STS: Spring Boot and Hibernate
2016-03-20 12:42
579 查看
上篇文章 STS: Spring Boot and MyBatis 讲了使用 MyBatis 访问数据库的事儿,现在再来说说如何使用 Hibernate 访问数据库。
工具:Spring Tool Suite Version: 3.7.3.RELEASE
数据库:MySQL Example Database: world
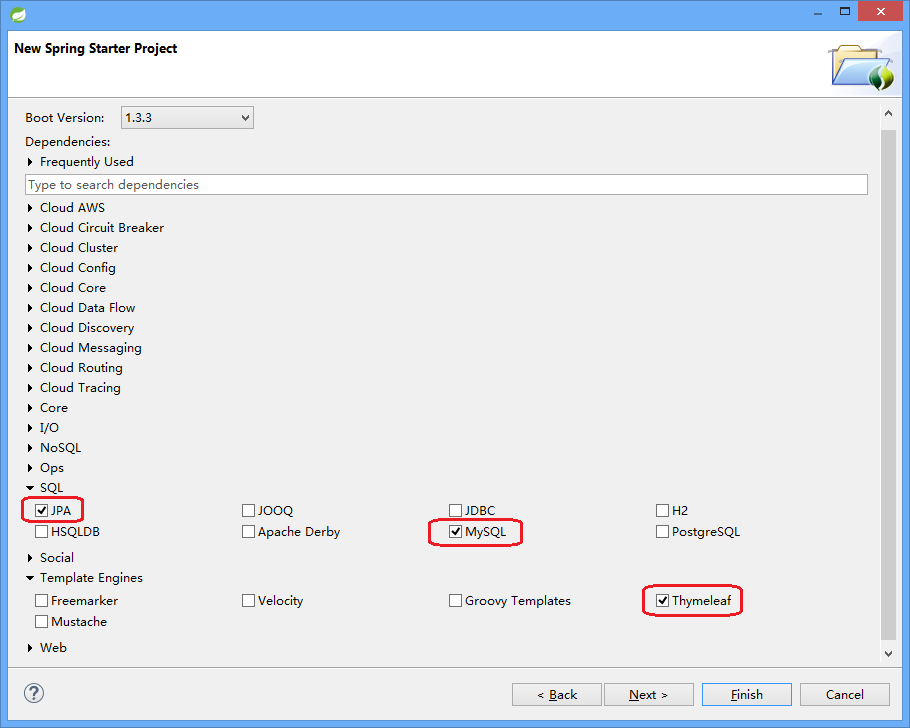
展开 src/main/java 节点,在 com.example 节点上点击鼠标右键,在弹出菜单上选择 New -> Folder 项,弹出 New Folder 对话框,Folder name 输入 domain,然后点击〖Finish〗按钮创建文件夹。
重复上面的步骤,依次创建 service 和 web 文件夹。完成后的项目结构如下图所示:
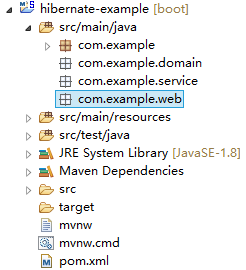
新创建的 Country.java 文件会自动打开,编辑文件内容如下:
新创建的 CountryDao.java 文件会自动打开,编辑文件内容如下:
新创建的 CountryService.java 文件会自动打开,编辑文件内容如下:
新创建的 CountryController.java 文件会自动打开,编辑文件内容如下:
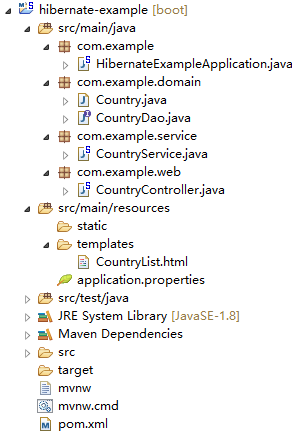
程序启动后,打开浏览器,在地址栏中输入 http://localhost:8080 即可查看运行结果。
运行结果如下图:
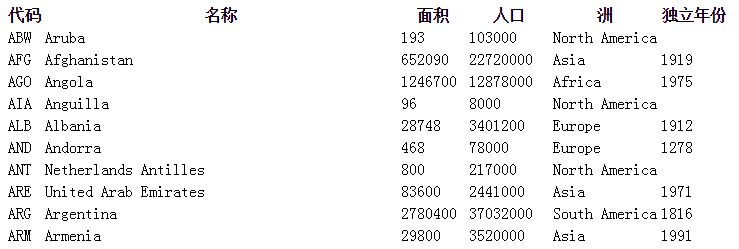
参考:Spring Boot:在Spring Boot中使用Mysql和JPA
工具:Spring Tool Suite Version: 3.7.3.RELEASE
数据库:MySQL Example Database: world
新建 Spring Starter Project
点击菜单 File -> New -> Spring Starter Project,打开 New Spring Starter Project 对话框,将 Name 改为 hibernate-example,然后点击〖Next >〗按钮,进入下一个对话框。展开 SQL 节点,选中 JPA 和 MySQL,再展开 Template Engines,选中 Thymeleaf。然后点击〖Finish〗按钮,创建 hibernate-example 项目。设置 DataSource 和 JPA 信息
展开 src/main/resources 节点,打开 application.properties 文件,输入如下内容:spring.datasource.driver-class-name=com.mysql.jdbc.Driver spring.datasource.url=jdbc:mysql://localhost:3306/world spring.datasource.username=root spring.datasource.password=xxxxx spring.jpa.database=mysql spring.jpa.show-sql=true spring.jpa.hibernate.ddl-auto=none spring.jpa.hibernate.naming-strategy=org.hibernate.cfg.DefaultNamingStrategy spring.jpa.properties.hibernate.dialect = org.hibernate.dialect.MySQL5Dialect
创建项目文件夹
参考 Spring 典型项目结构创建 domain、service 和 web 三个文件夹。展开 src/main/java 节点,在 com.example 节点上点击鼠标右键,在弹出菜单上选择 New -> Folder 项,弹出 New Folder 对话框,Folder name 输入 domain,然后点击〖Finish〗按钮创建文件夹。
重复上面的步骤,依次创建 service 和 web 文件夹。完成后的项目结构如下图所示:
创建实体模型
在 com.example.domain 节点上点击鼠标右键,在弹出菜单上选择 New -> Class 项,弹出 New Java Class 对话框,在 Name 栏输入 Country,然后点击〖Finish〗按钮创建 Country 类。新创建的 Country.java 文件会自动打开,编辑文件内容如下:
package com.example.domain; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.Table; import javax.validation.constraints.NotNull; import javax.validation.constraints.Null; @Entity @Table(name = "country") public class Country { @Id private String code; @NotNull private String name; @NotNull private String continent; @NotNull private String region; @NotNull private float surfaceArea; @Null private Integer indepYear; @NotNull private Integer population; public Country() { } public Country(String code) { this.code = code; } // Getter and setter methods public String getCode() { return code; } public void setCode(String code) { this.code = code; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getContinent() { return continent; } public void setContinent(String continent) { this.continent = continent; } public String getRegion() { return region; } public void setRegion(String region) { this.region = region; } public float getSurfaceArea() { return surfaceArea; } public void setSurfaceArea(float surfaceArea) { this.surfaceArea = surfaceArea; } public Integer getIndepYear() { return indepYear; } public void setIndepYear(Integer indepYear) { this.indepYear = indepYear; } public Integer getPopulation() { return population; } public void setPopulation(Integer population) { this.population = population; } }
