c++操作Office之Word
2016-07-25 14:57
375 查看
目的:通过c++访问Office,操作Word;
环境:win7 64位、vs2008、office2013、
步骤:1、首先打开vs2008,创建一个基于对话框的应用程序;
2、右键创建的项目,添加CLASS,选择MFC Class From TypeLib,
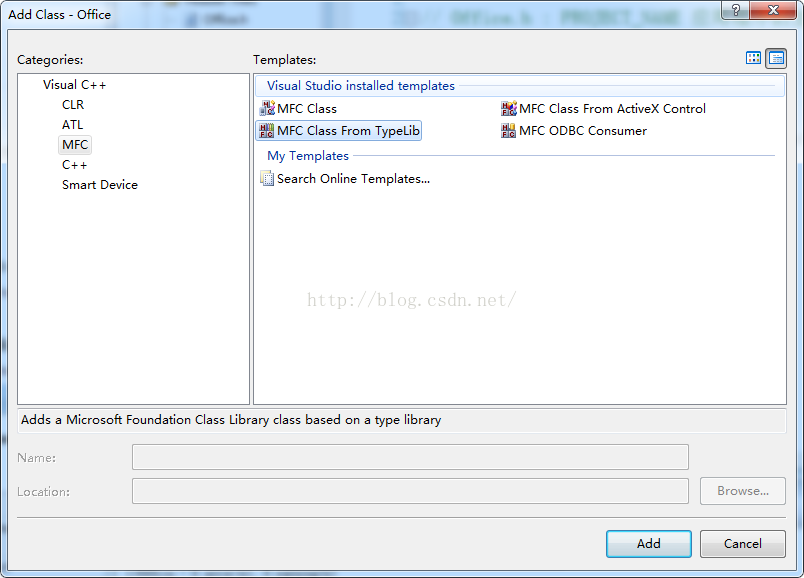
3、在接下来的对话框中选择File,然后从office应用程序选择需要的Office类型库,我的路径为:C:\Program Files\Microsoft Office\Office15\MSWORD.OLB;
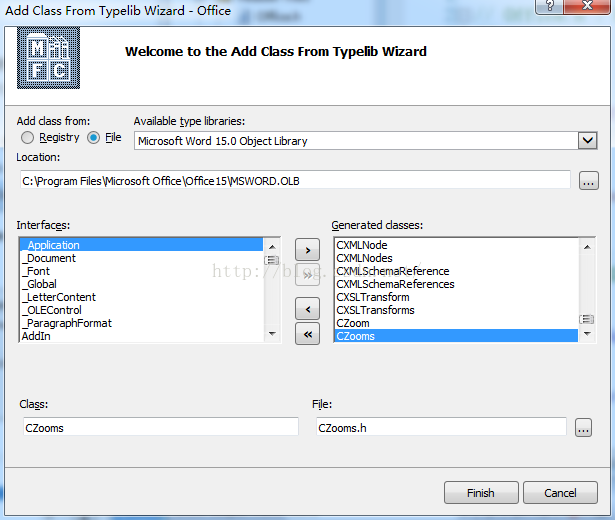
4、点击完成并可生成需要的文件类;然后初始化COM,找到APP的InitInstance()函数,在其中添加:
if (!AfxOleInit())
{
AfxMessageBox("Initialize COM context failed!");
return FALSE;
} 5、下面就是代码的实现,转自:http://blog.csdn.net/xxxxxx91116/article/details/8543473
WordOperate.h:
#include "msword.h"
#define wdCharacter 1
#define wdLine 5
#define wdCell 12
#define wdExtend 1
#define wdMove 0
using namespace myword;
#include "atlbase.h"
class CWordOperate
{
public:
CWordOperate();
virtual ~CWordOperate();
private:
_Application m_wdApp;
Documents m_wdDocs;
_Document m_wdDoc;
Selection m_wdSel;
Range m_wdRange;
public:
//操作
//**********************创建新文档*******************************************
BOOL CreateApp(); //创建一个新的WORD应用程序
BOOL CreateDocuments(); //创建一个新的Word文档集合
BOOL CreateDocument(); //创建一个新的Word文档
BOOL Create(); //创建新的WORD应用程序并创建一个新的文档
void ShowApp(); //显示WORD文档
void HideApp(); //隐藏word文档
//**********************打开文档*********************************************
BOOL OpenDocument(CString fileName);//打开已经存在的文档。
BOOL Open(CString fileName); //创建新的WORD应用程序并打开一个已经存在的文档。
BOOL SetActiveDocument(short i); //设置当前激活的文档。
//**********************保存文档*********************************************
BOOL SaveDocument(); //文档是以打开形式,保存。
BOOL SaveDocumentAs(CString fileName);//文档以创建形式,保存。
BOOL CloseDocument();
void CloseApp();
//**********************文本书写操作*****************************************
void WriteText(CString szText); //当前光标处写文本
void WriteNewLineText(CString szText, int nLineCount = 1); //换N行写字
void WriteEndLine(CString szText); //文档结尾处写文本
void WholeStory(); //全选文档内容
void Copy(); //复制文本内容到剪贴板
void InsertFile(CString fileName); //将本地的文件全部内容写入到当前文档的光标处。
//----------------------add by zxx--------------------------------------
//***********************光标操作********************************************
//上下按行选择
void SelectMoveDown(short lineCount, short unit);//有选择操作的移动
void NoneSelectMoveDown(short lineCount, short unit);//仅仅移动光标,不选中
void SelectMoveUp(short lineCount, short unit);//有选择操作的移动
void NoneSelectMoveUp(short lineCount, short unit);//仅仅移动光标,不选中
//左右按列选择
