Java 4X4棋盘游戏
2016-03-17 16:45
429 查看
分别用四种三个方块组成的图块填充棋盘,因而会空白一个,所以由用户指定一个棋盘方块,然后电脑计算出把剩余棋盘填充完。
黑色由用户指定:
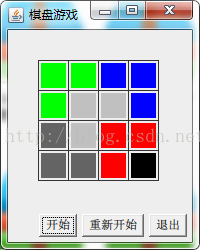
StarttMain.java代码:
package com.jz.start;
import javax.swing.UnsupportedLookAndFeelException;
import com.jz.algorithm.Algorithm;
import com.jz.ui.ChessJFrame;
/**
* 游戏开始
* @author Jayszxs
*
*/
public class StartMain {
public static void main(String[] args)
throws ClassNotFoundException, InstantiationException,
IllegalAccessException, UnsupportedLookAndFeelException {
ChessJFrame scj = new ChessJFrame();
scj.setVisible(true);
}
}
ChessBoard.java代码:
package com.jz.ui;
import java.awt.Color;
import java.awt.Cursor;
import java.awt.Graphics;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.awt.event.MouseMotionListener;
import java.io.Closeable;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
public class ChessBoard extends JPanel implements MouseListener{
public static final int MARGIN = 30; // 边距
public static final int GRID_SPAN = 30; // 网格间距
private final static int ROWS = 4; //行数
private final static int COLS = 4; //列数
Point[] chessList = new Point[(ROWS + 1) * (COLS + 1)]; // 初始每个数组元素为null
// XYDrawn[] pointDrawn = new XYDrawn[ROWS * COLS]; // 用来存储已经画的坐标
List<XYDrawn> xyDrawn = new ArrayList<XYDrawn>();
Color[] color = new Color[4];
int chessCount; // 当前棋盘的方块个数
int point;
public static boolean userStartPoint = false; //标记用户是否设置第一个方块
public ChessBoard() {
color[0] = new Color(255,0,0);
color[1] = new Color(0,255,0);
color[2] = new Color(0,0,255);
color[3] = new Color(100,100,100);
addMouseListener(this); //添加鼠标监听
addMouseMotionListener(new MouseMotionListener() { // 匿名内部类:设置光标移动时的图像
public void mouseDragged(MouseEvent e) {
}
public void mouseMoved(MouseEvent e) {
// 落在棋盘外,不能下
if (e.getX() < MARGIN || e.getX() > COLS * MARGIN + MARGIN ||
e.getY() < MARGIN || e.getY() > ROWS * MARGIN + MARGIN || userStartPoint) {
setCursor(new Cursor(Cursor.DEFAULT_CURSOR)); // 设置成默认形状
}else {
setCursor(new Cursor(Cursor.HAND_CURSOR)); // 设置成手型
}
}
});
}
// 1.开始绘制
public void paintComponent(Graphics g) {
super.paintComponent(g);
// 1.1绘制网格
for (int i = 0; i <= ROWS; i++) { // 画横线
g.drawLine(MARGIN, MARGIN + i * GRID_SPAN, MARGIN + COLS
* GRID_SPAN, MARGIN + i * GRID_SPAN);
}
for (int i = 0; i <= COLS; i++) {// 画直线
g.drawLine(MARGIN + i * GRID_SPAN, MARGIN, MARGIN + i * GRID_SPAN,
MARGIN + ROWS * GRID_SPAN);
}
// 1.2绘制方块
for (int i = 0; i < chessCount; i++) {
int xPos = chessList[i].getX() * GRID_SPAN + MARGIN; // 网格交叉点的x坐标
int yPos = chessList[i].getY() * GRID_SPAN + MARGIN;// 网格交叉点的y坐标
// System.out.println(xPos + " " + yPos);
// System.out.println(chessList[i].getX() + " " + chessList[i].getY());
g.setColor(chessList[i].getColor()); // 设置颜色
//绘制方块
g.fillRect(xPos - Point.DIAMETER / 2 + 15, yPos - Point.DIAMETER / 2 + 15,
Point.DIAMETER, Point.DIAMETER);
}
};
@Override
//覆盖鼠标按下方法
public void mousePressed(MouseEvent e) {
//判断用户是否设置第一个方块
if (userStartPoint) {
return;
}
int xIndex = (e.getX() - MARGIN) / GRID_SPAN; // 将鼠标点击的坐标位置转换成网格索引。
int yIndex = (e.getY() - MARGIN) / GRID_SPAN;
// System.out.println(xIndex + " " + yIndex);
// System.out.println(e.getX() + " " + e.getY());
// 落在棋盘外,不能下
if (e.getX() < MARGIN || e.getX() > COLS * MARGIN + MARGIN ||
e.getY() < MARGIN || e.getY() > ROWS * MARGIN + MARGIN) {
return;
}
