webpack configuration
2016-06-23 14:55
483 查看
原来来自 webpack 官网上的 API/Configuration:
http://webpack.github.io/docs/configuration.html
翻译如下:
webpack 需要一个配置对象,取决于你对webpack的使用,有两种方式来传递这个配置对象。
CLI
如果你使用 CLI,它会读取
node.js API
如果你是用的是 node.js API,你需要以参数的形式来传递这个配置对象。
多重配置
In both cases you can also use an array of configurations, which are processed in parallel. They share filesystem cache and watchers so this is more efficent than calling webpack multiple times.
简单的 配置对象 例子:
Default: process.cwd()
If you pass a string: The string is resolved to a module which is loaded upon startup.
If you pass an array: All modules are loaded upon startup. The last one is exported.
If you pass an object: Multiple entry bundles are created. The key is the chunk name. The value can be a string or an array.
NOTE: It is not possible to configure other options specific to entry points. If you need entry point specific configuration you need to use multiple configurations.
single entry
multiple entries
如果你的配置创建了不止一个“chunk”(多个入口或者使用了像 CommonsChunkPlugin 这样的插件),你应该用下面这些来替代以确保每个文件的名字都是唯一的。
[hash] is replaced by the hash of the compilation.
例如嵌入的
当文件的
config.js
index.html
使用 CDN 和 hashes 的更复杂的例子
config.js
Note:为了避免最终的
[id] is replaced by the id of the chunk.
[name] is replaced by the name of the chunk (or with the id when the chunk has no name).
[hash] is replaced by the hash of the compilation.
[chunkhash] is replaced by the hash of the chunk.
数组中每一项可以有下列这些属性:
+
+
+
+
+
A condition may be a RegExp (tested against absolute path), a string containing the absolute path, a function(absPath): bool, or an array of one of these combined with “and”.
IMPORTANT: The loaders here are resolved relative to the resource which they are applied to. This means they are not resolved relative to the configuration file. 如果你有
Example:
一个包含 pre 和 post loaders 的数组。
It’s matched against the full resolved request.
可以改善忽略大的文件夹时的性能。
这些文件并没有必要使用
+ exprContext:一个依赖表达式(i. e. require( expr ) )
+ wrappedContext:An expression plus pre- and/or suffixed string (i. e. require(“./templates/” + expr))
。。。。。后面的不太看得懂啊,不太明白这个选项是干啥的
Example:
一个带有索引的对象,key用来作为模块名,value是新的路径,就像是一次替换但是更聪明,如果key是以$符号结尾的,那么只有严格匹配的才会被替换。
如果 value 是一个相对路径,那么它会相对于包含 require( ) 的那个文件。
Example: 使用不同的alias设置来在
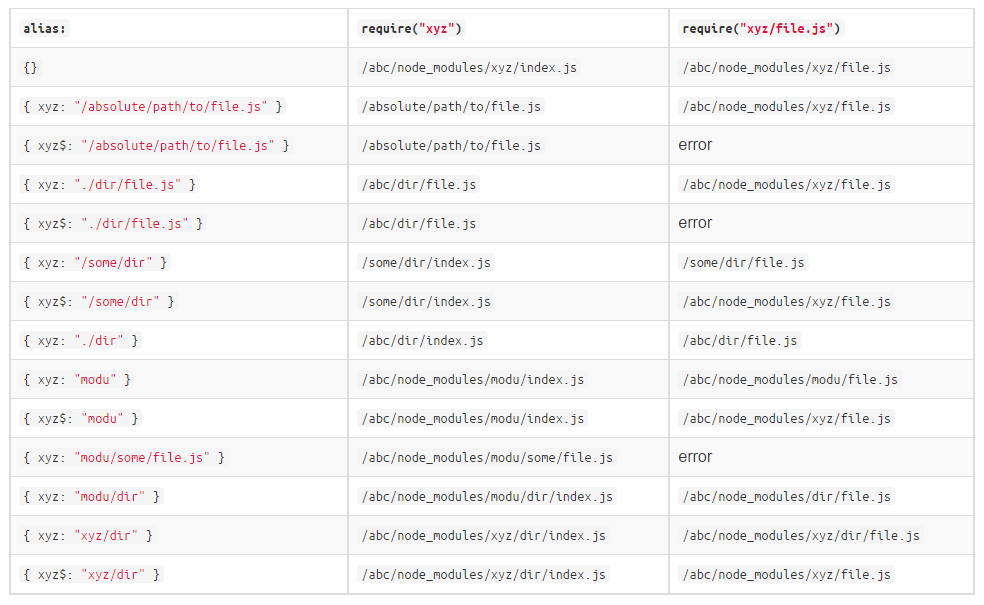
index.js may resolve to another file if defined in the package.json.
