection(); ps = (PreparedStatement) conn.prepareStatement(sql); rs = ps.executeQuery();//执行查询 while(rs.next()){ TelephoneBook tel=new TelephoneBook(); //存在一条数据就新建一个电话本对象,得到相应属性 tel.setID(rs.getInt(1)); tel.setName(rs.getString(2)); tel.setAge(rs.getInt(3)); tel.setSex(rs.getString(4)); tel.setTelephone(rs.getString(5)); tel.setQq(rs.getString(6)); tel.setAddress(rs.getString(7)); list.add(tel);//添加一条数据 } } catch (SQLException e) { e.printStackTrace(); } finally{ closeResultSet(rs); closePrepareStatement(ps); closeConnection(conn); } return list; }
关闭ResultSet、Statement和Connection方法
//关闭Connection public static void closeConnection(Connection conn){ if(conn!=null){ try { conn.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } //关闭Statement public static void closePrepareStatement(PreparedStatement ps){ if(ps!=null){ try { ps.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } //关闭ResultSet public static void closeResultSet(ResultSet rs){ if(rs!=null){ try { rs.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
(5)建一个TelBook类,作为程序运行的入口
public class TelBook { static Scanner sc=new Scanner(System.in); public static void main(String[] args) { System.out.println("电话本管理系统"); while(true){ System.out.println("1.添加\t2.删除\t3.修改\t4.查询\t5.查询所有\t0.退出"); System.out.println("选择业务:"); int n=sc.nextInt(); switch (n) { case 1: //1.添加 System.out.println("请输入要添加的姓名:"); String name=sc.next(); System.out.println("请输入要添加的年龄:"); int age=sc.nextInt(); System.out.println("请输入要添加的性别:"); String sex=sc.next(); System.out.println("请输入要添加的手机号:"); String phone=sc.next(); System.out.println("请输入要添加的QQ号:"); String qq=sc.next(); System.out.println("请输入要添加的地址:"); String address=sc.next(); TelephoneBook t=new TelephoneBook(); t.setName(name); t.setAge(age); t.setAddress(address); t.setQq(qq); t.setTelephone(phone); t.setSex(sex); int row=TelManager.add(t); if(row>0){ System.out.println("添加成功"); }else{ System.out.println("添加失败"); } break; case 2://删除 System.out.println("删除要删除的ID号:"); int ID=sc.nextInt(); TelephoneBook t1=TelManager.selectById(ID);// 判断id所对应的电话记录是否存在 if(t1!=null){ System.out.println("确定要删除么?(确定选1,取消选0)"); int select=sc.nextInt(); if(select==1){ int row1=TelManager.delete(ID); if(row1>0){ System.out.println("删除成功"); } else{ System.out.println("删除失败"); } }else{ break; } }else{ System.out.println("不存在"); } break; case 3://修改 System.out.println("请输入要修改人的ID:"); int ID1=sc.nextInt(); TelephoneBook tb=TelManager.selectById(ID1););// 判断id所对应的电话记录是否存在 System.out.println(tb); if(tb!=null){ System.out.println("输入要更新人的姓名:"); String newName=sc.next(); System.out.println("输入要更新人的年龄:"); int newAge=sc.nextInt(); System.out.println("输入要更新人的性别:"); String newSex=sc.next(); System.out.println("输入要更新人的电话:"); String newPhone=sc.next(); System.out.println("输入要更新人的qq:"); String newqq=sc.next(); System.out.println("输入要更新人的地址:"); String newAddress=sc.next(); tb.setID(ID1); tb.setName(newName); tb.setAge(newAge); tb.setSex(newSex); tb.setTelephone(newPhone); tb.setQq(newqq); tb.setAddress(newAddress); int rows=TelManager.update(tb); if(rows>0){ System.out.println("更新成功"); System.out.println(tb); }else{ System.out.println("更新失败"); } } else{ System.out.println("不存在"); } break; case 4://4.查询 System.out.println("请输入要查询的ID号:"); int id1=sc.nextInt(); TelephoneBook tb1=TelManager.selectById(id1); if(tb1!=null){ System.out.println(tb1); System.out.println("查询成功"); }else{ System.out.println("查询失败"); } break; case 5://查询全部 List<TelephoneBook> list=TelManager.selectAll(); for(TelephoneBook s:list){ System.out.println(s); } break; case 0: System.out.println("退出程序"); System.exit(0); } } } }
结果如下:通过在控制台的增删改查来操作数据库的数据
相关文章推荐
- Java初学者:图书管理小工具(MySQL版本)代码
- 连接Ubuntu系统服务器Mysql出错(错误:10061)/Jdbc连接远程数据库出错(java.net.ConnectException: Connection refused: connect)
- Java版本:图书管理系统
- Java连接Oracle数据库开发银行管理系统【二、设计篇】
- java剧院管理系统,数据存储mysql
- 【java实例】自己写的mysql数据库管理系统————MANAGER_FOR_MYSQL-1.0
- Java+MySQL实现学生信息管理系统源码
- 【自学笔记】简单java电话本管理系统v1.0源码
- Java+Mysql学生管理系统源码
- 职业生涯管理系统 servlet+javabean+mysql(一)软件的安装与配置
- Java做信息管理系统的数据库连接方法
- JAVA实现电话本管理系统
- java版本的学生管理系统
- Java版本 学生管理系统
- Visual Studio 连接 Mysql 实现一个选课管理系统--->提取数据的几种方法
- java内存和mysql连接管理的问题
- ictclas分词系统的使用,java调用,附带连接mysql进行读写。
- Java面向对象练习-电话本管理系统
- Java连接Oracle数据库开发银行管理系统【一、需求篇】
- Java成绩管理系统控制台版本