同或门(XNOR)电路的网络学习
2016-04-22 20:50
651 查看
主要内容,参考http://www.analyticsvidhya.com/blog/2016/04/neural-networks-python-theano/
本文使用Theano实现XNOR功能。对tensor的变量定义、自动求导,以及theano.function做一个简单学习。具体如下:
XNOR函数的网络表达:
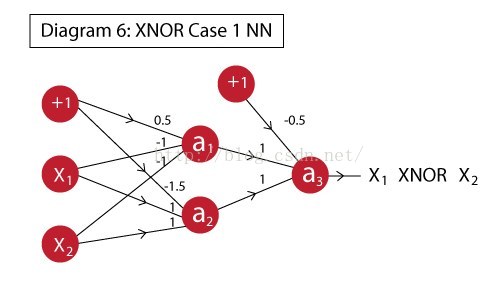
真值表:
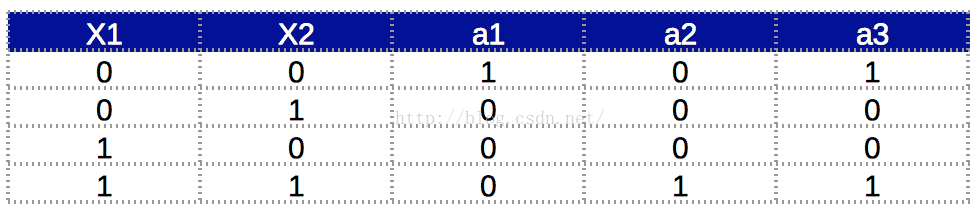
编程实现:
实验结果:
The outputs of the NN are:
The output for x1=0 | x2=0 is 1.00
The output for x1=0 | x2=1 is 0.00
The output for x1=1 | x2=0 is 0.00
The output for x1=1 | x2=1 is 1.00
The flow of cost during model run is as following:
本文使用Theano实现XNOR功能。对tensor的变量定义、自动求导,以及theano.function做一个简单学习。具体如下:
XNOR函数的网络表达:
真值表:
编程实现:
import theano import theano.tensor as T import numpy as np import matplotlib.pyplot as plt if __name__ == '__main__': #Define variables: x = T.matrix('x') w1 = theano.shared(np.random.rand(2,2)) # weight for layer1 w2 = theano.shared(np.random.rand(2)) b = theano.shared(np.ones(2)) # bias learning_rate = 1.; #Step2:Define mathematical expression a1 = 1/(1+T.exp(-T.dot(x,w1)-b[0])) a = 1/(1+T.exp(-T.dot(a1,w2)-b[1])) # predict output #Step3:Define gradient and update rule y = T.vector('y') #Actual output cost = -(y*T.log(a) + (1-y)*T.log(1-a)).sum() dw1,dw2,db = T.grad(cost,[w1,w2,b]) train = theano.function( inputs = [x,y], outputs = [a,cost], updates = [ [w1, w1-learning_rate*dw1], [w2, w2-learning_rate*dw2], [b, b-learning_rate*db] ] ) inputs = [ [0, 0], [0, 1], [1, 0], [1, 1] ] outputs = [1,0,0,1] #Iterate through all inputs and find outputs: cost = [] for iteration in range(1000): pred, cost_iter = train(inputs, outputs) cost.append(cost_iter) #Print the outputs: print 'The outputs of the NN are:' for i in range(len(inputs)): print 'The output for x1=%d | x2=%d is %.2f' % (inputs[i][0],inputs[i][1],pred[i]) #Plot the flow of cost: print '\nThe flow of cost during model run is as following:' plt.plot(cost)
实验结果:
The outputs of the NN are:
The output for x1=0 | x2=0 is 1.00
The output for x1=0 | x2=1 is 0.00
The output for x1=1 | x2=0 is 0.00
The output for x1=1 | x2=1 is 1.00
The flow of cost during model run is as following:
相关文章推荐
- Python学习十一——theano库符号求导示例代码
- ubuntu theano 安装成功,windows theano安装失败
- Theano 模块 基础知识篇
- Windows7+Anaconda+Theano+Pylearn2深度学习环境搭建
- NN学习笔记
- deep learning 个人理解及其实现工具
- unbutu12.04 64bit系统 安装theano+cuda5.5,并运行deeplearningtutorial里面的代码
- 深度学习笔记:windows10+visual studio 2013+cuda7.5+theano+lasagne环境配置
- 利用Theano实现简单手写识别
- Windows安装Theano
- exact nn search in hamming space
- Windows8.1下安装theano和CUDA
- Windows 7 64位 安装配置Theano 配置GPU
- theano的配置过程
- Win7 中安装Theano及配置CUDA以搭建GPU加速环境
- debian下安装Theano(附pydot问题解决方法)
- Theano(1):windows、linux下安装深度学习库Theano
- Theano(3):Theano【数据类型】与【代码初尝试】
- Theano(6):Theano条件语句,IfElse vs Switch
- Theano(7):Theano循环语句,Scan