java web 实现验证码
2016-06-20 22:12
381 查看
验证码的作用:通常的登录或者注册系统时,都会要求用户输入验证码,以此区别用户行为和计算机程序行为,目的是有人防止恶意注册、暴力破解密码等。
实现验证码的思路:用 server 实现随机生成数字和字母组成图片的功能,用 jsp 页面实现显示验证码和用户输入验证码的功能,再用 server 类分别获取图片和用户输入的数据,判断两个数据是否一致。
代码实现
1.编写数字、英文随机生成的 server 类,源码:
2.用于显示验证码的页面:
3.用于检验输入的验证码是否正确:
4.显示输入结构界面(输入验证码是否正确):
5.项目结构、效果截图:
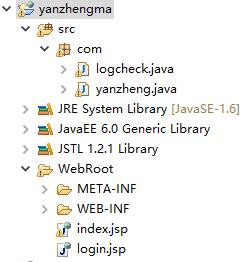
实现验证码的思路:用 server 实现随机生成数字和字母组成图片的功能,用 jsp 页面实现显示验证码和用户输入验证码的功能,再用 server 类分别获取图片和用户输入的数据,判断两个数据是否一致。
代码实现
1.编写数字、英文随机生成的 server 类,源码:
package com; import java.awt.Color; import java.awt.Font; import java.awt.Graphics; import java.awt.image.BufferedImage; import java.io.ByteArrayOutputStream; import java.io.IOException; import java.io.PrintWriter; import javax.imageio.ImageIO; import javax.servlet.ServletException; import javax.servlet.ServletOutputStream; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.http.HttpSession; public class logcheck extends HttpServlet { public logcheck() { super(); } public void destroy() { super.destroy(); } public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doPost(request, response); } /*实现的核心代码*/ public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("image/jpeg"); HttpSession session=request.getSession(); int width=60; int height=20; //设置浏览器不要缓存此图片 response.setHeader("Pragma", "No-cache"); response.setHeader("Cache-Control", "no-cache"); response.setDateHeader("Expires", 0); //创建内存图像并获得图形上下文 BufferedImage image=new BufferedImage(width, height,BufferedImage.TYPE_INT_RGB); Graphics g=image.getGraphics(); /* * 产生随机验证码 * 定义验证码的字符表 */ String chars="0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ"; char[] rands=new char[4]; for(int i=0;i<4;i++){ int rand=(int) (Math.random() *36); rands[i]=chars.charAt(rand); } /* * 产生图像 * 画背景 */ g.setColor(new Color(0xDCDCDC)); g.fillRect(0, 0, width, height); /* * 随机产生120个干扰点 */ for(int i=0;i<120;i++){ int x=(int)(Math.random()*width); int y=(int)(Math.random()*height); int red=(int)(Math.random()*255); int green=(int)(Math.random()*255); int blue=(int)(Math.random()*255); g.setColor(new Color(red,green,blue)); g.drawOval(x, y, 1, 0); } g.setColor(Color.BLACK); g.setFont(new Font(null, Font.ITALIC|Font.BOLD,18)); //在不同高度输出验证码的不同字符 g.drawString(""+rands[0], 1, 17); g.drawString(""+rands[1], 16, 15); g.drawString(""+rands[2], 31, 18); g.drawString(""+rands[3], 46, 16); g.dispose(); //将图像传到客户端 ServletOutputStream sos=response.getOutputStream(); ByteArrayOutputStream baos=new ByteArrayOutputStream(); ImageIO.write(image, "JPEG", baos); byte[] buffer=baos.toByteArray(); response.setContentLength(buffer.length); sos.write(buffer); baos.close(); sos.close(); session.setAttribute("checkcode", new String(rands)); } public void init() throws ServletException { // Put your code here } }
2.用于显示验证码的页面:
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>index</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <!-- <link rel="stylesheet" type="text/css" href="styles.css"> --> </head> <body> <form action="yanzheng" method="post"> <input type="text" name="name" size="5" maxlength="4"> <a href="index.jsp"><img border="0" src="logcheck"></a><br><br>      <input type="submit" value="提交"> </form> </body> </html>
3.用于检验输入的验证码是否正确:
package com; import java.io.IOException; import java.io.PrintWriter; import javax.jms.Session; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.http.HttpSession; public class yanzheng extends HttpServlet { public yanzheng() { super(); } public void destroy() { super.destroy(); } public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doPost(request, response); } /*核心代码*/ public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String info=null; /*获取输入的值*/ String value1=request.getParameter("name"); /*获取图片的值*/ HttpSession session=request.getSession(); String value2=(String)session.getAttribute("checkcode"); /*对比两个值(字母不区分大小写)*/ if(value2.equalsIgnoreCase(value1)){ info="验证码输入正确"; }else{ info="验证码输入错误"; } System.out.println(info); request.setAttribute("info", info); request.getRequestDispatcher("/login.jsp").forward(request, response); } public void init() throws ServletException { // Put your code here } }
4.显示输入结构界面(输入验证码是否正确):
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>My JSP 'login.jsp' starting page</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <!-- <link rel="stylesheet" type="text/css" href="styles.css"> --> </head> <body> <%=request.getAttribute("info") %> </body> </html>
5.项目结构、效果截图:
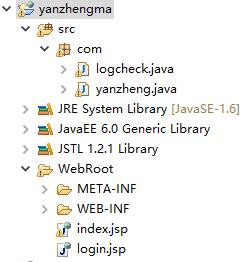

相关文章推荐
- Eclipse安装git插件以及关联导入GitHub项目
- JMeter学习-027-JMeter参数文件(脚本分发)路径问题:jmeter.threads.JMeterThread: Test failed! java.lang.IllegalArgumentException: File distributed.csv must exist and be readable解决方法
- Java的不可变类
- java 一只青蛙一次可以跳上1级台阶,也可以跳上2级。求该青蛙跳上一个n级的台阶总共有多少种跳法。
- Dubbo,Springmaven,Zookeeper整合
- java的面向对象思想
- java单例模式使用场景
- Java异常处理
- Java - PAT - 1042. 字符统计(20)
- Java Console 输出格式控制
- 约瑟夫问题的另类java实现
- java 大家都知道斐波那契数列,现在要求输入一个整数n,请你输出斐波那契数列的第n项。斐波那契数列的定义如下
- 《Java小游戏实现》:坦克大战(续一)
- 《Thinkinginjava》第6章-访问权限控制
- 关于SpringMVC使用@RequestBody注解接受json格式数据报415错误
- Java(线性代数--逆序数的求算)
- JDK环境变量的配置
- Ubuntu安装JDK
- Struts2输入校验field-validator type 可取得值
- Java进阶(三)多线程开发关键技术