[Android]使用BeanShell实现Android简易科学计算器
2016-06-12 23:21
357 查看
在Android实习中,为了实现一个科学计算器,需要自己实现计算类,偶然发现了BeanShell,其中的一个功能就是可以对一串数学表达式进行计算,所以使用该第三方jar包实现了简易的Android计算器。 Beanshell
(bsh) 是用Java写成的,一个小型的、免费的、可以下载的、嵌入式的Java源代码解释器,具有对象脚本语言特性。BeanShell执行 标准Java语句和表达式,另外包括一些脚本命令和语法。它将脚本化对象看作简单闭包方法(simple method closure)来支持,就如同在Perl和JavaScript中的一样。当然BeanShell能做的事还有很多,再次不再赘述。
这是jar包的下载地址:http://www.beanshell.org 或 http://download.csdn.net/detail/lqefn/204352
下面是实现过程,首先我们需要导入该jar包,本次使用IDE为Android Studio,首先创建工程之后,选择菜单栏的File,点击Project Structure。
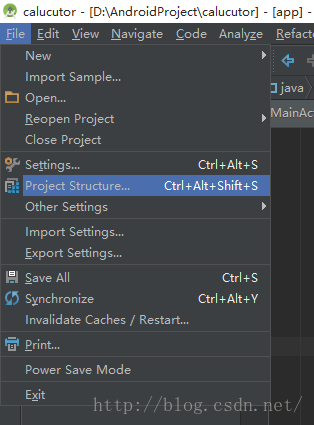
然后选中Dependencies,点击右侧加号,选择File dependency,然后选择下载好的jar包的路径。

下面是代码实现过程:首先是UI的xml代码,效果如下图
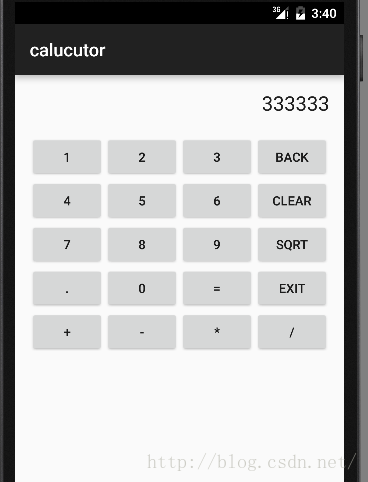
下面是MainActivity.java的代码。
以上就是计算器的全部实现过程,只是实现了大体功能,有很多操作BUG没有完全处理,也参考了很多前辈的资料,本人才疏学浅,难免有错误,希望大家评判指正。
(bsh) 是用Java写成的,一个小型的、免费的、可以下载的、嵌入式的Java源代码解释器,具有对象脚本语言特性。BeanShell执行 标准Java语句和表达式,另外包括一些脚本命令和语法。它将脚本化对象看作简单闭包方法(simple method closure)来支持,就如同在Perl和JavaScript中的一样。当然BeanShell能做的事还有很多,再次不再赘述。
这是jar包的下载地址:http://www.beanshell.org 或 http://download.csdn.net/detail/lqefn/204352
下面是实现过程,首先我们需要导入该jar包,本次使用IDE为Android Studio,首先创建工程之后,选择菜单栏的File,点击Project Structure。
然后选中Dependencies,点击右侧加号,选择File dependency,然后选择下载好的jar包的路径。
下面就可以在java中使用import bsh.Interpreter; 导入我们需要的包
使用BeanShell我们主要使用下面两行代码
Interpreter bsh = new Interpreter(); //声明Interpreter类 Number result = (Number)bsh.eval(exp); //将exp要计算的表达式传入,并用result接收结果
下面是代码实现过程:首先是UI的xml代码,效果如下图
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity" android:orientation="vertical" android:weightSum="1" android:id="@+id/linear1"> <TextView android:layout_width="fill_parent" android:layout_height="50dp" android:text="New Text" android:gravity="right" android:textAppearance="?android:attr/textAppearanceLarge" android:id="@+id/textView" /> <TableLayout android:layout_width="fill_parent" android:layout_height="wrap_content"> <TableRow android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:layout_width="wrap_content" android:layout_weight="1" android:layout_height="wrap_content" android:text="1" android:id="@+id/number1" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="2" android:layout_weight="1" android:id="@+id/number2" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="3" android:layout_weight="1" android:id="@+id/number3" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="back" android:layout_weight="1" android:id="@+id/back" /> </TableRow> <TableRow android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="4" android:layout_weight="1" android:id="@+id/number4" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="5" android:id="@+id/number5" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="6" android:id="@+id/number6" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="clear" android:id="@+id/clear" /> </TableRow> <TableRow android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="7" android:layout_weight="1" android:id="@+id/number7" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="8" android:id="@+id/number8" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="9" android:id="@+id/number9" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="sqrt" android:id="@+id/sqrt" /> </TableRow> <TableRow android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="." android:layout_weight="1" android:id="@+id/point" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="0" android:id="@+id/number0" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="=" android:id="@+id/result" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" acf5 android:text="exit" android:id="@+id/exit" /> </TableRow> <TableRow android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="+" android:layout_weight="1" android:id="@+id/add" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="-" android:id="@+id/reduce" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="*" android:id="@+id/multiply" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="/" android:id="@+id/divide" /> </TableRow> </TableLayout> </LinearLayout>
下面是MainActivity.java的代码。
import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; import java.util.Arrays; import bsh.EvalError; import bsh.Interpreter; public class MainActivity extends AppCompatActivity implements View.OnClickListener{ private Button number0,number1,number2,number3,number4,number5,number6,number7,number8, number9,add,reduce,divide,multiply,point,clear,back,exit,sqrt,result; private TextView textView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); init(); } public void init(){
