java代码读取excel文件,同时兼容2003和2007
2016-07-26 15:06
671 查看
后台服务器需要一个读取excel文件的工具,查了些资料,很多不能同时兼容2003和2007,整理了一下,加了一个判断,现在能同时兼容2003和2007,并且可以选择从表格的第几行开始读取。主要用到了apache的jar包,用到的包有(jar包官网下载地址:http://poi.apache.org/download.html):
dom4j-1.6.1.jar
xmlbeans-2.3.0.jar
poi-3.8-beta3-20110606.jar
poi-ooxml-3.8-beta3-20110606.jar
poi-examples-3.8-beta3-20110606.jar
poi-excelant-3.8-beta3-20110606.jar
poi-ooxml-schemas-3.8-beta3-20110606.jar
poi-scratchpad-3.8-beta3-20110606.jar
下面上代码:
package com.iris.readexcel;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.text.DecimalFormat;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFDataFormat;
import org.apache.poi.hssf.usermodel.HSSFDateUtil;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
/**
* 读取 excel表格,兼容2003和2007
* @author ChuanJing
*/
public class ReadExcel {
/** 总行数 */
private int totalRows = 0;
/** 总列数 */
private int totalCells = 0;
/** 错误信息 */
private String errorInfo;
/** 构造方法 */
public ReadExcel() {}
/**
* 得到总行数
*/
public int getTotalRows() {
return totalRows;
}
/**
* 得到总列数
*/
public int getTotalCells() {
return totalCells;
}
/**
* 得到错误信息
*/
public String getErrorInfo() {
return errorInfo;
}
/**
*
* 验证excel文件
*
* @param:filePath 文件完整路径
* @return 返回 true 表示文件没有问题
*/
public boolean validateExcel(String filePath) {
/** 检查文件名是否为空或者是否是Excel格式的文件 */
if (filePath == null || !(isExcel2003(filePath) || isExcel2007(filePath))) {
errorInfo = "文件不是excel格式";
return false;
}
/** 检查文件是否存在 */
File file = new File(filePath);
if (file == null || !file.exists()) {
errorInfo = "文件不存在";
return false;
}
return true;
}
/**
* 根据文件名读取excel文件
*
* @param filePath 文件完整路径
* @param ignoreRows 读取数据忽略的行数,比喻第一行不需要读入,忽略的行数为1
* @return:List 最后读取的结果,数据结构:List<List<String>>
*/
public List<List<String>> read(String filePath, int ignoreRows) {
List<List<String>> dataLst = new ArrayList<List<String>>();
InputStream is = null;
try {
/** 验证文件是否合法 */
if (!validateExcel(filePath)) {
System.out.println(errorInfo);
return null;
}
/** 判断文件的类型,是2003还是2007 */
boolean isExcel2003 = true;
if (isExcel2007(filePath)) {
isExcel2003 = false;
}
/** 调用本类提供的根据流读取的方法 */
File file = new File(filePath);
is = new FileInputStream(file);
dataLst = read(is, isExcel2003, ignoreRows);
is.close();
} catch (Exception ex) {
ex.printStackTrace();
} finally {
if (is != null) {
try {
is.close();
} catch (IOException e) {
is = null;
e.printStackTrace();
}
}
}
/** 返回最后读取的结果 */
return dataLst;
}
/**
* 根据流读取Excel文件
*
* @param inputStream
* @param isExcel2003 是否是2003的表格(xls格式)
* @param ignoreRows 读取数据忽略的行数,比喻第一行不需要读入,忽略的行数为1
* @return:List
*/
public List<List<String>> read(InputStream inputStream, boolean isExcel2003, int ignoreRows) {
List<List<String>> dataLst = null;
try {
