C#6.0新特性
2016-06-21 09:24
417 查看
C#6.0新特性
C#发展历程以及C#6.0新特性
一、C#发展历程
下图是自己整理列出了C#每次重要更新的时间及增加的新特性,对于了解C#这些年的发展历程,对C#的认识更加全面,是有帮助的。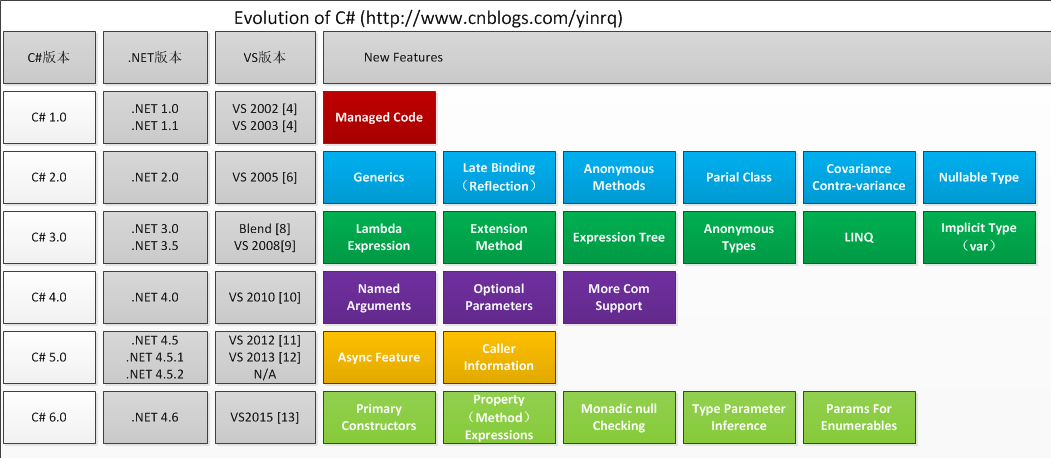
二、C#6.0新特性
1、字符串插值 (String Interpolation)字符串拼接优化
Before:
var Name = "joye.net"; var Results = "Hello" + Name;//直接拼接 var results1 = string.Format("Hello {0}", Name);//Format拼接
After:
var results2 = $"Hello {Name}"; //$拼接 var results= $"Hello {Name}{new Program().GetCnblogsSite()}";//{}可以直接插入代码
2、null检查运算符【 ?.】 (Monadic null checking)
null优化
Before:
public static string GetCnblogsSite() { return "http://www.cnblogs.com/yinrq"; }
Program pro = null; if(pro!=null) Console.WriteLine(GetCnblogsSite());
After:
Program pro = null; Console.WriteLine(pro?.GetCnblogsSite());
3、 自动属性初始化器(Initializers for auto-properties)
可以直接给自动属性赋值了,不需要写在构造函数中。
Before:
public class ClassA { private string Name{get;set;}; public ClassA() { Name = "joye.net"; } }
After:
public class ClassA { public string Name { get; set; } ="joye.net"; }
4、只读自动属性(Getter-only auto-properties)
只读自动属性可以直接初始化,或者在构造函数中初始化。
before
//缩小自动属性的访问权限 public class ClassA { public string Name { get; private set; } } //C#1.0实现 public class ClassA { private string Name = "joye.net"; public string Name { get { return Name; } } }
after:
public class ClassA { public string Name { get; } = "joye.net"; }
5、表达式方法体(Property Expressions && Method Expressions)
只读属性,只读索引器和方法都可以使用Lambda表达式作为Body。
一句话的方法体可以直接写成箭头函数,而不再需要大括号(分页控件http://www.cnblogs.com/yinrq/p/5586841.html就用到了属性表达式Property Expressions)
public class PagerInBase { /// <summary> /// 当前页 /// </summary> public int PageIndex { get; set; } /// <summary> /// 页数 /// </summary> public int PageSize { get; set; } //以前的写法 //public int Skip{get{return (PageIndex - 1) * PageSize}} //跳过序列中指定数量的元素 public int Skip => (PageIndex - 1) * PageSize; /// <summary> /// 请求URL /// </summary> public string RequetUrl => System.Web.HttpContext.Current.Request.Url.OriginalString; /// <summary> /// 构造函数给当前页和页数初始化 /// </summary> public PagerInBase() { if (PageIndex == 0) PageIndex = 1; if (PageSize == 0) PageSize = 10; } }
方法表达式(Method Expressions)
//before 的完整方法 public int Skip() { return (PageIndex - 1) * PageSize } //After C#6.0 方法表达式 public int Skip() => (PageIndex - 1) * PageSize;
6、using静态类(Static type using statements)
using System; using static System.Math; namespace ConsoleApplication1 { class Program { static void Main(string[] args) { Console.WriteLine(Log10(5) + PI); } } }
7、检查方法参数nameof表达式(nameof expressions)
这个很有用,原来写WPF中的ViewModel层的属性变化通知时,需要写字符串,或者使用MvvmLight等库中的帮助方法,可以直接传入属性,但由于是在运行时解析,会有少许性能损失。现在使用nameof运算符,保证重构安全和可读性,又提升了性能。
Before:
public static void Add(Person person) { if (person == null) { throw new ArgumentNullException("person"); } }
After:
public static void Add(Person person) { if (person == null) { throw new ArgumentNullException(nameof("person")); } }
8、带索引的对象初始化器(Index initializers )
直接通过索引进行对象的初始化
var dic = new Dictionary<int, string> { [0]="joye.net",[1]= "http://yinrq.cnblogs.com/",[2]= "Index initializers " };
9、catch和finally 中使用await (catch和finally 中的 await )
