Spring编程式事务管理(xml注入)
2016-07-27 18:55
561 查看
利用Spring,模拟银行转账进行编程式事务管理。
需要的jar包如下图
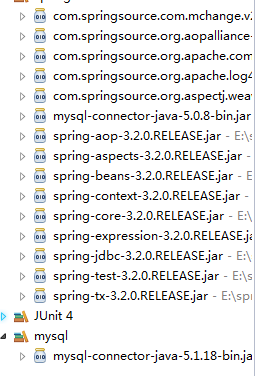
,对应的jar包在我的资源页有下载。项目名称叫spring-transaction
创建数据库和数据表,内容如下:
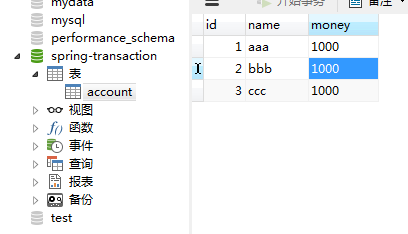
其中name:假定为用户的银行账号
money:为账号余额,初始化为1000
项目结构
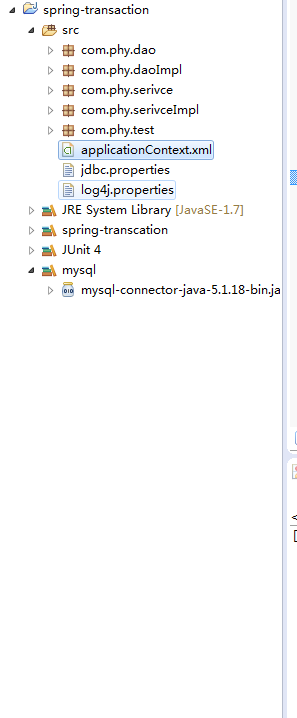
编写事务管理
编写Dao与DaoImpl
AccountDao.java
AccountDaoImpl.java
AccountServiceImpl.java
3.3 配置applicationContext.xml
jdbc.properies
xml文件中配置的${jdbc.driverClassName}与jdbc.properties中的属性名一一对应。
AccountTest.java
jar包资源:http://download.csdn.net/detail/s18579103565/9587919
源码资源:http://download.csdn.net/detail/s18579103565/9587942
需要的jar包如下图
,对应的jar包在我的资源页有下载。项目名称叫spring-transaction
创建数据库和数据表,内容如下:
其中name:假定为用户的银行账号
money:为账号余额,初始化为1000
项目结构
编写事务管理
编写Dao与DaoImpl
AccountDao.java
package com.phy.dao; /** * 转账案例的持久层接口 * @author zongjb * */ public interface AccountDao { /** * * @param out :为转出账户 * @param money :为转出金额 */ void out(String out,double money); /** * * @param in :转入账户 * @param money :转入金额 */ void in(String in,double money); }
AccountDaoImpl.java
package com.phy.daoImpl; import com.phy.dao.AccountDao; import org.springframework.jdbc.core.support.JdbcDaoSupport; /** * * @author zongjb * */ public class AccountDaoImpl extends JdbcDaoSupport implements AccountDao { @Override public void out(String out, double money) { String sql = "update account set money = money - ? where name = ?"; this.getJdbcTemplate().update(sql, money, out); } @Override public void in(String in, double money) { String sql = "update account set money = money + ? where name = ?"; this.getJdbcTemplate().update(sql, money,in); } }
3.2 Service与ServiceImpl AccountService.java
package com.phy.serivce; /** * 转账案例的业务处理层接口 * @author zongjb * */ public interface AccountService { /** * * @param out :转出账户 * @param in :转入账户 * @param money :转出(入)金额 */ void transfers(String out,String in,double money); }
AccountServiceImpl.java
package com.phy.serivceImpl; import org.springframework.transaction.TransactionStatus; import org.springframework.transaction.support.TransactionCallbackWithoutResult; import org.springframework.transaction.support.TransactionTemplate; import com.phy.dao.AccountDao; import com.phy.serivce.AccountService; /** * * @author zongjb * */ public class AccountServiceImpl implements AccountService { private AccountDao accountDao; private TransactionTemplate transactionTemplate; public void setTransactionTemplate(TransactionTemplate transactionTemplate) { this.transactionTemplate = transactionTemplate; } public void setAccountDao(AccountDao accountDao) { this.accountDao = accountDao; } @Override public void transfers(final String out, final String in,final double money) { ransactionTemplate.execute(new TransactionCallbackWithoutResult() { @Override protected void doInTransactionWithoutResult(TransactionStatus arg0) { accountDao.out(out, money); accountDao.in(in, money); } }); } }