/** 根据版本选择创建Workbook的方式 */
Workbook wb = null;
if (isExcel2003) {
wb = new HSSFWorkbook(inputStream);
} else {
wb = new XSSFWorkbook(inputStream);
}
dataLst = read(wb, ignoreRows);
} catch (IOException e) {
e.printStackTrace();
}
return dataLst;
}
/**
* 读取数据
*
* @param Workbook
* @param ignoreRows 读取数据忽略的行数,比喻第一行不需要读入,忽略的行数为1
* @reture:List<List<String>>
*/
private List<List<String>> read(Workbook wb, int ignoreRows) {
List<List<String>> dataLst = new ArrayList<List<String>>();
/** 得到第一个shell */
Sheet sheet = wb.getSheetAt(0);
/** 得到Excel的行数 */
this.totalRows = sheet.getPhysicalNumberOfRows();
/** 得到Excel的列数,不从表格的第一行得到列数,从忽略之后的,要读取的第一行 获取列数*/
if (this.totalRows >= 1 && sheet.getRow(ignoreRows) != null) {
this.totalCells = sheet.getRow(ignoreRows).getPhysicalNumberOfCells();
}
/** 循环Excel的行,不从表格的第一行循环,去掉忽略的行数*/
for (int r = ignoreRows; r < this.totalRows; r++) {
Row row = sheet.getRow(r);
if (row == null) {
continue;
}
List<String> rowLst = new ArrayList<String>();
/** 循环Excel的列 */
for (int c = 0; c <= this.getTotalCells(); c++) {
Cell cell = row.getCell(c);
String cellValue = "";
if (null != cell) {
// 以下是判断数据的类型
switch (cell.getCellType()) {
case HSSFCell.CELL_TYPE_NUMERIC: // 数字
// 如果数字是日期类型,就转换成日期
if (HSSFDateUtil.isCellDateFormatted(cell)) {// 处理日期格式、时间格式
SimpleDateFormat sdf = null;
if (cell.getCellStyle().getDataFormat() == HSSFDataFormat.getBuiltinFormat("h:mm")) {
sdf = new SimpleDateFormat("HH:mm");
} else {// 日期
sdf = new SimpleDateFormat("yyyy年MM月dd日");
}
Date date = cell.getDateCellValue();
cellValue = sdf.format(date);
} else if (cell.getCellStyle().getDataFormat() == 31) {
// 处理自定义日期格式:yyyy年m月d日(通过判断单元格的格式id解决,id的值是31)
SimpleDateFormat sdf = new SimpleDateFormat("yyyy年MM月dd日");
double value = cell.getNumericCellValue();
Date date = org.apache.poi.ss.usermodel.DateUtil.getJavaDate(value);
cellValue = sdf.format(date);
} else {
double value = cell.getNumericCellValue();
CellStyle style = cell.getCellStyle();
DecimalFormat format = new DecimalFormat();
String temp = style.getDataFormatString();
// 单元格设置成常规
if (temp.equals("General")) {
format.applyPattern("#");
}
cellValue = format.format(value);
}
break;
case HSSFCell.CELL_TYPE_STRING: // 字符串
cellValue = cell.getStringCellValue();
break;
case HSSFCell.CELL_TYPE_BOOLEAN: // Boolean
cellValue = cell.getBooleanCellValue() + "";
break;
case HSSFCell.CELL
4000
_TYPE_FORMULA: // 公式
cellValue = cell.getCellFormula() + "";
break;
case HSSFCell.CELL_TYPE_BLANK: // 空值
cellValue = "";
break;
case HSSFCell.CELL_TYPE_ERROR: // 故障
cellValue = "非法字符";
break;
default:
cellValue = "未知类型";
break;
}
}
rowLst.add(cellValue);
}
/** 保存第r行的第c列 */
dataLst.add(rowLst);
}
return dataLst;
}
/**
* 是否是2003的excel,返回true是2003
*
