Java 编写简单的服务器
2016-08-22 20:41
253 查看
结果图:
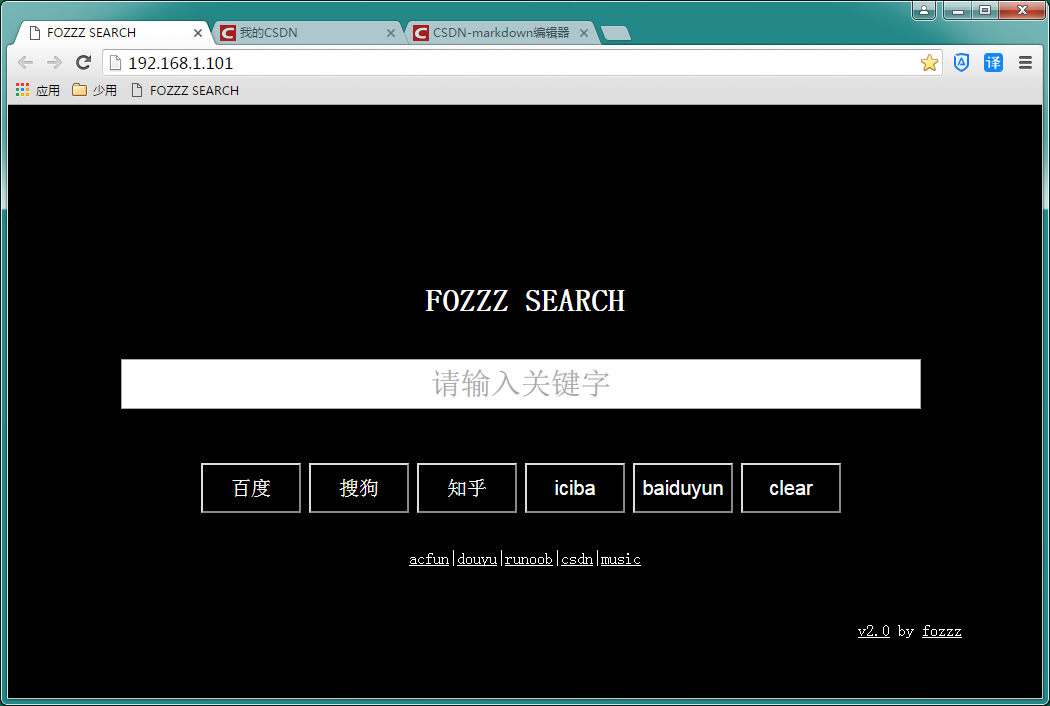
服务器功能主要是
–网页服务(访问网络地址,返回网页)
–收藏夹功能(读取其他文件,添加到服务器输出流中)
服务器功能主要是
–网页服务(访问网络地址,返回网页)
–收藏夹功能(读取其他文件,添加到服务器输出流中)
------------start.bat----------------- //启动服务器快捷方式 //默认放在D盘mysearch文件夹下 d: cd mysearch java com.fozzz.server.Server
--------add.txt------------ //收藏夹功能,放在d盘mysearch文件夹下 <a href="http://www.acfun.tv" target="_blank">acfun</a> <a>|</a> <a href="http://www.douyu.com" target="_blank">douyu</a> <a>|</a> <a href="http://www.runoob.com" target="_blank">runoob</a> <a>|</a> <a href="http://www.csdn.net" target="_blank">csdn</a> <a>|</a> <a href="http://music.163.com" target="_blank">music</a>
--------------服务器代码----------------- package com.fozzz.server; import java.io.BufferedReader; import java.io.File; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.io.PrintWriter; import java.net.ServerSocket; import java.net.Socket; public class Server { public static void main(String[] args) { // TODO Auto-generated method stub try { String path = "d:" + File.separator + "mysearch" + File.separator; //默认端口80 ServerSocket serverSocket = new ServerSocket(80); //服务器一直运行 while (true) { Socket accept = serverSocket.accept(); InputStream inputStream = accept.getInputStream(); // 得到请求链接 String url = new BufferedReader(new InputStreamReader(inputStream)).readLine(); String html = ""; try { //打得到请求网页名 html = url.split(" ")[1]; } catch (Exception e) { continue; } //设置默认网页为index.html if (html.equals("/")) { html = "index.html"; } //不处理图片请求 if (html.equals("/favicon.ico")) { continue; } String newPath = path + html; // 读取服务器本地文件 File file = new File(newPath); BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(new FileInputStream(file))); PrintWriter printWriter = new PrintWriter(accept.getOutputStream()); String readLine = null; //html标准头部 printWriter.write("HTTP/1.1 200 OK\r\n"); printWriter.write("Content-type:text/html;charset=UTF-8\r\n"); printWriter.write("\r\n"); //一行一行读取文件并输出到网页 while ((readLine = bufferedReader.readLine()) != null) { printWriter.write(readLine); //网页设置了收藏夹功能添加的位置 if (readLine.equals(" <!--add 4000 -->")) { // 读取add.txt文件内容 String addPath = path + "add.txt"; File addFile = new File(addPath); BufferedReader addFileReader = new BufferedReader( new InputStreamReader(new FileInputStream(addFile))); String readLine2 = null; while ((readLine2 = addFileReader.readLine()) != null) { System.out.println(readLine2); printWriter.write(readLine2); } addFileReader.close(); } } printWriter.flush(); printWriter.close(); bufferedReader.close(); accept.close(); } } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
----------------主页面--------------- <!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>FOZZZ SEARCH</title> <!--<link rel="icon" href="img/search.ico" type="image/x-icon" />--> <style> body { background-color: black; margin-left: 80px; margin-right: 80px; } .center { text-align: center; margin-top: 150px; } input { height: 50px; } .mytext { width: 800px; size: 500px; font-size: 30px; text-align: center; } .mybutton { width: 100px; font-size: 20px; color: #FFFFFF; cursor: pointer; background-color: #000000; } .mybutton:hover { color: #000000; background-color: #FFFFFF; border-color: #000000; } .myfoot { text-align: right; color: #ffffff; cursor: default; } a { color: #FFFFFF; } h1 { color: #FFFFFF; cursor: default; } </style> </head> <body> <div class="center"> <h1>FOZZZ SEARCH</h1><br /> <input type="text" class="mytext" id="keyword" value="" placeholder="请输入关键字" autofocus="autofocus" /> <br /><br /><br /><br /> <!