c# 局域网文件传输实例
2016-04-12 22:48
519 查看
一个基于c#的点对点局域网文件传输小案例,运行效果截图
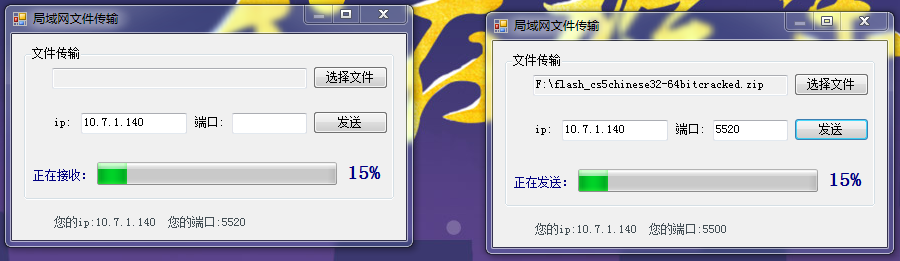
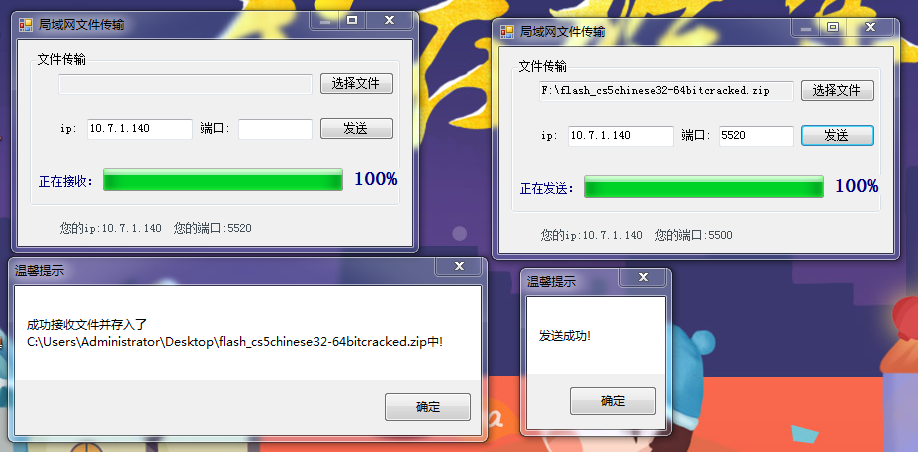
//界面窗体
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 文件传输
{
public partial class Form1 : Form
{
/// <summary>
/// 文件名
/// </summary>
private string fileName;
/// <summary>
/// 文件路径
/// </summary>
private string filePath;
/// <summary>
/// 文件大小
/// </summary>
private long fileSize;
public Form1()
{
InitializeComponent();
Thread.CurrentThread.IsBackground=true;
textBox2.Text = IpUtil.GetLocalIp();
label1.Text = "您的ip:" + IpUtil.GetLocalIp() + " 您的端口:" + IpUtil.GetRandomPort();
var s= new FileRecive(this);
new Thread(s.run).Start();
}
/// <summary>
/// 信息提示框
/// </summary>
/// <param name="msg"></param>
public void Tip(string msg) {
MessageBox.Show(msg,"温馨提示");
}
/// <summary>
/// 发送文件
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button2_Click(object sender, EventArgs e)
{
string ip = textBox2.Text;
string port =textBox3.Text;
if (fileName.Length == 0) {
Tip("请选择文件");
return;
}
if(ip.Length==0||port.ToString().Length==0){
Tip("端口和ip地址是必须的!");
return;
}
var c = new FileSend(this,new string[]{ip,port,fileName,filePath,fileSize.ToString()});
new Thread(c.Send).Start();
}
/// <summary>
/// 选择文件
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog dig = new OpenFileDialog();
dig.ShowDialog();
//获取文件名
this.fileName = dig.SafeFileName;
//获取文件路径
this.filePath = dig.FileName;
FileInfo f = new FileInfo(this.filePath);
//获取文件大小
this.fileSize = f.Length;
textBox1.Text = filePath;
}
/// <summary>
/// 更新进度条
/// </summary>
/// <param name="value"></param>
public void UpDateProgress(int value) {
this.progressBar1.Value=value;
this.label2.Text = value + "%";
System.Windows.Forms.Application.DoEvents();
}
/// <summary>
/// 修改状态
/// </summary>
/// <param name="state"></param>
public void SetState(string state) {
label5.Text = state;
}
/// <summary>
/// 退出程序
/// </summary>
public void Exit() {
Application.Exit();
}
}
}
//ip和端口工具类
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Text;
using System.Threading.Tasks;
namespace 文件传输
{
class IpUtil
{
/// <summary>
/// 获取本地ip地址
/// </summary>
/// <returns></returns>
public static string GetLocalIp()
{
string hostname = Dns.GetHostName();
IPHostEntry localhost = Dns.GetHostByName(hostname);
IPAddress localaddr = localhost.AddressList[0];
return localaddr.ToString();
}
/// <summary>
/// 产生随机端口
/// </summary>
/// <returns></returns>
public static int GetRandomPort() {
return new Random().Next(1000)+5000;
}
}
}
//文件发送类
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net.Sockets;
using System.Text;
using System.Threading.Tasks;
namespace 文件传输
{
//文件发送类
class FileSend
{
private TcpClient client;
private NetworkStream stream;
private string[] param;
private Form1 fm;
