Spring之DI简述
2016-05-02 11:39
519 查看
Spring的依赖注入原理,我的理解为:在xml配置文件中,“构造”对象。用引号的原因在于,在这里并不是真正的构造。只有当我们通过ApplicationContext来加载xxx.xml文件时,这些类的对象才会被真正构造。
然而,如何构造呢?简单的来说,Spring通过调用类的构造函数进行构造。构造函数大体分两种:默认构造函数与带参数的构造函数。也就是说,Spring中通过依赖注入来构建对象的最终方法依旧是通过:类的构造函数来完成,与我们手工通过new来构造对象,并没有什么不同。
Spring的DI大体分为两种:显示构造函数DI与setter方法DI。等等,不是说,都要用构造函数(反射除外)来构造对象吗?setter方法也可以构造对象?
首先,setter方法不能构造对象,它只能修改对象的属性而已。那为什么Spring存在setter方法DI呢?其实,当采用setter方法DI时,Spring先调用的类的默认构造函数,先生成一个对象,然后调用该对象的setter方法,来修改对象的属性值。所以,采用setter方法DI时,该类必须拥有默认构造函数,否则,会构造失败。
来通过代码具体说明。
先建立一个接口:
Bean配置文件如下:
测试主函数如下:
测试结果如下:
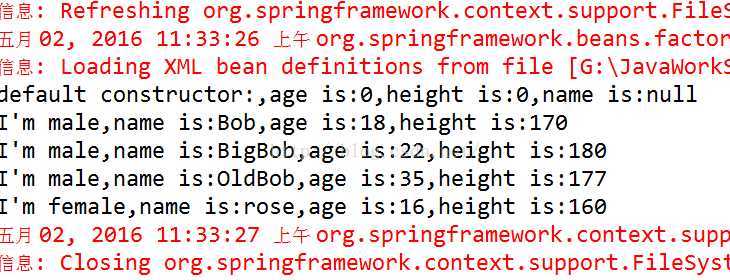
通过上述结果可以看出,setter方法DI,就是先调用了类的默认构造函数,然后在调用setter方法来修改该对象的属性。
在一个bean.xml文件中,一个bean的id值,相当于该对象的名字,即引用。所以,在一个bean.xml文件中,id不能重复。
然而,如何构造呢?简单的来说,Spring通过调用类的构造函数进行构造。构造函数大体分两种:默认构造函数与带参数的构造函数。也就是说,Spring中通过依赖注入来构建对象的最终方法依旧是通过:类的构造函数来完成,与我们手工通过new来构造对象,并没有什么不同。
Spring的DI大体分为两种:显示构造函数DI与setter方法DI。等等,不是说,都要用构造函数(反射除外)来构造对象吗?setter方法也可以构造对象?
首先,setter方法不能构造对象,它只能修改对象的属性而已。那为什么Spring存在setter方法DI呢?其实,当采用setter方法DI时,Spring先调用的类的默认构造函数,先生成一个对象,然后调用该对象的setter方法,来修改对象的属性值。所以,采用setter方法DI时,该类必须拥有默认构造函数,否则,会构造失败。
来通过代码具体说明。
先建立一个接口:
package com.bean.DI2; public interface People { public void say(); }在定义两个实现类:
package com.bean.DI2; public class Male implements People { private int age; private int height; private String name; public Male() { this.age=18; this.height=170; this.name="Bob"; } public Male(int age,int height,String name){ this.age=age; this.height=height; this.name=name; } public void setAge(int age) { this.age = age; } public void setHeight(int height) { this.height = height; } public void setName(String name) { this.name = name; } @Override public void say() { System.out.println("I'm male"+",name is:"+this.name+ ",age is:"+this.age+ ",height is:"+this.height); } }
package com.bean.DI2; public class Female implements People { private int age; private int height; private String name; public Female(){ this.age=0; this.height=0; this.name="null"; System.out.println("default constructor:"+ ",age is:"+this.age+ ",height is:"+this.height+ ",name is:"+this.name); } public Female(int age,int height,String name){ this.age=age; this.height=height; this.name=name; } public void setAge(int age) { this.age = age; } public void setHeight(int height) { this.height = height; } public void setName(String name) { this.name = name; } @Override public void say() { System.out.println("I'm female"+",name is:"+this.name+ ",age is:"+this.age+ ",height is:"+this.height); } }
Bean配置文件如下:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:c="http://www.springframework.org/schema/c" xmlns:p="http://www.springframework.org/schema/p" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!-- 调用默认构造函数 --> <bean id="male" class="com.bean.DI2.Male"> </bean> <!-- 构造函数注入 --> <bean id="male2" class="com.bean.DI2.Male" c:age="22" c:height="180" c:name="BigBob"> </bean> <!-- setter注入 --> <bean id="male3" class="com.bean.DI2.Male" p:age="35" p:height="177" p:name="OldBob"></bean> <!-- setter注入,必须拥有默认构造函数 --> <!-- setter原理为,先调用默认构造函数生成一个对象,然后在调用set方法,修改对象的属性 --> <bean id="female" class="com.bean.DI2.Female" p:age="16" p:height="160" p:name="rose"></bean> </beans>
测试主函数如下:
package com.bean.DI2; import org.springframework.context.support.FileSystemXmlApplicationContext; public class Main { public static void main(String[] args) { FileSystemXmlApplicationContext context=new FileSystemXmlApplicationContext("BeanXml\\DI2.xml"); Male male=(Male)context.getBean("male"); male.say(); Male male2=(Male)context.getBean("male2"); male2.say(); Male male3=(Male)context.getBean("male3"); male3.say(); Female female=(Female)context.getBean("female"); female.say(); context.close(); } }
测试结果如下:
通过上述结果可以看出,setter方法DI,就是先调用了类的默认构造函数,然后在调用setter方法来修改该对象的属性。
在一个bean.xml文件中,一个bean的id值,相当于该对象的名字,即引用。所以,在一个bean.xml文件中,id不能重复。
相关文章推荐
- Java 编译时多态和运行时多态
- Loadrunner中java Vuser协议脚本开发
- Hibernate hbm2java meta标签学习
- java学习-数组2
- 移位操作符
- 用Java写解谜RPG-3.MVC下的开始界面和所谓按钮
- java 实际场景下根节点 叶子节点 的不处理方法
- Java中的基础----JVM加载class文件的原理
- Java中String
- Java中String
- JAVA技术发展——你不知道的J2SE(三)
- Spring NamedParameterJdbcTemplate 详解
- java中将对象写入文件
- java多态性理解
- JAVAACRIPT基础教程(第8版)---第8章_处理事件
- Java数据类型
- 第N次重学Struts2之路(一)
- 【附源码】搭建MVC:Spring+FreeMarker+MyBatis
- 码农小汪-Spring-MVC 控制器4
- 初识java异常处理