java 模仿原版坦克大战
2016-03-11 17:01
417 查看
2015年的年底,花了四天的时间写了个坦克大战小游戏,界面和原版非常像,但是功能还略显不足。不多说了,直接上代码吧!
1:第一个类,窗体类。
package tankwar.copy;
import java.applet.Applet;
import java.applet.AudioClip;
import java.io.File;
import javax.swing.JFrame;
/**
* 1:创建窗体 2:坦克添加 3:障碍物添加与配置 4:坦克移动 5:发射子弹 6:碰撞检测等
*
* @author liurenyou
*
*/
public class TankWar extends JFrame {
public void initFrame() throws Exception {
this.setTitle("坦克大战");
this.setSize(1120, 690);
this.setLocationRelativeTo(null);
this.setResizable(false);
this.setDefaultCloseOperation(3);
GamePanel gp = new GamePanel();
this.add(gp);
this.setFocusable(false);
gp.setFocusable(true);
this.setVisible(true);
try {
File f = new File("images/start.wav");
AudioClip p = Applet.newAudioClip(f.toURI().toURL());
p.play();
} catch (Exception e) {
}
PlayMusic.playMusic("images/move.wav");
// PlayMusic.playMusic("images/life.wav");
// PlayMusic.playMusic("images/Nowin.wav");
// PlayMusic.playMusic("images/startHit.wav");
// PlayMusic.playMusic("images/bomb.wav");
new Thread() {
public void run() {
while (true) {
gp.repaint();
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
};
}.start();
}
}
2:第二个类,面板类,由于窗体太复杂,所以把面板添加到窗体上,通过控制面板来控制游戏。
package tankwar.copy;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import java.awt.image.BufferedImage;
import java.util.ArrayList;
import javax.swing.ImageIcon;
import javax.swing.JPanel;
public class GamePanel extends JPanel {
private Tank tank;
private Bullet bullet;
private EnemyTank enemyTank;
private EnemyBullet enemyBullet;
private Crafic crafic;
private ImageIcon icon = new ImageIcon("images/bird.png");
private ImageIcon icon1 = new ImageIcon("images/you.png");
public Image icon2;
public int[][] map;
public static ArrayList<EnemyTank> enemyTankList = new ArrayList<EnemyTank>();// 敌方坦克容器
public static ArrayList<Bullet> bulletList = new ArrayList<Bullet>();// 子弹容器
public static ArrayList<Crafic> craficList = new ArrayList<Crafic>();// 障碍物容器
public static ArrayList<EnemyBullet> enemyBulletList = new ArrayList<EnemyBullet>();// 敌方子弹容器
public GamePanel() {
tank = new Tank(this);// 我方坦克对象
initPanel();
tank.start();
// 创建敌方坦克对象
for (int i = 0; i < 3; i++) {
enemyTank = new EnemyTank(this);
enemyTank.start();
enemyTank.x += i * 400;
enemyTankList.add(enemyTank);
}
}
public Tank gettank() {// 获取Tank对象tank
return tank;
}
public EnemyTank getEnemyTank() {// 获取EnemyTank对象enemyTank
return enemyTank;
}
{// 代码块
map = ReadMap.readMap();
for (int i = 0; i < map.length; i++) {
for (int j = 0; j < map[i].length; j++) {
if (map[i][j] == 0) {
continue;
}
int x = j * 28;
int y = i * 23;
crafic = new Crafic(x, y, 28, 23);
if (map[i][j] == 1) {
crafic.image = new ImageIcon("images/brick.png");
} else if (map[i][j] == 2) {
crafic.image = new ImageIcon("images/stone.jpg");
}
craficList.add(crafic);
}
}
}
public void initPanel() {
KeyAdapter keyListener = new KeyAdapter() {
public void keyPressed(KeyEvent e) {
int code = e.getKeyCode();
if (code == 87) {// 向上
tank.deraction = 0;
} else if (code == 83) {// 向下
tank.deraction = 1;
} else if (code == 65) {// 向左
tank.deraction = 2;
} else if (code == 68) {// 向右
tank.deraction = 3;
} else if (code == 32) {// 空格
} else if (code == 74) {// j键发射子弹
tank.gun = true;
}
}
public void keyReleased(KeyEvent e) {
int code = e.getKeyCode();
if (code == 87) {// 向上
tank.deraction = 4;
} else if (code == 83) {// 向下
tank.deraction = 5;
} else if (code == 65) {// 向左
tank.deraction = 6;
} else if (code == 68) {// 向右
tank.deraction = 7;
} else if (code == 74) {// j
// tank.gun = false;
} else if (code == 32) {// 空格
}
}
};
this.addKeyListener(keyListener);
}
public void paint(Graphics g) {
BufferedImage bi = (BufferedImage) this.createImage(this.getWidth(), this.getHeight());
Graphics graphics = bi.getGraphics();
graphics.setColor(Color.BLACK);
graphics.fillRect(0, 0, 1365, 686);
for (int i = 0; i < craficList.size(); i++) {// 画障碍物
crafic = craficList.get(i);
graphics.drawImage(crafic.image.getImage(), crafic.x, crafic.y, null);
}
graphics.drawImage(icon.getImage(), 421, 620, null);// 画老鹰
graphics.drawImage(icon1.getImage(), 895, 0, null);// 画提示信息
for (int i = 0; i < enemyTankList.size(); i++) {
EnemyTank enemyTank = enemyTankList.get(i);
graphics.drawImage(enemyTank.image, enemyTank.x, enemyTank.y, null);// 画敌方坦克
}
graphics.drawImage(tank.getImage(), tank.getX(), tank.getY(), null);// 画坦克
for (int i = 0; i < bulletList.size(); i++) {
bullet = bulletList.get(i);
graphics.drawImage(bullet.getImage(), bullet.x, bullet.y, null);// 画我方子弹
}
for (int i = 0; i < enemyBulletList.size(); i++) {
enemyBullet = enemyBulletList.get(i);
graphics.drawImage(enemyBullet.image, enemyBullet.x, enemyBullet.y, null);// 画敌方子弹
}
g.drawImage(bi, 0, 0, null);
}
}
有窗体和面板了,就得往面板上添加一些元素,障碍物,坦克等等
3:第三个类,障碍物类
package tankwar.copy;
import javax.swing.ImageIcon;
/**
* 障碍物
*
* @author Administrator
*
*/
public class Crafic {
// 障碍物的xy坐标
public int x, y;
// 宽高度