public FileSend(Form1 fm,params string[] param) {
this.param = param;
this.fm = fm;
}
public void Send()
{
try
{
//连接接收端
this.client = new TcpClient(param[0], int.Parse(param[1]));
string msg = param[2] + "|" + param[4];
byte[] m = Encoding.UTF8.GetBytes(msg);
while (true){
this.stream = this.client.GetStream();
this.stream.Write(m, 0, m.Length);
this.stream.Flush();
byte[] data = new byte[1024];
int len = this.stream.Read(data, 0, data.Length);
msg = Encoding.UTF8.GetString(data, 0, len);
//对方要接收我发送的文件
if (msg.Equals("1"))
{
fm.SetState("正在发送:");
FileStream os = new FileStream(param[3], FileMode.OpenOrCreate);
data = new byte[1024];
//记录当前发送进度
long currentprogress = 0;
len=0;
while ((len = os.Read(data, 0, data.Length)) > 0) {
currentprogress += len;
//更新进度条
fm.UpDateProgress((int)(currentprogress*100/long.Parse(param[4])));
this.stream.Write(data,0,len);
}
os.Flush();
this.stream.Flush();
os.Close();
this.stream.Close();
fm.Tip("发送成功!");
fm.Exit();
}
}
}
catch (Exception e)
{
fm.Tip(e.Message);
}
}
}
}
//文件接收类
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 文件传输
{
/// <summary>
/// 文件接收类
/// </summary>
class FileRecive
{
private TcpListener server;
private Form1 fm;
private NetworkStream stream;
public FileRecive(Form1 fm) {
this.fm = fm;
try
{
this.server = new TcpListener(IPAddress.Parse(IpUtil.GetLocalIp()), IpUtil.GetRandomPort());
server.Start();
}
catch (Exception e) {
fm.Tip(e.Message);
}
}
/// <summary>
/// 接收文件
/// </summary>
public void run()
{
while (true)
{
try
{
TcpClient client = server.AcceptTcpClient();
this.stream = client.GetStream();
byte[] msgs = new byte[1024];
int len = this.stream.Read(msgs, 0, msgs.Length);
string msg = Encoding.UTF8.GetString(msgs, 0, len);
string[] tip = msg.Split('|');
if (DialogResult.Yes == MessageBox.Show(IpUtil.GetLocalIp() + "给您发了一个文件:" + tip[0] + "大小为:" + (long.Parse(tip[1]) / 1024) + "kb ,确定要接收吗?", "接收提醒", MessageBoxButtons.YesNo))
{
//将接收信息反馈给发送方
msg = "1";
msgs = Encoding.UTF8.GetBytes(msg);
this.stream.Write(msgs, 0, msgs.Length);
this.stream.Flush();
fm.SetState("正在接收:");
//开始接收文件
string path = @"C:\Users\Administrator\Desktop\" + tip[0];//接收文件的存储路径
FileStream os = new FileStream(path, FileMode.OpenOrCreate);
byte[] data = new byte[1024];
long currentprogress = 0;
int length = 0;
while ((length = this.stream.Read(data, 0, data.Length)) > 0)
{
currentprogress += length;
//更新进度条
fm.UpDateProgress((int)(currentprogress * 100 / long.Parse(tip[1])));
os.Write(data, 0, length);
}
os.Flush();
this.stream.Flush();
os.Close();
this.stream.Close();
fm.Tip("成功接收文件并存入了" + path + "中!");
fm.Exit();
}
}
catch (Exception e)
{
fm.Tip(e.Message);
}
}
}
}
}
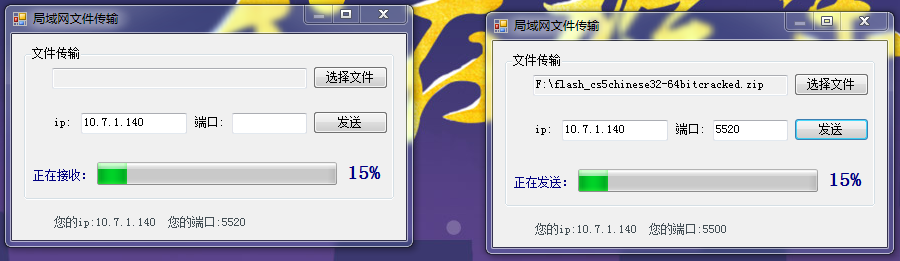
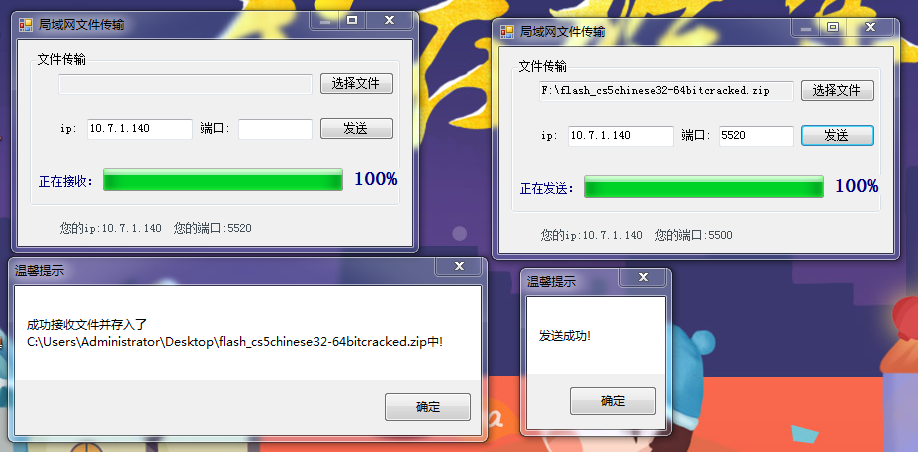
//界面窗体
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 文件传输
{
public partial class Form1 : Form
{
/// <summary>
