iOS开发-纯代码自定义TableViewCell
2016-04-16 00:00
435 查看
摘要: 纯代码自定义TableViewCell,Masonry自动布局,自适应高度
以一个评论的 cell 为例:
ZXY_XC_CommentCell.h
其中,的 heightForCellWithComment方法用于返回 cell 的高度,在控制器的UITableViewDelegate方法heightForRowAtIndexPath中调用
ZXY_XC_CommentCell.m 文件
实际展示的具体效果如下:
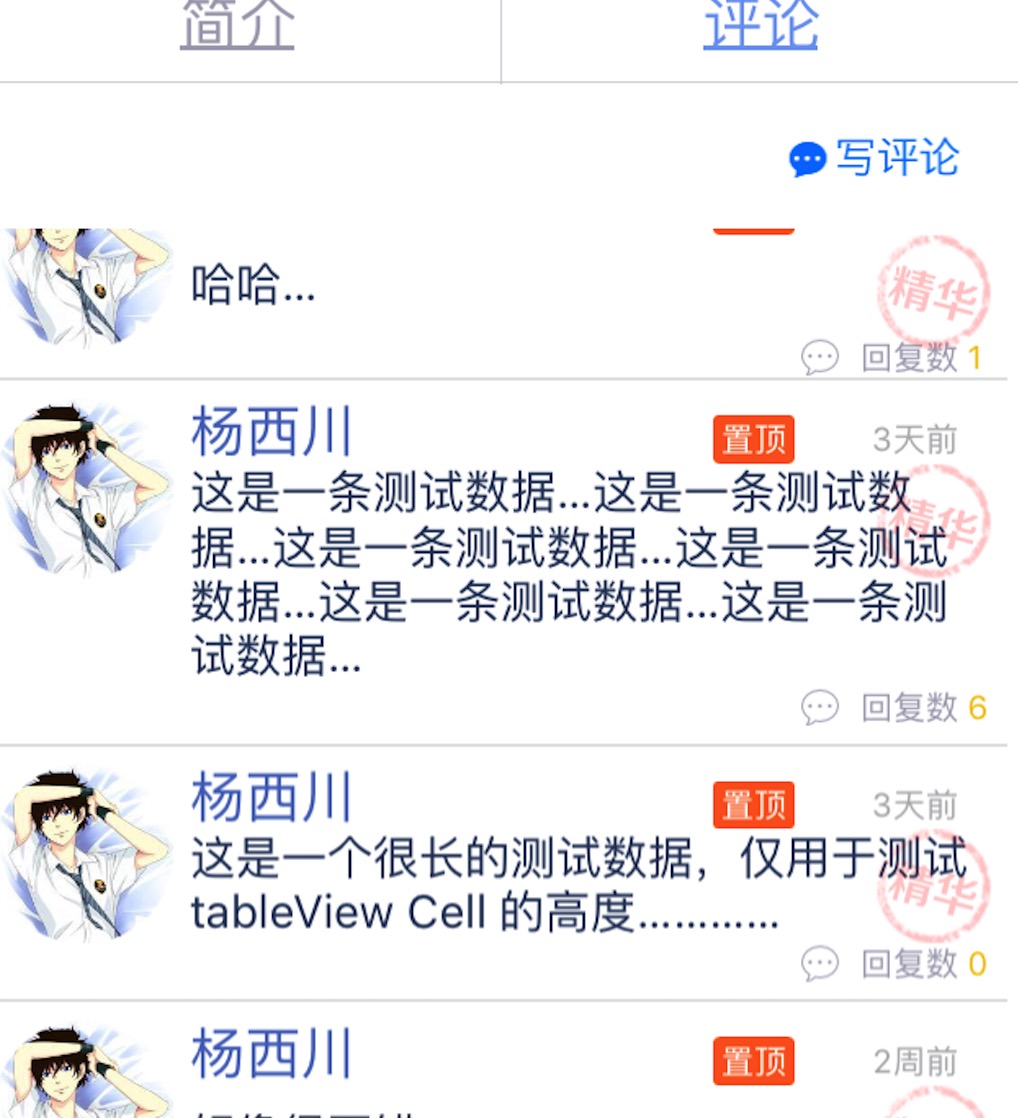
层级视图中的cell样式:
以一个评论的 cell 为例:
ZXY_XC_CommentCell.h
// // ZXY_XC_CommentCell.h // // Created by pro on 16/4/14. // Copyright © 2016年 蓝泰致铭. All rights reserved. // /** * 评论 cell * * Code by: * yangxichuan */ #import <UIKit/UIKit.h> #import "Comment.h" @interface ZXY_XC_CommentCell : UITableViewCell @property (nonatomic, strong) Comment * comment; + (CGFloat)heightForCellWithComment:(Comment *)comment; @end
其中,的 heightForCellWithComment方法用于返回 cell 的高度,在控制器的UITableViewDelegate方法heightForRowAtIndexPath中调用
- (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath { Comment *comment =_commentListArr[indexPath.row]; return [ZXY_XC_CommentCell heightForCellWithComment:comment]; }
ZXY_XC_CommentCell.m 文件
// // ZXY_XC_CommentCell.m // // Created by pro on 16/4/14. // Copyright © 2016年 蓝泰致铭. All rights reserved. // #import "ZXY_XC_CommentCell.h" #import "UIImageView+WebCache.h" #import "jumpPush.h" @interface ZXY_XC_CommentCell () @property (strong, nonatomic) UIImageView * backgroundImage; @property (strong, nonatomic) UIImageView * userHeaderImage; @property (strong, nonatomic) UILabel * userName; @property (strong, nonatomic) UILabel * userTime; @property (strong, nonatomic) UILabel * userContent; /**< 用户评论的内容 */ @property (strong, nonatomic) EDStarRating * userStar; @property (strong, nonatomic) UILabel * userComment; /**< lable:阅读人数:99 */ @property (strong, nonatomic) UILabel * topLabel; @property (strong, nonatomic) UIImageView * replyImage; @property (nonatomic, strong) UIImageView * essenceImage; /**< 精华图片 */ @end @implementation ZXY_XC_CommentCell /** 在使用xib来加载cell进行初始化时,会被调用。