Code Hunt 题解 00-04 (Java)
2016-04-30 10:37
603 查看
最近做了做微软的编程小游戏 Code Hunt,这是一个给你输入输出,让你反推程序代码,同时会根据你的代码精炼程度打分的小游戏。
强迫症同学们请小心哦~
链接:https://www.codehunt.com
作为一个java新手,最近刷这个刷了半天~ 给大家贴个题解(网上的怎么都是C#版本的)
(嗯,00-08中的好多都来自于网络。。。后面的就是自己做的了)
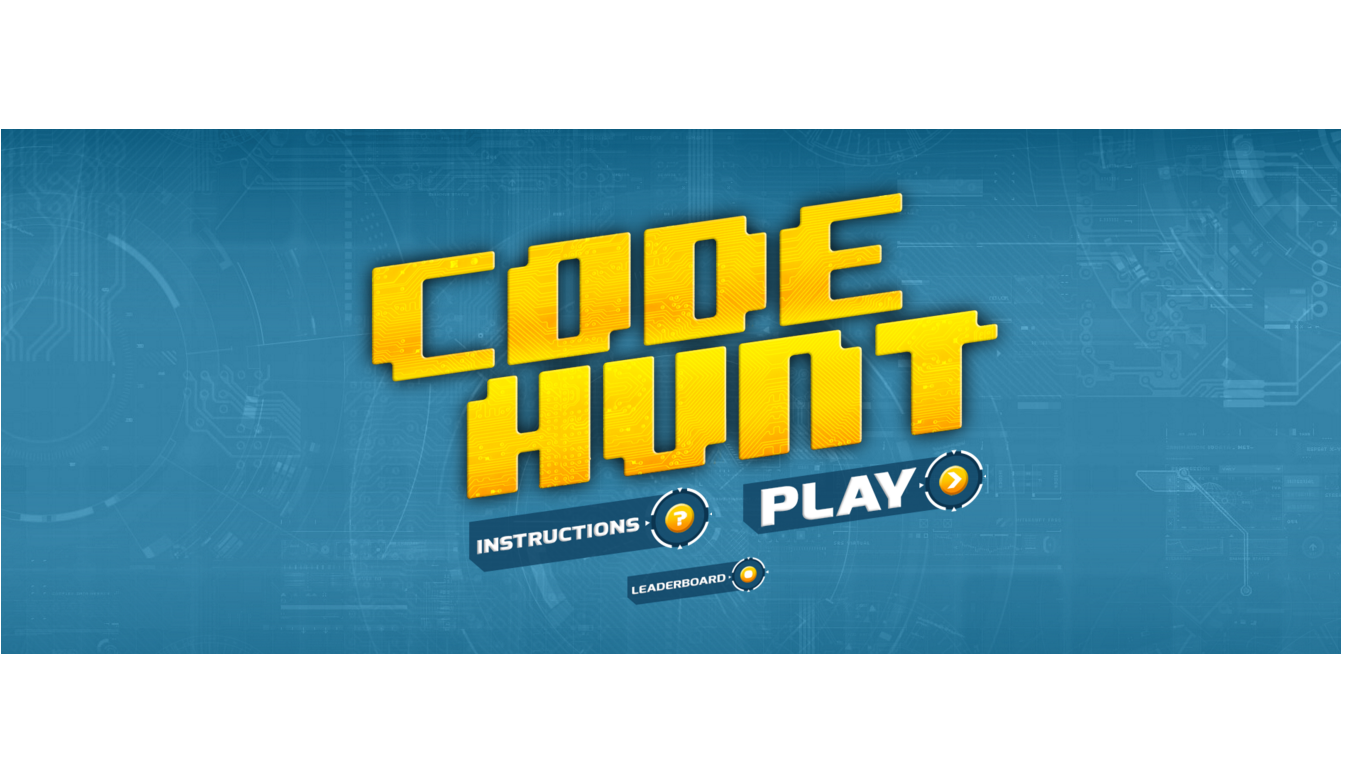
Sector 00 Training
Sector 01 Arithmetic
Sector 02 Loops
Sector 03 Loops 2
Sector 04 Conditionals
强迫症同学们请小心哦~
链接:https://www.codehunt.com
作为一个java新手,最近刷这个刷了半天~ 给大家贴个题解(网上的怎么都是C#版本的)
(嗯,00-08中的好多都来自于网络。。。后面的就是自己做的了)
Sector 00 Training
00.02 public class Program { public static int Puzzle(int x) { return x+1; } }
00.03 public class Program { public static int Puzzle(int x) { return x*2; } }
00.04 public class Program { public static int Puzzle(int x, int y) { return x+y; } }
Sector 01 Arithmetic
01.01 public class Program { public static int Puzzle(int x) { return -x; } }
01.02 public class Program { public static int Puzzle(int x) { return x-2; } }
01.03 public class Program { public static int Puzzle(int x) { return x*x; } }
01.04 public class Program { public static int Puzzle(int x) { return x*3; } }
01.05 public class Program { public static int Puzzle(int x) { return x/3; } }
01.06 public class Program { public static int Puzzle(int x) { return 4/x; } }
01.07 public class Program { public static int Puzzle(int x, int y) { return x-y; } }
01.08 public class Program { public static int Puzzle(int x, int y) { return x+2*y; } }
01.09 public class Program { public static int Puzzle(int x, int y) { return x*y; } }
01.10 public class Program { public static int Puzzle(int x, int y) { return x+y/3; } }
01.11 public class Program { public static int Puzzle(int x, int y) { return x/y; } }
01.12 public class Program { public static int Puzzle(int x) { return x%3; } }
01.13 public class Program { public static int Puzzle(int x) { return x%3+1; } }
01.14 public class Program { public static int Puzzle(int x) { return 10%x; } }
01.15 public class Program { public static int Puzzle(int x, int y, int z) { return (x+y+z)/3; } }
Sector 02 Loops
02.01 public class Program { public static int[] Puzzle(int n) { int[]puzzle=new int ; for (int i=0;i<n;i++) puzzle[i]=i; return puzzle; } }
02.02 public class Program { public static int[] Puzzle(int n) { int[]puzzle=new int ; for (int i=0;i<n;i++) puzzle[i]=i*n; return puzzle; } }
02.03 public class Program { public static int[] Puzzle(int n) { int[] puzzle=new int ; for (int i=0;i<n;i++) puzzle[i]=i*i; return puzzle; } }
02.04 public class Program { public static int Puzzle(int[] v) { int sum=0; for (int i=0;i<v.length;i++) sum+=v[i]; return sum; } }
02.05 <必须直接写公式才有三星> public class Program { public static int Puzzle(int n) { return (n*(n-1)*(2*n-1))/6; } }
02.06 <这题非常迷。。。replace()方法以后会常用> public class Program { public static int Puzzle(String s) { String s2=s.replace("a",""); return s.length()-s2.length(); } }
02.07 public class Program { public static int Puzzle(String s, char x) { String s1=s.replace(x+"",""); return s.length()-s1.length(); } }
