UVA 1349 Optimal Bus Route Design(网络流)
2016-04-16 15:46
621 查看
题意:给n个点的有向带权图,找若干个有向圈,使得每个点恰好属于一个圈,要求权和尽量小。
思路:每个点恰好属于一个有向圈,意味着每一个点都有一个唯一的后继,反过来,只要每一个点都有唯一的后继,每个点一定恰好属于一个圈,说明每个点出度为1,入度为1,然后拆点做网络流吧
Description
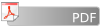
A big city wants to improve its bus transportation system. One of the improvement is to add scenic routes which go es through attractive places. Your task is to construct a bus-route-plan for sight-seeing buses
in a city.
You are given a set of scenic lo cations. For each of these given lo cations, there should be only one bus route that passes this lo cation, and that bus route should pass this lo cation exactly once. The number
of bus routes is unlimited. However, each route should contain at least two scenic lo cations.
From location i to location j , there may or may not be a connecting street. If there is a street from location i to
location j , then we say j is an out-neighbor of i . The length of the street from i to j is d (i, j) .
The streets might be one way. So it may happen that there is a street from i to j , but no street from j to i . In case
there is a street from i to j and also a street from j to i , the lengths d (i, j) and d (j, i) might
be different. The route of each bus must follow the connecting streets and must be a cycle. For example, the route of Bus A might be from location 1 to location 2, from location 2 to location 3, and then from location 3 to location 1. The route of Bus B might
be from location 4 to location 5, then from location 5 to location 4. The length of a bus route is the sum of the lengths of the streets in this bus route. The total length of the bus-route-plan is the sum of the lengths of all the bus routes used in the plan.
A bus-route-plan is optimal if it has the minimum total length. You are required to compute the total length of an optimal bus-route-plan.
are denoted by positive integers 1, 2,..., n . The next n lines are information about connecting streets between these lo cations. The i -th line of these n lines
consists of an even number of positive integers and a 0 at the end. The first integer is a lo cation jwhich is an out-neighbor of location i , and the second integer is d (i, j) .
The third integer is another location j' which is an out-neighbor of i , and the fourth integer is d (i, j') , and so on. In general,
the (2k - 1) th integer is a location t which is an out-neighbor of location i , and the 2k th integer is d (i, t) .
The next case starts immediately after these n lines. A line consisting of a single ` 0' indicates the end of the input file.
Each test case has at most 99 locations. The length of each street is a positive integer less than 100.
a letter `N'.
思路:每个点恰好属于一个有向圈,意味着每一个点都有一个唯一的后继,反过来,只要每一个点都有唯一的后继,每个点一定恰好属于一个圈,说明每个点出度为1,入度为1,然后拆点做网络流吧
#include<bits/stdc++.h> using namespace std; #define LL long long const int maxn = 2005; #define INF 1e9 struct Edge { int from,to,cap,flow,cost; Edge(int u,int v,int c,int f,int w):from(u),to(v),cap(c),flow(f),cost(w){} }; struct MCMF { int n,m; vector<Edge>edges; vector<int>G[maxn]; int inq[maxn]; int d[maxn]; int p[maxn]; int a[maxn]; void init(int n) { this->n=n; for (int i =0;i<n;i++) G[i].clear(); edges.clear(); } void AddEdge(int from,int to,int cap,int cost) { edges.push_back(Edge(from,to,cap,0,cost)); edges.push_back(Edge(to,from,0,0,-cost)); m = edges.size(); G[from].push_back(m-2); G[to].push_back(m-1); } bool BellmanFord(int s,int t,int &flow,LL &cost) { for (int i = 0;i<n;i++) d[i]=INF; memset(inq,0,sizeof(inq)); d[s]=0; inq[s]=1; p[s]=0; a[s]=INF; queue<int>q; q.push(s); while (!q.empty()) { int u = q.front(); q.pop(); inq[u]=0; for (int i =0;i<G[u].size();i++){ Edge&e = edges[G[u][i]]; if (e.cap>e.flow&&d[e.to]>d[u]+e.cost){ d[e.to]=d[u]+e.cost; p[e.to]=G[u][i]; a[e.to]=min(a[u],e.cap-e.flow); if (!inq[e.to]) { q.push(e.to); inq[e.to]=1; } } } } if (d[t]==INF) return false; flow+=a[t]; cost+=(LL)d[t]*(LL)a[t]; for (int u = t;u!=s;u=edges[p[u]].from){ edges[p[u]].flow+=a[t]; edges[p[u]^1].flow-=a[t]; } return true; } int MincostMaxflow(int s,int t,LL &cost) { int flow = 0; cost = 0; while (BellmanFord(s,t,flow,cost)); return flow; } }mc; int main() { int n,m; while (scanf("%d",&n)!=EOF && n) { mc.init(2*n+2); for (int u = 0;u<n;u++) { int v,w; while (scanf("%d",&v)!=EOF && v) { scanf("%d",&w); v--; mc.AddEdge(u,v+n,1,w); } mc.AddEdge(2*n,u,1,0); mc.AddEdge(u+n,n*2+1,1,0); } LL cost=0; int flow = mc.MincostMaxflow(n*2,n*2+1,cost); if (flow < n) printf("N\n"); else printf("%lld\n",cost); } }
Description
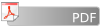
A big city wants to improve its bus transportation system. One of the improvement is to add scenic routes which go es through attractive places. Your task is to construct a bus-route-plan for sight-seeing buses
in a city.