可以在这里进行一些初始化操作 */ - (instancetype)initWithCoder:(NSCoder *)aDecoder { if (self = [super initWithCoder: aDecoder]) { // } return self; } /** 初始化方法,自定义 cell时,不清楚高度,可以在这里添加子空间 */ - (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier { if (self = [super initWithStyle:style reuseIdentifier:reuseIdentifier]) { [self setupUI]; } return self; } /** 使用xib初始化cell,并完成IBoutlet 及IBAction 的关联后,可以在这里设置它们的默认值或配置 */ - (void)awakeFromNib { [super awakeFromNib]; } /** 在这里可以布局cell中的元素,当cell的frame改变或旋转时会触发这个方法 */ - (void)layoutSubviews { } /** 当需要在上下文中绘制时可以在这里处理 */ - (void)drawRect:(CGRect)rect { } - (void)setSelected:(BOOL)selected animated:(BOOL)animated { [super setSelected:selected animated:animated]; // Configure the view for the selected state } #pragma mark - 基本的内部控件 - (void)setupUI { _userName = [[UILabel alloc]init]; _userName.textColor = RGB(80, 112, 193); _userHeaderImage = [[UIImageView alloc]init]; _userHeaderImage.layer.cornerRadius = 55 / 2.0; //图片宽度的一般 _userHeaderImage.layer.masksToBounds = YES; _userTime = [[UILabel alloc]init]; _userTime.textColor = RGB(178, 179, 181); _userTime.textAlignment = NSTextAlignmentRight; _userTime.font = [UIFont systemFontOfSize:10]; _userContent = [[UILabel alloc]init]; _userContent.font = [UIFont systemFontOfSize:14]; _userContent.textAlignment = NSTextAlignmentLeft; _userContent.textColor = RGB(40, 60, 97); _userContent.numberOfLines = 0; _userComment = [[UILabel alloc]init]; _userComment.font = [UIFont systemFontOfSize:10]; _replyImage = [[UIImageView alloc]init]; _replyImage.image = ImageNamed(@"course_icon_comment_s"); _essenceImage = [[UIImageView alloc]init]; _essenceImage.image = ImageNamed(@"icon_digest"); _essenceImage.hidden = YES; _topLabel = [[UILabel alloc] init]; _topLabel.backgroundColor = RGB(252, 97, 33); _topLabel.font = [UIFont systemFontOfSize:10]; _topLabel.textAlignment = NSTextAlignmentCenter; _topLabel.textColor = [UIColor