* @param filePath 文件完整路径
* @return boolean true代表是2003
*/
public static boolean isExcel2003(String filePath) {
return filePath.matches("^.+\\.(?i)(xls)$");
}
/**
* 是否是2007的excel,返回true是2007
*
* @param filePath 文件完整路径
* @return boolean true代表是2007
*/
public static boolean isExcel2007(String filePath) {
return filePath.matches("^.+\\.(?i)(xlsx)$");
}
}
下面是测试类:
package com.iris.readexcel;
import java.util.List;
/**
* 测试类
*
* @author ChuanJing
*
*/
public class TestReadExcel {
public static void main(String[] args) throws Exception {
ReadExcel re = new ReadExcel();
List<List<String>> list = re.read("C:\\Users\\Iris004\\Desktop\\测试表格.xls", 5);//忽略前5行
// 遍历读取结果
if (list != null) {
for (int i = 0; i < list.size(); i++) {
System.out.print("第" + (6+i) + "行");
List<String> cellList = list.get(i);
for (int j = 0; j < cellList.size(); j++) {
System.out.print(" 第" + (j + 1) + "列值:");
System.out.print(" " + cellList.get(j));
}
System.out.println();
}
}
}
}
上面是简单的测试一下,就不上图了。
最后我开了4条线程,每条线程单独读取一个表格,然后把读取的数据写入到单独的一个txt文本中,每个表格都是13000多行,在我机器上大概15秒跑完(CPU:Intel Pentium G645 2.9G,内存:4G)。下面是第4条线程读取表格写入文件的部分截图 和 表格截图:
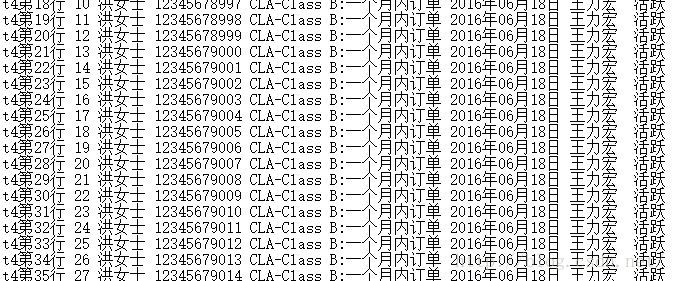
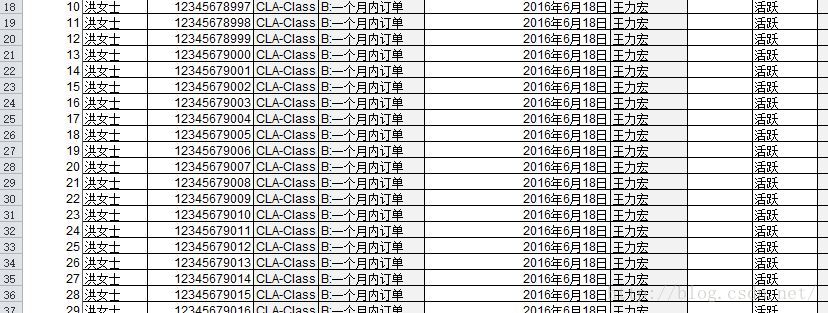
最后附上源码下载地址:http://download.csdn.net/detail/chuan_jing/9586676
dom4j-1.6.1.jar
xmlbeans-2.3.0.jar
poi-3.8-beta3-20110606.jar
poi-ooxml-3.8-beta3-20110606.jar
poi-examples-3.8-beta3-20110606.jar
poi-excelant-3.8-beta3-20110606.jar
poi-ooxml-schemas-3.8-beta3-20110606.jar
poi-scratchpad-3.8-beta3-20110606.jar
下面上代码:
package com.iris.readexcel;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.text.DecimalFormat;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFDataFormat;
import org.apache.poi.hssf.usermodel.HSSFDateUtil;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
/**
* 读取 excel表格,兼容2003和2007
* @author ChuanJing
*/
public class ReadExcel {
/** 总行数 */
private int totalRows = 0;
/** 总列数 */
private int totalCells = 0;
/** 错误信息 */
private String errorInfo;
/** 构造方法 */
public ReadExcel() {}
/**
* 得到总行数
*/
public int getTotalRows() {
return totalRows;
}
/**
* 得到总列数
*/
public int getTotalCells() {
return totalCells;
}
/**
* 得到错误信息
*/
public String getErrorInfo() {
return errorInfo;
}
/**
*
* 验证excel文件
*
* @param:filePath 文件完整路径
* @return 返回 true 表示文件没有问题
*/
public boolean validateExcel(String filePath) {
/** 检查文件名是否为空或者是否是Excel格式的文件 */
if (filePath == null || !(isExcel2003(filePath) || isExcel2007(filePath))) {
errorInfo = "文件不是excel格式";
return false;
}
/** 检查文件是否存在 */
File file = new File(filePath);
if (file == null || !file.exists()) {
errorInfo = "文件不存在";
return false;
}
return true;
}
/**
* 根据文件名读取excel文件
*
* @param filePath 文件完整路径
* @param ignoreRows 读取数据忽略的行数,比喻第一行不需要读入,忽略的行数为1