void SelectMoveLeft(short charCount, short unit);//有选择操作的移动
void NoneSelectMoveLeft(short charCount, short unit);//
void SelectMoveRight(short charCount, short unit);//有选择操作的移动
void NoneSelectMoveRight(short charCount, short unit);//
void MoveToFirst();
void MoveToNextPage();
void TypeParagraph();
void PasteAndFormat();
void Paste();
void TypeBackspace(int count);
};WordOperate.cpp
CWordOperate::CWordOperate()
{
}
CWordOperate::~CWordOperate()
{
}
//操作
BOOL CWordOperate::CreateApp()
{
COleException pe;
if (!m_wdApp.CreateDispatch(_T("Word.Application"), &pe))
{
AfxMessageBox("Application创建失败,请确保安装了word 2000或以上版本!", MB_OK|MB_ICONWARNING);
pe.ReportError();
throw &pe;
return FALSE;
}
return TRUE;
}
BOOL CWordOperate::CreateDocuments()
{
if (FALSE == CreateApp())
{
return FALSE;
}
m_wdDocs.AttachDispatch(m_wdApp.GetDocuments());
if (!m_wdDocs.m_lpDispatch)
{
AfxMessageBox("Documents创建失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
return TRUE;
}
BOOL CWordOperate::CreateDocument()
{
if (!m_wdDocs.m_lpDispatch)
{
AfxMessageBox("Documents为空!", MB_OK|MB_ICONWARNING);
return FALSE;
}
COleVariant varTrue(short(1),VT_BOOL),vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR);
CComVariant Template(_T("")); //没有使用WORD的文档模板
CComVariant NewTemplate(false),DocumentType(0),Visible;
m_wdDocs.Add(&Template,&NewTemplate,&DocumentType,&Visible);
//得到document变量
m_wdDoc = m_wdApp.GetActiveDocument();
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到selection变量
m_wdSel = m_wdApp.GetSelection();
if (!m_wdSel.m_lpDispatch)
{
AfxMessageBox("Select获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到Range变量
m_wdRange = m_wdDoc.Range(vOptional,vOptional);
if(!m_wdRange.m_lpDispatch)
{
AfxMessageBox("Range获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
return TRUE;
}
BOOL CWordOperate::Create()
{
if (FALSE == CreateDocuments())
{
return FALSE;
}
return CreateDocument();
}
void CWordOperate::ShowApp()
{
m_wdApp.SetVisible(TRUE);
}
void CWordOperate::HideApp()
{
m_wdApp.SetVisible(FALSE);
}
BOOL CWordOperate::OpenDocument(CString fileName)
{
if (!m_wdDocs.m_lpDispatch)
{
AfxMessageBox("Documents为空!", MB_OK|MB_ICONWARNING);
return FALSE;
}
COleVariant vTrue((short)TRUE),
vFalse((short)FALSE),
vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR),
vZ((short)0);
COleVariant vFileName(_T(fileName));
//得到document变量
m_wdDoc.AttachDispatch(m_wdDocs.Open(
vFileName, // FileName
vTrue, // Confirm Conversion.
