C++ 中string类的三种模拟实现方式
2016-03-31 21:16
519 查看
1.原始版本(拷贝构造和赋值运算符重载时,需要重新开辟空间)
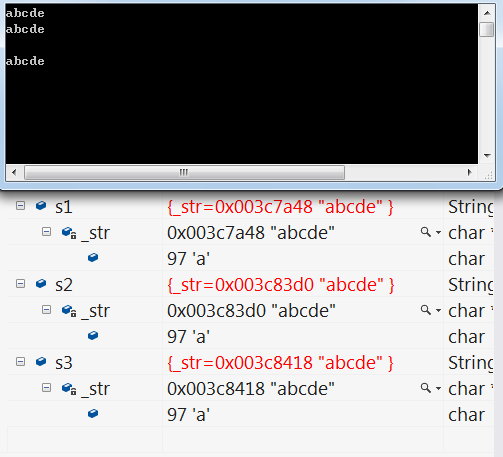
2.引用计数方式(拷贝构造时和赋值运算符重载时,只需对其引用计数器操作)
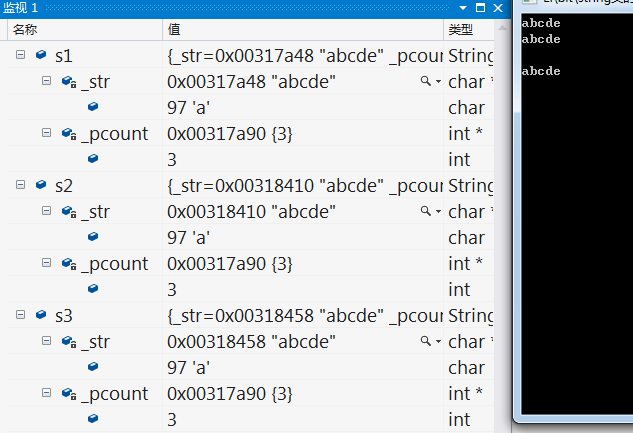
3.现代写法(拷贝构造和赋值运算符重载时,只进行指针的交换,没有开辟额外的空间,采用值传方式进行拷贝构造和赋值,最后节约了空间,只不过相对于前两种多调用了一次拷贝构函数,以时间换取空间)
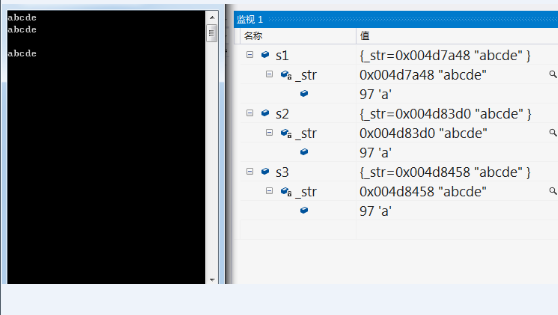
这就是三种模拟string类的实现方式。
#include <iostream> #include <string> using namespace std; class String { friend ostream& operator<<(ostream& os, const String& S); public: String(char* str = "") :_str(new char[strlen(str)+1]) { strcpy(_str, str); } String(const String& S) :_str(new char[strlen(S._str)+1]) { strcpy(_str, S._str); } String& operator=(const String& S) { if(_str != S._str) { delete[] _str; _str = new char[strlen(S._str)+1]; strcpy(_str, S._str); } return *this; } ~String() { if(_str != NULL) { delete[] _str; } } private: char* _str; }; ostream& operator<<(ostream& os, const String& S) { os<<S._str; return os; } int main() { String s1("abcde"); cout<<s1<<endl; String s2(s1); cout<<s2<<endl; String s3; cout<<s3<<endl; s3 = s2; cout<<s3<<endl; system("pause"); return 0; }测试结果:
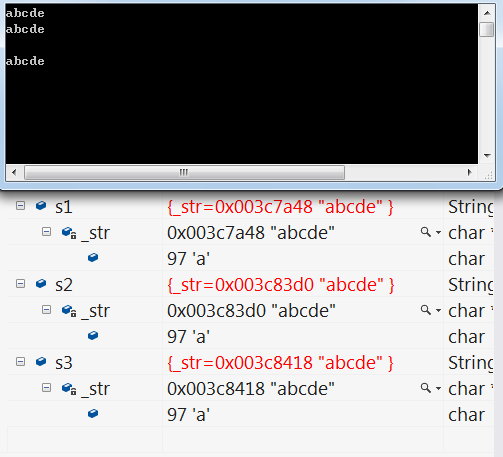
2.引用计数方式(拷贝构造时和赋值运算符重载时,只需对其引用计数器操作)
#include <iostream> #include <string> using namespace std; class String { friend ostream& operator<<(ostream& os, const String& S); public: String(char* str = "") :_str(new char[strlen(str)+1]),_pcount(new int(1)) { strcpy(_str, str); } String(const String& S) :_str(new char[strlen(S._str)+1]) ,_pcount(S._pcount) { strcpy(_str, S._str); ++(*_pcount); } String& operator=(const String& S) { if(_str != S._str) { delete[] _str; delete _pcount; _str = new char[strlen(S._str)+1]; _pcount = S._pcount; strcpy(_str, S._str); ++(*_pcount); } return *this; } ~String() { if(--(*_pcount) == 