* @return:List 最后读取的结果,数据结构:List<List<String>>
*/
public List<List<String>> read(String filePath, int ignoreRows) {
List<List<String>> dataLst = new ArrayList<List<String>>();
InputStream is = null;
try {
/** 验证文件是否合法 */
if (!validateExcel(filePath)) {
System.out.println(errorInfo);
return null;
}
/** 判断文件的类型,是2003还是2007 */
boolean isExcel2003 = true;
if (isExcel2007(filePath)) {
isExcel2003 = false;
}
/** 调用本类提供的根据流读取的方法 */
File file = new File(filePath);
is = new FileInputStream(file);
dataLst = read(is, isExcel2003, ignoreRows);
is.close();
} catch (Exception ex) {
ex.printStackTrace();
} finally {
if (is != null) {
try {
is.close();
} catch (IOException e) {
is = null;
e.printStackTrace();
}
}
}
/** 返回最后读取的结果 */
return dataLst;
}
/**
* 根据流读取Excel文件
*
* @param inputStream
* @param isExcel2003 是否是2003的表格(xls格式)
* @param ignoreRows 读取数据忽略的行数,比喻第一行不需要读入,忽略的行数为1
* @return:List
*/
public List<List<String>> read(InputStream inputStream, boolean isExcel2003, int ignoreRows) {
List<List<String>> dataLst = null;
try {
/** 根据版本选择创建Workbook的方式 */
Workbook wb = null;
if (isExcel2003) {
wb = new HSSFWorkbook(inputStream);
} else {
wb = new XSSFWorkbook(inputStream);
}
dataLst = read(wb, ignoreRows);
} catch (IOException e) {
e.printStackTrace();
}
return dataLst;
}
/**
* 读取数据
*
* @param Workbook
* @param ignoreRows 读取数据忽略的行数,比喻第一行不需要读入,忽略的行数为1
* @reture:List<List<String>>
*/
private List<List<String>> read(Workbook wb, int ignoreRows) {
List<List<String>> dataLst = new ArrayList<List<String>>();
/** 得到第一个shell */
Sheet sheet = wb.getSheetAt(0);
/** 得到Excel的行数 */
this.totalRows = sheet.getPhysicalNumberOfRows();
/** 得到Excel的列数,不从表格的第一行得到列数,从忽略之后的,要读取的第一行 获取列数*/
if (this.totalRows >= 1 && sheet.getRow(ignoreRows) != null) {
this.totalCells = sheet.getRow(ignoreRows).getPhysicalNumberOfCells();
}
/** 循环Excel的行,不从表格的第一行循环,去掉忽略的行数*/
for (int r = ignoreRows; r < this.totalRows; r++) {
Row row = sheet.getRow(r);
if (row == null) {
continue;
}
List<String> rowLst = new ArrayList<String>();
/** 循环Excel的列 */
for (int c = 0; c <= this.getTotalCells(); c++) {
Cell cell = row.getCell(c);
String cellValue = "";
if (null != cell) {
// 以下是判断数据的类型
switch (cell.getCellType()) {
case HSSFCell.CELL_TYPE_NUMERIC: // 数字
// 如果数字是日期类型,就转换成日期
if (HSSFDateUtil.isCellDateFormatted(cell)) {// 处理日期格式、时间格式
SimpleDateFormat sdf = null;
if (cell.getCellStyle().getDataFormat() == HSSFDataFormat.getBuiltinFormat("h:mm")) {
sdf = new SimpleDateFormat("HH:mm");
} else {// 日期
sdf = new SimpleDateFormat("yyyy年MM月dd日");
}
Date date = cell.getDateCellValue();
cellValue = sdf.format(date);
} else if (cell.getCellStyle().getDataFormat() == 31) {
// 处理自定义日期格式:yyyy年m月d日(通过判断单元格的格式id解决,id的值是31)