public int width, height;
public ImageIcon image;
public Crafic(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
}
4:第四个类,读地图类。//我的障碍物是这样定义的,0代表砖块,1代表铁块,等等,所以我的地图是通过一个文件来储存的,里面是01串,然后通过这个类来读地图
package tankwar.copy;
import java.io.FileInputStream;
public class ReadMap {
/**
* 工具方法
*
* @return
*/
public static int[][] readMap() {
int[][] map = null;
try {
FileInputStream fis = new FileInputStream("images/image1");
// 先定义一个字节数组
byte[] bytes = new byte[fis.available()];
fis.read(bytes);
// 编程字符串
String str = new String(bytes).trim();
// 总行数
int row = 0;
int column = 0;
// 总行数
String[] strs = str.split("\r\n");
row = strs.length;
// 确定列数
if (strs != null && strs.length >= 1) {
String[] allColumn = strs[0].split(",");
column = allColumn.length;
}
map = new int[row][column];
// 存数据
for (int i = 0; i < strs.length; i++) {
String value = strs[i];
String[] vs = value.split(",");
for (int j = 0; j < vs.length; j++) {
String v = vs[j];
map[i][j] = Integer.parseInt(v);
}
}
} catch (Exception e) {
e.printStackTrace();
}
return map;
}
}
5:第五个类:我方坦克类
package tankwar.copy;
import java.awt.Image;
import java.awt.Rectangle;
import javax.swing.ImageIcon;
public class Tank extends Thread {
public boolean up = false, down = false, left = false, right = false;// 坦克方向
public int deraction = 4;// 定义坦克方向 0是上 1是下 2左 3是右 默认是上
public Crafic crafic;
public int speed = 1;// 坦克初始速度
public boolean gun = false;// 初始子弹默认不开
public int x = 284, y = 614;// 坦克初始坐标
public int width = 55, height = 35;// 坦克宽高
private Image image = new ImageIcon("images/up1.png").getImage();// 第一张坦克图片
private GamePanel gp;
public Tank(GamePanel gp) {// 构造函数
this.gp = gp;
new Thread() {
public void run() {
while (true) {
if (gun) {// 如果发射了子弹才添加
Bullet bullet = new Bullet(gp, x, y, deraction);
bullet.start();
System.out.println(GamePanel.bulletList.size());
GamePanel.bulletList.add(bullet);
}
try {
Thread.sleep(300);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
};
}.start();
}
public void run() {
while (true) {
if (deraction == 0) {// 向上
image = new ImageIcon("images/up1.png").getImage();
deraction = 0;
Rectangle tankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (tankRect.intersects(craficRect)) {// 碰撞到了
if (tankRect.y + tankRect.height - craficRect.y != 1
&& tankRect.x + tankRect.width - craficRect.x != 1
&& craficRect.x + craficRect.width - tankRect.x != 1) {
speed = 0;
break;
}
}
}
if (y > 0 && y <= 646) {
y -= speed;
}
speed = 1;
}
if (deraction == 1) {// 向下
deraction = 1;
image = new ImageIcon("images/down1.png").getImage();
Rectangle tankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (tankRect.intersects(craficRect)) {// 碰撞到了
if (craficRect.y + craficRect.height - tankRect.y != 1
&& tankRect.x + tankRect.width - craficRect.x != 1
&& craficRect.x + craficRect.width - tankRect.x != 1) {
speed = 0;
break;
}
}
}
if (y >= 0 && y <= 625) {
y += speed;
}
speed = 1;
}
if (deraction == 2) {// 向左
deraction = 2;
image = new ImageIcon("images/left1.png").getImage();
Rectangle tankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (tankRect.intersects(craficRect)) {// 碰撞到了
if (tankRect.x + tankRect.width - craficRect.x != 1
&& craficRect.y + craficRect.height - tankRect.y != 1
&& tankRect.y + tankRect.height - craficRect.y != 1) {// 特殊情况
speed = 0;
break;
}
}
}
if (x >= 0 && x <= 895 - width + 1) {
x -= speed;
}
speed = 1;
}
if (deraction == 3) {// 向右
deraction = 3;
image = new ImageIcon("images/right1.png").getImage();
Rectangle tankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (tankRect.intersects(craficRect)) {// 碰撞到了
if (craficRect.x + craficRect.width - tankRect.x != 1
&& craficRect.y + craficRect.height - tankRect.y != 1
&& tankRect.y + tankRect.height - craficRect.y != 1) {// 特殊情况
speed = 0;
break;
}
}
}
if (x >= -1 && x <= 895 - width) {
x += speed;
}
speed = 1;
}
try {
Thread.sleep(4);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public Image getImage() {
return image;
}
public void setImage(Image image) {
this.image = image;
}
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
public int getY() {
return y;
}
public void setY(int y) {
this.y = y;
}
public int getSpeed() {
return speed;
}
public void setSpeed(int speed) {
this.speed = speed;
}
}
6:第六个类,我方坦克子弹类
package tankwar.copy;
import java.awt.Image;
import java.awt.Rectangle;
import javax.swing.ImageIcon;
public class Bullet extends Thread {
private Tank tank;
private Bullet bullet;
private EnemyBullet enemy_bullet;
public int speed = 1;
public int x = 28 * 4, y = 28 * 4;// 子弹坐标
public int deraction = 0;
private GamePanel gp;
private Image image;// 第一张子弹图片
public Image image1;
public boolean aimFlag = false;// 打中为true,打中墙壁或砖或j铁块或敌方坦克
private Crafic crafic;
private int width, height;
public Bullet(GamePanel gp, int x, int y, int deraction) {
this.gp = gp;
this.x = x;
this.y = y;
this.deraction = deraction;
this.tank = gp.gettank();
if ((deraction == 0 || deraction == 4) && tank.gun) {// 产生向上子弹