创建数据访问对象(DAO)
在 com.example.domain 节点上点击鼠标右键,在弹出菜单上选择 New -> Interface 项,弹出 New Java Interface 对话框,在 Name 栏输入 CountryDao,然后点击〖Finish〗按钮创建 CountryDao 接口。新创建的 CountryDao.java 文件会自动打开,编辑文件内容如下:
package com.example.domain; import javax.transaction.Transactional; import org.springframework.data.repository.CrudRepository; import com.example.domain.Country; @Transactional public interface CountryDao extends CrudRepository<Country, String> { }
创建 Service
在 com.example.service 节点上点击鼠标右键,在弹出菜单上选择 New -> Class 项,弹出 New Java Class 对话框,在 Name 栏输入 CountryService,然后点击〖Finish〗按钮创建 CountryService 类。新创建的 CountryService.java 文件会自动打开,编辑文件内容如下:
package com.example.service; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import com.example.domain.*; @Service public class CountryService { @Autowired private CountryDao countryDao; public Iterable<Country> findAll() { return countryDao.findAll(); } }
创建 Controller
在 com.example.web 节点上点击鼠标右键,在弹出菜单上选择 New -> Class 项,弹出 New Java Class 对话框,在 Name 栏输入 CountryController,然后点击〖Finish〗按钮创建 CountryController 类。新创建的 CountryController.java 文件会自动打开,编辑文件内容如下:
package com.example.web; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import com.example.service.CountryService; @Controller public class CountryController { @Autowired private CountryService countryService; @RequestMapping("/") public String countryList(Model model) { model.addAttribute("countries", countryService.findAll() ); return "countryList"; } }
创建 Html 模板
在 src/main/resources 下面的 templates 文件夹内创建 CountryList.html 文件,编辑文件内容如下:<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>国家</title> </head> <body> <table> <thead> <tr> <th>代码</th> <th>名称</th> <th>面积</th> <th>人口</th> <th>洲</th> <th>独立年份</th> </tr> </thead> <tbody> <tr th:each="country : ${countries}"> <td th:text="${country.code}"></td> <td th:text="${country.name}"></td> <td th:text="${#numbers.formatDecimal(country.surfaceArea,1,0)}"></td> <td th:text="${country.population}"></td> <td th:text="${country.continent}"></td> <td th:text="${country.indepYear}"></td> </tr> </tbody> </table> </body> </html>
完成后的项目结构
经过上面这些步骤,我们已经完成了这个项目。最终的项目结构如下图所示:运行程序
在项目名称 hibernate-example 上点击鼠标右键,在弹出菜单上选择 Run As -> Spring Boot App。程序启动后,打开浏览器,在地址栏中输入 http://localhost:8080 即可查看运行结果。
运行结果如下图:
参考:Spring Boot:在Spring Boot中使用Mysql和JPA
相关文章推荐
- 一个jar包里的网站
- 一个jar包里的网站之文件上传
- 一个jar包里的网站之返回对媒体类型
- Spring整合Quartz(JobDetailBean方式)
- Spring整合Quartz(JobDetailBean方式)
- Hibernate Oracle sequence的使用技巧
- 模拟Spring的简单实现
- jsp Hibernate批量更新和批量删除处理代码
- jsp hibernate的分页代码第1/3页
- spring+html5实现安全传输随机数字密码键盘
- Spring中属性注入详解
- SpringMVC框架下JQuery传递并解析Json格式的数据是如何实现的
- Hibernate环境搭建与配置方法(Hello world配置文件版)
- JAVA+Hibernate 无限级分类
- SSH整合中 hibernate托管给Spring得到SessionFactory
- jsp hibernate 数据保存操作的原理
- struts2 spring整合fieldError问题
- hibernate中的增删改查实现代码
- 解决hibernate+mysql写入数据库乱码
- java优化hibernate性能的几点建议