在C#5.0中,await关键字是不能出现在catch和finnaly块中的。而C#6.0可以
try { res = await Resource.OpenAsync(…); // You could do this } catch (ResourceException e) { await Resource.LogAsync(res, e); // Now you can do this } finally { if (res != null) await res.CloseAsync(); // finally and do this. }
10、内联out参数(Inline declarations for out params)
before
int x; int.TryParse("123", out x);
after:
int.TryParse("123", out int x);
11、无参数的结构体构造函数(Parameterless constructors in structs)
public struct MyStruct { public int A { get; } public int B { get; } public MyStruct(int a, int b) { A = a; B = b; } public MyStruct(): this(0, 1) { } }
WriteLine(new MyStruct().ToString()); WriteLine(default(MyStruct).ToString());
三、代码
using System; using System.Collections.Generic; using static System.Console; namespace ConsoleApplication1 { public class MyClass { public int A { get; set; } public int B { get; set; } = 1; public string Separator { get; } = "/"; public string SeparatorSpaces { get; } = string.Empty; public double Value => (double)A / B; public int this[int index] => index == 0 ? A : B; public int this[string index] => index == "A" ? A : B; public override string ToString() => "{A}{SeparatorSpaces}{Separator}{SeparatorSpaces}{B}"; public void Print() => WriteLine(ToString()); public MyClass() { } public MyClass(int a, int b) { A = a; B = b; } public MyClass(int a, int b, string separatorSpaces) : this(a, b) { SeparatorSpaces = separatorSpaces; if (string.IsNullOrEmpty(separatorSpaces)) { throw new ArgumentNullException(nameof(separatorSpaces)); } } public static readonly Dictionary<string, MyClass> Dic = new Dictionary<string, MyClass> { ["zero"] = new MyClass(), ["one"] = new MyClass(1, 1), ["half"] = new MyClass(1, 2), ["quarter"] = new MyClass(1, 4), ["infinity"] = new MyClass(1, 0), }; } public struct MyStruct { public int A { get; } public int B { get; } public MyStruct(int a, int b) { A = a; B = b; } public MyStruct(): this(0, 1) { } public override string ToString() => "{A}{B}"; } class Program { static void Main(string[] args) { foreach (var f in MyClass.Dic) { WriteLine("{f.Key} : {f.Value.Value}"); } var fraction = new MyClass(1, 3, " "); fraction.Print(); try { fraction = new MyClass(1, 2, null); } catch (ArgumentNullException e) { if (e.ParamName == "separatorSpaces") WriteLine("separatorSpaces can not be null"); } MyClass v; MyClass.Dic.TryGetValue("harf", out v); v?.Print(); var a = v?.A; WriteLine(a == null); var b = v?["B"]; WriteLine(b == null); WriteLine(v?.ToString() == null); WriteLine(new MyStruct().ToString()); WriteLine(default(MyStruct).ToString()); } } }
相关文章推荐
- C# note
- C#发展历程以及C#6.0新特性
- 如何通过C#.NET中的反射机制来创建C#.NET泛型类的实例,并调用其方法??
- .NET平台开源项目速览(16)C#写PDF文件类库PDF File Writer介绍
- C# 使用IENUMERABLE,YIELD
- 【C#】笔试知识点
- C#干掉自己
- C#和.NET版本
- c#上传下载ftp(支持断点续传)
- C# 简单的写入EXCEL操作
- 登山-C#-两个小知识点,字符串补全,在TextBox中捕获回车键
- C#部分类与部分方法
- 登山-C#-对密码进行加密存储
- 工大助手--C#之DataGrid
- C#写爬虫,版本V2.0
- C# II: Class ViewModelBase and RelayCommand in MVVM
- C#中事件的使用
- c# BarTender打印
- Office Word 是如何根据字体的 OS/2 表等信息计算「单倍行距」的
- c#虚拟打印机