image = new ImageIcon("images/upGun.png").getImage();
this.x = this.x + 15;
this.y = this.y - 20;
tank.gun = false;
}
if ((deraction == 1 || deraction == 5) && tank.gun) {// 产生向下子弹
image = new ImageIcon("images/downGun.png").getImage();
this.x = this.x + 17;
this.y = this.y + 35;
tank.gun = false;
}
if ((deraction == 2 || deraction == 6) && tank.gun) {// 产生向左子弹
image = new ImageIcon("images/leftGun.png").getImage();
this.x = this.x - 25;
this.y = this.y + 11;
tank.gun = false;
}
if ((deraction == 3 || deraction == 7) && tank.gun) {// 产生向右子弹
image = new ImageIcon("images/rightGun.png").getImage();
this.x = this.x + tank.width;
this.y = this.y + tank.height / 2 - 3;
tank.gun = false;
}
}
public void run() {
while (true) {
// 判断子弹和敌方坦克的碰撞问题
Rectangle bulletRect = new Rectangle(x, y, 25, 15);
for (int i = 0; i < GamePanel.enemyTankList.size(); i++) {
EnemyTank enemyTank = GamePanel.enemyTankList.get(i);
Rectangle enemyTankRect = new Rectangle(enemyTank.x, enemyTank.y, 55, 35);
if (bulletRect.intersects(enemyTankRect)) {
aimFlag = true;
if (i == 0) {
enemyTank.x = 15;
enemyTank.y = 15;
}
if (i == 1) {
enemyTank.x = 450;
enemyTank.y = 15;
}
if (i == 2) {
enemyTank.x = 800;
enemyTank.y = 15;
}
}
}
if (((deraction == 0 || deraction == 4) && tank.gun)
|| (GamePanel.bulletList.size() != 0 && (deraction == 0 || deraction == 4))) {
y -= speed;
Rectangle BulletRect = new Rectangle(x, y, 23, 20);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (BulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.bulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (((deraction == 1 || deraction == 5) && tank.gun)
|| (GamePanel.bulletList.size() != 0 && (deraction == 1 || deraction == 5))) {
y += speed;
Rectangle BulletRect = new Rectangle(x, y, 21, 18);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (BulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.bulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (((deraction == 2 || deraction == 6) && tank.gun)
|| (GamePanel.bulletList.size() != 0 && (deraction == 2 || deraction == 6))) {
x -= speed;
Rectangle BulletRect = new Rectangle(x, y, 27, 14);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (BulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.bulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (((deraction == 3 || deraction == 7) && tank.gun)
|| (GamePanel.bulletList.size() != 0 && (deraction == 3 || deraction == 7))) {
x += speed;
Rectangle BulletRect = new Rectangle(x, y, 25, 15);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (BulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.bulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (x <= 0 || x >= 870 || y <= 0 || y >= 672) {// 如果碰到墙壁
GamePanel.bulletList.remove(this);// 删除子弹
return;// 结束线程
}
try {
Thread.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public Image getImage() {
return image;
}
public void setImage(Image image) {
this.image = image;
}
}
7:敌方坦克类
package tankwar.copy;
import java.awt.Image;
import java.awt.Rectangle;
import java.util.Random;
import javax.swing.ImageIcon;
public class EnemyTank extends Thread {
public int x = 15, y = 15;// 敌方坦克坐标
public int width = 55, height = 35;// 敌方坦克宽高
public Image image;// 敌方坦克图片
public int speed = 1;// 敌方坦克速度
public int speed1 = speed;
public int deraction = 1;// 敌方坦克方向 0是上 1是下 2是左 3是右 默认初始为下
public GamePanel gp;
public Crafic crafic;
public EnemyTank(GamePanel gp) {
this.gp = gp;
// 发射子弹线程
new Thread() {
public void run() {
while (true) {
EnemyBullet enemyBullet = new EnemyBullet(gp, x, y, deraction);
enemyBullet.start();
GamePanel.enemyBulletList.add(enemyBullet);
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
};
}.start();
}
public EnemyTank() {
}// 无参构造器
public void run() {
while (true) {
Random random = new Random();
if (deraction == 0) {// 上
image = new ImageIcon("images/Dup.png").getImage();
y -= speed;
Rectangle enemytankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemytankRect.intersects(craficRect) || y <= 0) {// 碰撞到了
if (enemytankRect.y + enemytankRect.height - craficRect.y != 1
&& enemytankRect.x + enemytankRect.width - craficRect.x != 1
&& craficRect.x + craficRect.width - enemytankRect.x != 1) {
speed = 0;
deraction = random.nextInt(4);
for (int j = 0; j < 10; j++) {
if (deraction == 0)
deraction = random.nextInt(4);
}
break;
}
}
}
speed = speed1;
}
if (deraction == 1) {// 下
image = new ImageIcon("images/dDown.png").getImage();
y += speed;
Rectangle enemytankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemytankRect.intersects(craficRect) || y >= 690 - 70) {// 碰撞到了
if (craficRect.y + craficRect.height - enemytankRect.y != 1
&& enemytankRect.x + enemytankRect.width - craficRect.x != 1
&& craficRect.x + craficRect.width - enemytankRect.x != 1) {
speed = 0;
deraction = random.nextInt(4);
for (int j = 0; j < 10; j++) {
if (deraction == 1)
deraction = random.nextInt(4);
}
break;
}
}
}
speed = speed1;
}