vFalse, // ReadOnly.
vFalse, // AddToRecentFiles.
vOptional, // PasswordDocument.
vOptional, // PasswordTemplate.
vOptional, // Revert.
vOptional
b46d
, // WritePasswordDocument.
vOptional, // WritePasswordTemplate.
vOptional, // Format. // Last argument for Word 97
vOptional, // Encoding // New for Word 2000/2002
vOptional, // Visible
//如下4个是word2003需要的参数。本版本是word2000。
vOptional, // OpenAndRepair
vZ, // DocumentDirection wdDocumentDirection LeftToRight
vOptional, // NoEncodingDialog
vOptional
) // Close Open parameters
); // Close AttachDispatch
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到selection变量
m_wdSel = m_wdApp.GetSelection();
if (!m_wdSel.m_lpDispatch)
{
AfxMessageBox("Select获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到全部DOC的Range变量
m_wdRange = m_wdDoc.Range(vOptional,vOptional);
if(!m_wdRange.m_lpDispatch)
{
AfxMessageBox("Range获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
return TRUE;
}
BOOL CWordOperate::Open(CString fileName)
{
if (FALSE == CreateDocuments())
{
return FALSE;
}
//HideApp();
return OpenDocument(fileName);
}
BOOL CWordOperate::SetActiveDocument(short i)
{
COleVariant vIndex(_T(i)),vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR);
m_wdDoc.AttachDispatch(m_wdDocs.Item(vIndex));
m_wdDoc.Activate();
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到selection变量
m_wdSel = m_wdApp.GetSelection();
if (!m_wdSel.m_lpDispatch)
{
AfxMessageBox("Select获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到全部DOC的Range变量
m_wdRange = m_wdDoc.Range(vOptional,vOptional);
if(!m_wdRange.m_lpDispatch)
{
AfxMessageBox("Range获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
HideApp();
return TRUE;
}
BOOL CWordOperate::SaveDocument()
{
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
m_wdDoc.Save();
return TRUE;
}
BOOL CWordOperate::SaveDocumentAs(CString fileName)
{
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
COleVariant vTrue((short)TRUE),
vFalse((short)FALSE),
vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR);
COleVariant vFileName(_T(fileName));
m_wdDoc.SaveAs(
vFileName, //VARIANT* FileName
vOptional, //VARIANT* FileFormat
vOptional, //VARIANT* LockComments
vOptional, //VARIANT* Password
vOptional, //VARIANT* AddToRecentFiles
vOptional, //VARIANT* WritePassword
vOptional, //VARIANT* ReadOnlyRecommended
vOptional, //VARIANT* EmbedTrueTypeFonts
vOptional, //VARIANT* SaveNativePictureFormat
vOptional, //VARIANT* SaveFormsData
vOptional, //VARIANT* SaveAsAOCELetter
vOptional, //VARIANT* ReadOnlyRecommended
vOptional, //VARIANT* EmbedTrueTypeFonts
vOptional, //VARIANT* SaveNativePictureFormat
vOptional, //VARIANT* SaveFormsData
vOptional //VARIANT* SaveAsAOCELetter
);
return TRUE;
}
BOOL CWordOperate::CloseDocument()
{
COleVariant vTrue((short)TRUE),
vFalse((short)FALSE),
vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR);
m_wdDoc.Close(vFalse, // SaveChanges.
vTrue, // OriginalFormat.
vFalse // RouteDocument.
);
//AfxMessageBox("c1");
m_wdDoc.AttachDispatch(m_wdApp.GetActiveDocument());
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
// AfxMessageBox("c2");
//得到selection变量
m_wdSel = m_wdApp.GetSelection();
if (!m_wdSel.m_lpDispatch)
{
AfxMessageBox("Select获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
// AfxMessageBox("c3");
//得到全部DOC的Range变量
m_wdRange = m_wdDoc.Range(vOptional,vOptional);
if(!m_wdRange.m_lpDispatch)
{
AfxMessageBox("Range获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
// AfxMessageBox("c4");
return TRUE;
}
void CWordOperate::CloseApp()
{
COleVariant vTrue((short)TRUE),
vFalse((short)FALSE),
vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR);
m_wdDoc.Save();
m_wdApp.Quit(vFalse, // SaveChanges.
vTrue, // OriginalFormat.
vFalse // RouteDocument.