Point ch = new Point(xIndex, yIndex, Color.black);
xyDrawn.add(new XYDrawn(xIndex, yIndex));
chessList[chessCount++] = ch;
repaint(); // 通知系统重新绘制
userStartPoint = true; //标记用户已经设置第一个方块
// System.out.println(ch.getX() + " " + ch.getY());
}
@Override
//覆盖鼠标点击方法
public void mouseClicked(MouseEvent e) {
}
@Override
//覆盖鼠标输入方法
public void mouseEntered(MouseEvent e) {
}
@Override
//覆盖鼠标退出方法
public void mouseExited(MouseEvent e) {
}
@Override
//覆盖鼠标释放方法
public void mouseReleased(MouseEvent e) {
}
public void BinarySearch(int x, int y, int col) {
if (!userStartPoint) {
return;
}
int x1, y1;
List<XYDrawn> xAndy = new ArrayList<XYDrawn>();
// 1
if ((x < 2 && x >= 0) && (y < 2 && y >= 0)) {
if (x == 0){
x1 = x + 1;
}else {
x1 = x - 1;
}
if (y == 0) {
y1 = y + 1;
} else {
y1 = y - 1;
}
xAndy.add(new XYDrawn(x1, y1));
xAndy.add(new XYDrawn(x1, y));
xAndy.add(new XYDrawn(x, y1));
// 2
}else if ((x < 4 && x >= 2) && (y < 2 && y >= 0)) {
if (x == 2){
x1 = x + 1;
}else {
x1 = x - 1;
}
if (y == 0) {
y1 = y + 1;
} else {
y1 = y - 1;
}
xAndy.add(new XYDrawn(x1, y1));
xAndy.add(new XYDrawn(x1, y));
xAndy.add(new XYDrawn(x, y1));
// 3
} else if ((x < 2 && x >= 0) && (y < 4 && y >= 2)) {
if (x == 0){
x1 = x + 1;
}else {
x1 = x - 1;
}
if (y == 2) {
y1 = y + 1;
} else {
y1 = y - 1;
}
xAndy.add(new XYDrawn(x1, y1));
xAndy.add(new XYDrawn(x1, y));
xAndy.add(new XYDrawn(x, y1));
// 4
} else {
if (x == 2){
x1 = x + 1;
}else {
x1 = x - 1;
}
if (y == 2) {
y1 = y + 1;
} else {
y1 = y - 1;
}
xAndy.add(new XYDrawn(x1, y1));
xAndy.add(new XYDrawn(x1, y));
xAndy.add(new XYDrawn(x, y1));
}
for (XYDrawn xy : xAndy) {
Point ch = new Point(xy.getX(), xy.getY(), color[col]);
//xyDrawn.add(xy);
chessList[chessCount++] = ch;
repaint(); // 通知系统重新绘制
}
}
public void BinarySearch2(int x, int y) {
if ((x < 2 && x >= 0) && (y < 2 && y >= 0)) {
Point ch = new Point(2, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 1));
ch = new Point(1, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 2));
ch = new Point(2, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 2));
repaint(); // 通知系统重新绘制
}else if ((x < 4 && x >= 2) && (y < 2 && y >= 0)) {
Point ch = new Point(1, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 1));
ch = new Point(1, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 2));
ch = new Point(2, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 2));
repaint(); // 通知系统重新绘制
} else if ((x < 2 && x >= 0) && (y < 4 && y >= 2)) {
Point ch = new Point(1, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 1));
ch = new Point(2, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 1));
ch = new Point(2, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 2));
repaint(); // 通知系统重新绘制
} else {
Point ch =
4000
new Point(1, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 1));
ch = new Point(2, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 1));
ch = new Point(1, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 2));
repaint(); // 通知系统重新绘制
}
}
public void restartGame() {
// 清除棋子
for (int i = 0; i < chessList.length; i++)
chessList[i] = null;
repaint();
chessCount = 0;
point = 0;
userStartPoint = false;
xyDrawn = new ArrayList<XYDrawn>();
}
public void goGame() {
int xIndex = chessList[0].getX();
int yIndex = chessList[0].getY();
BinarySearch2(xIndex, yIndex);
int i = 0;