if (deraction == 2) {// 左
image = new ImageIcon("images/dleft.png").getImage();
x -= speed;
Rectangle enemytankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemytankRect.intersects(craficRect) || x <= 0 || x == 28 * 5 || x == 28 * 10 || x == 19 * 28
|| x == 24 * 28) {// 碰撞到了
if (enemytankRect.x + enemytankRect.width - craficRect.x != 1
&& craficRect.y + craficRect.height - enemytankRect.y != 1
&& enemytankRect.y + enemytankRect.height - craficRect.y != 1) {// 特殊情况
speed = 0;
deraction = random.nextInt(4);
for (int j = 0; j < 10; j++) {
if (deraction == 2)
deraction = random.nextInt(4);
}
break;
}
}
}
speed = speed1;
}
if (deraction == 3) {// 右
image = new ImageIcon("images/dRight.png").getImage();
x += speed;
Rectangle enemytankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemytankRect.intersects(craficRect) || x >= 895 - 55 || x == 28 * 5 || x == 28 * 10
|| x == 19 * 28 || x == 24 * 28) {// 碰撞到了
if (craficRect.x + craficRect.width - enemytankRect.x != 1
&& craficRect.y + craficRect.height - enemytankRect.y != 1
&& enemytankRect.y + enemytankRect.height - craficRect.y != 1) {// 特殊情况
speed = 0;
deraction = random.nextInt(4);
for (int j = 0; j < 10; j++) {
if (deraction == 3)
deraction = random.nextInt(4);
}
break;
}
}
}
speed = speed1;
}
try {
Thread.sleep(5);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
8:敌方坦克子弹类
package tankwar.copy;
import java.awt.Image;
import java.awt.Rectangle;
import javax.swing.ImageIcon;
public class EnemyBullet extends Thread {
public int x, y;
public int speed = 1;
public Image image;
public Image image1;
public GamePanel gp;
public Tank tank;
public Crafic crafic;
public int deraction = 0;// 方向
public boolean gun = true;
public EnemyBullet() {
}
public EnemyBullet(GamePanel gp, int x, int y, int dereaction) {
this.gp = gp;
this.tank = gp.gettank();
this.deraction = dereaction;
this.x = x;
this.y = y;
if (deraction == 0) {// 上
image = new ImageIcon("images/upGun.png").getImage();
this.x = this.x + 17;
this.y = this.y - 15;
}
if (deraction == 1) {// 下
image = new ImageIcon("images/downGun.png").getImage();
this.x = this.x + 18;
this.y = this.y + 33;
}
if (deraction == 2) {// 左
image = new ImageIcon("images/leftGun.png").getImage();
this.x = this.x - 28;
this.y = this.y + 13;
}
if (deraction == 3) {// 右
image = new ImageIcon("images/rightGun.png").getImage();
this.x = this.x + 50;
this.y = this.y + 10;
}
}
public void run() {
while (true) {
if (deraction == 0) {
y -= speed;
Rectangle enemyBulletRect = new Rectangle(x, y, 23, 20);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemyBulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (deraction == 1) {
y += speed;
Rectangle enemyBulletRect = new Rectangle(x, y, 21, 18);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemyBulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (deraction == 2) {
x -= speed;
Rectangle enemyBulletRect = new Rectangle(x, y, 27, 14);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemyBulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (deraction == 3) {
x += speed;
Rectangle enemyBulletRect = new Rectangle(x, y, 25, 15);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemyBulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (x <= 0 || x >= 870 || y <= 0 || y >= 672) {// 如果碰到墙壁
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
Rectangle enemyBulletRect = new Rectangle(x, y, 23, 20);
Rectangle TankRect = new Rectangle(tank.x, tank.y, 55, 35);
if (enemyBulletRect.intersects(TankRect)) {// 敌方子弹碰到我的坦克
tank.x = 284;
tank.y = 614;
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
Rectangle HomeRect = new Rectangle(421, 620, 56, 46);
if (enemyBulletRect.intersects(HomeRect)) {// 敌方子弹碰到我家
image1 = new ImageIcon("images/over.png").getImage();
}
try {
Thread.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
9,播放音乐类
package tankwar.copy;
import java.io.FileInputStream;
import sun.audio.AudioData;
import sun.audio.AudioPlayer;
import sun.audio.AudioStream;
import sun.audio.ContinuousAudioDataStream;
public class PlayMusic {
public String music1;
public static void playMusic(String music1) throws Exception {
FileInputStream fis = new FileInputStream(music1);
AudioStream music = new AudioStream(fis);
AudioData data = music.getData();
ContinuousAudioDataStream cad = new ContinuousAudioDataStream(data);
AudioPlayer.player.start(cad);
}
}
10,主类
package tankwar.copy;
public class Test {
public static void main(String[] args) throws Exception {
new TankWar().initFrame();
}
}
值得一提的是地图的制作方法,利用01串来制作地图















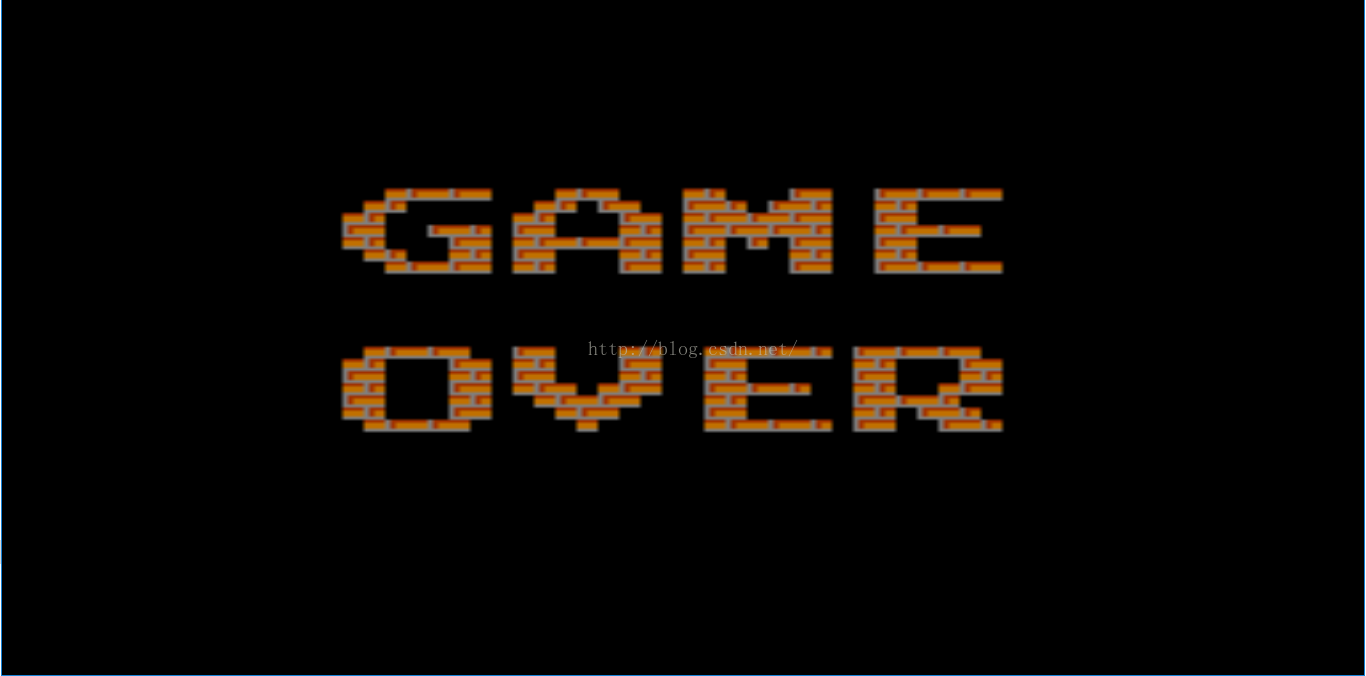
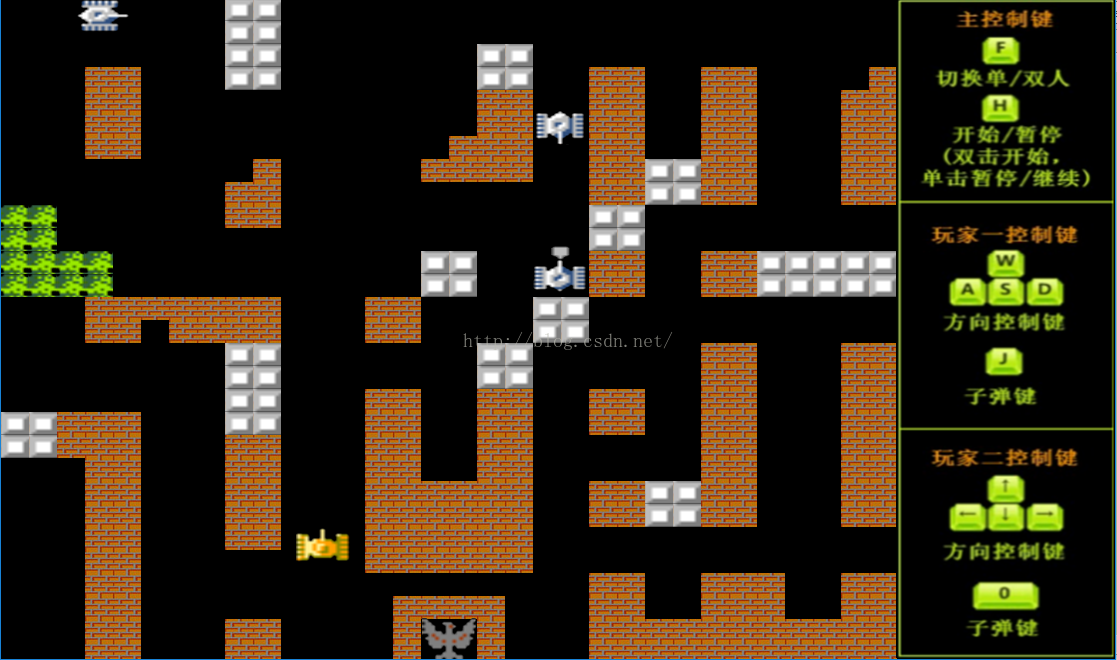
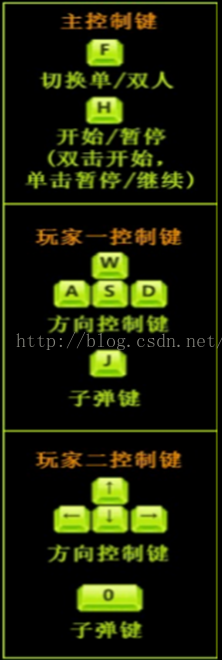
1:第一个类,窗体类。
package tankwar.copy;
import java.applet.Applet;
import java.applet.AudioClip;
import java.io.File;
import javax.swing.JFrame;
/**
* 1:创建窗体 2:坦克添加 3:障碍物添加与配置 4:坦克移动 5:发射子弹 6:碰撞检测等
*
* @author liurenyou
*
*/
public class TankWar extends JFrame {