);
//释放内存申请资源
m_wdRange.ReleaseDispatch();
m_wdSel.ReleaseDispatch();
m_wdDoc.ReleaseDispatch();
m_wdDocs.ReleaseDispatch();
m_wdApp.ReleaseDispatch();
}
void CWordOperate::WriteText(CString szText)
{
m_wdSel.TypeText(szText);
}
void CWordOperate::WriteNewLineText(CString szText, int nLineCount /**//* = 1 */)
{
int i;
if (nLineCount <= 0)
{
nLineCount = 0;
}
for (i = 0; i < nLineCount; i++)
{
m_wdSel.TypeParagraph();
}
WriteText(szText);
}
void CWordOperate::WriteEndLine(CString szText)
{
m_wdRange.InsertAfter(szText);
}
void CWordOperate::WholeStory()
{
m_wdRange.WholeStory();
}
void CWordOperate::Copy()
{
m_wdSel.Copy();
//m_wdSel.CopyFormat();
}
void CWordOperate::TypeParagraph()
{
m_wdSel.TypeParagraph();
}
void CWordOperate::PasteAndFormat()
{
m_wdSel.PasteAndFormat(0);
}
void CWordOperate::Paste()
{
m_wdSel.Paste();
//m_wdSel.PasteFormat();
}
void CWordOperate::TypeBackspace(int count)
{
for(int i = 0; i < count; i++)
m_wdSel.TypeBackspace();
}
环境:win7 64位、vs2008、office2013、
步骤:1、首先打开vs2008,创建一个基于对话框的应用程序;
2、右键创建的项目,添加CLASS,选择MFC Class From TypeLib,
3、在接下来的对话框中选择File,然后从office应用程序选择需要的Office类型库,我的路径为:C:\Program Files\Microsoft Office\Office15\MSWORD.OLB;
4、点击完成并可生成需要的文件类;然后初始化COM,找到APP的InitInstance()函数,在其中添加:
if (!AfxOleInit())
{
AfxMessageBox("Initialize COM context failed!");
return FALSE;
} 5、下面就是代码的实现,转自:http://blog.csdn.net/xxxxxx91116/article/details/8543473
WordOperate.h:
#include "msword.h"
#define wdCharacter 1
#define wdLine 5
#define wdCell 12
#define wdExtend 1
#define wdMove 0
using namespace myword;
#include "atlbase.h"
class CWordOperate
{
public:
CWordOperate();
virtual ~CWordOperate();
private:
_Application m_wdApp;
Documents m_wdDocs;
_Document m_wdDoc;
Selection m_wdSel;
Range m_wdRange;
public:
//操作
//**********************创建新文档*******************************************
BOOL CreateApp(); //创建一个新的WORD应用程序
BOOL CreateDocuments(); //创建一个新的Word文档集合
BOOL CreateDocument(); //创建一个新的Word文档
BOOL Create(); //创建新的WORD应用程序并创建一个新的文档
void ShowApp(); //显示WORD文档
void HideApp(); //隐藏word文档
//**********************打开文档*********************************************
BOOL OpenDocument(CString fileName);//打开已经存在的文档。
BOOL Open(CString fileName); //创建新的WORD应用程序并打开一个已经存在的文档。
BOOL SetActiveDocument(short i); //设置当前激活的文档。
//**********************保存文档*********************************************
BOOL SaveDocument(); //文档是以打开形式,保存。
BOOL SaveDocumentAs(CString fileName);//文档以创建形式,保存。
BOOL CloseDocument();
void CloseApp();
//**********************文本书写操作*****************************************
void WriteText(CString szText); //当前光标处写文本
void WriteNewLineText(CString szText, int nLineCount = 1); //换N行写字
void WriteEndLine(CString szText); //文档结尾处写文本
void WholeStory(); //全选文档内容
void Copy(); //复制文本内容到剪贴板
void InsertFile(CString fileName); //将本地的文件全部内容写入到当前文档的光标处。
//----------------------add by zxx--------------------------------------
//***********************光标操作********************************************
