gPRC 小试牛刀
2016-06-05 19:00
489 查看
gRPC :A high performance, open source, general RPC framework that puts mobile and HTTP/2 first.
Building on the HTTP/2 standard brings many capabilities such as bidirectional streaming, flow control, header compression, multiplexing requests over a single TCP connection and more.
These features save battery life and data usage on mobile devices while speeding up services and web applications running in the cloud.
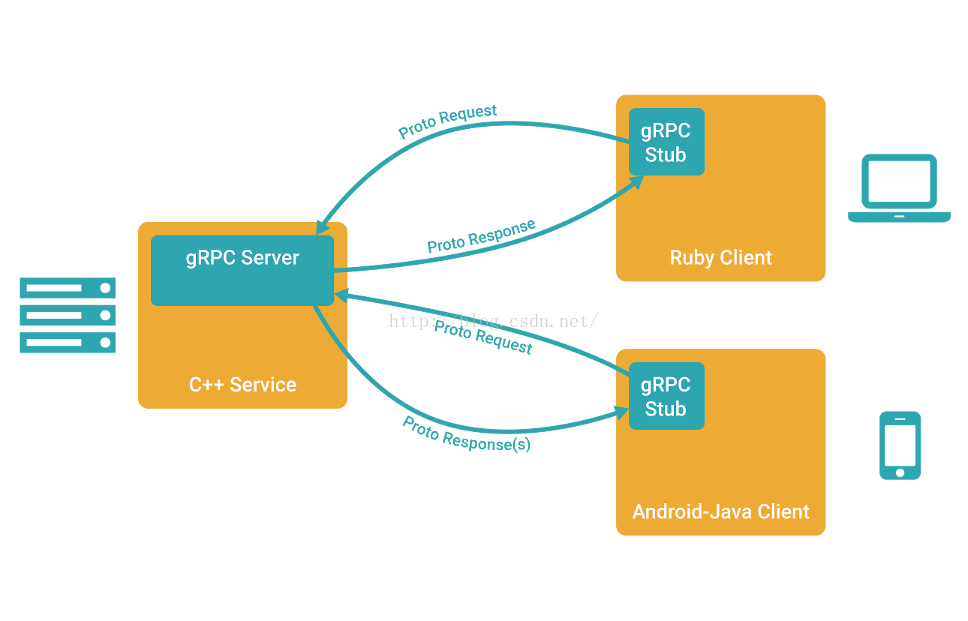
In gRPC a client application
can directly call methods on a server application
on a different machine as if it was a local object, making it easier for you to create distributed applications and services. As in many RPC systems, gRPC is based around the idea of defining aservice,
specifying the methods that can be called remotely with their parameters and return types. On the server side, the server implements this interface and runs a gRPC server to handle client calls. On the client side, the client has a stub that
provides exactly the same methods as the server.
gRPC clients and servers can run and talk to each other in a variety of environments - from servers inside Google to your
own desktop - and can be written in any of gRPC's supported languages.
在gRPC中,客户端应用程序可以像本地方法调用一样调用在不同物理机器上的服务端应用程序。这样很容易创建分布式应用程序和服务。
与许多RPC系统一样,gRPC基于定义服务,指定方法,参数以及返回值。在服务器端,服务实现定义的接口,运行gRPC服务来处理客户端调用。在客户端,客户端游一个stub直接提供同样的方法。
By
default gRPC uses protocol buffers,
Google’s mature open source mechanism for serializing structured data (although it can be used with other data formats such as JSON).
默认情况下,gRPC使用protocal
buffers 消息协议来序列化和反序列化数据。 同时也可以使用JSON数据格式。
Google has been using a single general-purpose RPC infrastructure called Stubby to connect the large number of microservices running within and across our data centers for over a decade. Our internal systems have long embraced the microservice architecture
gaining popularity today. Having a uniform, cross-platform RPC infrastructure has allowed for the rollout of fleet-wide improvements in efficiency, security, reliability and behavioral analysis critical to supporting the incredible growth seen in that period.