public void initFrame() throws Exception {
this.setTitle("坦克大战");
this.setSize(1120, 690);
this.setLocationRelativeTo(null);
this.setResizable(false);
this.setDefaultCloseOperation(3);
GamePanel gp = new GamePanel();
this.add(gp);
this.setFocusable(false);
gp.setFocusable(true);
this.setVisible(true);
try {
File f = new File("images/start.wav");
AudioClip p = Applet.newAudioClip(f.toURI().toURL());
p.play();
} catch (Exception e) {
}
PlayMusic.playMusic("images/move.wav");
// PlayMusic.playMusic("images/life.wav");
// PlayMusic.playMusic("images/Nowin.wav");
// PlayMusic.playMusic("images/startHit.wav");
// PlayMusic.playMusic("images/bomb.wav");
new Thread() {
public void run() {
while (true) {
gp.repaint();
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
};
}.start();
}
}
2:第二个类,面板类,由于窗体太复杂,所以把面板添加到窗体上,通过控制面板来控制游戏。
package tankwar.copy;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import java.awt.image.BufferedImage;
import java.util.ArrayList;
import javax.swing.ImageIcon;
import javax.swing.JPanel;
public class GamePanel extends JPanel {
private Tank tank;
private Bullet bullet;
private EnemyTank enemyTank;
private EnemyBullet enemyBullet;
private Crafic crafic;
private ImageIcon icon = new ImageIcon("images/bird.png");
private ImageIcon icon1 = new ImageIcon("images/you.png");
public Image icon2;
public int[][] map;
public static ArrayList<EnemyTank> enemyTankList = new ArrayList<EnemyTank>();// 敌方坦克容器
public static ArrayList<Bullet> bulletList = new ArrayList<Bullet>();// 子弹容器
public static ArrayList<Crafic> craficList = new ArrayList<Crafic>();// 障碍物容器
public static ArrayList<EnemyBullet> enemyBulletList = new ArrayList<EnemyBullet>();// 敌方子弹容器
public GamePanel() {
tank = new Tank(this);// 我方坦克对象
initPanel();
tank.start();
// 创建敌方坦克对象
for (int i = 0; i < 3; i++) {
enemyTank = new EnemyTank(this);
enemyTank.start();
enemyTank.x += i * 400;
enemyTankList.add(enemyTank);
}
}
public Tank gettank() {// 获取Tank对象tank
return tank;
}
public EnemyTank getEnemyTank() {// 获取EnemyTank对象enemyTank
return enemyTank;
}
{// 代码块
map = ReadMap.readMap();
for (int i = 0; i < map.length; i++) {
for (int j = 0; j < map[i].length; j++) {
if (map[i][j] == 0) {
continue;
}
int x = j * 28;
int y = i * 23;
crafic = new Crafic(x, y, 28, 23);
if (map[i][j] == 1) {
crafic.image = new ImageIcon("images/brick.png");
} else if (map[i][j] == 2) {
crafic.image = new ImageIcon("images/stone.jpg");
}
craficList.add(crafic);
}
}
}
public void initPanel() {
KeyAdapter keyListener = new KeyAdapter() {
public void keyPressed(KeyEvent e) {
int code = e.getKeyCode();
if (code == 87) {// 向上
tank.deraction = 0;
} else if (code == 83) {// 向下
tank.deraction = 1;
} else if (code == 65) {// 向左
tank.deraction = 2;
} else if (code == 68) {// 向右
tank.deraction = 3;
} else if (code == 32) {// 空格
} else if (code == 74) {// j键发射子弹
tank.gun = true;
}
}
public void keyReleased(KeyEvent e) {
int code = e.getKeyCode();
if (code == 87) {// 向上
tank.deraction = 4;
} else if (code == 83) {// 向下
tank.deraction = 5;
} else if (code == 65) {// 向左
tank.deraction = 6;
} else if (code == 68) {// 向右
tank.deraction = 7;
} else if (code == 74) {// j
// tank.gun = false;
} else if (code == 32) {// 空格
}
}
};
this.addKeyListener(keyListener);
}
public void paint(Graphics g) {
BufferedImage bi = (BufferedImage) this.createImage(this.getWidth(), this.getHeight());
Graphics graphics = bi.getGraphics();
graphics.setColor(Color.BLACK);
graphics.fillRect(0, 0, 1365, 686);
for (int i = 0; i < craficList.size(); i++) {// 画障碍物
crafic = craficList.get(i);
graphics.drawImage(crafic.image.getImage(), crafic.x, crafic.y, null);
}
graphics.drawImage(icon.getImage(), 421, 620, null);// 画老鹰
graphics.drawImage(icon1.getImage(), 895, 0, null);// 画提示信息
for (int i = 0; i < enemyTankList.size(); i++) {
EnemyTank enemyTank = enemyTankList.get(i);
graphics.drawImage(enemyTank.image, enemyTank.x, enemyTank.y, null);// 画敌方坦克
}
graphics.drawImage(tank.getImage(), tank.getX(), tank.getY(), null);// 画坦克
for (int i = 0; i < bulletList.size(); i++) {
bullet = bulletList.get(i);
graphics.drawImage(bullet.getImage(), bullet.x, bullet.y, null);// 画我方子弹
}
for (int i = 0; i < enemyBulletList.size(); i++) {
enemyBullet = enemyBulletList.get(i);
graphics.drawImage(enemyBullet.image, enemyBullet.x, enemyBullet.y, null);// 画敌方子弹
}
g.drawImage(bi, 0, 0, null);
}
}
有窗体和面板了,就得往面板上添加一些元素,障碍物,坦克等等
3:第三个类,障碍物类
package tankwar.copy;
import javax.swing.ImageIcon;
/**
* 障碍物
*
* @author Administrator
*
*/
public class Crafic {
// 障碍物的xy坐标
public int x, y;
// 宽高度
public int width, height;
public ImageIcon image;
public Crafic(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
}
4:第四个类,读地图类。//我的障碍物是这样定义的,0代表砖块,1代表铁块,等等,所以我的地图是通过一个文件来储存的,里面是01串,然后通过这个类来读地图
package tankwar.copy;
import java.io.FileInputStream;
public class ReadMap {
/**
* 工具方法
*
* @return
*/
public static int[][] readMap() {
int[][] map = null;
try {