for (XYDrawn xy : xyDrawn) {
BinarySearch(xy.getX(), xy.getY(), i);
if (i >= 3) {
return;
}
i++;
}
}
}
ChessJFrame.java代码:
package com.jz.ui;
import java.awt.BorderLayout;
import java.awt.Button;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.Action;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
/**
* 游戏框架类
* @author Jayszxs
*
*/
public class ChessJFrame extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
private ChessBoard chessBoard; //棋盘面板
private JPanel toolbar; //工具条面板
//开始、重新开始、退出按钮
// public static Button startButton = new Button("开始");
// public static Button restartButton = new Button("重新开始");
// public static Button exitButton = new Button("退出");
public static Button startButton;
public static Button restartButton;
public static Button exitButton;
/**
* 棋盘游戏框架初始化
* @throws UnsupportedLookAndFeelException
* @throws IllegalAccessException
* @throws InstantiationException
* @throws ClassNotFoundException
*/
public ChessJFrame()
throws ClassNotFoundException, InstantiationException,
IllegalAccessException, UnsupportedLookAndFeelException {
setBounds(800, 300, 200, 250); //设置框架的大小
setTitle("棋盘游戏"); //设置框架名称
//设置窗口的风格
// String lookAndFeel = "com.sun.java.swing.plaf.windows.WindowsLookAndFeel";
// UIManager.setLookAndFeel(lookAndFeel);
// 1.初始化工具条面板和棋盘面板
toolbar = new JPanel();
chessBoard = new ChessBoard();
// 2.初始化按钮
startButton = new Button("开始");
restartButton = new Button("重新开始");
exitButton = new Button("退出");
// 3.将工具条面板用FlowLayout布局
toolbar.setLayout(new FlowLayout(FlowLayout.RIGHT));
// 4.将按钮添加到工具条面板上
toolbar.add(startButton); //开始按钮
toolbar.add(restartButton); //重新开始按钮
toolbar.add(exitButton); //退出按钮
// 5.将工具条面板布局到面板的下方
add(toolbar,BorderLayout.SOUTH);
// 将棋盘添加到面板中
add(chessBoard);
// 6.设置界面默认关闭事件
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 7.自动识别大小
//pack();
// 8.将按钮添加到监听器
MyItemListener lis = new MyItemListener();
startButton.addActionListener(lis);
restartButton.addActionListener(lis);
exitButton.addActionListener(lis);
}
/**
* 事件监听器
* @author Jayszxs
*
*/
private class MyItemListener implements ActionListener{
@Override
/**
* 事件监听器类
*/
public void actionPerformed(ActionEvent e) {
// 1.取的事件源
Object obj = e.getSource();
// 2.判断取的事件是什么
if (obj == ChessJFrame.startButton) {
//取的“开始”按钮
chessBoard.goGame();
}else if (obj == ChessJFrame.restartButton) {
//取的“重新开始”按钮
chessBoard.restartGame();
}else if (obj == ChessJFrame.exitButton) {
//取的“退出”按钮
if (JOptionPane.showConfirmDialog(null, "是否退出?", "提示",
JOptionPane.YES_NO_OPTION) == 1) { //提示用户是否退出
return;
}
System.exit(0);
}
}
}
}
Point.java代码:
package com.jz.ui;
import java.awt.Color;
/**
* 方块的设计
* @author Jayszxs
*
*/
public class Point {
private int x; // 棋盘中的x索引
private int y; // 棋盘中的y索引
private Color color;//颜色
public static final int DIAMETER = 25;//直径
public Point(int x, int y, Color color) {
this.x = x;
this.y = y;
this.color = color;
}
public int getX() {// 拿到棋盘中的x索引
return x;
}
public int getY() {// 拿到棋盘中的Y索引
return y;
}
public Color getColor() {//得到颜色
return color;
}
}
XYDrawn.java代码:
package com.jz.ui;
public class XYDrawn {
private int x;
private int y;
public XYDrawn(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
}
黑色由用户指定:
StarttMain.java代码:
package com.jz.start;
import javax.swing.UnsupportedLookAndFeelException;
import com.jz.algorithm.Algorithm;