3.3 配置applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd"> <!--(1)扫描存放在classpa 4000 th下,名为jdbc.properties的文件--> <context:property-placeholder location="classpath:jdbc.properties"/> <!-- (2) 配置c3p0连接池,取名为dataSource --> <bean name="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource"> <property name="driverClass" value="${jdbc.driverClassName}"/> <property name="jdbcUrl" value="${jdbc.url}"/> <property name="user" value="${jdbc.username}"/> <property name="password" value="${jdbc.password}"/> </bean> <bean name="accountService" class="com.phy.serviceImpl.AccountServiceImpl"> <property name="accountDao" ref="accountDao"/> <!-- (5)注入事务管理模板 --> <property name="transactionTemplate" ref="transactionTemplate"/> </bean> <bean name="accountDao" class="com.phy.daoImpl.AccountDaoImpl"> <property name="dataSource" ref="dataSource"/> </bean> <!--(3)配置事务管理器 --> <!-- 可以直接写name="dataSrouce",是因为在DataSourceTransactionManget类中有一个setDataSource方法,可以通过set方法直接注入 --> <bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <property name="dataSource" ref="dataSource"/> </bean> <!-- (4)配置事务管理的模板:Spring为了简化事务管理的代码而提供的类 --> <!-- 直接注入的理由同上 --> <bean id="transactionTemplate" class="org.springframework.transaction.support.TransactionTemplate"> <property name="transactionManager" ref="transactionManager"/> </bean> </beans>
jdbc.properies
jdbc.driverClassName=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql://localhost:3306:spring-transaction jdbc.username=root jdbc.password=123456
xml文件中配置的${jdbc.driverClassName}与jdbc.properties中的属性名一一对应。
AccountTest.java
package com.phy.test; import javax.annotation.Resource; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; import com.phy.serivce.AccountService; /** * 编程式事务管理 * @author zongjb * */ @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration("classpath:applicationContext.xml") public class AccountTest { @Resource(name="accountService") private AccountService accountService; @Test public void testAccount(){ accountService.transfers("aaa","bbb",200d); } }
jar包资源:http://download.csdn.net/detail/s18579103565/9587919
源码资源:http://download.csdn.net/detail/s18579103565/9587942
相关文章推荐
- 一个jar包里的网站
- 一个jar包里的网站之文件上传
- 一个jar包里的网站之返回对媒体类型
- Spring和ThreadLocal
- Spring Boot 开发微服务
- Spring AOP动态代理-切面
- 【DevOps】为什么我们永远疲于奔命?
- Spring整合Quartz(JobDetailBean方式)
- Spring整合Quartz(JobDetailBean方式)
- 网络管理之IP地址篇
- 文件的读出 编辑 管理
- SQL Server 2008 R2 应用及多服务器管理
- SQL Server触发器和事务用法示例
- SQL Server误区30日谈 第1天 正在运行的事务在服务器故障转移后继续执行
- 浅析SQL Server中包含事务的存储过程
- Mysql中的事务是什么如何使用
- MySql的事务使用与示例详解
- C#分布式事务的超时处理实例分析
- C#中的事务用法实例分析