c++;每周一些题(3)
2016-05-14 17:51
489 查看

class Solution { public: ListNode* FindKthToTail(ListNode* pListHead, unsigned int k) { if(pListHead==NULL||k==0){ return NULL; } ListNode* fast=pListHead; ListNode* slow=pListHead; while(fast){ fast=fast->next; if(k==0){ slow=slow->next; } else --k; } if(k==0){ return slow; } return NULL; } };

class Solution { public: ListNode* ReverseList(ListNode* pHead) { if(pHead==NULL) return NULL; ListNode* newHead=pHead; ListNode* tmp=pHead->next; newHead->next=NULL; while(tmp){ ListNode* next=tmp->next; tmp->next=newHead; newHead=tmp; tmp=next; } return newHead; } };
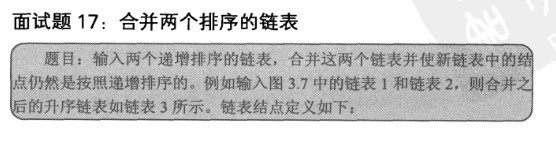
class Solution { public: ListNode* Merge(ListNode* pHead1, ListNode* pHead2) { if(pHead1==NULL) return pHead2; if(pHead2==NULL) return pHead1; if(pHead1==NULL&&pHead2==NULL) return NULL; ListNode* tmp1=pHead1,*tmp2=pHead2,*tmp=NULL,*newHead=NULL; while(tmp1&&tmp2){ if(tmp1->val>=tmp2->val){ if(newHead==NULL){ newHead=tmp2; tmp=newHead; } else { tmp->next=tmp2; tmp=tmp->next; } tmp2=tmp2->next; } else{ if(newHead==NULL){ newHead=tmp1; tmp=newHead; }else { tmp->next=tmp1; tmp=tmp->next; } tmp1=tmp1->next; } } if(tmp2) tmp->next=tmp2; if(tmp1) tmp->next=tmp1; return newHead; } };

题意分析:1.是子结构代表2个树 数据域相同 并不是指针相同
2.可能子树结束父树 未结束
3.逻辑必须清楚,活用&& ,||符号
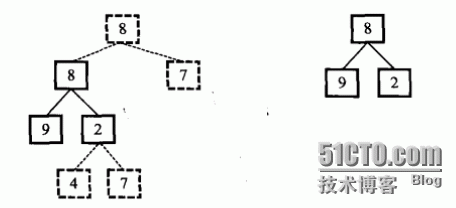
/* struct TreeNode { int val; struct TreeNode *left; struct TreeNode *right; TreeNode(int x) : val(x), left(NULL), right(NULL) { } };*/ class Solution { public: bool HasSubtree(TreeNode* pRoot1, TreeNode* pRoot2) { if(pRoot1==NULL||pRoot2==NULL) return false; if(pRoot1->val==pRoot2->val){ if(hasSubtree(pRoot1, pRoot2)) return true; } if(HasSubtree(pRoot1->left,pRoot2)||\ HasSubtree(pRoot1->right, pRoot2)) return true; return false; } bool hasSubtree(TreeNode* pRoot1, TreeNode* pRoot2) { if((pRoot1==NULL&&pRoot2!=NULL)) return false; if(pRoot2==NULL) return true; if(pRoot1->val==pRoot2->val) { if(hasSubtree(pRoot1->left,pRoot2->left)&&\ hasSubtree(pRoot1->right,pRoot2->right)) return true; return false; } return false; } };
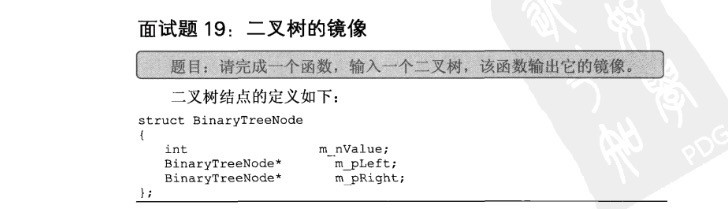
/* struct TreeNode { int val; struct TreeNode *left; struct TreeNode *right; TreeNode(int x) : val(x), left(NULL), right(NULL) { } };*/ class Solution { public: void Mirror(TreeNode *pRoot) { if(pRoot==NULL) return; TreeNode* tmp=pRoot->left; pRoot->left=pRoot->right; pRoot->right=tmp; Mirror(pRoot->left); Mirror(pRoot->right); } };

注意:用C++ vector 创建二维数组:
1.创建时用双重循环
2.操作时 写入数据必须用push_back();
3.读取数据 可用数组方法 arr[x][x];
class Solution { public: vector<int> printMatrix(vector<vector<int> > matrix) { vector<int> arr; if (matrix.size() == 0 || matrix[0].size() == 0) return arr; int num = matrix.size()*matrix[0].size() - 1; int tag = 0, flag = 0; int index = 0, row = 0, line = 0; while (1){ if (flag == 0){ for (; row<((int)matrix[0].size() - tag); row++){ arr.push_back( matrix[line][row]); index++; } if (index > num) return arr; flag++; line++; row--; } if (flag == 1){ for (; line<((int)matrix.size() - tag); line++){ arr.push_back(matrix[line][row]); index++; } if (index >num) return arr; flag++; line--; row--; } if (flag == 2){ for (; row >= tag; row--){ arr.push_back(matrix[line][row]); index++; } if (index > num) return arr; flag++; line--; row++; } if (flag == 3){ for (; line>tag; line--){ arr.push_back(matrix[line][row]); index++; } if (index > num) return arr; flag = 0; line++; row++; } tag++; } } };

class Solution { stack<int> _val,_min; public: void push(int value) { _val.push(value); if(_min.empty()){ _min.push(value); } else{ if(_min.top()>value) _min.push(value); } } void pop() { if(!_val.empty()){ if(!_min.empty()&&_min.top()==_val.top()) _min.pop(); _val.pop(); } } int top() { return 0; } int min() { if(!_min.empty()) return _min.top(); else return -1; } };
相关文章推荐
- C++;每周一些题(2)
- C++拷贝构造函数(深拷贝,浅拷贝)
- C++多重继承与二义性避免
- 第7周 C语言程序设计(新2版) 练习2-4 删除s1中与s2相匹配的字符
- C语言中的复制函数(strcpy和memcpy)
- C++输入流标志位
- C 函数值传递和指针传递的效率问题
- VC++ ^和gcnew
- c++中构造函数初始化的方法以及主要区别
- 使用jni实现在Java中调用C++的方法
- The Castle
- 关于C和c++中的文件结构
- c++ const_cast and reinterpret_cast
- 结合一道面试题 看c语言运算符的执行顺序
- 第7周 C语言程序设计(新2版) 练习2-3 字符串转换成等价整型值
- c语言编写猜数字游戏
- 2014第五届蓝桥杯C/C++程序设计本科B组决赛
- C++表达式new与delete知识详解
- C++ 使用const指针来传递对象
- VC++ MFC TRACE无法输出的问题解决