import com.jz.ui.ChessJFrame;
/**
* 游戏开始
* @author Jayszxs
*
*/
public class StartMain {
public static void main(String[] args)
throws ClassNotFoundException, InstantiationException,
IllegalAccessException, UnsupportedLookAndFeelException {
ChessJFrame scj = new ChessJFrame();
scj.setVisible(true);
}
}
ChessBoard.java代码:
package com.jz.ui;
import java.awt.Color;
import java.awt.Cursor;
import java.awt.Graphics;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.awt.event.MouseMotionListener;
import java.io.Closeable;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
public class ChessBoard extends JPanel implements MouseListener{
public static final int MARGIN = 30; // 边距
public static final int GRID_SPAN = 30; // 网格间距
private final static int ROWS = 4; //行数
private final static int COLS = 4; //列数
Point[] chessList = new Point[(ROWS + 1) * (COLS + 1)]; // 初始每个数组元素为null
// XYDrawn[] pointDrawn = new XYDrawn[ROWS * COLS]; // 用来存储已经画的坐标
List<XYDrawn> xyDrawn = new ArrayList<XYDrawn>();
Color[] color = new Color[4];
int chessCount; // 当前棋盘的方块个数
int point;
public static boolean userStartPoint = false; //标记用户是否设置第一个方块
public ChessBoard() {
color[0] = new Color(255,0,0);
color[1] = new Color(0,255,0);
color[2] = new Color(0,0,255);
color[3] = new Color(100,100,100);
addMouseListener(this); //添加鼠标监听
addMouseMotionListener(new MouseMotionListener() { // 匿名内部类:设置光标移动时的图像
public void mouseDragged(MouseEvent e) {
}
public void mouseMoved(MouseEvent e) {
// 落在棋盘外,不能下
if (e.getX() < MARGIN || e.getX() > COLS * MARGIN + MARGIN ||
e.getY() < MARGIN || e.getY() > ROWS * MARGIN + MARGIN || userStartPoint) {
setCursor(new Cursor(Cursor.DEFAULT_CURSOR)); // 设置成默认形状
}else {
setCursor(new Cursor(Cursor.HAND_CURSOR)); // 设置成手型
}
}
});
}
// 1.开始绘制
public void paintComponent(Graphics g) {
super.paintComponent(g);
// 1.1绘制网格
for (int i = 0; i <= ROWS; i++) { // 画横线
g.drawLine(MARGIN, MARGIN + i * GRID_SPAN, MARGIN + COLS
* GRID_SPAN, MARGIN + i * GRID_SPAN);
}
for (int i = 0; i <= COLS; i++) {// 画直线
g.drawLine(MARGIN + i * GRID_SPAN, MARGIN, MARGIN + i * GRID_SPAN,
MARGIN + ROWS * GRID_SPAN);
}
// 1.2绘制方块
for (int i = 0; i < chessCount; i++) {
int xPos = chessList[i].getX() * GRID_SPAN + MARGIN; // 网格交叉点的x坐标
int yPos = chessList[i].getY() * GRID_SPAN + MARGIN;// 网格交叉点的y坐标
// System.out.println(xPos + " " + yPos);
// System.out.println(chessList[i].getX() + " " + chessList[i].getY());
g.setColor(chessList[i].getColor()); // 设置颜色
//绘制方块
g.fillRect(xPos - Point.DIAMETER / 2 + 15, yPos - Point.DIAMETER / 2 + 15,
Point.DIAMETER, Point.DIAMETER);
}
};
@Override
//覆盖鼠标按下方法
public void mousePressed(MouseEvent e) {
//判断用户是否设置第一个方块
if (userStartPoint) {
return;
}
int xIndex = (e.getX() - MARGIN) / GRID_SPAN; // 将鼠标点击的坐标位置转换成网格索引。
int yIndex = (e.getY() - MARGIN) / GRID_SPAN;
// System.out.println(xIndex + " " + yIndex);
// System.out.println(e.getX() + " " + e.getY());
// 落在棋盘外,不能下
if (e.getX() < MARGIN || e.getX() > COLS * MARGIN + MARGIN ||
e.getY() < MARGIN || e.getY() > ROWS * MARGIN + MARGIN) {
return;
}
Point ch = new Point(xIndex, yIndex, Color.black);
xyDrawn.add(new XYDrawn(xIndex, yIndex));
chessList[chessCount++] = ch;
repaint(); // 通知系统重新绘制
userStartPoint = true; //标记用户已经设置第一个方块
// System.out.println(ch.getX() + " " + ch.getY());
}
@Override
//覆盖鼠标点击方法
public void mouseClicked(MouseEvent e) {
