Struts2系列:(24) 国际化(二)案例-快速入门
2016-07-05 01:23
771 查看
1、案例
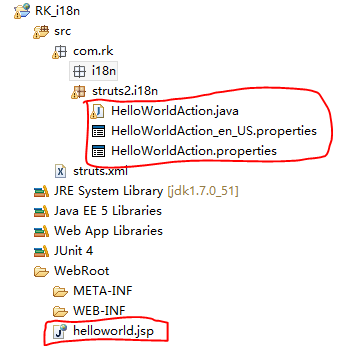
1.1、创建Action类
在com.rk.struts2.i18n包下创建HelloWorldAction.java文件,内容如下:
1.2、创建properties文件,存储国际化数据
在com.rk.struts2.i18n包下创建2个properties文件:HelloWorldAction.properties和HelloWorldAction_en_US.properties。
HelloWorldAction.properties
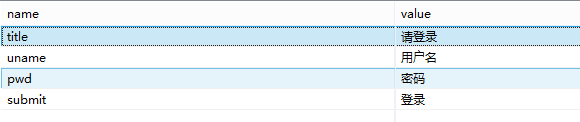
HelloWorldAction_en_US.properties
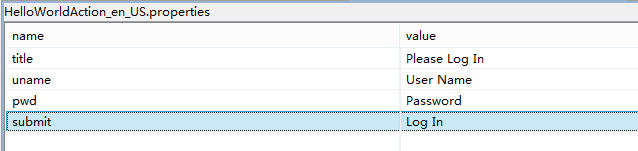
【扩展阅读】
Struts2提供了放置properties文件位置的选择,其中一种就是将properties文件放置在action class同一个包内,并且properties文件的名字也与action class文件一致。
Struts 2 provides many options for where and how you make your properties files available. One of the options is to associate the properties file, by name, with the action class. When Struts 2 creates the ResourceBundles that it makes available to a given request, one of the first bundles it attempts to instantiate is a bundle with a name that matches the current action.
通常我们要提供一个default properties file。下面是解释这样做带来的好处。
There’s a good reason for this. We’re assuming that English is the default language for the application. If you want to make another language the default language, then it should go into the default properties file. If a request comes in that specifies an unsupported locale, then the default locale will be used. If we had put the English language resources in a PortfolioHomePage_en.properties file and left the default properties file empty, then requests for unsupported languages would have nothing to fall back on. Thus, it’s always important to use the default properties file for your default language.
当我们创建好这些properties文件之后,将这些文件放置在action class所在的package下面。Struts2 framework,在每一次接收到request请求时,就会自动初始化properties文件,它还会根据浏览器提供了Locale信息创建ResourceBundle对象。
Once we’ve created these files, we just need to put them into the package directory structure next to the action class itself. With these two files in place, the framework automatically instantiates the PortfolioHomePage ResourceBundle every time a request comes in for the PortfolioHomePage action. Furthermore, the framework will have automatically determined the user’s locale based upon information sent from the browser, and created the ResourceBundle accordingly.
1.3、在JSP中获取国际化数据
在WebRoot目录下,建立helloworld.jsp文件,内容如下:
最后发布项目,进行访问 http://127.0.0.1:8080/i18n/helloworld.action
【扩展阅读】
接下来,看我们如何取出国际化文件的值。
Now, let’s see how we can pull messages out of those bundles. The following snippet shows the most common way of retrieving localized text, the Struts 2 text tag:
As you can see, the Struts 2 text tag is simple. It’s similar to the Struts 2 property tag, but it takes a ResourceBundle key instead of an OGNL expression.
Now, we’ve replaced that locale-sensitive message with a Struts 2 text tag that retrieves a ResourceBundle message based on the key passed in to the name attribute.
2、Struts2的i18n功能的背后实现机制
在上面的案例当中,我们可以看到使用Struts2的i18n功能是非常容易的:只需要提供properties文件,并且在JSP页面进行取值就可以了。接下来,我们看一下背后的实现机制。可能你已经猜到了,这与一组接口和ValueStack有关系。我们首先要介绍的就是com.opensymphony.xwork2.TextProvider接口。
In the previous demonstration, we saw how easy it can be to take advantage of the i18n features of Struts 2 to provide localized message text in your pages. We now take a look at the framework mechanics that drive this. As you might’ve guessed, it’s going to involve a couple of interfaces and the ValueStack. First, we introduce the main character in this mystery, a shady guy known as the TextProvider.