/// 文件名
/// </summary>
private string fileName;
/// <summary>
/// 文件路径
/// </summary>
private string filePath;
/// <summary>
/// 文件大小
/// </summary>
private long fileSize;
public Form1()
{
InitializeComponent();
Thread.CurrentThread.IsBackground=true;
textBox2.Text = IpUtil.GetLocalIp();
label1.Text = "您的ip:" + IpUtil.GetLocalIp() + " 您的端口:" + IpUtil.GetRandomPort();
var s= new FileRecive(this);
new Thread(s.run).Start();
}
/// <summary>
/// 信息提示框
/// </summary>
/// <param name="msg"></param>
public void Tip(string msg) {
MessageBox.Show(msg,"温馨提示");
}
/// <summary>
/// 发送文件
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button2_Click(object sender, EventArgs e)
{
string ip = textBox2.Text;
string port =textBox3.Text;
if (fileName.Length == 0) {
Tip("请选择文件");
return;
}
if(ip.Length==0||port.ToString().Length==0){
Tip("端口和ip地址是必须的!");
return;
}
var c = new FileSend(this,new string[]{ip,port,fileName,filePath,fileSize.ToString()});
new Thread(c.Send).Start();
}
/// <summary>
/// 选择文件
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog dig = new OpenFileDialog();
dig.ShowDialog();
//获取文件名
this.fileName = dig.SafeFileName;
//获取文件路径
this.filePath = dig.FileName;
FileInfo f = new FileInfo(this.filePath);
//获取文件大小
this.fileSize = f.Length;
textBox1.Text = filePath;
}
/// <summary>
/// 更新进度条
/// </summary>
/// <param name="value"></param>
public void UpDateProgress(int value) {
this.progressBar1.Value=value;
this.label2.Text = value + "%";
System.Windows.Forms.Application.DoEvents();
}
/// <summary>
/// 修改状态
/// </summary>
/// <param name="state"></param>
public void SetState(string state) {
label5.Text = state;
}
/// <summary>
/// 退出程序
/// </summary>
public void Exit() {
Application.Exit();
}
}
}
//ip和端口工具类
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Text;
using System.Threading.Tasks;
namespace 文件传输
{
class IpUtil
{
/// <summary>
/// 获取本地ip地址
/// </summary>
/// <returns></returns>
public static string GetLocalIp()
{
string hostname = Dns.GetHostName();
IPHostEntry localhost = Dns.GetHostByName(hostname);
IPAddress localaddr = localhost.AddressList[0];
return localaddr.ToString();
}
/// <summary>
/// 产生随机端口
/// </summary>
/// <returns></returns>
public static int GetRandomPort() {
return new Random().Next(1000)+5000;
}
}
}
//文件发送类
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net.Sockets;
using System.Text;
using System.Threading.Tasks;
namespace 文件传输
{
//文件发送类
class FileSend
{
private TcpClient client;
private NetworkStream stream;
private string[] param;
private Form1 fm;
public FileSend(Form1 fm,params string[] param) {
this.param = param;
this.fm = fm;
}
public void Send()
{
try
{
//连接接收端
this.client = new TcpClient(param[0], int.Parse(param[1]));
string msg = param[2] + "|" + param[4];
byte[] m = Encoding.UTF8.GetBytes(msg);
while (true){