//初始化函数,设置按钮监听 number0= (Button) findViewById(R.id.number0); number1= (Button) findViewById(R.id.number1); number2= (Button) findViewById(R.id.number2); number3= (Button) findViewById(R.id.number3); number4= (Button) findViewById(R.id.number4); number5= (Button) findViewById(R.id.number5); number6= (Button) findViewById(R.id.number6); number7= (Button) findViewById(R.id.number7); number8= (Button) findViewById(R.id.number8); number9= (Button) findViewById(R.id.number9); result= (Button) findViewById(R.id.result); point= (Button) findViewById(R.id.point); add= (Button) findViewById(R.id.add); reduce= (Button) findViewById(R.id.reduce); back= (Button) findViewById(R.id.back); clear= (Button) findViewById(R.id.clear); divide= (Button) findViewById(R.id.divide); exit= (Button) findViewById(R.id.exit); sqrt= (Button) findViewById(R.id.sqrt); multiply= (Button) findViewById(R.id.multiply); textView= (TextView) findViewById(R.id.textView); textView.setText(""); result.setOnClickListener(this); number0.setOnClickListener(this); number1.setOnClickListener(this); number2.setOnClickListener(this); number3.setOnClickListener(this); number4.setOnClickListener(this); number5.setOnClickListener(this); number6.setOnClickListener(this); number7.setOnClickListener(this); number8.setOnClickListener(this); number9.setOnClickListener(this); back.setOnClickListener(this); clear.setOnClickListener(this); exit.setOnClickListener(this); sqrt.setOnClickListener(this); multiply.setOnClickListener(this); add.setOnClickListener(this); reduce.setOnClickListener(this); point.setOnClickListener(this); divide.setOnClickListener(this); }
//计算,传入需要计算的字符串,返回结果字符串 private String getResult(String exp){ Interpreter bsh = new Interpreter(); Number result = null; try { exp = filterExp(exp); result = (Number)bsh.eval(exp); } catch (EvalError e) { e.printStackTrace(); return "算数公式错误"; } exp = result.doubleValue()+""; if(exp.endsWith(".0")) exp = exp.substring(0, exp.indexOf(".0")); return exp; }
//<span style="color: rgb(128, 128, 128); font-family: Consolas, 'Bitstream Vera Sans Mono', 'Courier New', Courier, monospace; font-size: 13.3333px; line-height: 14.6667px; white-space: pre;">因为计算过程中,全程需要有小数参与,所以需要过滤一下</span> private String filterExp(String exp) { String num[] = exp.split(""); String temp = null; int begin=0,end=0; for (int i = 1; i < num.length; i++) { temp = num[i]; if(temp.matches("[+-/()*]")){ if(temp.equals(".")) continue; end = i - 1; temp = exp.substring(begin, end); if(temp.trim().length() > 0 && temp.indexOf(".")<0) num[i-1] = num[i-1]+".0"; begin = end + 1; } } return Arrays.toString(num).replaceAll("[\\[\\], ]", ""); } @Override public void onClick(View v) { switch (v.getId()){ case R.id.number0: textView.append("0"); break; case R.id.number1: textView.append("1"); break; case R.id.number2: textView.append("2"); break; case R.id.number3: textView.append("3"); break; case R.id.number4: textView.append("4"); break; case R.id.number5: textView.append("5"); break; case R.id.number6: textView.append("6"); break; case R.id.number7: textView.append("7"); break; case R.id.number8: textView.append("8"); break; case R.id.number9: textView.append("9"); break; case R.id.exit: finish(); break; case R.id.add: textView.append("+"); break; case R.id.reduce: textView.append("-"); break; case R.id.back: String s = textView.getText().toString(); if(s.length()>0) { textView.setText("" + s.substring(0, s.length() - 1)); } break; case R.id.point: textView.append("."); break; case R.id.clear: textView.setText(""); break; case R.id.sqrt: if(textView.getText().toString().length()>0) { double num = Double.valueOf(textView.getText().toString()); num = Math.sqrt(num); textView.setText("" + num); } break; case R.id.multiply: textView.append("*"); break; case R.id.divide: textView.append("/"); break; case R.id.result: String result=getResult(textView.getText().toString()); textView.setText(result); break; } } }
以上就是计算器的全部实现过程,只是实现了大体功能,有很多操作BUG没有完全处理,也参考了很多前辈的资料,本人才疏学浅,难免有错误,希望大家评判指正。
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件
- SourceProvider.getJniDirectories