dubbo入门实例代码
2016-04-30 23:51
369 查看
首先看一下代码结构组织结构:
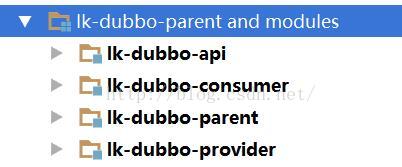
(一)lk-dubbo-parent主要用于组织三个工程,只是pom文件
(二) lk-dubbo-api代码主要是接口和实体类
(三)lk-dubbo-provider中实现dubbo服务端.
1.pom.xml
2.spring配置文件applicationContext.xml:
3.Provider.java实现类
4.DemoServiceImpl.java实现类
(四)lk-dubbo-consumer工程
1.pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd ">
<!-- 消费方应用名,用于计算依赖关系,不是匹配条件,不要与提供方一样 -->
<dubbo:application name="demo_consumer" />
<!-- 使用zookeeper注册中心暴露服务地址 -->
<!-- <dubbo:registry address="multicast://224.5.6.7:1234" /> -->
<dubbo:registry address="zookeeper://127.0.0.1:2181" />
<!-- 生成远程服务代理,可以像使用本地bean一样使用demoService -->
<dubbo:reference id="demoService"
interface="com.tgb.lk.demo.dubbo.api.DemoService" />
<!-- 使用spring默认的类注入方式,注意:需要引入lk-dubbo-provider工程 -->
<!--<bean id="demoService" class="com.tgb.lk.demo.dubbo.provider.DemoServiceImpl"></bean>-->
</beans>
3.Consumer.java实现类
代码下载地址:
http://download.csdn.net/detail/lk_blog/9507435
(一)lk-dubbo-parent主要用于组织三个工程,只是pom文件
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <!--<parent>--> <!--<artifactId>lk-demo-parent</artifactId>--> <!--<groupId>com.tgb.lk</groupId>--> <!--<version>1.0.0-SNAPSHOT</version>--> <!--<relativePath>../../lk-demo-parent/pom.xml</relativePath>--> <!--</parent>--> <modelVersion>4.0.0</modelVersion> <version>1.0.0-SNAPSHOT</version> <groupId>com.tgb.lk</groupId> <artifactId>lk-dubbo-parent</artifactId> <packaging>pom</packaging> <modules> <module>../lk-dubbo-api</module> <module>../lk-dubbo-provider</module> <module>../lk-dubbo-consumer</module> </modules> <properties> <spring.version>4.2.4.RELEASE</spring.version> <dubbo.version>2.5.3</dubbo.version> <zookeeper.version>3.4.7</zookeeper.version> <zkclient.version>0.7</zkclient.version> </properties> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.3</version> <configuration> <encoding>UTF-8</encoding> </configuration> </plugin> </plugins> </build> </project>
(二) lk-dubbo-api代码主要是接口和实体类
package com.tgb.lk.demo.dubbo.api; import java.io.Serializable; public class User implements Serializable { private static final long serialVersionUID = 1L; private int age; private String name; private String sex; public User() { super(); } public User(int age, String name, String sex) { super(); this.age = age; this.name = name; this.sex = sex; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getSex() { return sex; } public void setSex(String sex) { this.sex = sex; } }
package com.tgb.lk.demo.dubbo.api; import java.util.List; public interface DemoService { String sayHello(String name); public List<User> getUsers(); }
(三)lk-dubbo-provider中实现dubbo服务端.
1.pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <parent> <artifactId>lk-dubbo-parent</artifactId> <groupId>com.tgb.lk</groupId> <version>1.0.0-SNAPSHOT</version> <relativePath>../lk-dubbo-parent/pom.xml</relativePath> </parent> <modelVersion>4.0.0</modelVersion> <groupId>com.tgb.lk</groupId> <artifactId>lk-dubbo-provider</artifactId> <dependencies> <dependency> <groupId>com.tgb.lk</groupId> <artifactId>lk-dubbo-api</artifactId> <version>1.0.0-SNAPSHOT</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>dubbo</artifactId> <version>${dubbo.version}</version> <exclusions> <exclusion> <groupId>org.springframework</groupId> <artifactId>spring</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>com.101tec</groupId> <artifactId>zkclient</artifactId> <version>${zkclient.version}</version> <exclusions> <exclusion> <groupId>javax.mail</groupId> <artifactId>mail</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>org.apache.zookeeper</groupId> <artifactId>zookeeper</artifactId> <version>${zookeeper.version}</version> </dependency> </dependencies> </project>
2.spring配置文件applicationContext.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd "> <!-- 具体的实现bean --> <bean id="demoService" class="com.tgb.lk.demo.dubbo.provider.DemoServiceImpl" /> <!-- 提供方应用信息,用于计算依赖关系 --> <dubbo:application name="demo_provider" /> <!-- 使用multicast广播注册中心暴露服务地址 <dubbo:registry address="multicast://224.5.6.7:1234" /> --> <!-- 使用zookeeper注册中心暴露服务地址 --> <dubbo:registry address="zookeeper://127.0.0.1:2181" /> <!-- 用dubbo协议在20880端口暴露服务 --> <dubbo:protocol name="dubbo" port="20882" /> <!-- 声明需要暴露的服务接口 --> <dubbo:service interface="com.tgb.lk.demo.dubbo.api.DemoService" ref="demoService" /> </beans>
3.Provider.java实现类
package com.tgb.lk.demo.dubbo.provider; import org.springframework.context.support.ClassPathXmlApplicationContext; public class Provider { public static void main(String[] args) throws Exception { ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext( new String[] { "applicationContext.xml" }); context.start(); System.out.println("启动成功"); System.in.read(); // 为保证服务一直开着,利用输入流的阻塞来模拟 } }