SimpleDateFormat sdf = new SimpleDateFormat("yyyy年MM月dd日");
double value = cell.getNumericCellValue();
Date date = org.apache.poi.ss.usermodel.DateUtil.getJavaDate(value);
cellValue = sdf.format(date);
} else {
double value = cell.getNumericCellValue();
CellStyle style = cell.getCellStyle();
DecimalFormat format = new DecimalFormat();
String temp = style.getDataFormatString();
// 单元格设置成常规
if (temp.equals("General")) {
format.applyPattern("#");
}
cellValue = format.format(value);
}
break;
case HSSFCell.CELL_TYPE_STRING: // 字符串
cellValue = cell.getStringCellValue();
break;
case HSSFCell.CELL_TYPE_BOOLEAN: // Boolean
cellValue = cell.getBooleanCellValue() + "";
break;
case HSSFCell.CELL
4000
_TYPE_FORMULA: // 公式
cellValue = cell.getCellFormula() + "";
break;
case HSSFCell.CELL_TYPE_BLANK: // 空值
cellValue = "";
break;
case HSSFCell.CELL_TYPE_ERROR: // 故障
cellValue = "非法字符";
break;
default:
cellValue = "未知类型";
break;
}
}
rowLst.add(cellValue);
}
/** 保存第r行的第c列 */
dataLst.add(rowLst);
}
return dataLst;
}
/**
* 是否是2003的excel,返回true是2003
*
* @param filePath 文件完整路径
* @return boolean true代表是2003
*/
public static boolean isExcel2003(String filePath) {
return filePath.matches("^.+\\.(?i)(xls)$");
}
/**
* 是否是2007的excel,返回true是2007
*
* @param filePath 文件完整路径
* @return boolean true代表是2007
*/
public static boolean isExcel2007(String filePath) {
return filePath.matches("^.+\\.(?i)(xlsx)$");
}
}
下面是测试类:
package com.iris.readexcel;
import java.util.List;
/**
* 测试类
*
* @author ChuanJing
*
*/
public class TestReadExcel {
public static void main(String[] args) throws Exception {
ReadExcel re = new ReadExcel();
List<List<String>> list = re.read("C:\\Users\\Iris004\\Desktop\\测试表格.xls", 5);//忽略前5行
// 遍历读取结果
if (list != null) {
for (int i = 0; i < list.size(); i++) {
System.out.print("第" + (6+i) + "行");
List<String> cellList = list.get(i);
for (int j = 0; j < cellList.size(); j++) {
System.out.print(" 第" + (j + 1) + "列值:");
System.out.print(" " + cellList.get(j));
}
System.out.println();
}
}
}
}
上面是简单的测试一下,就不上图了。
最后我开了4条线程,每条线程单独读取一个表格,然后把读取的数据写入到单独的一个txt文本中,每个表格都是13000多行,在我机器上大概15秒跑完(CPU:Intel Pentium G645 2.9G,内存:4G)。下面是第4条线程读取表格写入文件的部分截图 和 表格截图:
最后附上源码下载地址:http://download.csdn.net/detail/chuan_jing/9586676
相关文章推荐
- php读取EXCEL文件 php excelreader读取excel文件
- Android读取Excel文件
- Android读取excel文件内容
- 使用poi导入Excel表格中的数据,表格的版本必须是2007以上
- Python读取Excel文件
- javaweb学习总结(五)——Servlet开发(一)
- Java自动内存管理机制(四) 垃圾收集算法
- Java工程师成神之路~
- java--时间字符串类型转换为可存入数据库时间类型的方法
- 自己手动写个线程池
- java 多线程 操作 CountDownLatch、Semaphore和CyclicBarrier
- 访问对象属性的点表示法和方括号表示法的区别
- Java设计模式—— 职责链模式
- Java imageIO处理图像
- 对Java中File I/O的理解
- Spring和resteasy集成三种方式
- 如何改变Myeclipse编辑区背景色
- Java项目JUnit简单使用
- Myeclipse快捷键记录
- (10)Spring和Hibernate整合过程中遇到的问题