/abc/node_modules may resolve in /node_modules too.
必须是绝对路径,不要使用 ./app/modules 这种
Example:
http://webpack.github.io/docs/configuration.html
翻译如下:
webpack 需要一个配置对象,取决于你对webpack的使用,有两种方式来传递这个配置对象。
CLI
如果你使用 CLI,它会读取
webpack.config.js(或者通过
--config参数来指定的文件)。这个文件会输出配置对象。
module.exports = { // configuration };
node.js API
如果你是用的是 node.js API,你需要以参数的形式来传递这个配置对象。
webpack({ // configuration }, callback);
多重配置
In both cases you can also use an array of configurations, which are processed in parallel. They share filesystem cache and watchers so this is more efficent than calling webpack multiple times.
配置对象内容
提示:记住你并不需要在配置中使用纯粹的JSON,你可以使用任何你想要的 JavaScript 语句,它仅仅就是一个 node.js 模块。简单的 配置对象 例子:
{ context: __dirname + "/app", entry: "./entry", output: { path: __dirname + "/dist", filename: "bundle.js" } }
context
The base directory (absolute path!) for resolving the entry option. If output.pathinfo is set, the included pathinfo is shortened to this directory.Default: process.cwd()
entry
打包的入口If you pass a string: The string is resolved to a module which is loaded upon startup.
If you pass an array: All modules are loaded upon startup. The last one is exported.
entry: ["./entry", "./entry2"]
If you pass an object: Multiple entry bundles are created. The key is the chunk name. The value can be a string or an array.
{ entry: { page1: "./page1", page2: ["./entry1", "./entry2"] }, output: { // Make sure to use [name] or [id] in output.filename // when using multiple entry points filename: "[name].bundle.js", chunkFilename: "[id].bundle.js" } }
NOTE: It is not possible to configure other options specific to entry points. If you need entry point specific configuration you need to use multiple configurations.
output
影响编译输出的选项,output选项告诉 webpack 怎样将文件编译到磁盘中。注意,可能会有多个入口(entry),但是只能指定一个 output
output.filename
指定在磁盘中输出的每个文件的名字,不能使用绝对路径!output.path选项决定了文件在磁盘空间中的位置,
filename用来为单个文件命名。
single entry
{ entry: './src/app.js', output: { filename: 'bundle.js', path: __dirname + '/build' } } // wirtes to disk: ./build/bundle.js
multiple entries
如果你的配置创建了不止一个“chunk”(多个入口或者使用了像 CommonsChunkPlugin 这样的插件),你应该用下面这些来替代以确保每个文件的名字都是唯一的。
[name]is replaced by the name of the chunk.
[hash]is replaced by the hash of the compilation.
[chunkhash]is replaced by the hash of the chunk.
{ entry: { app: './src/app.js', search: './src/search.js' }, output: { filename: '[name].js', path: __dirname + '/build' } } // wirtes to disk: ./build/app.js, ./build/search.js
output.path
the output directory as absolute path (required).[hash] is replaced by the hash of the compilation.
output.publicPath
publicPath指定了输出的文件的静态URL地址,当在浏览器中被引用时。
例如嵌入的
<script>或者
<link>标签, 引用的图片等。
当文件的
href或
url()不同于他的本地路径时(
path所指定的),就会用到
publicPath。当你希望在其他的域名或者CDN中使用部分或全部的输出文件时就会非常有用,The Webpack Dev Server also uses this to determine the path where the output files are expected to be served from. As with path you can use the [hash] substitution for a better caching profile.
config.js
output: { path: "/home/proj/public/assets", publicPath: "/assets/" }
index.html
<head> <link href="/assets/spinner.gif" /> </head>
使用 CDN 和 hashes 的更复杂的例子
config.js
output: { path: "/home/proj/cdn/assets/[hash]", publicPath: "http://cdn.example.com/assets/[hash]/" }
Note:为了避免最终的
publicPath在编译的时候无法被识别,it can be left blank and set dynamically at runtime in the entry point file. If you don’t know the publicPath while compiling you can omit it and set webpack_public_path on your entry point.
__webpack_public_path__ = myRuntimePublicPath // rest of your application entry
output.chunkFilename
The filename of non-entry chunks as relative path inside the output.path directory.[id] is replaced by the id of the chunk.