whiteColor]; _topLabel.layer.cornerRadius = 2; _topLabel.layer.masksToBounds = YES; _topLabel.text = LocalizedStringFromKey(PublicTop); _topLabel.hidden = YES; UIView *line = [[UIView alloc]init]; line.backgroundColor = courseLineColor; [self.contentView addSubview: _userHeaderImage]; [self.contentView addSubview: _userTime]; [self.contentView addSubview: _userName]; [self.contentView addSubview: _userContent]; [self.contentView addSubview: _userComment]; [self.contentView addSubview: _replyImage]; [self.contentView addSubview: _essenceImage]; [self.contentView addSubview: _topLabel]; [self.contentView addSubview: line]; [_userHeaderImage makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(self.contentView.mas_top).offset(5); make.left.equalTo(self.contentView.mas_left).offset(5); make.width.equalTo(55); make.height.equalTo(55); }]; [_userName makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(self.contentView.mas_top).offset(5); make.left.equalTo(_userHeaderImage.mas_right).offset(5); make.height.equalTo(20); make.width.equalTo(100); }]; [_userTime makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(10); make.right.equalTo(self.contentView.mas_right).offset(-20); make.width.greaterThanOrEqualTo(45); make.height.equalTo(15); }]; [_topLabel makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(10); make.right.equalTo(_userTime.mas_left).offset(-5); make.width.equalTo(25); make.height.equalTo(15); }]; [_userContent makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(self.contentView.mas_top).offset(25); make.right.equalTo(self.contentView.mas_right).equalTo(-10); make.left.equalTo(_userHeaderImage.mas_right).offset(5); make.height.greaterThanOrEqualTo(30); }]; [_essenceImage makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(self.contentView.mas_top).offset(25); make.right.equalTo(self.contentView.mas_right).offset(-10); make.height.equalTo(35); make.width.equalTo(35); }]; [_userComment makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(_userContent.mas_bottom).offset(1); make.right.equalTo(_userContent.mas_right).offset(0); make.height.equalTo(13); make.width.greaterThanOrEqualTo(40); }]; [_replyImage makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(_userContent.mas_bottom).offset(0); make.right.equalTo(_userComment.mas_left).offset(-5); make.width.equalTo(15); make.height.equalTo(15); }]; [line makeConstraints:^(MASConstraintMaker *make) { make.left.equalTo(self.contentView.mas_left).offset(5); make.right.equalTo(self.contentView.mas_right).offset(-5); make.height.equalTo(1); make.bottom.equalTo(self.contentView.mas_bottom).offset(0); }]; _userHeaderImage.userInteractionEnabled = YES; UITapGestureRecognizer *headerImageTap = [[UITapGestureRecognizer alloc]initWithTarget: self action: @selector(headerImageTapAction)]; [_userHeaderImage addGestureRecognizer:headerImageTap]; } /** 头像点击事件,点击评论用户头像,跳转到用户个人信息页面 */ - (void)headerImageTapAction { [[jumpPush sharedInstance] pushToPersonInfoControllerWithUserID:_comment.user_id withUserName:_comment.user_name]; } - (void)setComment:(Comment *)comment { _comment = comment; _userTime.text = [[ConfigarationCase configaraTionInstance] timeFormatterWithTimeStr: _comment.create_time]; _userName.text = _comment.user_name; _userContent.text = _comment.content; NSString *imageUrl = _discuss.head_photo; UIImage *defaultImage = ImageNamed(@"common_icon_default_user_logo"); if ([imageUrl isKindOfClass:[NSNull class]] || imageUrl.length == 0 || [imageUrl hasSuffix:@"gif"]) { _userHeaderImage.image = defaultImage; } else { [_userHeaderImage sd_setImageWithURL:[NSURL URLWithString:imageUrl] placeholderImage:defaultImage]; } if ([_comment.stickie isEqualToString:@"1"]) _topLabel.hidden = NO; if ([comment.essence isEqualToString:@"1"]) _essenceImage.hidden = NO; NSString *creditstr = [[ConfigarationCase