4.DemoServiceImpl.java实现类
package com.tgb.lk.demo.dubbo.provider; import com.tgb.lk.demo.dubbo.api.DemoService; import com.tgb.lk.demo.dubbo.api.User; import java.util.ArrayList; import java.util.List; public class DemoServiceImpl implements DemoService { public String sayHello(String name) { System.out.println("threadName: "+Thread.currentThread()+" content:"+name); try { Thread.sleep(1); } catch (InterruptedException e) { e.printStackTrace(); } return "Hello " + name; } public List getUsers() { List<User> list = new ArrayList<User>(); User u1 = new User(); u1.setName("jack"); u1.setAge(20); u1.setSex("m"); User u2 = new User(); u2.setName("tom"); u2.setAge(21); u2.setSex("m"); User u3 = new User(); u3.setName("rose"); u3.setAge(19); u3.setSex("w"); list.add(u1); list.add(u2); list.add(u3); return list; } }
(四)lk-dubbo-consumer工程
1.pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <parent> <artifactId>lk-dubbo-parent</artifactId> <groupId>com.tgb.lk</groupId> <version>1.0.0-SNAPSHOT</version> <relativePath>../lk-dubbo-parent/pom.xml</relativePath> </parent> <modelVersion>4.0.0</modelVersion> <groupId>com.tgb.lk</groupId> <artifactId>lk-dubbo-consumer</artifactId> <dependencies> <dependency> <groupId>com.tgb.lk</groupId> <artifactId>lk-dubbo-api</artifactId> <version>1.0.0-SNAPSHOT</version> </dependency> <!--<dependency>--> <!--<groupId>com.tgb.lk</groupId>--> <!--<artifactId>lk-dubbo-provider</artifactId>--> <!--<version>1.0.0-SNAPSHOT</version>--> <!--</dependency>--> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>dubbo</artifactId> <version>${dubbo.version}</version> <exclusions> <exclusion> <groupId>org.springframework</groupId> <artifactId>spring</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>com.101tec</groupId> <artifactId>zkclient</artifactId> <version>${zkclient.version}</version> <exclusions> <exclusion> <groupId>javax.mail</groupId> <artifactId>mail</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>org.apache.zookeeper</groupId> <artifactId>zookeeper</artifactId> <version>${zookeeper.version}</version> </dependency> </dependencies> </project>
</pre><p></p><pre>2.spring配置文件applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd ">
<!-- 消费方应用名,用于计算依赖关系,不是匹配条件,不要与提供方一样 -->
<dubbo:application name="demo_consumer" />
<!-- 使用zookeeper注册中心暴露服务地址 -->
<!-- <dubbo:registry address="multicast://224.5.6.7:1234" /> -->
<dubbo:registry address="zookeeper://127.0.0.1:2181" />
<!-- 生成远程服务代理,可以像使用本地bean一样使用demoService -->
<dubbo:reference id="demoService"
interface="com.tgb.lk.demo.dubbo.api.DemoService" />
<!-- 使用spring默认的类注入方式,注意:需要引入lk-dubbo-provider工程 -->
<!--<bean id="demoService" class="com.tgb.lk.demo.dubbo.provider.DemoServiceImpl"></bean>-->
</beans>
3.Consumer.java实现类
package com.tgb.lk.demo.dubbo.consumer; import com.tgb.lk.demo.dubbo.api.DemoService; import com.tgb.lk.demo.dubbo.api.User; import org.springframework.context.support.ClassPathXmlApplicationContext; import java.util.List; import java.util.concurrent.*; public class Consumer { public static void main(String[] args) throws Exception { ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext( new String[]{"applicationContext.xml"}); context.start(); final DemoService demoService = (DemoService) context.getBean("demoService"); //测试简单返回值 String hello = demoService.sayHello("tom"); System.out.println(hello); //测试复杂返回值 List<User> list = demoService.getUsers(); if (list != null && list.size() > 0) { for (int i = 0; i < list.size(); i++) { System.out.println(list.get(i)); } } //测试性能 ExecutorService threadPool = Executors.newFixedThreadPool(10); int count = 10000; long start = System.currentTimeMillis(); Future<Integer> future = null; for (int i = 1; i <= count; i++) { final int j = i; future = threadPool.submit(new Callable<Integer>() { public Integer call() throws Exception { demoService.sayHello("tom " + j); return j; } }); } if (future != null && future.get() == count) { //远程调用完返回后记录结束时间 long end = System.currentTimeMillis(); System.out.println("consume time " + (end - start) + "ms"); } System.in.read(); } }
代码下载地址:
http://download.csdn.net/detail/lk_blog/9507435
相关文章推荐
- java 建造者模式
- 【NYOJ-100】 1的个数
- 给定两个字符串,请编写程序,确定其中一个字符串的字符重新排列后,能否变成另一个字符串。这里规定大小写为不同字符,且考虑字符串重点空格。 给定一个string stringA和一个string stri
- Java中交集、并集、差集的实现
- Java多线程编程(一)
- ContentProvider
- 请实现一个算法,确定一个字符串的所有字符是否全都不同。这里我们要求不允许使用额外的存储结构
- C++中的重载 覆盖 隐藏的规律
- 20145302张薇《Java程序设计》第九周学习总结
- 20145238-荆玉茗 《Java程序设计》第9周学习总结
- java设计模式
- 以一则LUA实例说明敏捷开发中“分离构造和使用”原则
- Qt浅谈之五十一QT_OpenGL
- Spring高级应用之注入嵌套Bean
- java环境变量设置
- 第一个Java程序
- Spring高级应用之注入各类集合
- HDU3820 Golden Eggs(最小割)
- C++模板
- Python学习笔记15