}
@Override
//覆盖鼠标输入方法
public void mouseEntered(MouseEvent e) {
}
@Override
//覆盖鼠标退出方法
public void mouseExited(MouseEvent e) {
}
@Override
//覆盖鼠标释放方法
public void mouseReleased(MouseEvent e) {
}
public void BinarySearch(int x, int y, int col) {
if (!userStartPoint) {
return;
}
int x1, y1;
List<XYDrawn> xAndy = new ArrayList<XYDrawn>();
// 1
if ((x < 2 && x >= 0) && (y < 2 && y >= 0)) {
if (x == 0){
x1 = x + 1;
}else {
x1 = x - 1;
}
if (y == 0) {
y1 = y + 1;
} else {
y1 = y - 1;
}
xAndy.add(new XYDrawn(x1, y1));
xAndy.add(new XYDrawn(x1, y));
xAndy.add(new XYDrawn(x, y1));
// 2
}else if ((x < 4 && x >= 2) && (y < 2 && y >= 0)) {
if (x == 2){
x1 = x + 1;
}else {
x1 = x - 1;
}
if (y == 0) {
y1 = y + 1;
} else {
y1 = y - 1;
}
xAndy.add(new XYDrawn(x1, y1));
xAndy.add(new XYDrawn(x1, y));
xAndy.add(new XYDrawn(x, y1));
// 3
} else if ((x < 2 && x >= 0) && (y < 4 && y >= 2)) {
if (x == 0){
x1 = x + 1;
}else {
x1 = x - 1;
}
if (y == 2) {
y1 = y + 1;
} else {
y1 = y - 1;
}
xAndy.add(new XYDrawn(x1, y1));
xAndy.add(new XYDrawn(x1, y));
xAndy.add(new XYDrawn(x, y1));
// 4
} else {
if (x == 2){
x1 = x + 1;
}else {
x1 = x - 1;
}
if (y == 2) {
y1 = y + 1;
} else {
y1 = y - 1;
}
xAndy.add(new XYDrawn(x1, y1));
xAndy.add(new XYDrawn(x1, y));
xAndy.add(new XYDrawn(x, y1));
}
for (XYDrawn xy : xAndy) {
Point ch = new Point(xy.getX(), xy.getY(), color[col]);
//xyDrawn.add(xy);
chessList[chessCount++] = ch;
repaint(); // 通知系统重新绘制
}
}
public void BinarySearch2(int x, int y) {
if ((x < 2 && x >= 0) && (y < 2 && y >= 0)) {
Point ch = new Point(2, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 1));
ch = new Point(1, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 2));
ch = new Point(2, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 2));
repaint(); // 通知系统重新绘制
}else if ((x < 4 && x >= 2) && (y < 2 && y >= 0)) {
Point ch = new Point(1, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 1));
ch = new Point(1, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 2));
ch = new Point(2, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 2));
repaint(); // 通知系统重新绘制
} else if ((x < 2 && x >= 0) && (y < 4 && y >= 2)) {
Point ch = new Point(1, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 1));
ch = new Point(2, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 1));
ch = new Point(2, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 2));
repaint(); // 通知系统重新绘制
} else {
Point ch =
4000
new Point(1, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 1));
ch = new Point(2, 1, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(2, 1));
ch = new Point(1, 2, Color.lightGray);
chessList[chessCount++] = ch;
xyDrawn.add(new XYDrawn(1, 2));
repaint(); // 通知系统重新绘制
}
}
public void restartGame() {
// 清除棋子
for (int i = 0; i < chessList.length; i++)
chessList[i] = null;
repaint();
chessCount = 0;
point = 0;
userStartPoint = false;
xyDrawn = new ArrayList<XYDrawn>();
}
public void goGame() {
int xIndex = chessList[0].getX();
int yIndex = chessList[0].getY();
BinarySearch2(xIndex, yIndex);
int i = 0;
for (XYDrawn xy : xyDrawn) {
BinarySearch(xy.getX(), xy.getY(), i);
if (i >= 3) {
return;
}
i++;
}
}
}
ChessJFrame.java代码:
package com.jz.ui;
import java.awt.BorderLayout;
import java.awt.Button;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.Action;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