com.opensymphony.xwork2.TextProvider接口提供了getText()的重载方法,这个方法的主要功能是根据key从ResourceBundle中获取相应的国际化资源数据。在Struts2框架中,ActionSupport类实现了TextProvider接口,因此如果我们写的Action class继承自ActionSupport,就能够自动获得Struts2的i18n功能的支持。
The com.opensymphony.xwork2.TextProvider interface exposes an overloaded method getText(). The many versions of this method have at their heart the retrieval of a message value based on a key. In other words, the TextProvider is the guy who takes your key and tracks down an associated message text from the ResourceBundles that it knows about. This guy pretty much handles all of the i18n duties of the framework. While you can always implement your own TextProvider, a default implementation is provided by the framework. The helper class ActionSupport provides this default implementation. Thus, as long as you have your action classes extend ActionSupport, you automatically get the built-in i18n support.
我们知道,当Struts2框架处理request时,第一件要做的事情就是创建action的对象,并把action对象放入到ValueStack上。如果我们的action class继承自ActionSupport类,那么TextProvider也会被自动放入到ValueStack上。ActionSupport类提供了对TextProvider接口的默认实现,它会加载与当前action同名的ResouceBundle(同名的properties文件)和其它的文件。
As you already know, when the framework begins processing a request, one of the first things that it does is create the action object and push it onto the ValueStack. If your action extends ActionSupport, you’ve automatically pushed a TextProvider onto the ValueStack as well. Very convenient. The default implementation of the TextProvider loads the ResourceBundles that have names matching the current action, as well as several others.
由于TextProvider在ValueStack上,ResouceBundle上存储的国际化的message信息,就可以被框架的其它部分访问到。例如,Stuts2的text标签就是专门用于国际化的标签,它会向ValueStack上的ResouceBundle提供一个key,从而获取相应的国际化资源数据。
With our TextProvider on the ValueStack, our ResourceBundle messages are available to every part of the framework. The Struts 2 text tag looks for a TextProvider on the ValueStack and asks that provider to retrieve the message based on the key you provide it:
由于TextProvider放置在ValueStack上,我们可以通过OGNL来调用getText()方法。
Since the TextProvider is on the ValueStack, we can also access its getText() method with OGNL. This allows us to pull ResourceBundle messages into many places, including other Struts 2 tags. In order to do this, we take advantage of OGNL’s ability to call methods on objects. The following use of the Struts 2 property tag is absolutely equivalent to the previous Struts 2 text tag:
注意:property的value属性接受的值,并不是字符串类型,它接收OGNL表达式,因此不需要%{expression}进行转换。
Note that the value attribute of the property tag is a nonstring attribute, thus we don’t have to force OGNL evaluation with the %{expression} syntax.
Struts2实现i18n的思路:(1)(2)(3)
(1)让我们的action类继承自ActionSupport,因此也继承对TextProvider接口的默认实现
(2)创建一些properties文件,注意文件命名和存放位置
(3)使用Struts2的text标签或使用ONGL调用TextProvider的getText()方法获取国际化数据资源
Now, you pretty much know how Struts 2 i18n and l10n work. It’s as easy as 1-2-3.
1 Make your actions extend ActionSupport, so that they inherit the default TextProvider implementation.
2 Put some properties files somewhere they can be found by the default TextProvider, such as in a properties file with a name mirroring the action.
