Spring与Logback整合
2016-06-17 12:44
351 查看
最近项目中使用了logback作为日志处理,可是对这个不是很熟悉,所以就写些博客记录下学习的过程。本篇博客是这系列的第一篇。
因为我在logback中需要使用到发送Email,为了便于在本地Eclipse中方便测试,你需要安装一个Eclipse Email plugin,具体参考mailsnag。
logback的官方网站http://logback.qos.ch/manual/configuration.html 。
1. 在web.xml文件中配置spring与logback整合需要的listener.
2. 这里我们使用了自己的SpringMVCLogbackConfigListener, 而这个listener实际上继承了ch.qos.logback.ext.spring.web.LogbackConfigListener.
A property can be defined for insertion in local scope, in context scope, or in system scope. Local scope is the default. Although it is possible to read variables from the OS environment, it is not possible to write into the
OS environment.
LOCAL SCOPE A property with local scope exists from the point of its definition in a configuration file until the end of interpretation/execution of said configuration file. As a corollary, each time a configuration file is parsed
and executed, variables in local scope are defined anew.
CONTEXT SCOPE A property with context scope is inserted into the context and lasts as long as the context or until it is cleared. Once defined, a property in context scope is part of the context. As such, it is available in all
logging events, including those sent to remote hosts via serialization.
SYSTEM SCOPE A property with system scope is inserted into the JVM's system properties and lasts as long as the JVM or until it is cleared.
Properties are looked up in the the local scope first, in the context scope second, in the system properties scope third, and in the OS environment last.
During substitution, properties are looked up in the local scope first, in the context scope second, in the system properties scope third, and in the OS environment fourth and last.
The scope attribute of the <property> element, <define> element or the <insertFromJNDI> element can be used to set the scope of a property. The scope attribute admits "local", "context" and "system" strings as possible values. If not specified, the scope is
always assumed to be "local".
原文请参考:http://logback.qos.ch/manual/configuration.html
那么我的具体配置如下:
5. 启动服务,访问页面并且将Email Messages 插件启动:
5.1 主页如下:
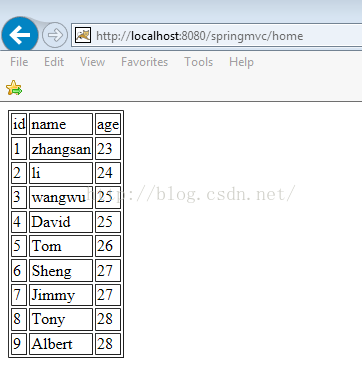
5.2 我们收到了邮件。

双击可以打开这个邮件。
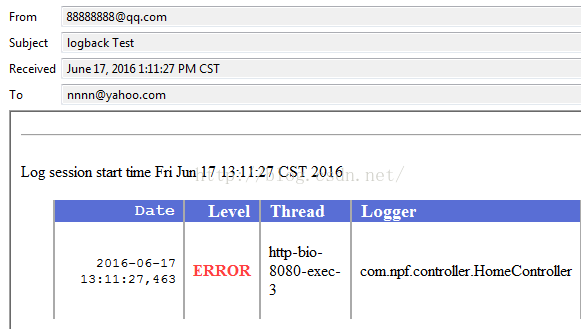
5.3 我们可以找到本地磁盘的log文件,并打开查看。
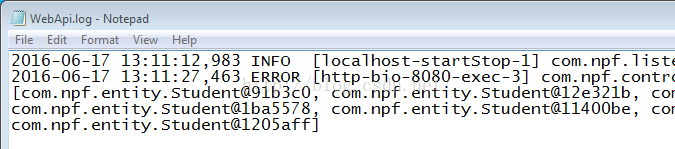
6. 这个博客用到的源代码已托管到GitHub, 如果你感兴趣,可以查看Spring与Logback整合
因为我在logback中需要使用到发送Email,为了便于在本地Eclipse中方便测试,你需要安装一个Eclipse Email plugin,具体参考mailsnag。
logback的官方网站http://logback.qos.ch/manual/configuration.html 。
1. 在web.xml文件中配置spring与logback整合需要的listener.
<context-param> <param-name>logbackConfigLocation</param-name> <param-value>classpath:log/logback.xml</param-value> </context-param> <listener> <listener-class>com.npf.listener.SpringMVCLogbackConfigListener</listener-class> </listener>
2. 这里我们使用了自己的SpringMVCLogbackConfigListener, 而这个listener实际上继承了ch.qos.logback.ext.spring.web.LogbackConfigListener.
package com.npf.listener; import javax.servlet.ServletContextEvent; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.slf4j.impl.StaticLoggerBinder; import ch.qos.logback.classic.LoggerContext; import ch.qos.logback.ext.spring.web.LogbackConfigListener; public class SpringMVCLogbackConfigListener extends LogbackConfigListener{ private final static Logger LOGGER = LoggerFactory.getLogger(SpringMVCLogbackConfigListener.class); @Override public void contextInitialized(ServletContextEvent paramServletContextEvent) { super.contextInitialized(paramServletContextEvent); LoggerContext localLoggerContext = (LoggerContext)StaticLoggerBinder.getSingleton().getLoggerFactory(); String smtpHost = localLoggerContext.getProperty("smtpHost"); LOGGER.info("this smtpHost is {}",smtpHost); } }3. 配置logback.xml, 这里有一个小坑。在logback.xml有这么一个配置:
<property scope="context" resource="config/ENVspecifi.properties" />我当初scope="context"没有写,就总是会发现Spring不能把properties属性注入到logback中,非常郁闷。后来查阅了官方文档才知道:
A property can be defined for insertion in local scope, in context scope, or in system scope. Local scope is the default. Although it is possible to read variables from the OS environment, it is not possible to write into the
OS environment.