/**
* 游戏框架类
* @author Jayszxs
*
*/
public class ChessJFrame extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
private ChessBoard chessBoard; //棋盘面板
private JPanel toolbar; //工具条面板
//开始、重新开始、退出按钮
// public static Button startButton = new Button("开始");
// public static Button restartButton = new Button("重新开始");
// public static Button exitButton = new Button("退出");
public static Button startButton;
public static Button restartButton;
public static Button exitButton;
/**
* 棋盘游戏框架初始化
* @throws UnsupportedLookAndFeelException
* @throws IllegalAccessException
* @throws InstantiationException
* @throws ClassNotFoundException
*/
public ChessJFrame()
throws ClassNotFoundException, InstantiationException,
IllegalAccessException, UnsupportedLookAndFeelException {
setBounds(800, 300, 200, 250); //设置框架的大小
setTitle("棋盘游戏"); //设置框架名称
//设置窗口的风格
// String lookAndFeel = "com.sun.java.swing.plaf.windows.WindowsLookAndFeel";
// UIManager.setLookAndFeel(lookAndFeel);
// 1.初始化工具条面板和棋盘面板
toolbar = new JPanel();
chessBoard = new ChessBoard();
// 2.初始化按钮
startButton = new Button("开始");
restartButton = new Button("重新开始");
exitButton = new Button("退出");
// 3.将工具条面板用FlowLayout布局
toolbar.setLayout(new FlowLayout(FlowLayout.RIGHT));
// 4.将按钮添加到工具条面板上
toolbar.add(startButton); //开始按钮
toolbar.add(restartButton); //重新开始按钮
toolbar.add(exitButton); //退出按钮
// 5.将工具条面板布局到面板的下方
add(toolbar,BorderLayout.SOUTH);
// 将棋盘添加到面板中
add(chessBoard);
// 6.设置界面默认关闭事件
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 7.自动识别大小
//pack();
// 8.将按钮添加到监听器
MyItemListener lis = new MyItemListener();
startButton.addActionListener(lis);
restartButton.addActionListener(lis);
exitButton.addActionListener(lis);
}
/**
* 事件监听器
* @author Jayszxs
*
*/
private class MyItemListener implements ActionListener{
@Override
/**
* 事件监听器类
*/
public void actionPerformed(ActionEvent e) {
// 1.取的事件源
Object obj = e.getSource();
// 2.判断取的事件是什么
if (obj == ChessJFrame.startButton) {
//取的“开始”按钮
chessBoard.goGame();
}else if (obj == ChessJFrame.restartButton) {
//取的“重新开始”按钮
chessBoard.restartGame();
}else if (obj == ChessJFrame.exitButton) {
//取的“退出”按钮
if (JOptionPane.showConfirmDialog(null, "是否退出?", "提示",
JOptionPane.YES_NO_OPTION) == 1) { //提示用户是否退出
return;
}
System.exit(0);
}
}
}
}
Point.java代码:
package com.jz.ui;
import java.awt.Color;
/**
* 方块的设计
* @author Jayszxs
*
*/
public class Point {
private int x; // 棋盘中的x索引
private int y; // 棋盘中的y索引
private Color color;//颜色
public static final int DIAMETER = 25;//直径
public Point(int x, int y, Color color) {
this.x = x;
this.y = y;
this.color = color;
}
public int getX() {// 拿到棋盘中的x索引
return x;
}
public int getY() {// 拿到棋盘中的Y索引
return y;
}
public Color getColor() {//得到颜色
return color;
}
}
XYDrawn.java代码:
package com.jz.ui;
public class XYDrawn {
private int x;
private int y;
public XYDrawn(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
}
相关文章推荐
- java对世界各个时区(TimeZone)的通用转换处理方法(转载)
- java-注解annotation
- java-模拟tomcat服务器
- java-用HttpURLConnection发送Http请求.
- java-WEB中的监听器Lisener
- Android IPC进程间通讯机制
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- 介绍一款信息管理系统的开源框架---jeecg
- 聚类算法之kmeans算法java版本
- java实现 PageRank算法
- PropertyChangeListener简单理解
- c++11 + SDL2 + ffmpeg +OpenAL + java = Android播放器
- 插入排序
- 冒泡排序
- 堆排序
- 快速排序
- 二叉查找树