//上下按行选择
void SelectMoveDown(short lineCount, short unit);//有选择操作的移动
void NoneSelectMoveDown(short lineCount, short unit);//仅仅移动光标,不选中
void SelectMoveUp(short lineCount, short unit);//有选择操作的移动
void NoneSelectMoveUp(short lineCount, short unit);//仅仅移动光标,不选中
//左右按列选择
void SelectMoveLeft(short charCount, short unit);//有选择操作的移动
void NoneSelectMoveLeft(short charCount, short unit);//
void SelectMoveRight(short charCount, short unit);//有选择操作的移动
void NoneSelectMoveRight(short charCount, short unit);//
void MoveToFirst();
void MoveToNextPage();
void TypeParagraph();
void PasteAndFormat();
void Paste();
void TypeBackspace(int count);
};WordOperate.cpp
CWordOperate::CWordOperate()
{
}
CWordOperate::~CWordOperate()
{
}
//操作
BOOL CWordOperate::CreateApp()
{
COleException pe;
if (!m_wdApp.CreateDispatch(_T("Word.Application"), &pe))
{
AfxMessageBox("Application创建失败,请确保安装了word 2000或以上版本!", MB_OK|MB_ICONWARNING);
pe.ReportError();
throw &pe;
return FALSE;
}
return TRUE;
}
BOOL CWordOperate::CreateDocuments()
{
if (FALSE == CreateApp())
{
return FALSE;
}
m_wdDocs.AttachDispatch(m_wdApp.GetDocuments());
if (!m_wdDocs.m_lpDispatch)
{
AfxMessageBox("Documents创建失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
return TRUE;
}
BOOL CWordOperate::CreateDocument()
{
if (!m_wdDocs.m_lpDispatch)
{
AfxMessageBox("Documents为空!", MB_OK|MB_ICONWARNING);
return FALSE;
}
COleVariant varTrue(short(1),VT_BOOL),vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR);
CComVariant Template(_T("")); //没有使用WORD的文档模板
CComVariant NewTemplate(false),DocumentType(0),Visible;
m_wdDocs.Add(&Template,&NewTemplate,&DocumentType,&Visible);
//得到document变量
m_wdDoc = m_wdApp.GetActiveDocument();
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到selection变量
m_wdSel = m_wdApp.GetSelection();
if (!m_wdSel.m_lpDispatch)
{
AfxMessageBox("Select获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到Range变量
m_wdRange = m_wdDoc.Range(vOptional,vOptional);
if(!m_wdRange.m_lpDispatch)
{
AfxMessageBox("Range获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
return TRUE;
}
BOOL CWordOperate::Create()
{
if (FALSE == CreateDocuments())
{
return FALSE;
}
return CreateDocument();
}
void CWordOperate::ShowApp()
{
m_wdApp.SetVisible(TRUE);
}
void CWordOperate::HideApp()
{
m_wdApp.SetVisible(FALSE);
}
BOOL CWordOperate::OpenDocument(CString fileName)
{
if (!m_wdDocs.m_lpDispatch)
{
AfxMessageBox("Documents为空!", MB_OK|MB_ICONWARNING);
return FALSE;
}
COleVariant vTrue((short)TRUE),
vFalse((short)FALSE),
vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR),
vZ((short)0);
COleVariant vFileName(_T(fileName));
//得到document变量
m_wdDoc.AttachDispatch(m_wdDocs.Open(
vFileName, // FileName
vTrue, // Confirm Conversion.
vFalse, // ReadOnly.
vFalse, // AddToRecentFiles.
vOptional, // PasswordDocument.
vOptional, // PasswordTemplate.
vOptional, // Revert.
vOptional
b46d
, // WritePasswordDocument.
vOptional, // WritePasswordTemplate.