[name] is replaced by the name of the chunk (or with the id when the chunk has no name).
[hash] is replaced by the hash of the compilation.
[chunkhash] is replaced by the hash of the chunk.
module
options affecting the normal modules ( NormalModuleFactory )module.loaders
An array of automatically applied loaders.数组中每一项可以有下列这些属性:
+
test: 必须匹配的条件
+
exclude: 不能匹配的条件
+
include: 一个包含路径或者文件的数组,其中的文件会被这个 loader 所 transformed
+
loader: 利用“!”来分隔loader的一个字符串
+
loaders: 包含 loader 的数组。
A condition may be a RegExp (tested against absolute path), a string containing the absolute path, a function(absPath): bool, or an array of one of these combined with “and”.
IMPORTANT: The loaders here are resolved relative to the resource which they are applied to. This means they are not resolved relative to the configuration file. 如果你有
loaders是通过npm进行安装的,而且你的
node_modules文件夹并不是在所有源文件的父级,那么webpack可能会找不到相应的
loader,你需要将
node_modules文件夹的绝对路径添加到
resolveLoader.root选项中去。
resolveLoader: {root: path.join(__dirname, "node_modules")}
Example:
module: { loaders: [{ // "test" is commonly used to match the file extension test: /\.jsx$/, // "include" is commonly used to match the directories include: [ path.resolve(__dirname, "app/src"), path.resolve(__dirname, "app/test") ], // "exclude" should be used to exclude exceptions // try to prefer "include" when possible // the "loader" loader: "babel-loader" // or "babel" because webpack adds the "-loader" automatically }] }
module.preLoaders, module.postLoaders
就像module.loaders一样的语法。
一个包含 pre 和 post loaders 的数组。
module.noParse
一个正则表达式或者正则表达式数组,对于相匹配的文件不进行解析。It’s matched against the full resolved request.
可以改善忽略大的文件夹时的性能。
这些文件并没有必要使用
require、
define或其他类似的,但是可以使用
exports和
module.exports。
automatically created contexts defaults module.xxxContextXxx
有多种选项可以设置自动创建context的默认配置,这里我们区分了三种自动创建的方式+ exprContext:一个依赖表达式(i. e. require( expr ) )
+ wrappedContext:An expression plus pre- and/or suffixed string (i. e. require(“./templates/” + expr))
。。。。。后面的不太看得懂啊,不太明白这个选项是干啥的
Example:
{ module: { // Disabled handling of unknown requires unknownContextRegExp: /$^/, unknownContextCritical: false, // Disabled handling of requires with a single expression exprContextRegExp: /$^/, exprContextCritical: false, // Warn for every expression in require wrappedContextCritical: true } }
resolve
影响模块解析的选项resolve.alias
用其他的模块或路径来替换模块。一个带有索引的对象,key用来作为模块名,value是新的路径,就像是一次替换但是更聪明,如果key是以$符号结尾的,那么只有严格匹配的才会被替换。
如果 value 是一个相对路径,那么它会相对于包含 require( ) 的那个文件。
Example: 使用不同的alias设置来在
/abc/entry.js文件中调用模块。
index.js may resolve to another file if defined in the package.json.
/abc/node_modules may resolve in /node_modules too.
resolve.root
包含你的模块的文件夹(绝对路径),也可以是一个文件夹数组, This setting should be used to add individual directories to the search path.必须是绝对路径,不要使用 ./app/modules 这种
Example:
var path = require('path'); //... resolve: { root: [ path.resolve('./app/modules'), path.resolve('./vendor/modules') ] }
相关文章推荐
- webpack bable-loader升级无法编译jsx es6
- Webpack 实现 Node.js 代码热替换
- Webpack 实现 AngularJS 的延迟加载
- webpack中引用jquery的简单实现
- ReactJS学习笔记——npm、JSX、webpack
- webpack共用于前后端的小坑
- React 相关文档
- 在现有 server 中集成 webpack + react 热加载
- Electron+React+Webpack+Vscode应用桌面开发平台搭建
- webpack使用的一些看法
- webpack使用babel 6打包react报错
- ERROR-1:React
- ERROR-2:React
- ERROR-3:React
- webpack不是内部命令问题
- Webpack 学习笔记
- Webpack your bags(中文翻译)
- [译] Webpack 用来做模块热替换(hot module replacement)
- 让我们开始用es6来写代码--gulp构建es6
- 当React遇到了es6,让gulp来搭桥