JAVA Netty例子
2016-07-13 21:21
375 查看
Netty jar : https://bintray.com/netty/downloads/netty/5.0.0.Alpha2
类图(代码详见下方):
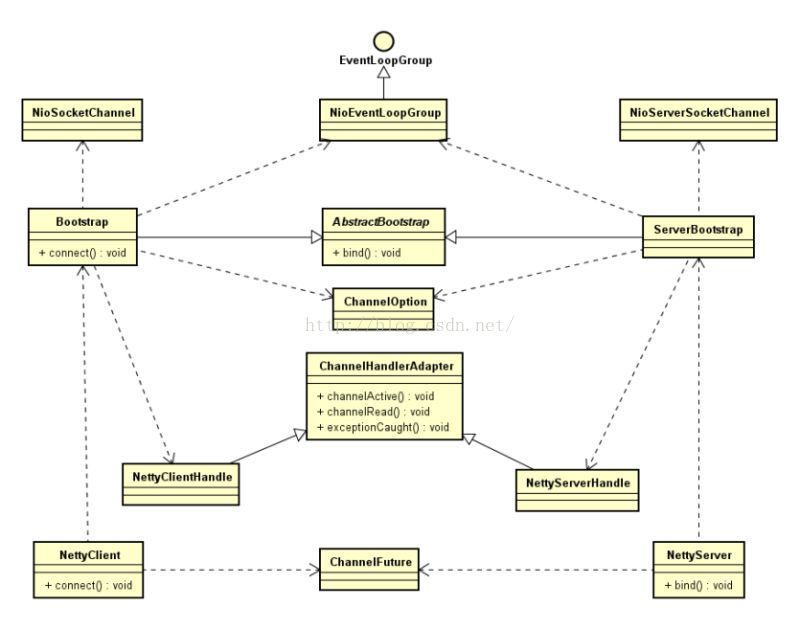
NettyServer.java
NettyServerHandle.java
NettyClient.java
NettyClientHandle.java
类图(代码详见下方):
NettyServer.java
package netty; import io.netty.bootstrap.ServerBootstrap; import io.netty.channel.ChannelFuture; import io.netty.channel.ChannelInitializer; import io.netty.channel.ChannelOption; import io.netty.channel.EventLoopGroup; import io.netty.channel.nio.NioEventLoopGroup; import io.netty.channel.socket.SocketChannel; import io.netty.channel.socket.nio.NioServerSocketChannel; public class NettyServer { public void bind(int port){ EventLoopGroup bossGroup = new NioEventLoopGroup() ; EventLoopGroup workGroup = new NioEventLoopGroup() ; try { ServerBootstrap b = new ServerBootstrap(); b.group(bossGroup,workGroup) .channel(NioServerSocketChannel.class) .option(ChannelOption.SO_BACKLOG, 1024) .childHandler(new ChannelInitializer<SocketChannel>() { @Override protected void initChannel(SocketChannel socketChannel) throws Exception { socketChannel.pipeline().addLast(new NettyServerHandle()); } }); ChannelFuture f = b.bind(port).sync(); f.channel().closeFuture().sync(); } catch (InterruptedException e) { e.printStackTrace(); } finally { bossGroup.shutdownGracefully(); workGroup.shutdownGracefully(); } } public static void main(String[] args) { new NettyServer().bind(1986); } }
NettyServerHandle.java
package netty; import io.netty.buffer.ByteBuf; import io.netty.buffer.Unpooled; import io.netty.channel.ChannelHandlerAdapter; import io.netty.channel.ChannelHandlerContext; public class NettyServerHandle extends ChannelHandlerAdapter { @Override public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception { ByteBuf buf = (ByteBuf)msg; byte[] req = new byte[buf.readableBytes()]; buf.readBytes(req); String body = new String(req,"UTF-8"); System.out.println("receive : " + body); String send = body + "2"; ByteBuf sendBuf = Unpooled.copiedBuffer(send.getBytes()); ctx.write(sendBuf); } @Override public void channelReadComplete(ChannelHandlerContext ctx) throws Exception { ctx.flush(); } @Override public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception { ctx.close(); } }
NettyClient.java
package netty; import io.netty.bootstrap.Bootstrap; import io.netty.channel.ChannelFuture; import io.netty.channel.ChannelInitializer; import io.netty.channel.ChannelOption; import io.netty.channel.EventLoopGroup; import io.netty.channel.nio.NioEventLoopGroup; import io.netty.channel.socket.SocketChannel; import io.netty.channel.socket.nio.NioSocketChannel; public class NettyClient { public void connect(int port,String host){ EventLoopGroup workGroup = new NioEventLoopGroup() ; try { Bootstrap b = new Bootstrap(); b.group(workGroup) .channel(NioSocketChannel.class) .option(ChannelOption.TCP_NODELAY, true); b.handler(new ChannelInitializer<SocketChannel>() { @Override protected void initChannel(SocketChannel socketChannel) throws Exception { socketChannel.pipeline().addLast(new NettyClientHandle()); } }); ChannelFuture f = b.connect(host, port).sync(); f.channel().closeFuture().sync(); } catch (InterruptedException e) { e.printStackTrace(); } finally { workGroup.shutdownGracefully(); } } public static void main(String[] args) { new NettyClient().connect(1986,"127.0.0.1"); } }
NettyClientHandle.java
package netty; import io.netty.buffer.ByteBuf; import io.netty.buffer.Unpooled; import io.netty.channel.ChannelHandlerAdapter; import io.netty.channel.ChannelHandlerContext; import java.io.UnsupportedEncodingException; public class NettyClientHandle extends ChannelHandlerAdapter { private ByteBuf clientBuf; public NettyClientHandle() { String send = null; try { send = new String("1".getBytes(),"UTF-8"); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } System.out.println("client init: " + send); clientBuf = Unpooled.buffer(send.getBytes().length); clientBuf.writeBytes(send.getBytes()); } @Override public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception { ByteBuf buf = (ByteBuf)msg; byte[] req = new byte[buf.readableBytes()]; buf.readBytes(req); // 加上这句,可以避免乱码 String body = new String(req,"UTF-8"); System.out.println("now is : " + body); } @Override public void channelActive(ChannelHandlerContext ctx) throws Exception { System.out.println("client send "); ctx.writeAndFlush(clientBuf); } @Override public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception { ctx.close(); } }