3 Start pulling messages into your pages with the Struts 2 text tag or by hitting the getText() method with direct OGNL.
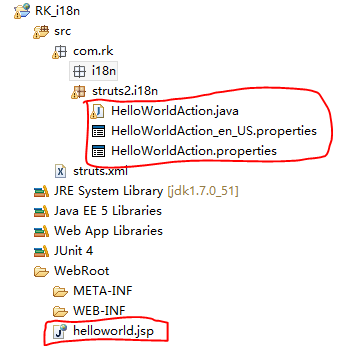
1.1、创建Action类
在com.rk.struts2.i18n包下创建HelloWorldAction.java文件,内容如下:
package com.rk.struts2.i18n; import com.opensymphony.xwork2.ActionSupport; public class HelloWorldAction extends ActionSupport { @Override public String execute() throws Exception { return "success"; } }在struts.xml中进行配置
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.3//EN" "http://struts.apache.org/dtds/struts-2.3.dtd"> <struts> <constant name="struts.enable.DynamicMethodInvocation" value="true" /> <constant name="struts.devMode" value="true" /> <package name="default" namespace="/" extends="struts-default"> <action name="helloworld" class="com.rk.struts2.i18n.HelloWorldAction"> <result name="success">/helloworld.jsp</result> </action> </package> </struts>
1.2、创建properties文件,存储国际化数据
在com.rk.struts2.i18n包下创建2个properties文件:HelloWorldAction.properties和HelloWorldAction_en_US.properties。
HelloWorldAction.properties
title=\u8BF7\u767B\u5F55 uname=\u7528\u6237\u540D pwd=\u5BC6\u7801 submit=\u767B\u5F55
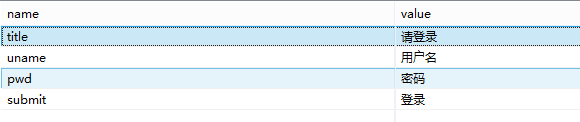
HelloWorldAction_en_US.properties
title=Please Log In uname=User Name pwd=Password submit=Log In
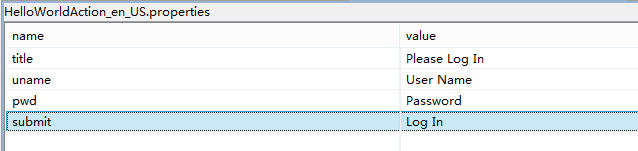
【扩展阅读】
Struts2提供了放置properties文件位置的选择,其中一种就是将properties文件放置在action class同一个包内,并且properties文件的名字也与action class文件一致。
Struts 2 provides many options for where and how you make your properties files available. One of the options is to associate the properties file, by name, with the action class. When Struts 2 creates the ResourceBundles that it makes available to a given request, one of the first bundles it attempts to instantiate is a bundle with a name that matches the current action.
通常我们要提供一个default properties file。下面是解释这样做带来的好处。
There’s a good reason for this. We’re assuming that English is the default language for the application. If you want to make another language the default language, then it should go into the default properties file. If a request comes in that specifies an unsupported locale, then the default locale will be used. If we had put the English language resources in a PortfolioHomePage_en.properties file and left the default properties file empty, then requests for unsupported languages would have nothing to fall back on. Thus, it’s always important to use the default properties file for your default language.
当我们创建好这些properties文件之后,将这些文件放置在action class所在的package下面。Struts2 framework,在每一次接收到request请求时,就会自动初始化properties文件,它还会根据浏览器提供了Locale信息创建ResourceBundle对象。
Once we’ve created these files, we just need to put them into the package directory structure next to the action class itself. With these two files in place, the framework automatically instantiates the PortfolioHomePage ResourceBundle every time a request comes in for the PortfolioHomePage action. Furthermore, the framework will have automatically determined the user’s locale based upon information sent from the browser, and created the ResourceBundle accordingly.
1.3、在JSP中获取国际化数据
在WebRoot目录下,建立helloworld.jsp文件,内容如下:
<%@ page language="java" contentType="text/html; charset=UTF-8"%> <%@taglib uri="/struts-tags" prefix="s" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title><s:text name="title"/></title> </head> <body> <s:text name="uname"/><input type="text"/><br/> <s:text name="pwd"/><input type="password"/><br/> <input type="submit" value="<s:text name="submit"/>"/><br/> </body> </html>
最后发布项目,进行访问 http://127.0.0.1:8080/i18n/helloworld.action
【扩展阅读】
接下来,看我们如何取出国际化文件的值。
Now, let’s see how we can pull messages out of those bundles. The following snippet shows the most common way of retrieving localized text, the Struts 2 text tag:
<s:text name="title"/> <s:text name="uname"/> <s:text name="pwd"/> <s:text name="submit"/>Struts2的text标签和property标签很相似,但是它的name属性中的值是ResouceBundle的key,而不是OGNL表达式。
As you can see, the Struts 2 text tag is simple. It’s similar to the Struts 2 property tag, but it takes a ResourceBundle key instead of an OGNL expression.
Now, we’ve replaced that locale-sensitive message with a Struts 2 text tag that retrieves a ResourceBundle message based on the key passed in to the name attribute.
2、Struts2的i18n功能的背后实现机制
在上面的案例当中,我们可以看到使用Struts2的i18n功能是非常容易的:只需要提供properties文件,并且在JSP页面进行取值就可以了。接下来,我们看一下背后的实现机制。可能你已经猜到了,这与一组接口和ValueStack有关系。我们首先要介绍的就是com.opensymphony.xwork2.TextProvider接口。
In the previous demonstration, we saw how easy it can be to take advantage of the i18n features of Struts 2 to provide localized message text in your pages. We now take a look at the framework mechanics that drive this. As you might’ve guessed, it’s going to involve a couple of interfaces and the ValueStack. First, we introduce the main character in this mystery, a shady guy known as the TextProvider.