this.stream = this.client.GetStream();
this.stream.Write(m, 0, m.Length);
this.stream.Flush();
byte[] data = new byte[1024];
int len = this.stream.Read(data, 0, data.Length);
msg = Encoding.UTF8.GetString(data, 0, len);
//对方要接收我发送的文件
if (msg.Equals("1"))
{
fm.SetState("正在发送:");
FileStream os = new FileStream(param[3], FileMode.OpenOrCreate);
data = new byte[1024];
//记录当前发送进度
long currentprogress = 0;
len=0;
while ((len = os.Read(data, 0, data.Length)) > 0) {
currentprogress += len;
//更新进度条
fm.UpDateProgress((int)(currentprogress*100/long.Parse(param[4])));
this.stream.Write(data,0,len);
}
os.Flush();
this.stream.Flush();
os.Close();
this.stream.Close();
fm.Tip("发送成功!");
fm.Exit();
}
}
}
catch (Exception e)
{
fm.Tip(e.Message);
}
}
}
}
//文件接收类
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 文件传输
{
/// <summary>
/// 文件接收类
/// </summary>
class FileRecive
{
private TcpListener server;
private Form1 fm;
private NetworkStream stream;
public FileRecive(Form1 fm) {
this.fm = fm;
try
{
this.server = new TcpListener(IPAddress.Parse(IpUtil.GetLocalIp()), IpUtil.GetRandomPort());
server.Start();
}
catch (Exception e) {
fm.Tip(e.Message);
}
}
/// <summary>
/// 接收文件
/// </summary>
public void run()
{
while (true)
{
try
{
TcpClient client = server.AcceptTcpClient();
this.stream = client.GetStream();
byte[] msgs = new byte[1024];
int len = this.stream.Read(msgs, 0, msgs.Length);
string msg = Encoding.UTF8.GetString(msgs, 0, len);
string[] tip = msg.Split('|');
if (DialogResult.Yes == MessageBox.Show(IpUtil.GetLocalIp() + "给您发了一个文件:" + tip[0] + "大小为:" + (long.Parse(tip[1]) / 1024) + "kb ,确定要接收吗?", "接收提醒", MessageBoxButtons.YesNo))
{
//将接收信息反馈给发送方
msg = "1";
msgs = Encoding.UTF8.GetBytes(msg);
this.stream.Write(msgs, 0, msgs.Length);
this.stream.Flush();
fm.SetState("正在接收:");
//开始接收文件
string path = @"C:\Users\Administrator\Desktop\" + tip[0];//接收文件的存储路径
FileStream os = new FileStream(path, FileMode.OpenOrCreate);
byte[] data = new byte[1024];
long currentprogress = 0;
int length = 0;
while ((length = this.stream.Read(data, 0, data.Length)) > 0)
{
currentprogress += length;
//更新进度条
fm.UpDateProgress((int)(currentprogress * 100 / long.Parse(tip[1])));
os.Write(data, 0, length);
}
os.Flush();
this.stream.Flush();
os.Close();
this.stream.Close();
fm.Tip("成功接收文件并存入了" + path + "中!");
fm.Exit();
}
}
catch (Exception e)
{
fm.Tip(e.Message);
}
}
}
}
}
相关文章推荐
- 程序读取Excel,单元格内容超过255被截断解决方法
- C#基础(三)
- C#Winform限制TextBox文本框只能输入文本的格式
- C# directShow IAMStreamSelect切换音轨
- C#Winform得到指定的文件的位置
- C# 过滤SerialPort端口
- C#中的索引器 [ ]
- [转]C#调用FFMPEG,并异步读取输出信息的代码
- C# 递归算法与冒泡
- C# IndexOf、LastIndexOf、IndexOfAny,LastIndexOfAny
- Intel RealSense C# 入门
- c# 定时器的实现
- C#中的 int?是什么意思
- C# 多线程
- u3d 修改新的C#脚本模板-NewBehaviourScript.c
- 委托与事件总结
- C#基础概念 从新理解继承多态
- c#反射机制
- C#实现Excel动态生成PivotTable
- c#中DataTable和DataSet区别