Stubby has many great features - however, it's not based on any standard and is too tightly coupled to our internal infrastructure to be considered suitable for public release. With the advent of SPDY, HTTP/2, and QUIC, many of these same features have appeared
in public standards, together with other features that Stubby does not provide. It became clear that it was time to rework Stubby to take advantage of this standardization, and to extend its applicability to mobile, IoT, and Cloud use-cases.
http://www.grpc.io/docs/ https://github.com/grpc/grpc-java https://developers.googleblog.com/2015/02/introducing-grpc-new-open-source-http2.html
创建一个maven项目,使用gRPC-java实现。添加依赖。
定义服务helloworld.proto
文件:
然后通过maven插件利用protoc-3.0.0-beta-2-windows-x86_64.exe
和 protoc-gen-grpc-java-0.14.0-windows-x86_64.exe 来生成代码。
在pom.xml中新增插件:
运行maven install 会在target/generated-sources 文件夹下面生成代码。
gRPC端服务器代码如下:HelloWorldServer.java
gRPC客户端代码:HelloWorldClient.java
Building on the HTTP/2 standard brings many capabilities such as bidirectional streaming, flow control, header compression, multiplexing requests over a single TCP connection and more.
These features save battery life and data usage on mobile devices while speeding up services and web applications running in the cloud.
In gRPC a client application
can directly call methods on a server application
on a different machine as if it was a local object, making it easier for you to create distributed applications and services. As in many RPC systems, gRPC is based around the idea of defining aservice,
specifying the methods that can be called remotely with their parameters and return types. On the server side, the server implements this interface and runs a gRPC server to handle client calls. On the client side, the client has a stub that
provides exactly the same methods as the server.
gRPC clients and servers can run and talk to each other in a variety of environments - from servers inside Google to your
own desktop - and can be written in any of gRPC's supported languages.
在gRPC中,客户端应用程序可以像本地方法调用一样调用在不同物理机器上的服务端应用程序。这样很容易创建分布式应用程序和服务。
与许多RPC系统一样,gRPC基于定义服务,指定方法,参数以及返回值。在服务器端,服务实现定义的接口,运行gRPC服务来处理客户端调用。在客户端,客户端游一个stub直接提供同样的方法。
By
default gRPC uses protocol buffers,
Google’s mature open source mechanism for serializing structured data (although it can be used with other data formats such as JSON).
默认情况下,gRPC使用protocal
buffers 消息协议来序列化和反序列化数据。 同时也可以使用JSON数据格式。
Motivation
Google has been using a single general-purpose RPC infrastructure called Stubby to connect the large number of microservices running within and across our data centers for over a decade. Our internal systems have long embraced the microservice architecturegaining popularity today. Having a uniform, cross-platform RPC infrastructure has allowed for the rollout of fleet-wide improvements in efficiency, security, reliability and behavioral analysis critical to supporting the incredible growth seen in that period.
Stubby has many great features - however, it's not based on any standard and is too tightly coupled to our internal infrastructure to be considered suitable for public release. With the advent of SPDY, HTTP/2, and QUIC, many of these same features have appeared
in public standards, together with other features that Stubby does not provide. It became clear that it was time to rework Stubby to take advantage of this standardization, and to extend its applicability to mobile, IoT, and Cloud use-cases.
http://www.grpc.io/docs/ https://github.com/grpc/grpc-java https://developers.googleblog.com/2015/02/introducing-grpc-new-open-source-http2.html
创建一个maven项目,使用gRPC-java实现。添加依赖。
<dependency> <groupId>io.grpc</groupId> <artifactId>grpc-all</artifactId> <version>0.14.0</version> </dependency>在依赖中可以发现,gRPC-java依赖netty-codec-http2 ,也就是使用netty作为网络层,使用netty中的http2作为协议层。
定义服务helloworld.proto
文件:
syntax = "proto3"; option java_multiple_files = true; option java_package = "io.grpc.examples.helloworld"; option java_outer_classname = "HelloWorldProto"; option objc_class_prefix = "HLW"; package helloworld; // The greeting service definition. service Greeter { // Sends a greeting rpc SayHello (HelloRequest) returns (HelloReply) {} } // The request message containing the user's name. message HelloRequest { string name = 1; } // The response message containing the greetings message HelloReply { string message = 1; }在maven项目中,新增src/main/proto文件夹,并且把helloworld.proto文件放在该文件夹下面。
然后通过maven插件利用protoc-3.0.0-beta-2-windows-x86_64.exe
和 protoc-gen-grpc-java-0.14.0-windows-x86_64.exe 来生成代码。
在pom.xml中新增插件:
<build> <extensions> <extension> <groupId>kr.motd.maven</groupId> <artifactId>os-maven-plugin</artifactId> <version>1.4.1.Final</version> </extension> </extensions> <plugins> <plugin> <groupId>org.xolstice.maven.plugins</groupId> <artifactId>protobuf-maven-plugin</artifactId> <version>0.5.0</version> <configuration> <!-- The version of protoc must match protobuf-java. If you don't depend on protobuf-java directly, you will be transitively depending on the protobuf-java version that grpc depends on. --> <protocArtifact>com.google.protobuf:protoc:3.0.0-beta-2:exe:${os.detected.classifier}</protocArtifact> <pluginId>grpc-java</pluginId> <pluginArtifact>io.grpc:protoc-gen-grpc-java:0.14.0:exe:${os.detected.classifier}</pluginArtifact> </configuration> <executions> <execution> <goals> <goal>compile</goal> <goal>compile-custom</goal> </goals> </execution> </executions> </plugin> </plugins> </build>
运行maven install 会在target/generated-sources 文件夹下面生成代码。
gRPC端服务器代码如下:HelloWorldServer.java
/** * Server that manages startup/shutdown of a {@code Greeter} server. */ public class HelloWorldServer { private static final Logger logger = Logger.getLogger(HelloWorldServer.class.getName()); /* The port on which the server should run */ private int port = 50051; private Server server; private void start() throws IOException { server = ServerBuilder.forPort(port) .addService(new GreeterImpl()) .build() .start(); logger.info("Server started, listening on " + port); Runtime.getRuntime().addShutdownHook(new Thread() { @Override public void run() { // Use stderr here since the logger may have been reset by its JVM shutdown hook. System.err.println("*** shutting down gRPC server since JVM is shutting down"); HelloWorldServer.this.stop(); System.err.println("*** server shut down"); } }); } private void stop() { if (server != null) { server.shutdown(); } } /** * Await termination on the main thread since the grpc library uses daemon threads. */ private void blockUntilShutdown() throws InterruptedException { if (server != null) { server.awaitTermination(); } } /** * Main launches the server from the command line. */ public static void main(String[] args) throws IOException, InterruptedException { final HelloWorldServer server = new HelloWorldServer(); server.start(); server.blockUntilShutdown(); } private class GreeterImpl extends GreeterGrpc.AbstractGreeter { @Override public void sayHello(HelloRequest req, StreamObserver<HelloReply> responseObserver) { System.out.println("======sayHello========"); HelloReply reply = HelloReply.newBuilder().setMessage("Hello " + req.getName()).build(); responseObserver.onNext(reply); responseObserver.onCompleted(); } } }
gRPC客户端代码:HelloWorldClient.java
/** * A simple client that requests a greeting from the {@link HelloWorldServer}. */ public class HelloWorldClient { private static final Logger logger = Logger.getLogger(HelloWorldClient.class.getName()); private final ManagedChannel channel; private final GreeterGrpc.GreeterBlockingStub blockingStub; /** Construct client connecting to HelloWorld server at {@code host:port}. */ public HelloWorldClient(String host, int port) { channel = ManagedChannelBuilder.forAddress(host, port) .usePlaintext(true) .build(); blockingStub = GreeterGrpc.newBlockingStub(channel); } public void shutdown() throws InterruptedException { channel.shutdown().awaitTermination(5, TimeUnit.SECONDS); } /** Say hello to server. */ public void greet(String name) { logger.info("Will try to greet " + name + " ..."); HelloRequest request = HelloRequest.newBuilder().setName(name).build(); HelloReply response; try { response = blockingStub.sayHello(request); } catch (StatusRuntimeException e) { logger.log(Level.WARNING, "RPC failed: {0}", e.getStatus()); return; } logger.info("Greeting: " + response.getMessage()); } /** * Greet server. If provided, the first element of {@code args} is the name to use in the * greeting. */ public static void main(String[] args) throws Exception { HelloWorldClient client = new HelloWorldClient("localhost", 50051); try { /* Access a service running on the local machine on port 50051 */ String user = "world"; if (args.length > 0) { user = args[0]; /* Use the arg as the name to greet if provided */ } client.greet(user); } finally { client.shutdown(); } } }
相关文章推荐
- java对世界各个时区(TimeZone)的通用转换处理方法(转载)
- java-注解annotation
- java-模拟tomcat服务器
- java-用HttpURLConnection发送Http请求.
- java-WEB中的监听器Lisener
- Android IPC进程间通讯机制
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- RPC failed; result=22, HTTP code = 411
- 介绍一款信息管理系统的开源框架---jeecg
- 聚类算法之kmeans算法java版本
- java实现 PageRank算法
- PropertyChangeListener简单理解
- c++11 + SDL2 + ffmpeg +OpenAL + java = Android播放器
- 插入排序
- 冒泡排序
- 堆排序
- 快速排序