LOCAL SCOPE A property with local scope exists from the point of its definition in a configuration file until the end of interpretation/execution of said configuration file. As a corollary, each time a configuration file is parsed
and executed, variables in local scope are defined anew.
CONTEXT SCOPE A property with context scope is inserted into the context and lasts as long as the context or until it is cleared. Once defined, a property in context scope is part of the context. As such, it is available in all
logging events, including those sent to remote hosts via serialization.
SYSTEM SCOPE A property with system scope is inserted into the JVM's system properties and lasts as long as the JVM or until it is cleared.
Properties are looked up in the the local scope first, in the context scope second, in the system properties scope third, and in the OS environment last.
During substitution, properties are looked up in the local scope first, in the context scope second, in the system properties scope third, and in the OS environment fourth and last.
The scope attribute of the <property> element, <define> element or the <insertFromJNDI> element can be used to set the scope of a property. The scope attribute admits "local", "context" and "system" strings as possible values. If not specified, the scope is
always assumed to be "local".
原文请参考:http://logback.qos.ch/manual/configuration.html
那么我的具体配置如下:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE logback> <configuration debug="false" scan="false" scanPeriod="3 seconds"> <property scope="context" resource="config/ENVspecifi.properties" /> <appender name="consoleAppender" class="ch.qos.logback.core.ConsoleAppender"> <encoder> <pattern>%date %-5level [%thread] %logger - %m%n</pattern> </encoder> </appender> <appender name="rollingFileAppender" class="ch.qos.logback.core.rolling.RollingFileAppender"> <file>${log.dir}/WebApi.log</file> <rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy"> <fileNamePattern>${log.dir}/WebApi-%d{yyyy-MM-dd}.%i.log </fileNamePattern> <timeBasedFileNamingAndTriggeringPolicy class="ch.qos.logback.core.rolling.SizeAndTimeBasedFNATP"> <maxFileSize>1MB</maxFileSize> </timeBasedFileNamingAndTriggeringPolicy> </rollingPolicy> <encoder> <pattern>%date %-5level [%thread] %logger - %m%n</pattern> </encoder> </appender> <appender name="accessRollingFileAppender" class="ch.qos.logback.core.rolling.RollingFileAppender"> <file>${log.dir}/access.log</file> <rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy"> <fileNamePattern>${log.dir}/access-%d{yyyy-MM-dd}.log</fileNamePattern> <maxHistory>30</maxHistory> </rollingPolicy> <encoder> <pattern>%date - %m%n</pattern> </encoder> <append>false</append> </appender> <appender name="smtpAppender" class="ch.qos.logback.classic.net.SMTPAppender"> <filter class="ch.qos.logback.classic.filter.LevelFilter"> <level>error</level> <onMatch>ACCEPT</onMatch> <onMismatch>DENY</onMismatch> </filter> <smtpHost>${smtpHost}</smtpHost> <smtpPort>${smtpPort}</smtpPort> <to>${to}</to> <from>${from}</from> <subject>logback Test</subject> <layout class="ch.qos.logback.classic.html.HTMLLayout"> <pattern>%date%level%thread%logger%message</pattern> </layout> </appender> <logger name="com" level="debug"> <appender-ref ref="rollingFileAppender" /> <appender-ref ref="accessRollingFileAppender" /> <appender-ref ref="smtpAppender" /> </logger> <root level="error"> <appender-ref ref="consoleAppender" /> </root> </configuration>4.我的controller如下,因为我需要测试邮件,所以将logger设置为了error.
package com.npf.controller; import java.util.Map; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import com.npf.service.StudentService; @Controller public class HomeController { private final static Logger LOGGER = LoggerFactory.getLogger(HomeController.class); @Autowired @Qualifier("studentServiceImpl") private StudentService studentService; @RequestMapping(value={"/","/home"}) public String showHomePage(Map<String,Object> map){ map.put("stuList", studentService.getStudents()); LOGGER.error("this student list is {}", studentService.getStudents()); return "home"; } }
5. 启动服务,访问页面并且将Email Messages 插件启动:
5.1 主页如下:
5.2 我们收到了邮件。
双击可以打开这个邮件。
5.3 我们可以找到本地磁盘的log文件,并打开查看。
6. 这个博客用到的源代码已托管到GitHub, 如果你感兴趣,可以查看Spring与Logback整合
相关文章推荐
- but no declaration can be found for element 'mvc:resources'(springmvc 常见错误)
- java的引用总结
- java前后台之间传值的几种方式
- 基于Eclipse Maven的Spring4/Spring-MVC/Hibernate4整合之五:Hibernate的事务管理、手动回滚
- 深入springmvc
- java构造树,多级菜单
- spring mvc数据绑定
- JAVA JDK1.5-1.9新特性
- spring注解 总结
- Spring 攻略第004讲
- springMVC源码下载地址
- Java封装、继承、多态三大特征的理解
- Java检查型异常和非检查型异常
- SpringMVC访问静态资源的三种方式
- java检查异常与非检查异常
- Java Calendar 类的格式操作
- git diff java 实现
- java的HashMap与ConcurrentHashMap
- Eclipse中SVN过滤指定文件夹或文件下内容
- apktool编译和反编译apk与ecplise多渠道打包