PyQt圆形按钮
2016-07-19 00:00
387 查看
不规则形状的控件一直是图形界面设计的不可缺少的一项,但是有些控件的不规则实现却比较困难。这篇文字专注于圆形按钮的实现,此实现方法比较简单,没有任何难点,且看实现效果。
按钮初始图片:

Hovered图片:
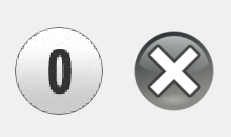
Pressed图片:

当然,将图片设置为rgb色值也可以,只需要稍稍修改即可。
圆形按钮实现的关键代码只有一句:
painter_path.addEllipse(1,1, button_rect.width()-2, button_rect.height()-2)
painter.setClipPath(painter_path)
稍微百度或者其他搜索一下,即可知道addEllipse方法是得到一个椭圆,而圆是椭圆的一个特殊例子,具体实现方法,请仔细参阅源代码。
本实例的运行效果如下图:
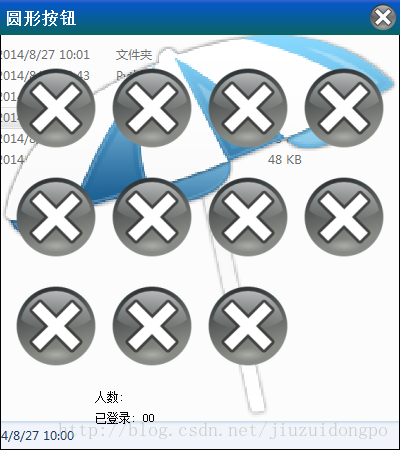
主要按钮实现源码如下:
#coding:utf-8
'''
Created on 2014年8月26日
@author : GuoWu
'''
from PyQt4.QtGui import *
from PyQt4.QtCore import *
class AeroButton(QPushButton):
def __init__(self,a,b,c,parent=None):
super(AeroButton,self).__init__(parent)
#self.setEnabled(True)
self.a = a
self.b = b
self.c = c
self.hovered = False
self.pressed = False
self.color = QColor(Qt.gray)
self.hightlight = QColor(Qt.lightGray)
self.shadow = QColor(Qt.black)
self.opacity = 1.0
self.roundness = 0
def paintEvent(self,event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
if (self.isEnabled()):
if self.hovered:
self.color=self.hightlight.darker(250)
else:
self.color = QColor(50,50,50)
button_rect = QRect(self.geometry())
painter.setPen(QPen(QBrush(Qt.red),2.0))
painter_path = QPainterPath()
#painter_path.addRoundedRect(1, 1, button_rect.width() - 2, button_rect.height() - 2, self.roundness, self.roundness)
painter_path.addEllipse(1, 1, button_rect.width()-2, button_rect.height()-2)
painter.setClipPath(painter_path)
if self.isEnabled():
if (self.pressed == False and self.hovered == False):
icon_size = self.iconSize()
icon_position = self.calculateIconPosition(button_rect,icon_size)
painter.setOpacity(1.0)
painter.drawPixmap(icon_position, QPixmap(QIcon(self.a).pixmap(icon_size)))
elif (self.hovered == True and self.pressed == False):
icon_size = self.iconSize()
icon_position = self.calculateIconPosition(button_rect,icon_size)
painter.setOpacity(1.0)
painter.drawPixmap(icon_position, QPixmap(QIcon(self.b).pixmap(icon_size)))
elif self.pressed == True:
icon_size = self.iconSize()
icon_position = self.calculateIconPosition(button_rect,icon_size)
painter.setOpacity(1.0)
painter.drawPixmap(icon_position, QPixmap(QIcon(self.c).pixmap(icon_size)))
else:
icon_size = self.iconSize()
icon_position = self.calculateIconPosition(button_rect,icon_size)
painter.setOpacity(1.0)
painter.drawPixmap(icon_position, QPixmap(QIcon(self.a).pixmap(icon_size)))
def enterEvent(self,event):
self.hovered = True
self.repaint()
QPushButton.enterEvent(self,event)
def leaveEvent(self,event):
self.hovered = False;
self.repaint()
QPushButton.leaveEvent(self,event)
def mousePressEvent(self,event):
self.pressed = True
self.repaint()
QPushButton.mousePressEvent(self,event)
def mouseReleaseEvent(self,event):
self.pressed = False
self.repaint()
QPushButton.mouseReleaseEvent(self,event)
def calculateIconPosition(self,button_rect,icon_size):
x = (button_rect.width() / 2) - (icon_size.width() / 2)
y = (button_rect.height() / 2) - (icon_size.height() / 2)
width = icon_size.width()
height = icon_size.height()
icon_position = QRect()
icon_position.setX(x)
icon_position.setY(y)
icon_position.setWidth(width)
icon_position.setHeight(height)
return icon_position
本文参考某个Qt的圆形按钮实现程序,对其表示感谢。
技术略低,不喜勿喷。
邮箱528081202@qq.com,求交流指教。
按钮初始图片:

Hovered图片:
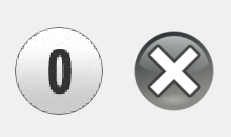
Pressed图片:

当然,将图片设置为rgb色值也可以,只需要稍稍修改即可。
圆形按钮实现的关键代码只有一句:
painter_path.addEllipse(1,1, button_rect.width()-2, button_rect.height()-2)
painter.setClipPath(painter_path)
稍微百度或者其他搜索一下,即可知道addEllipse方法是得到一个椭圆,而圆是椭圆的一个特殊例子,具体实现方法,请仔细参阅源代码。
本实例的运行效果如下图:
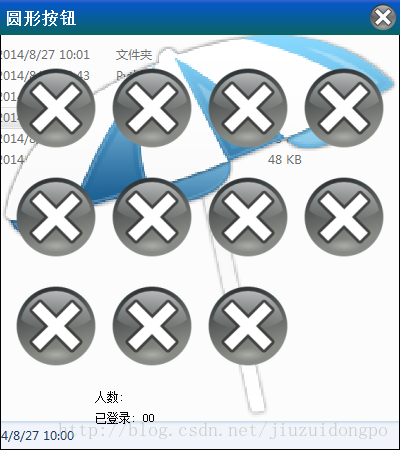
主要按钮实现源码如下:
#coding:utf-8
'''
Created on 2014年8月26日
@author : GuoWu
'''
from PyQt4.QtGui import *
from PyQt4.QtCore import *
class AeroButton(QPushButton):
def __init__(self,a,b,c,parent=None):
super(AeroButton,self).__init__(parent)
#self.setEnabled(True)
self.a = a
self.b = b
self.c = c
self.hovered = False
self.pressed = False
self.color = QColor(Qt.gray)
self.hightlight = QColor(Qt.lightGray)
self.shadow = QColor(Qt.black)
self.opacity = 1.0
self.roundness = 0
def paintEvent(self,event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
if (self.isEnabled()):
if self.hovered:
self.color=self.hightlight.darker(250)
else:
self.color = QColor(50,50,50)
button_rect = QRect(self.geometry())
painter.setPen(QPen(QBrush(Qt.red),2.0))
painter_path = QPainterPath()
#painter_path.addRoundedRect(1, 1, button_rect.width() - 2, button_rect.height() - 2, self.roundness, self.roundness)
painter_path.addEllipse(1, 1, button_rect.width()-2, button_rect.height()-2)
painter.setClipPath(painter_path)
if self.isEnabled():
if (self.pressed == False and self.hovered == False):
icon_size = self.iconSize()
icon_position = self.calculateIconPosition(button_rect,icon_size)
painter.setOpacity(1.0)
painter.drawPixmap(icon_position, QPixmap(QIcon(self.a).pixmap(icon_size)))
elif (self.hovered == True and self.pressed == False):
icon_size = self.iconSize()
icon_position = self.calculateIconPosition(button_rect,icon_size)
painter.setOpacity(1.0)
painter.drawPixmap(icon_position, QPixmap(QIcon(self.b).pixmap(icon_size)))
elif self.pressed == True:
icon_size = self.iconSize()
icon_position = self.calculateIconPosition(button_rect,icon_size)
painter.setOpacity(1.0)
painter.drawPixmap(icon_position, QPixmap(QIcon(self.c).pixmap(icon_size)))
else:
icon_size = self.iconSize()
icon_position = self.calculateIconPosition(button_rect,icon_size)
painter.setOpacity(1.0)
painter.drawPixmap(icon_position, QPixmap(QIcon(self.a).pixmap(icon_size)))
def enterEvent(self,event):
self.hovered = True
self.repaint()
QPushButton.enterEvent(self,event)
def leaveEvent(self,event):
self.hovered = False;
self.repaint()
QPushButton.leaveEvent(self,event)
def mousePressEvent(self,event):
self.pressed = True
self.repaint()
QPushButton.mousePressEvent(self,event)
def mouseReleaseEvent(self,event):
self.pressed = False
self.repaint()
QPushButton.mouseReleaseEvent(self,event)
def calculateIconPosition(self,button_rect,icon_size):
x = (button_rect.width() / 2) - (icon_size.width() / 2)
y = (button_rect.height() / 2) - (icon_size.height() / 2)
width = icon_size.width()
height = icon_size.height()
icon_position = QRect()
icon_position.setX(x)
icon_position.setY(y)
icon_position.setWidth(width)
icon_position.setHeight(height)
return icon_position
本文参考某个Qt的圆形按钮实现程序,对其表示感谢。
技术略低,不喜勿喷。
邮箱528081202@qq.com,求交流指教。
相关文章推荐
- PyQt按钮右键菜单
- PyQt中QLabel背景与字体的一些设置
- PyQt自定义选择输入框(类似QQ登录输入框)
- 利用Qt的QDateTimeEdit设置起始时间(即开始时间大于结束时间)
- vs2010+qt插件,开发i环境搭建
- Qt中一些常用的格式转换
- Qt之窗口动画(下坠、抖动、透明度)(还有好多相关帖子)
- Qt之Windows资源文件(.rc文件)
- Qt学习(四)—实例涂鸦画板mspaint
- QT第一天学习
- qt5集成libcurl实现tftp和ftp的方法之三:搭建FTP时,当ftpServer端默认登录的目录不是根目录/时,有一个bug会产生的解决办法
- Qt之图标切分与合并(关键是使用QPixmap的copy函数来拷贝整张图片的某个区域)
- qt day1
- Qt-crateor 编译 ROS
- 简单的QT绘图程序(把全部的点都记录下来,然后在paintEvent里使用drawLine函数进行绘制,貌似效率很低。。。)
- VS_QT中配置qDebug输出
- VS2008下搭建QT环境
- QT5程序打包发布
- QT各种乱码解决方案
- 从无到有,从动态链编到静态链编,与清华大学合作完成了一个Qt项目