configaraTionInstance]getFilePeoplenum:_comment.reply_count]; creditstr = [NSString stringWithFormat:@"%@ %@",LocalizedStringFromKey(Pubicreplys),creditstr]; NSMutableAttributedString *creditString = [[NSMutableAttributedString alloc]initWithString:creditstr]; [creditString addAttribute: NSForegroundColorAttributeName value: ContentColor range: NSMakeRange(0, 4)]; [creditString addAttribute: NSForegroundColorAttributeName value: publicYellowColor range: NSMakeRange(4, creditstr.length -4)]; _userComment.attributedText = creditString; } + (CGFloat)heightForCellWithDiscuss:(Discuss *)discuss { NSString *commentStr = discuss.content; UIFont *font = [UIFont systemFontOfSize:14]; CGSize size = CGSizeMake(220.0f, CGFLOAT_MAX); // CGSize labelSize = [commentStr sizeWithFont:font constrainedToSize:size lineBreakMode:NSLineBreakByCharWrapping];//该方法已经被 ios7所弃用.使用下面的方法,具体参数请自行研究官方文档 CGSize labelSize = [commentStr boundingRectWithSize:size options:NSStringDrawingUsesLineFragmentOrigin attributes:nil context:commentStr]; return labelSize.height; } - (void)setHighlighted:(BOOL)highlighted animated:(BOOL)animated { [super setHighlighted:highlighted animated:animated]; if(highlighted){ self.backgroundColor = RGBA(237, 245, 255, 1); }else{ self.backgroundColor = [UIColor clearColor]; } } @end
实际展示的具体效果如下:
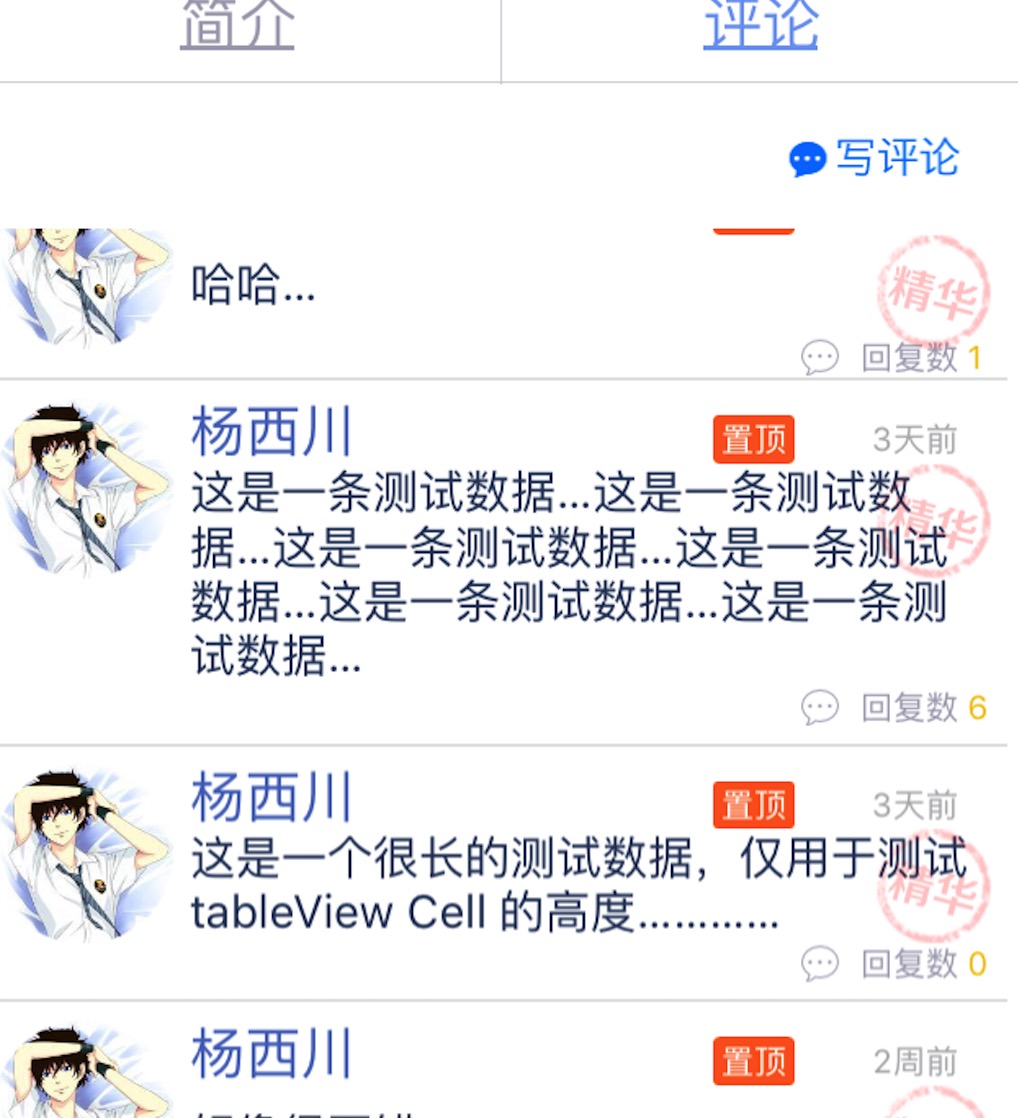
层级视图中的cell样式:
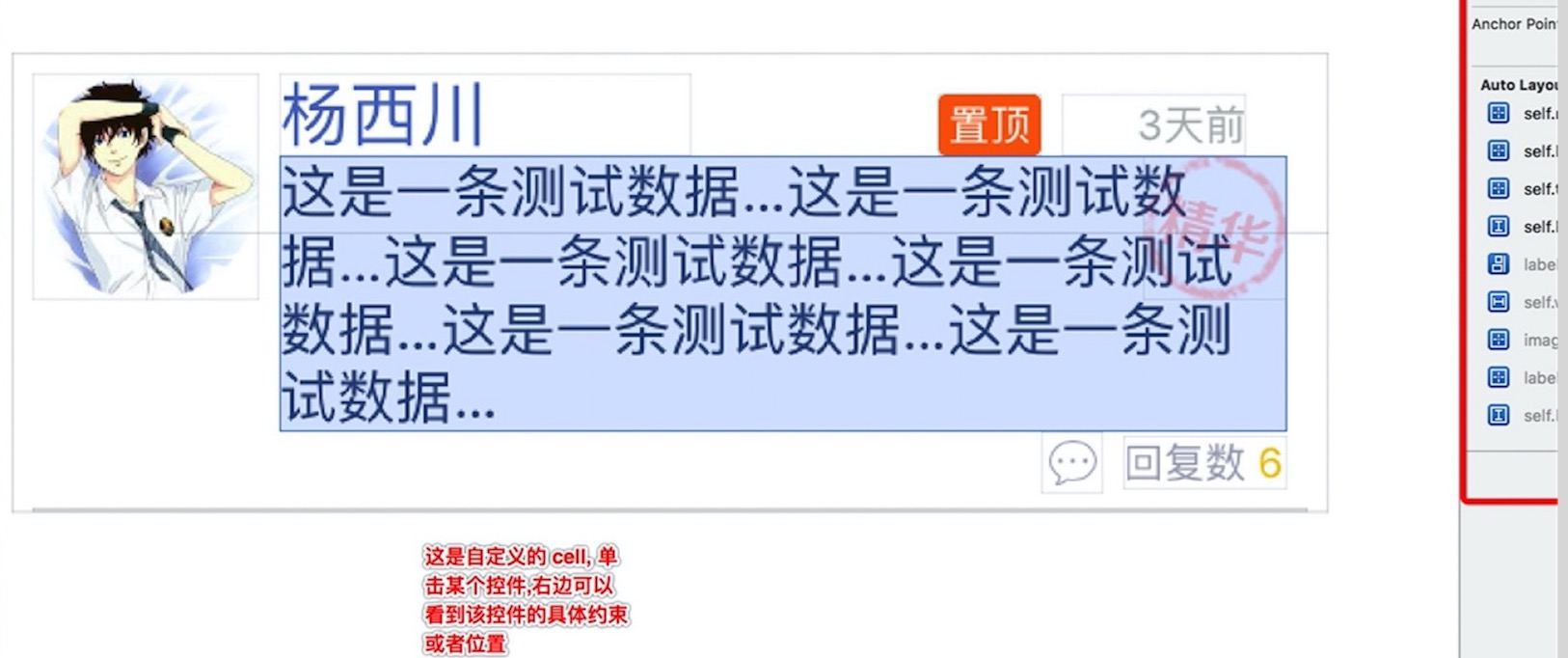
相关文章推荐
- iOS:tableView表头下拉放大的效果
- iOS之事件响应的简单介绍
- HDU 1017 A Mathematical Curiosity(枚举+mod)
- iOS 滑动返回功能
- 解决办法:ios模拟器键盘不弹出
- iOS使用代码截图
- iOS调用系统功能发邮件
- iOS tableViewCell自适应高度 第三发类库
- XCode-LibrarySearchPath和FramewrokSearchPath
- iOS开发 ☞ 数字格式化NSNumberFormatter
- iOS长按textView复制粘贴显示中文
- (array.count - 1 )的坑
- iOS个人整理43-第三方开发平台的使用--环信、ShareSDK和科大讯飞
- iOS个人整理42-FMDB
- iOS 复杂对象持久化 归档和反归档的过程
- iOS 沙盒机制
- iOS小技巧 - 隐藏LaunchScreen的状态栏
- iOS学习笔记-----内存管理初探
- iOS Simulator功能介绍关于Xamarin IOS开发
- 非常好用的iOS正则表达式