C++实现稀疏矩阵的压缩存储、转置、快速转置
2016-05-21 14:54
706 查看
/*稀疏矩阵的压缩存储、转置、快速转置*/ #include <iostream> using namespace std; #include <vector> //三元组 template<class T> struct Triple { size_t _row; size_t _col; T _value; Triple(size_t row = 0, size_t col = 0, const T& value = T()) :_row(row) ,_col(col) ,_value(value) {} }; template<class T> class SparseMatrix { public: SparseMatrix(T* a = NULL, size_t M = 0, size_t N = 0, const T& invalid = T()) :_rowSize(M) ,_colSize(N) ,_invalid(invalid) { for (size_t i = 0; i < M; ++i) { for (size_t j = 0; j < N; ++j) { if (a[i*N+j] != _invalid) { Triple<T> t; t._row = i; t._col = j; t._value = a[i*N+j]; _a.push_back(t); } } } } void Display() { size_t index = 0; for (size_t i = 0; i < _rowSize; ++i) { for (size_t j = 0; j < _colSize; ++j) { if (index < _a.size() && (_a[index]._row == i) && (_a[index]._col == j)) { cout<<_a[index++]._value<<" "; } else { cout<<_invalid<<" "; } } cout<<endl; } } //矩阵转置 时间复杂度为 O(有效数据的个数*原矩阵的列数) SparseMatrix<T> Transport() { SparseMatrix<T> sm; sm._colSize = _rowSize; sm._rowSize = _colSize; sm._invalid = _invalid; for (size_t i = 0; i < _colSize; ++i) { size_t index = 0; while (index < _a.size()) { if (_a[index]._col == i) { Triple<T> t; t._row = _a[index]._col; t._col = _a[index]._row; t._value = _a[index]._value; sm._a.push_back(t); } ++index; } } return sm; } //快速转置 时间复杂度为O(有效数据的个数+原矩阵的列数) SparseMatrix<T> FastTransport() { SparseMatrix<T> sm; sm._rowSize = _colSize; sm._colSize = _rowSize; sm._invalid = _invalid; int* RowCounts = new int[_colSize]; int* RowStart = new int [_colSize]; memset(RowCounts, 0, sizeof(int)*_colSize); memset(RowStart, 0, sizeof(int)*_colSize); size_t index = 0; while (index < _a.size()) { ++RowCounts[_a[index]._col]; ++index; } for (size_t i = 1; i < _colSize; ++i) { RowStart[i] = RowStart[i-1] + RowCounts[i-1]; } index = 0; sm._a.resize(_a.size()); while (index < sm._a.size()) { Triple<T> t; t._row = _a[index]._col; t._col = _a[index]._row; t._value = _a[index]._value; sm._a[RowStart[_a[index]._col]] = t; ++RowStart[_a[index]._col]; ++index; } delete[] RowCounts; delete[] RowStart; return sm; } protected: vector<Triple<T>> _a; size_t _rowSize; size_t _colSize; T _invalid; }; void Test() { int array [6][5] = { {1, 0, 3, 0, 5}, {0, 0, 0, 0, 0}, {0, 0, 0, 0, 0}, {2, 0, 4, 0, 6}, {0, 0, 0, 0, 0}, {0, 0, 0, 0, 0} }; SparseMatrix<int> sm1((int*)array, 6, 5, 0); sm1.Display(); cout<<endl; //SprseMatrix<int> sm2 = sm1.Transport(); SparseMatrix<int> sm2 = sm1.FastTransport(); sm2.Display(); } int main() { Test(); return 0; }
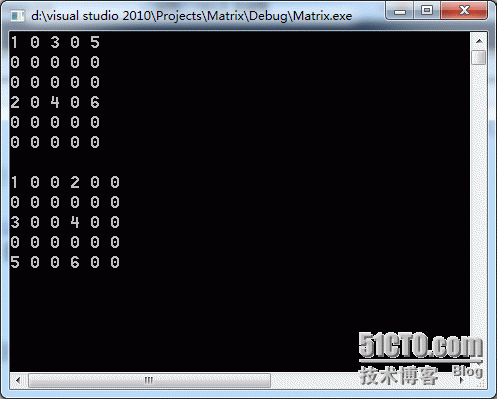
本文出自 “zgw285763054” 博客,请务必保留此出处http://zgw285763054.blog.51cto.com/11591804/1775680
相关文章推荐
- C++实现对称矩阵的压缩存储
- C++第六次作业
- C++实验6—数组合并
- c++中成员初始化列表的问题
- 第六次C++上机作业
- 为什么使用指针比使用对象本身更好?
- C语言动态分配内存
- C语言回调函数 2
- 求最短路径———Dijkstra算法和Floyd算法
- C语言结构体占用空间内存大小解析
- C语言知识体系框架
- 第十三周项目 4 立体类族共有的抽象类
- 第十三周项目 3 形状类族的中的纯虚函数
- 【C语言】用mktime函数获取一个日期是星期几
- 二叉树的深度优先遍历与广度优先遍历 [ C++ 实现 ]
- c++11标准——泛型算法
- IOS中的Block在C++中的运用
- C++随笔:.NET CoreCLR之corleCLR核心探索之coreconsole(2)
- 第十三周项目 动物这样叫 2.3
- 第十三周项目 动物这样叫2.2