com.opensymphony.xwork2.TextProvider接口提供了getText()的重载方法,这个方法的主要功能是根据key从ResourceBundle中获取相应的国际化资源数据。在Struts2框架中,ActionSupport类实现了TextProvider接口,因此如果我们写的Action class继承自ActionSupport,就能够自动获得Struts2的i18n功能的支持。
public class ActionSupport implements Action, Validateable, ValidationAware, TextProvider, LocaleProvider, Serializable { //... }
The com.opensymphony.xwork2.TextProvider interface exposes an overloaded method getText(). The many versions of this method have at their heart the retrieval of a message value based on a key. In other words, the TextProvider is the guy who takes your key and tracks down an associated message text from the ResourceBundles that it knows about. This guy pretty much handles all of the i18n duties of the framework. While you can always implement your own TextProvider, a default implementation is provided by the framework. The helper class ActionSupport provides this default implementation. Thus, as long as you have your action classes extend ActionSupport, you automatically get the built-in i18n support.
我们知道,当Struts2框架处理request时,第一件要做的事情就是创建action的对象,并把action对象放入到ValueStack上。如果我们的action class继承自ActionSupport类,那么TextProvider也会被自动放入到ValueStack上。ActionSupport类提供了对TextProvider接口的默认实现,它会加载与当前action同名的ResouceBundle(同名的properties文件)和其它的文件。
As you already know, when the framework begins processing a request, one of the first things that it does is create the action object and push it onto the ValueStack. If your action extends ActionSupport, you’ve automatically pushed a TextProvider onto the ValueStack as well. Very convenient. The default implementation of the TextProvider loads the ResourceBundles that have names matching the current action, as well as several others.
由于TextProvider在ValueStack上,ResouceBundle上存储的国际化的message信息,就可以被框架的其它部分访问到。例如,Stuts2的text标签就是专门用于国际化的标签,它会向ValueStack上的ResouceBundle提供一个key,从而获取相应的国际化资源数据。
With our TextProvider on the ValueStack, our ResourceBundle messages are available to every part of the framework. The Struts 2 text tag looks for a TextProvider on the ValueStack and asks that provider to retrieve the message based on the key you provide it:
<s:text name="title"/>
由于TextProvider放置在ValueStack上,我们可以通过OGNL来调用getText()方法。
Since the TextProvider is on the ValueStack, we can also access its getText() method with OGNL. This allows us to pull ResourceBundle messages into many places, including other Struts 2 tags. In order to do this, we take advantage of OGNL’s ability to call methods on objects. The following use of the Struts 2 property tag is absolutely equivalent to the previous Struts 2 text tag:
<s:property value="getText('title')"/>
注意:property的value属性接受的值,并不是字符串类型,它接收OGNL表达式,因此不需要%{expression}进行转换。
Note that the value attribute of the property tag is a nonstring attribute, thus we don’t have to force OGNL evaluation with the %{expression} syntax.
Struts2实现i18n的思路:(1)(2)(3)
(1)让我们的action类继承自ActionSupport,因此也继承对TextProvider接口的默认实现
(2)创建一些properties文件,注意文件命名和存放位置
(3)使用Struts2的text标签或使用ONGL调用TextProvider的getText()方法获取国际化数据资源
Now, you pretty much know how Struts 2 i18n and l10n work. It’s as easy as 1-2-3.
1 Make your actions extend ActionSupport, so that they inherit the default TextProvider implementation.
2 Put some properties files somewhere they can be found by the default TextProvider, such as in a properties file with a name mirroring the action.
3 Start pulling messages into your pages with the Struts 2 text tag or by hitting the getText() method with direct OGNL.
相关文章推荐
- JQuery+Strusts1.x无刷新登录
- java struts常见错误以及原因分析
- Struts之logic标签库详解
- 通过实例深入学习Java的Struts框架中的OGNL表达式使用
- Java的Struts框架中append标签与generator标签的使用
- PHP中使用gettext解决国际化问题的例子(i18n)
- struts2的select标签用法实例分析
- SSH框架网上商城项目第20战之在线支付平台
- JavaWeb Struts文件上传功能实现详解
- 在Java的Struts框架下进行web编程的入门教程
- 详解Java的Struts框架中注释的用法
- java中struts 框架的实现
- 利用Java的Struts框架实现电子邮件发送功能
- 在Java的Struts框架中ONGL表达式的基础使用入门
- 详解Java的Struts框架中栈值和OGNL的使用
- Java的Struts框架简介与环境配置教程
- Java的Struts框架中的if/else标签使用详解
- 简单说明Java的Struts框架中merge标签的使用方法
- 详解Java的Struts框架中上传文件和客户端验证的实现
- Java的Struts框架中Action的编写与拦截器的使用方法