-- 作者:lzslov@qq.com 时间:2016-07-16 描述:button --> <input type="button" class="mybutton" name="" id="baidu" value="百度" /> <input type="button" class="mybutton" name="" id="sougou" value="搜狗" /> <input type="button" class="mybutton" name="" id="zhihu" value="知乎" /> <input type="button" class="mybutton" name="" id="iciba" value="iciba" /> <input type="button" class="mybutton" name="" id="baiduyun" value="baiduyun" /> <input type="button" class="mybutton" name="" id="clear" value="clear" /> <br /><br /><br /> <!--add--> </div> <!-- 作者:lzslov@qq.com 时间:2016-07-16 描述:foot --> <div class="myfoot"> <br /><br /><br /> <a href="version.html" target="_blank">v2.0</a> by <a href="qq:1239031206">fozzz</a> </div> </body> <!-- 作者:lzslov@qq.com 时间:2016-07-16 描述:js --> <script> var baidu = "https://www.baidu.com/s?ie=UTF-8&wd="; var sougou = "https://www.sogou.com/web?query="; var zhihu = "https://m.zhihu.com/search?type=content&q="; var iciba = "http://www.iciba.com/"; var baiduyun = "http://www.sobaidupan.com/search.asp?wd="; document.getElementById("baidu").onclick = function() { var keyword = document.getElementById("keyword").value; window.open(baidu + keyword); }; document.getElementById("sougou").onclick = function() { var keyword = document.getElementById("keyword").value; window.open(sougou + keyword); }; document.getElementById("zhihu").onclick = function() { var keyword = document.getElementById("keyword").value; window.open(zhihu + keyword); }; document.getElementById("iciba").onclick = function() { var keyword = document.getElementById("keyword").value; window.open(iciba + keyword); }; document.getElementById("baiduyun").onclick = function() { var keyword = document.getElementById("keyword").value; window.open(baiduyun + keyword); }; cfa2 document.getElementById("clear").onclick = function() { document.getElementById("keyword").value = ""; document.getElementById("keyword").focus(); }; document.getElementById("keyword").onkeypress = function(event) { if(event.keyCode == 13) { var keyword = document.getElementById("keyword").value; window.open(sougou + keyword); } }; </script> </html>
----------------版本页面--------------- <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>VERSION</title> <!--<link rel="icon" href="img/search.ico" type="image/x-icon" />--> <style> body { background-color: #000000; margin-right: 50px; } table { color: #FFFFFF; font-size: 20px; } .center { text-align: center; } .myleft{ text-align: left; } </style> </head> <body> <div class="center"> <table border="0" width="100%" cellspacing="20" cellpadding="8"> <tr> <th>VERSION</th> <th class="myleft">UPDATE CONTENT</th> <th>TIME</th> </tr> <tr> <td>1.0</td> <td class="myleft">初建index,version页面<br />index页面:百度,搜狗,知乎搜索功能</td> <td width="10%">2016-07-16</td> </tr> <tr> <td>1.1</td> <td class="myleft">index页面:金山词霸,clear功能</td> <td>2016-07-17</td> </tr> <tr> <td>1.2</td> <td class="myleft">index页面:输入框enter键直接进入搜狗搜索功能</td> <td>2016-07-17</td> </tr> <tr> <td>1.3</td> <td class="myleft">index页面:优化知乎网页为手机页面</td> <td>2016-07-18</td> </tr> <tr> <td>1.4</td> <td class="myleft">index页面:百度云资源搜索功能</td> <td>2016-08-11</td> </tr><tr> <td>2.0</td> <td class="myleft">收藏夹功能,运行在java服务器上</td> <td>2016-08-21</td> </tr> </table> </div> </body> </html>
相关文章推荐
- JAVA编写最简单的服务器
- JAVA编写的一个简单的Socket实现的HTTP响应服务器
- JAVA编写的一个简单的Socket实现的HTTP响应服务器进阶版
- JAVA编写的一个简单的Socket实现的HTTP响应服务器
- JAVA 编写一个多线程的简单Web服务器
- Java编写一个简单的TCP通信程序。服务器发送一条字符串,客户端接收该信息并显示。
- JAVA编写的一个简单的Socket实现的HTTP响应服务器
- JAVA编写的一个简单的Socket实现的HTTP响应服务器
- 简单的JAVA HTML服务器
- 用java实现的一个简单web服务器程序
- Java 利用套接字Socket实现简单的服务器与客户端通信
- java开发的一个简单的本地web服务器
- 基于tcp服务器的多线程版-java-简单
- 网络编程--简单实现javaftp服务器
- 简单的用 Java Socket 编写的 HTTP 服务器应用,帮助学习HTTP协议(支持POST信息打印)
- 想不到Java也能让我基于TCP/IP编写程序,还是如此简单!
- Java编写的简单的计算器
- 简单Java web服务器代码
- 利用socket编写简单的web 服务器
- 简单的用 Java Socket 编写的 HTTP 服务器应用,帮助学习HTTP协议