FileInputStream fis = new FileInputStream("images/image1");
// 先定义一个字节数组
byte[] bytes = new byte[fis.available()];
fis.read(bytes);
// 编程字符串
String str = new String(bytes).trim();
// 总行数
int row = 0;
int column = 0;
// 总行数
String[] strs = str.split("\r\n");
row = strs.length;
// 确定列数
if (strs != null && strs.length >= 1) {
String[] allColumn = strs[0].split(",");
column = allColumn.length;
}
map = new int[row][column];
// 存数据
for (int i = 0; i < strs.length; i++) {
String value = strs[i];
String[] vs = value.split(",");
for (int j = 0; j < vs.length; j++) {
String v = vs[j];
map[i][j] = Integer.parseInt(v);
}
}
} catch (Exception e) {
e.printStackTrace();
}
return map;
}
}
5:第五个类:我方坦克类
package tankwar.copy;
import java.awt.Image;
import java.awt.Rectangle;
import javax.swing.ImageIcon;
public class Tank extends Thread {
public boolean up = false, down = false, left = false, right = false;// 坦克方向
public int deraction = 4;// 定义坦克方向 0是上 1是下 2左 3是右 默认是上
public Crafic crafic;
public int speed = 1;// 坦克初始速度
public boolean gun = false;// 初始子弹默认不开
public int x = 284, y = 614;// 坦克初始坐标
public int width = 55, height = 35;// 坦克宽高
private Image image = new ImageIcon("images/up1.png").getImage();// 第一张坦克图片
private GamePanel gp;
public Tank(GamePanel gp) {// 构造函数
this.gp = gp;
new Thread() {
public void run() {
while (true) {
if (gun) {// 如果发射了子弹才添加
Bullet bullet = new Bullet(gp, x, y, deraction);
bullet.start();
System.out.println(GamePanel.bulletList.size());
GamePanel.bulletList.add(bullet);
}
try {
Thread.sleep(300);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
};
}.start();
}
public void run() {
while (true) {
if (deraction == 0) {// 向上
image = new ImageIcon("images/up1.png").getImage();
deraction = 0;
Rectangle tankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (tankRect.intersects(craficRect)) {// 碰撞到了
if (tankRect.y + tankRect.height - craficRect.y != 1
&& tankRect.x + tankRect.width - craficRect.x != 1
&& craficRect.x + craficRect.width - tankRect.x != 1) {
speed = 0;
break;
}
}
}
if (y > 0 && y <= 646) {
y -= speed;
}
speed = 1;
}
if (deraction == 1) {// 向下
deraction = 1;
image = new ImageIcon("images/down1.png").getImage();
Rectangle tankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (tankRect.intersects(craficRect)) {// 碰撞到了
if (craficRect.y + craficRect.height - tankRect.y != 1
&& tankRect.x + tankRect.width - craficRect.x != 1
&& craficRect.x + craficRect.width - tankRect.x != 1) {
speed = 0;
break;
}
}
}
if (y >= 0 && y <= 625) {
y += speed;
}
speed = 1;
}
if (deraction == 2) {// 向左
deraction = 2;
image = new ImageIcon("images/left1.png").getImage();
Rectangle tankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (tankRect.intersects(craficRect)) {// 碰撞到了
if (tankRect.x + tankRect.width - craficRect.x != 1
&& craficRect.y + craficRect.height - tankRect.y != 1
&& tankRect.y + tankRect.height - craficRect.y != 1) {// 特殊情况
speed = 0;
break;
}
}
}
if (x >= 0 && x <= 895 - width + 1) {
x -= speed;
}
speed = 1;
}
if (deraction == 3) {// 向右
deraction = 3;
image = new ImageIcon("images/right1.png").getImage();
Rectangle tankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (tankRect.intersects(craficRect)) {// 碰撞到了
if (craficRect.x + craficRect.width - tankRect.x != 1
&& craficRect.y + craficRect.height - tankRect.y != 1
&& tankRect.y + tankRect.height - craficRect.y != 1) {// 特殊情况
speed = 0;
break;
}
}
}
if (x >= -1 && x <= 895 - width) {
x += speed;
}
speed = 1;
}
try {
Thread.sleep(4);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public Image getImage() {
return image;
}
public void setImage(Image image) {
this.image = image;
}
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
public int getY() {
return y;
}
public void setY(int y) {
this.y = y;
}
public int getSpeed() {
return speed;
}
public void setSpeed(int speed) {
this.speed = speed;
}
}
6:第六个类,我方坦克子弹类
package tankwar.copy;
import java.awt.Image;
import java.awt.Rectangle;
import javax.swing.ImageIcon;
public class Bullet extends Thread {
private Tank tank;
private Bullet bullet;
private EnemyBullet enemy_bullet;
public int speed = 1;
public int x = 28 * 4, y = 28 * 4;// 子弹坐标
public int deraction = 0;
private GamePanel gp;
private Image image;// 第一张子弹图片
public Image image1;
public boolean aimFlag = false;// 打中为true,打中墙壁或砖或j铁块或敌方坦克
private Crafic crafic;
private int width, height;
public Bullet(GamePanel gp, int x, int y, int deraction) {
this.gp = gp;
this.x = x;
this.y = y;
this.deraction = deraction;
this.tank = gp.gettank();
if ((deraction == 0 || deraction == 4) && tank.gun) {// 产生向上子弹
image = new ImageIcon("images/upGun.png").getImage();
this.x = this.x + 15;
this.y = this.y - 20;
tank.gun = false;
}
if ((deraction == 1 || deraction == 5) && tank.gun) {// 产生向下子弹
image = new ImageIcon("images/downGun.png").getImage();
this.x = this.x + 17;
this.y = this.y + 35;
tank.gun = false;
}
if ((deraction == 2 || deraction == 6) && tank.gun) {// 产生向左子弹