You are given a set of scenic lo cations. For each of these given lo cations, there should be only one bus route that passes this lo cation, and that bus route should pass this lo cation exactly once. The number
of bus routes is unlimited. However, each route should contain at least two scenic lo cations.
From location i to location j , there may or may not be a connecting street. If there is a street from location i to
location j , then we say j is an out-neighbor of i . The length of the street from i to j is d (i, j) .
The streets might be one way. So it may happen that there is a street from i to j , but no street from j to i . In case
there is a street from i to j and also a street from j to i , the lengths d (i, j) and d (j, i) might
be different. The route of each bus must follow the connecting streets and must be a cycle. For example, the route of Bus A might be from location 1 to location 2, from location 2 to location 3, and then from location 3 to location 1. The route of Bus B might
be from location 4 to location 5, then from location 5 to location 4. The length of a bus route is the sum of the lengths of the streets in this bus route. The total length of the bus-route-plan is the sum of the lengths of all the bus routes used in the plan.
A bus-route-plan is optimal if it has the minimum total length. You are required to compute the total length of an optimal bus-route-plan.
Input
The input file consists of a number of test cases. The first line of each test case is a positive integer n , which is the number of locations. These n locationsare denoted by positive integers 1, 2,..., n . The next n lines are information about connecting streets between these lo cations. The i -th line of these n lines
consists of an even number of positive integers and a 0 at the end. The first integer is a lo cation jwhich is an out-neighbor of location i , and the second integer is d (i, j) .
The third integer is another location j' which is an out-neighbor of i , and the fourth integer is d (i, j') , and so on. In general,
the (2k - 1) th integer is a location t which is an out-neighbor of location i , and the 2k th integer is d (i, t) .
The next case starts immediately after these n lines. A line consisting of a single ` 0' indicates the end of the input file.
Each test case has at most 99 locations. The length of each street is a positive integer less than 100.
Output
The output contains one line for each test case. If the required bus-route-plan exists, then the output is a positive number, which is the total length of an optimal bus-route-plan. Otherwise, the output isa letter `N'.
Sample Input
3 2 2 3 1 0 1 1 3 2 0 1 3 2 7 0 8 2 3 3 1 0 3 3 1 1 4 4 0 1 2 2 7 0 5 4 6 7 0 4 4 3 9 0 7 4 8 5 0 6 2 5 8 8 1 0 6 6 7 2 0 3 2 1 0 3 1 0 2 1 0 0
Sample Output
7 25 N
相关文章推荐
- 网络流二十四题之二 —— 太空飞行计划问题(SHUT)
- 安卓与HTTPS
- HttpServletResponse 和 HttpServletRequest的应用场景
- 网络的介数中心性(betweenness)及计算方法
- iOS应用访问不了网络的解决方法 App Transport Security has blocked a cleartext HTTP
- HttpClient 教程
- 网络:YYModel 使用(JSON到模型的转换)
- 网络:RSA 加密
- 网络:对称加密
- 网络:自定义模型转 JSON
- hdu4971A simple brute force problem[【网络流最大流】
- 南邮第八届程序设计竞赛之网络预选赛小结 一(错题集)
- 网络:提交 JSON 到服务器中
- OKHttp源码解析
- [学习笔记]Java网络编程之TCP通讯
- VMware10中的Linux系统利用NAT网络连接方式访问外网配置
- [学习笔记]Java网络编程之UDP通讯
- CentOS7 最小化安装后启用无线连接网络
- 网络:上传文件(单个与多个)
- HTTP 访问接口封装,app开发中常用