vOptional, // Format. // Last argument for Word 97
vOptional, // Encoding // New for Word 2000/2002
vOptional, // Visible
//如下4个是word2003需要的参数。本版本是word2000。
vOptional, // OpenAndRepair
vZ, // DocumentDirection wdDocumentDirection LeftToRight
vOptional, // NoEncodingDialog
vOptional
) // Close Open parameters
); // Close AttachDispatch
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到selection变量
m_wdSel = m_wdApp.GetSelection();
if (!m_wdSel.m_lpDispatch)
{
AfxMessageBox("Select获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到全部DOC的Range变量
m_wdRange = m_wdDoc.Range(vOptional,vOptional);
if(!m_wdRange.m_lpDispatch)
{
AfxMessageBox("Range获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
return TRUE;
}
BOOL CWordOperate::Open(CString fileName)
{
if (FALSE == CreateDocuments())
{
return FALSE;
}
//HideApp();
return OpenDocument(fileName);
}
BOOL CWordOperate::SetActiveDocument(short i)
{
COleVariant vIndex(_T(i)),vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR);
m_wdDoc.AttachDispatch(m_wdDocs.Item(vIndex));
m_wdDoc.Activate();
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到selection变量
m_wdSel = m_wdApp.GetSelection();
if (!m_wdSel.m_lpDispatch)
{
AfxMessageBox("Select获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
//得到全部DOC的Range变量
m_wdRange = m_wdDoc.Range(vOptional,vOptional);
if(!m_wdRange.m_lpDispatch)
{
AfxMessageBox("Range获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
HideApp();
return TRUE;
}
BOOL CWordOperate::SaveDocument()
{
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
m_wdDoc.Save();
return TRUE;
}
BOOL CWordOperate::SaveDocumentAs(CString fileName)
{
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
COleVariant vTrue((short)TRUE),
vFalse((short)FALSE),
vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR);
COleVariant vFileName(_T(fileName));
m_wdDoc.SaveAs(
vFileName, //VARIANT* FileName
vOptional, //VARIANT* FileFormat
vOptional, //VARIANT* LockComments
vOptional, //VARIANT* Password
vOptional, //VARIANT* AddToRecentFiles
vOptional, //VARIANT* WritePassword
vOptional, //VARIANT* ReadOnlyRecommended
vOptional, //VARIANT* EmbedTrueTypeFonts
vOptional, //VARIANT* SaveNativePictureFormat
vOptional, //VARIANT* SaveFormsData
vOptional, //VARIANT* SaveAsAOCELetter
vOptional, //VARIANT* ReadOnlyRecommended
vOptional, //VARIANT* EmbedTrueTypeFonts
vOptional, //VARIANT* SaveNativePictureFormat
vOptional, //VARIANT* SaveFormsData
vOptional //VARIANT* SaveAsAOCELetter
);
return TRUE;
}
BOOL CWordOperate::CloseDocument()
{
COleVariant vTrue((short)TRUE),
vFalse((short)FALSE),
vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR);
m_wdDoc.Close(vFalse, // SaveChanges.
vTrue, // OriginalFormat.
vFalse // RouteDocument.
);
//AfxMessageBox("c1");
m_wdDoc.AttachDispatch(m_wdApp.GetActiveDocument());
if (!m_wdDoc.m_lpDispatch)
{
AfxMessageBox("Document获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
// AfxMessageBox("c2");
//得到selection变量
m_wdSel = m_wdApp.GetSelection();
if (!m_wdSel.m_lpDispatch)
{
AfxMessageBox("Select获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
// AfxMessageBox("c3");
//得到全部DOC的Range变量
m_wdRange = m_wdDoc.Range(vOptional,vOptional);
if(!m_wdRange.m_lpDispatch)
{
AfxMessageBox("Range获取失败!", MB_OK|MB_ICONWARNING);
return FALSE;
}
// AfxMessageBox("c4");
return TRUE;
}
void CWordOperate::CloseApp()
{
COleVariant vTrue((short)TRUE),
vFalse((short)FALSE),
vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR);
m_wdDoc.Save();
m_wdApp.Quit(vFalse, // SaveChanges.
vTrue, // OriginalFormat.
vFalse // RouteDocument.