image = new ImageIcon("images/leftGun.png").getImage();
this.x = this.x - 25;
this.y = this.y + 11;
tank.gun = false;
}
if ((deraction == 3 || deraction == 7) && tank.gun) {// 产生向右子弹
image = new ImageIcon("images/rightGun.png").getImage();
this.x = this.x + tank.width;
this.y = this.y + tank.height / 2 - 3;
tank.gun = false;
}
}
public void run() {
while (true) {
// 判断子弹和敌方坦克的碰撞问题
Rectangle bulletRect = new Rectangle(x, y, 25, 15);
for (int i = 0; i < GamePanel.enemyTankList.size(); i++) {
EnemyTank enemyTank = GamePanel.enemyTankList.get(i);
Rectangle enemyTankRect = new Rectangle(enemyTank.x, enemyTank.y, 55, 35);
if (bulletRect.intersects(enemyTankRect)) {
aimFlag = true;
if (i == 0) {
enemyTank.x = 15;
enemyTank.y = 15;
}
if (i == 1) {
enemyTank.x = 450;
enemyTank.y = 15;
}
if (i == 2) {
enemyTank.x = 800;
enemyTank.y = 15;
}
}
}
if (((deraction == 0 || deraction == 4) && tank.gun)
|| (GamePanel.bulletList.size() != 0 && (deraction == 0 || deraction == 4))) {
y -= speed;
Rectangle BulletRect = new Rectangle(x, y, 23, 20);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (BulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.bulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (((deraction == 1 || deraction == 5) && tank.gun)
|| (GamePanel.bulletList.size() != 0 && (deraction == 1 || deraction == 5))) {
y += speed;
Rectangle BulletRect = new Rectangle(x, y, 21, 18);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (BulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.bulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (((deraction == 2 || deraction == 6) && tank.gun)
|| (GamePanel.bulletList.size() != 0 && (deraction == 2 || deraction == 6))) {
x -= speed;
Rectangle BulletRect = new Rectangle(x, y, 27, 14);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (BulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.bulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (((deraction == 3 || deraction == 7) && tank.gun)
|| (GamePanel.bulletList.size() != 0 && (deraction == 3 || deraction == 7))) {
x += speed;
Rectangle BulletRect = new Rectangle(x, y, 25, 15);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (BulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.bulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (x <= 0 || x >= 870 || y <= 0 || y >= 672) {// 如果碰到墙壁
GamePanel.bulletList.remove(this);// 删除子弹
return;// 结束线程
}
try {
Thread.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public Image getImage() {
return image;
}
public void setImage(Image image) {
this.image = image;
}
}
7:敌方坦克类
package tankwar.copy;
import java.awt.Image;
import java.awt.Rectangle;
import java.util.Random;
import javax.swing.ImageIcon;
public class EnemyTank extends Thread {
public int x = 15, y = 15;// 敌方坦克坐标
public int width = 55, height = 35;// 敌方坦克宽高
public Image image;// 敌方坦克图片
public int speed = 1;// 敌方坦克速度
public int speed1 = speed;
public int deraction = 1;// 敌方坦克方向 0是上 1是下 2是左 3是右 默认初始为下
public GamePanel gp;
public Crafic crafic;
public EnemyTank(GamePanel gp) {
this.gp = gp;
// 发射子弹线程
new Thread() {
public void run() {
while (true) {
EnemyBullet enemyBullet = new EnemyBullet(gp, x, y, deraction);
enemyBullet.start();
GamePanel.enemyBulletList.add(enemyBullet);
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
};
}.start();
}
public EnemyTank() {
}// 无参构造器
public void run() {
while (true) {
Random random = new Random();
if (deraction == 0) {// 上
image = new ImageIcon("images/Dup.png").getImage();
y -= speed;
Rectangle enemytankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemytankRect.intersects(craficRect) || y <= 0) {// 碰撞到了
if (enemytankRect.y + enemytankRect.height - craficRect.y != 1
&& enemytankRect.x + enemytankRect.width - craficRect.x != 1
&& craficRect.x + craficRect.width - enemytankRect.x != 1) {
speed = 0;
deraction = random.nextInt(4);
for (int j = 0; j < 10; j++) {
if (deraction == 0)
deraction = random.nextInt(4);
}
break;
}
}
}
speed = speed1;
}
if (deraction == 1) {// 下
image = new ImageIcon("images/dDown.png").getImage();
y += speed;
Rectangle enemytankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemytankRect.intersects(craficRect) || y >= 690 - 70) {// 碰撞到了
if (craficRect.y + craficRect.height - enemytankRect.y != 1
&& enemytankRect.x + enemytankRect.width - craficRect.x != 1
&& craficRect.x + craficRect.width - enemytankRect.x != 1) {
speed = 0;
deraction = random.nextInt(4);
for (int j = 0; j < 10; j++) {
if (deraction == 1)
deraction = random.nextInt(4);
}
break;
}
}
}
speed = speed1;
}
if (deraction == 2) {// 左
image = new ImageIcon("images/dleft.png").getImage();
x -= speed;
Rectangle enemytankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemytankRect.intersects(craficRect) || x <= 0 || x == 28 * 5 || x == 28 * 10 || x == 19 * 28
|| x == 24 * 28) {// 碰撞到了
if (enemytankRect.x + enemytankRect.width - craficRect.x != 1