0) { delete[] _str; delete _pcount; } } private: char* _str; int* _pcount; }; ostream& operator<<(ostream& os, const String& S) { os<<S._str; return os; } int main() { String s1("abcde"); cout<<s1<<endl; String s2(s1); cout<<s2<<endl; String s3; cout<<s3<<endl; s3 = s2; cout<<s3<<endl; system("pause"); return 0; }测试结果:
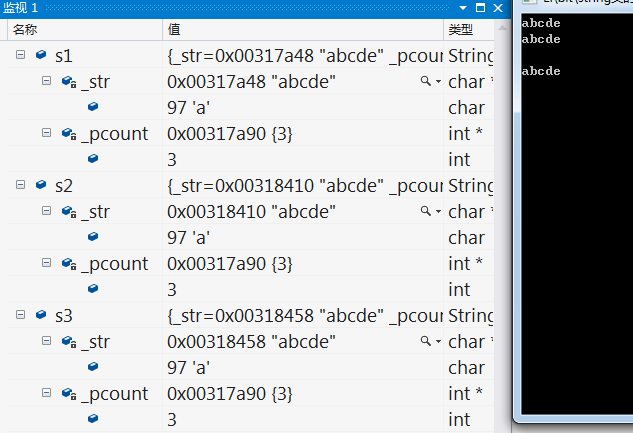
3.现代写法(拷贝构造和赋值运算符重载时,只进行指针的交换,没有开辟额外的空间,采用值传方式进行拷贝构造和赋值,最后节约了空间,只不过相对于前两种多调用了一次拷贝构函数,以时间换取空间)
#include <iostream> #include <string> using namespace std; class String { friend ostream& operator<<(ostream& os, const String& S); public: String(char *str = "") //构造函数 :_str(new char[strlen(str)+1]) { strcpy(_str, str); } String(const String& str) //拷贝构造函数 :_str(NULL) { String tmp(str._str); swap(_str, tmp._str); } String& operator=(String str) //赋值运算符重载 { swap(_str, str._str); return *this; } ~String() //析构函数 { if(_str != NULL) { delete[] _str; } } private: char* _str; }; ostream& operator<<(ostream& os, const String& S) { os<<S._str; return os; } int main() { String s1("abcde"); cout<<s1<<endl; String s2(s1); cout<<s2<<endl; String s3; cout<<s3<<endl; s3 = s2; cout<<s3<<endl; system("pause"); return 0; }测试结果:
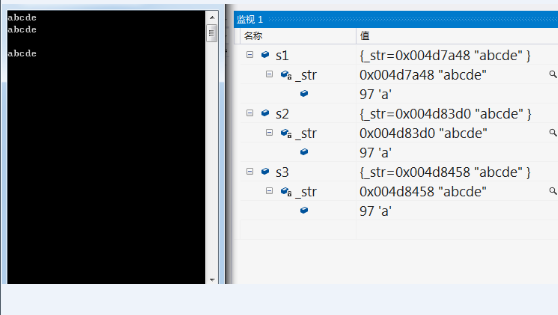
这就是三种模拟string类的实现方式。
相关文章推荐
- 空间两大拓展功能 引爆企业发展需求
- 空间支持多子网站 有效降低建站成本
- 奔月互联免费50m/5g/asp/ftp/可绑米空间
- 三维免费空间提供多款免费ASP空间(1G)
- Ruby中require、load、include、extend的区别介绍
- 空间页面CSS说明
- vbscript include的办法实现代码第1/2页
- 解析C++编程中的#include和条件编译
- PHP脚本中include文件出错解决方法
- 最省空间的计数器
- Flex include和import ActionScript代码
- set_include_path在win和linux下的区别
- php include加载文件两种方式效率比较
- How to Auto Include a Javascript File
- 浅谈ASP.NET的include的使用方法
- java/jsp中 中文问题详解
- php相对当前文件include其它文件的方法
- JSP计数器的制作
- php include和require的区别深入解析
- PHP include_path设置技巧分享