);
//释放内存申请资源
m_wdRange.ReleaseDispatch();
m_wdSel.ReleaseDispatch();
m_wdDoc.ReleaseDispatch();
m_wdDocs.ReleaseDispatch();
m_wdApp.ReleaseDispatch();
}
void CWordOperate::WriteText(CString szText)
{
m_wdSel.TypeText(szText);
}
void CWordOperate::WriteNewLineText(CString szText, int nLineCount /**//* = 1 */)
{
int i;
if (nLineCount <= 0)
{
nLineCount = 0;
}
for (i = 0; i < nLineCount; i++)
{
m_wdSel.TypeParagraph();
}
WriteText(szText);
}
void CWordOperate::WriteEndLine(CString szText)
{
m_wdRange.InsertAfter(szText);
}
void CWordOperate::WholeStory()
{
m_wdRange.WholeStory();
}
void CWordOperate::Copy()
{
m_wdSel.Copy();
//m_wdSel.CopyFormat();
}
void CWordOperate::TypeParagraph()
{
m_wdSel.TypeParagraph();
}
void CWordOperate::PasteAndFormat()
{
m_wdSel.PasteAndFormat(0);
}
void CWordOperate::Paste()
{
m_wdSel.Paste();
//m_wdSel.PasteFormat();
}
void CWordOperate::TypeBackspace(int count)
{
for(int i = 0; i < count; i++)
m_wdSel.TypeBackspace();
}
void CWordOperate::InsertFile(CString fileName) { COleVariant vFileName(fileName), vTrue((short)TRUE), vFalse((short)FALSE), vOptional((long)DISP_E_PARAMNOTFOUND, VT_ERROR), vNull(_T("")); /**//* void InsertFile(LPCTSTR FileName, VARIANT* Range, VARIANT* ConfirmConversions, VARIANT* Link, VARIANT* Attachment); */ m_wdSel.InsertFile( fileName, vNull, vFalse, vFalse, vFalse ); } void CWordOperate::SelectMoveDown(short lineCount, short unit)//有选择操作的移动 { m_wdSel.MoveDown(COleVariant(unit), COleVariant((short)lineCount),COleVariant((short)wdExtend)); } void CWordOperate::NoneSelectMoveDown(short lineCount, short unit)//仅仅移动光标,不选中 { m_wdSel.MoveDown(COleVariant(unit), COleVariant((short)lineCount),COleVariant((short)wdMove)); } void CWordOperate::SelectMoveUp(short lineCount, short unit)//有选择操作的移动 { m_wdSel.MoveUp(COleVariant(unit), COleVariant((short)lineCount),COleVariant((short)wdExtend)); } void CWordOperate::NoneSelectMoveUp(short lineCount, short unit)//仅仅移动光标,不选中 { m_wdSel.MoveUp(COleVariant(unit), COleVariant((short)lineCount),COleVariant((short)wdMove)); } void CWordOperate::SelectMoveLeft(short charCount, short unit)//有选择操作的移动 { m_wdSel.MoveLeft(COleVariant(unit), COleVariant((short)charCount),COleVariant((short)wdExtend)); } void CWordOperate::NoneSelectMoveLeft(short charCount, short unit)// { m_wdSel.MoveLeft(COleVariant(unit), COleVariant((short)charCount),COleVariant((short)wdMove)); } void CWordOperate::SelectMoveRight(short charCount, short unit)//有选择操作的移动 { m_wdSel.MoveRight(COleVariant(unit), COleVariant((short)charCount),COleVariant((short)wdExtend)); } void CWordOperate::NoneSelectMoveRight(short charCount, short unit)// { m_wdSel.MoveRight(COleVariant(unit), COleVariant((short)charCount),COleVariant((short)wdMove)); } void CWordOperate::MoveToFirst() { m_wdSel.GoTo(COleVariant((short)1), COleVariant((short)2), COleVariant((short)0), COleVariant("1")); } void CWordOperate::MoveToNextPage() { m_wdSel.GoTo(COleVariant((short)1), COleVariant((short)2), COleVariant((short)1), COleVariant("")); }
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- 不借助 Wine 和云服务:新项目能让 Linux 完整运行微软 Office 套件
- 关于指针的一些事情
- WPS Office:Linux 上的 Microsoft Office 的免费替代品
- c++ primer 第五版 笔记前言
- [软件咨询]WPS2012正式版已发布 金山Office移动版4.0发布
- share_ptr的几个注意点
- 用Coldfusion生成 OFFICE 文件的代码
- 重现 Office 2007 中的菜单和工具栏的方法
- Lua中调用C++函数示例
- Lua教程(一):在C++中嵌入Lua脚本
- Lua教程(二):C++和Lua相互传递数据示例
- 微软Word 2007数学插件 Microsoft Math 提供下载
- Office Word九条常用技巧
- Microsoft Office 2007 SP1 简体中文正式版 升级包官方下载地址
- 官方 WPS office 2005 个人精装版 下载
- C#实现简单合并word文档的方法
- powershell操作word详解
- C#生成Word文档代码示例