&& craficRect.y + craficRect.height - enemytankRect.y != 1
&& enemytankRect.y + enemytankRect.height - craficRect.y != 1) {// 特殊情况
speed = 0;
deraction = random.nextInt(4);
for (int j = 0; j < 10; j++) {
if (deraction == 2)
deraction = random.nextInt(4);
}
break;
}
}
}
speed = speed1;
}
if (deraction == 3) {// 右
image = new ImageIcon("images/dRight.png").getImage();
x += speed;
Rectangle enemytankRect = new Rectangle(x, y, width, height);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemytankRect.intersects(craficRect) || x >= 895 - 55 || x == 28 * 5 || x == 28 * 10
|| x == 19 * 28 || x == 24 * 28) {// 碰撞到了
if (craficRect.x + craficRect.width - enemytankRect.x != 1
&& craficRect.y + craficRect.height - enemytankRect.y != 1
&& enemytankRect.y + enemytankRect.height - craficRect.y != 1) {// 特殊情况
speed = 0;
deraction = random.nextInt(4);
for (int j = 0; j < 10; j++) {
if (deraction == 3)
deraction = random.nextInt(4);
}
break;
}
}
}
speed = speed1;
}
try {
Thread.sleep(5);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
8:敌方坦克子弹类
package tankwar.copy;
import java.awt.Image;
import java.awt.Rectangle;
import javax.swing.ImageIcon;
public class EnemyBullet extends Thread {
public int x, y;
public int speed = 1;
public Image image;
public Image image1;
public GamePanel gp;
public Tank tank;
public Crafic crafic;
public int deraction = 0;// 方向
public boolean gun = true;
public EnemyBullet() {
}
public EnemyBullet(GamePanel gp, int x, int y, int dereaction) {
this.gp = gp;
this.tank = gp.gettank();
this.deraction = dereaction;
this.x = x;
this.y = y;
if (deraction == 0) {// 上
image = new ImageIcon("images/upGun.png").getImage();
this.x = this.x + 17;
this.y = this.y - 15;
}
if (deraction == 1) {// 下
image = new ImageIcon("images/downGun.png").getImage();
this.x = this.x + 18;
this.y = this.y + 33;
}
if (deraction == 2) {// 左
image = new ImageIcon("images/leftGun.png").getImage();
this.x = this.x - 28;
this.y = this.y + 13;
}
if (deraction == 3) {// 右
image = new ImageIcon("images/rightGun.png").getImage();
this.x = this.x + 50;
this.y = this.y + 10;
}
}
public void run() {
while (true) {
if (deraction == 0) {
y -= speed;
Rectangle enemyBulletRect = new Rectangle(x, y, 23, 20);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemyBulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (deraction == 1) {
y += speed;
Rectangle enemyBulletRect = new Rectangle(x, y, 21, 18);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemyBulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (deraction == 2) {
x -= speed;
Rectangle enemyBulletRect = new Rectangle(x, y, 27, 14);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemyBulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (deraction == 3) {
x += speed;
Rectangle enemyBulletRect = new Rectangle(x, y, 25, 15);
for (int i = 0; i < GamePanel.craficList.size(); i++) {
crafic = GamePanel.craficList.get(i);
if (crafic != null) {
Rectangle craficRect = new Rectangle(crafic.x, crafic.y, crafic.width, crafic.height);
if (enemyBulletRect.intersects(craficRect)) {// 子弹碰撞到障碍物
if (gp.map[crafic.y / 23][crafic.x / 28] == 2)
GamePanel.craficList.remove(i);
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
}
}
}
if (x <= 0 || x >= 870 || y <= 0 || y >= 672) {// 如果碰到墙壁
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
Rectangle enemyBulletRect = new Rectangle(x, y, 23, 20);
Rectangle TankRect = new Rectangle(tank.x, tank.y, 55, 35);
if (enemyBulletRect.intersects(TankRect)) {// 敌方子弹碰到我的坦克
tank.x = 284;
tank.y = 614;
GamePanel.enemyBulletList.remove(this);// 删除子弹
return;// 结束线程
}
Rectangle HomeRect = new Rectangle(421, 620, 56, 46);
if (enemyBulletRect.intersects(HomeRect)) {// 敌方子弹碰到我家
image1 = new ImageIcon("images/over.png").getImage();
}
try {
Thread.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
9,播放音乐类
package tankwar.copy;
import java.io.FileInputStream;
import sun.audio.AudioData;
import sun.audio.AudioPlayer;
import sun.audio.AudioStream;
import sun.audio.ContinuousAudioDataStream;
public class PlayMusic {
public String music1;
public static void playMusic(String music1) throws Exception {
FileInputStream fis = new FileInputStream(music1);
AudioStream music = new AudioStream(fis);
AudioData data = music.getData();
ContinuousAudioDataStream cad = new ContinuousAudioDataStream(data);
AudioPlayer.player.start(cad);
}
}
10,主类
package tankwar.copy;
public class Test {
public static void main(String[] args) throws Exception {
new TankWar().initFrame();
}
}
值得一提的是地图的制作方法,利用01串来制作地图
相关文章推荐
- java对世界各个时区(TimeZone)的通用转换处理方法(转载)
- java-注解annotation
- java-模拟tomcat服务器
- java-用HttpURLConnection发送Http请求.
- java-WEB中的监听器Lisener
- Android IPC进程间通讯机制
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- 介绍一款信息管理系统的开源框架---jeecg
- 聚类算法之kmeans算法java版本
- java实现 PageRank算法
- PropertyChangeListener简单理解
- c++11 + SDL2 + ffmpeg +OpenAL + java = Android播放器
- 插入排序
- 冒泡排序
- 堆排序
- 快速排序
- 二叉查找树