Java实现“汽车租赁项目”
2016-07-18 11:27
501 查看
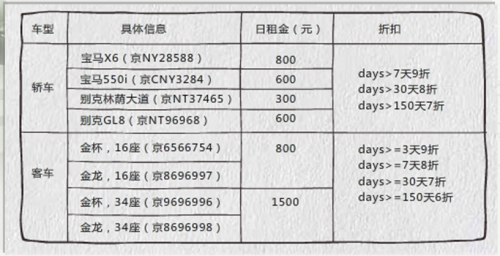
1、创建租车cab父类(抽象)
package study; // 创建抽象租车cab父类 public abstract class cab { // 创建cab具有的公共属性 private String brand; // 车辆的品牌 private String licencePlateNumber; // 车辆的车牌号码 private double dayRent; // 车辆的日租金 // cab的空构造方法 public cab() { } /**cab的有参构造方法 * @param brand * @param licencePlateNumber * @param dayRent */ public cab(String brand, String licencePlateNumber, double dayRent) { this.brand = brand; this.licencePlateNumber = licencePlateNumber; this.dayRent = dayRent; } //创建cab的车辆租赁rent方法 public abstract void rent(); // 创建cab的计算租金的calcRent方法 public abstract void calcRent(); // 创建cab的get、set方法 public String getBrand() { return brand; } public void setBrand(String brand) { this.brand = brand; } public String getLicencePlateNumber() { return licencePlateNumber; } public void setLicencePlateNumber(String licencePlateNumber) { this.licencePlateNumber = licencePlateNumber; } public double getDayRent() { return dayRent; } public void setDayRent(double dayRent) { this.dayRent = dayRent; } }2、创建汽车car子类继承cab父类
package study; import java.util.Scanner; // 创建汽车car子类 public class car extends cab { Scanner input = new Scanner(System.in); int days = 0;// 定义客户租赁的天数 // car的私有属性 private String type; // car的空构造方法 public car() { } /** car的有参构造方法 * @param brand * @param licencePlateNumber * @param dayRent */ public car(String brand, String licencePlateNumber, double dayRent,String type) { super(brand, licencePlateNumber, dayRent); this.type = type ; } // 重写cab的车辆租赁rent方法 public void rent(){ // System.out.println("===========欢迎光临汽车租赁系统=============="+"\n");// 用户提示信息 System.out.println("请输入您要租赁的汽车品牌:1.宝马\t2.别克");// 用户选择提示信息 // 获取用户输入的数字(选项)1或者2 int brand1 = input.nextInt(); // 判断用户输入的数字 switch (brand1){ case 1:// 第一种情况为宝马 super.setBrand("宝马") ; System.out.println("请输入您要租赁的汽车型号:1.X6\t2.550i"); // 提示客户输入信息 int type1 = input.nextInt(); // 获取用户输入信息存储到type1变量中 if(type1==1){ this.type = "X6"; super.setLicencePlateNumber("京NY28588") ; super.setDayRent(800); }else{ type = "550i"; super.setLicencePlateNumber("京CNY3284"); super.setDayRent(600); } break; case 2:// 第一种情况为别克 super.setBrand("别克") ; System.out.println("请输入您要租赁的汽车型号:1.林荫大道\t2.GL8"); // 提示客户输入信息 int type2 = input.nextInt();// 获取用户输入信息存储到type2变量中 if(type2==1){ this.type = "林荫大道"; super.setLicencePlateNumber("京NT37465"); super.setDayRent(300); }else{ this.type = "GL8"; super.setLicencePlateNumber("京NT96968"); super.setDayRent(600); } break; default: } } // 重写租金计算的方法 public void calcRent(){ // double discount = 1.0;// 定义折扣变量并赋初始值为1,即不打折扣 System.out.println("请输入您要租赁的天数:");// 提示用户输入租赁的天数 days = input.nextInt();// 将获取的天数存储到days变量中 if(days<=7){ System.out.println("您成功的租赁了"+super.getBrand()+"\t"+type+" ;"+"车牌号为:"+super.getLicencePlateNumber()+" "+";"+"您需要支付的金额为:"+super.getDayRent()*(days)*1+"元人民币。"); }else if(7<days&&days<=30){ System.out.println("您成功的租赁了"+super.getBrand()+"\t"+type+" ;"+"车牌号为:"+super.getLicencePlateNumber()+" "+";"+"您需要支付的金额为:"+super.getDayRent()*(days)*(0.9)+"元人民币。"); }else if(30<days&&days<=150){ System.out.println("您成功的租赁了"+super.getBrand()+"\t"+type+" ;"+"车牌号为:"+super.getLicencePlateNumber()+" "+";"+"您需要支付的金额为:"+super.getDayRent()*(days)*(0.8)+"元人民币。"); }else{ System.out.println("您成功的租赁了"+super.getBrand()+"\t"+type+" ;"+"车牌号为:"+super.getLicencePlateNumber()+" "+";"+"您需要支付的金额为:"+super.getDayRent()*(days)*(0.7)+"元人民币。"); } } // car的get、set方法 public String getType() { return type; } public void setType(String type) { this.type = type; } }3、创建测试类一
package study; // 创建测试类 public class Demo { public static void main(String[] args) { cab car = new car (); car.rent(); car.calcRent(); } }编译结果一:
===========欢迎光临汽车租赁系统============== 请输入您要租赁的汽车品牌:1.宝马 2.别克 2 请输入您要租赁的汽车类型:1.林荫大道 2.GL8 1 请输入您要租赁的天数: 1 您成功的租赁了别克 林荫大道 ;车牌号为:京NT37465 ;您需要支付的金额为:300.0元人民币。4、增加一个bus子类
package study; import java.util.Scanner; //创建客车bus子类 public class bus extends cab { Scanner input = new Scanner(System.in); int days = 0;// 定义客户租赁的天数 // bus的私有属性 private int seat; // bus的空构造方法 public bus() { } /** bus的有参构造方法 * @param brand * @param licencePlateNumber * @param dayRent */ public bus(String brand, String licencePlateNumber, double dayRent,int seat) { super(brand, licencePlateNumber, dayRent); this.seat = seat ; } // 重写bus的车辆租赁rent方法 public void rent(){ // System.out.println("===========欢迎光临汽车租赁系统=============="+"\n");// 用户提示信息 System.out.println("请输入您要租赁的客车品牌:1.金杯\t2.金龙");// 用户选择提示信息 // 获取用户输入的数字(选项)1或者2 int brand1 = input.nextInt(); // 判断用户输入的数字 switch (brand1){ case 1:// 第一种情况为金杯 super.setBrand("金杯") ; System.out.println("请输入您要租赁的客车座位数:1.16\t2.34"); // 提示客户输入信息 int seat1 = input.nextInt(); // 获取用户输入信息存储到type1变量中 if(seat1==1){ this.seat = 16; super.setLicencePlateNumber("京6566754") ; super.setDayRent(800); }else{ this.seat = 34; super.setLicencePlateNumber("京9696996"); super.setDayRent(1500); } break; case 2:// 第一种情况为金龙 super.setBrand("金龙") ; System.out.println("请输入您要租赁的汽车型号:1.16\t2.34"); // 提示客户输入信息 int seat2 = input.nextInt();// 获取用户输入信息存储到type2变量中 if(seat2==1){ this.seat = 16; super.setLicencePlateNumber("京8696997"); super.setDayRent(300); }else{ this.seat = 34; super.setLicencePlateNumber("京8696998"); super.setDayRent(1500); } break; default: } } // 重写租金计算的方法 public void calcRent(){ // double discount = 1.0;// 定义折扣变量并赋初始值为1,即不打折扣 System.out.println("请输入您要租赁的天数:");// 提示用户输入租赁的天数 days = input.nextInt();// 将获取的天数存储到days变量中 if(days<=7){ System.out.println("您成功的租赁了"+super.getBrand()+"\t"+seat+" ;"+"车牌号为:"+super.getLicencePlateNumber()+" "+";"+"您需要支付的金额为:"+super.getDayRent()*(days)*1+"元人民币。"); }else if(7<days&&days<=30){ System.out.println("您成功的租赁了"+super.getBrand()+"\t"+seat+" ;"+"车牌号为:"+super.getLicencePlateNumber()+" "+";"+"您需要支付的金额为:"+super.getDayRent()*(days)*(0.9)+"元人民币。"); }else if(30<days&&days<=150){ System.out.println("您成功的租赁了"+super.getBrand()+"\t"+seat+" ;"+"车牌号为:"+super.getLicencePlateNumber()+" "+";"+"您需要支付的金额为:"+super.getDayRent()*(days)*(0.8)+"元人民币。"); }else{ System.out.println("您成功的租赁了"+super.getBrand()+"\t"+seat+" ;"+"车牌号为:"+super.getLicencePlateNumber()+" "+";"+"您需要支付的金额为:"+super.getDayRent()*(days)*(0.7)+"元人民币。"); } } // car的get、set方法 public int getSeat() { return seat; } public void setSeat(int seat) { this.seat = seat; } }修改测试类
package study; import java.util.Scanner; // 创建测试类 public class Demo { public static void main(String[] args) { System.out.println("===========欢迎光临汽车租赁系统=============="+"\n"); System.out.println("请输入您要租赁的车辆类型:1.汽车\t2.客车"); Scanner input = new Scanner(System.in); int type = input.nextInt(); if(type==1){ cab car = new car (); car.rent(); car.calcRent(); }else{ cab bus = new bus (); bus.rent(); bus.calcRent(); } } }编译结果二:
===========欢迎光临汽车租赁系统============== 请输入您要租赁的车辆类型:1.汽车 2.客车 1 请输入您要租赁的汽车品牌:1.宝马 2.别克 1 请输入您要租赁的汽车型号:1.X6 2.550i 1 请输入您要租赁的天数: 23 您成功的租赁了宝马X6 ;车牌号为:京NY28588 ;您需要支付的金额为:16560.0元人民币。
本文出自 “技术进阶” 博客,请务必保留此出处http://vipnoon.blog.51cto.com/7589908/1827335
相关文章推荐
- java-守护线程与非守护线程
- java学习笔记2
- JAVA list+for循环实现分页
- java四种对象引用类型区别
- 精简JRE
- Spring mvc + pushlet实现向特定用户实时推送信息
- SpringMVC简单的登录
- javaweb基础(37)_mysql数据库自动生成主键
- struts2中redirect action的参数传递
- ScheduleThreadPoolExecutor源码分析
- Spring基础——在 Spring Config 文件中基于 XML 的 Bean 的自动装配
- spring配置: Annotation vs XML
- 【spring 5】AOP:spring中对于AOP的的实现
- 【spring 5】AOP:spring中对于AOP的的实现
- java使用listIterator逆序arraylist示例分享
- java中使用sax解析xml,以实体类集合的方式接受xml解析的值
- 优先队列及java实现和用法
- Struts2:前后台的数据交互
- spring事务的理解与测试
- Eclipse中添加Github上的项目