Sector 03 Loops 2
03.01 <写成递归才有三星> public class Program { public static int Puzzle(int number, int power) { return power<=0? 1:Puzzle(number,power-1)*number; } }
03.02 public class Program { public static int Puzzle(int i) { return i<=0? 1:i*Puzzle(i-1); } }
03.03 public class Program { public static int Puzzle(int lowerBound, int upperBound) { return lowerBound>upperBound? 1: upperBound*Puzzle(lowerBound,upperBound-1); } }
03.04 <神奇的整除> public class Program { public static int Puzzle(int n) { if (n<=2) return 0; return ((n-1)/2) *((n-1)/2+1); } }
03.05 public class Program { public static int Puzzle(int upperBound) { return (upperBound)*(upperBound+1)*(upperBound+2)/6; } }
03.06 <replaceAll()方法用到正则表达式!这题是照抄网上大神们的。。。。还有trim()方法会常用> public class Program { public static String Puzzle(String word) { return word.replaceAll("[a-z]","_ ").trim(); } }
03.07 public class Program { public static String Puzzle(String s) { int m = 5; char[] c = new char[s.length()]; for (int i=0;i<s.length();i++) { c[i]=(char)((s.charAt(i)-'a'+m)%26+'a'); } return new String(c); } }
03.08 public class Program { public static int Puzzle(int x) { return (int)(Math.log10(x))+1; } }
Sector 04 Conditionals
04.01 public class Program { public static Boolean Puzzle(Boolean x, Boolean y) { return x || y; } }
04.02 public class Program { public static Boolean Puzzle(Boolean x, Boolean y) { return x && y; } }
04.03 public class Program { public static Boolean Puzzle(int x) { return (x<50); } }
04.04 public class Program { public static Boolean Puzzle(int x, int y) { return x<y; } }
04.05 public class Program { public static int Puzzle(int i) { return (int)(Math.signum(i)); } }
04.06 public class Program { public static Boolean Puzzle(int i, int j) { return i>j; } }
04.07 public class Program { public static int Puzzle(int i) { return i<100? 2:3; } }
04.08 public class Program { public static String Puzzle(int i) { return i%2==0? "even": "odd"; } }
04.09 public class Program { public static String Puzzle(int i) { return i%5==0?"multiple of 5":"not a multiple of 5"; } }
04.10 public class Program { public static String Puzzle(int i, int x) { return i%x==0? "multiple of " + x : "not a multiple of " + x; } }
04.11 public class Program { public static String Puzzle(int i, int j, int k) { if (j==2*k && i==2*j) return("yes!"); return ("no"); } }
04.12 <多试几次就出来了> public class Program { public static int Puzzle(int i) { return i>14? 21: i>7? 7:0; } }
相关文章推荐
- Struts2 S2 – 032远程代码执行漏洞分析报告
- ubuntu 14.04 java开发环境搭建 jdk 以及 inteliJ IDEA安装
- Java 中泛型的协变
- 【Java】使用javaassist修改jar包
- java-----String的intern关键字
- Java 性能笔记:自动装箱/拆箱
- Spring注解详解
- <Java中的继承和组合之间的联系和区别>
- Java基础之理解Annotation(与@有关,即是注释)
- Java中正则表达式的几种用法
- 如何查看JDK以及JAVA框架的源码
- Eclipse启动报错”java was started but returned exit code=13”
- 高吞吐低延迟Java应用的垃圾回收优化
- 成为JavaGC专家(1)—深入浅出Java垃圾回收机制
- java字符串处理
- 五、JAVA数组
- Java类和对象及实例
- 【JAVA实例】代码生成器的原理讲解以及实际使用
- 【JAVA实例】代码生成器的原理讲解以及实际使用
- java mail 邮件创建--基本原理