SPRING IN ACTION 第4版笔记-第七章Advanced Spring MVC-005- 异常处理@ResponseStatus、@ExceptionHandler、@ControllerAdvice
2016-03-06 11:14
751 查看
No matter what happens, good or bad, the outcome of a servlet request is a servlet response. If an exception occurs during request processing, the outcome is still a servlet response. Somehow, the exception must be translated into a response.
Spring offers a handful of ways to translate exceptions to responses:
Certain Spring exceptions are automatically mapped to specific HTTP status codes.
An exception can be annotated with @ResponseStatus to map it to an HTTP status code.
A method can be annotated with @ExceptionHandler to handle the exception.
一、利用HTTP status codes的便利性
1.让Spring自动匹配Spring的自定义异常
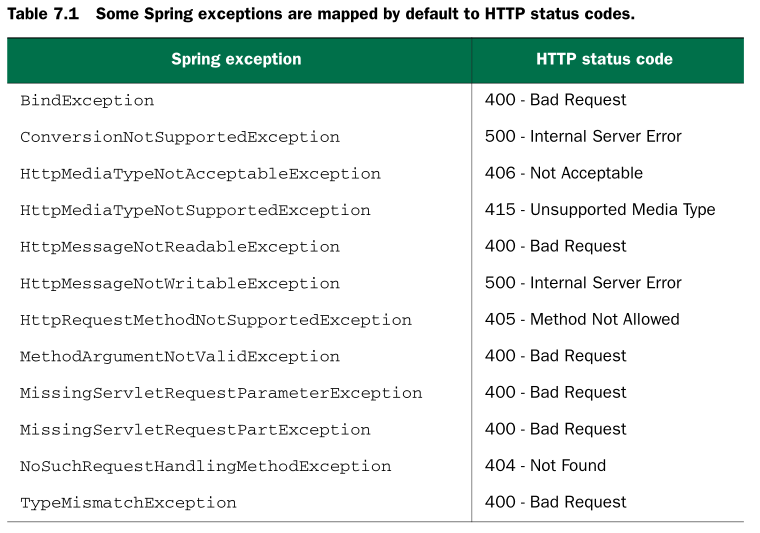
The exceptions in table 7.1 are usually thrown by Spring itself as the result of something going wrong in DispatcherServlet or while performing validation. For example, if DispatcherServlet can’t find a controller method suitable to handle a request,a NoSuchRequestHandlingMethodException will be thrown, resulting in a response
with a status code of 404 (Not Found).
2.自己来匹配
(1)假设consider the following request-handling method from SpittleController that could result in an HTTP 404 status (but doesn’t):
if findOne() returns null , then a SpittleNotFoundException is thrown. For now, SpittleNotFoundException is a simple unchecked exception that looks like this:
If the spittle() method is called on to handle a request, and the given ID comes up empty, the SpittleNotFoundException will (by default) result in a response with a 500 (Internal Server Error) status code. In fact, in the event of any exception that isn’t otherwise mapped, the response will always have a 500 status code. But you can change that by mapping SpittleNotFoundException otherwise.
When SpittleNotFoundException is thrown, it’s a situation where a requested resource isn’t found. The HTTP status code of 404 is precisely the appropriate response status code when a resource isn’t found. So, let’s use @ResponseStatus to
map SpittleNotFoundException to HTTP status code 404.
After introducing this @ResponseStatus annotation, if a SpittleNotFoundException were to be thrown from a controller method, the response would have a status code of 404 and a reason of Spittle Not Found.
运行结果:
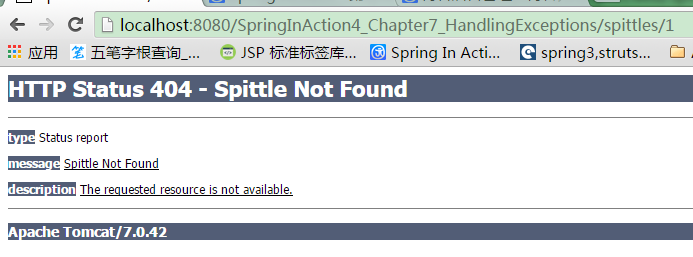
二、在controller级别处理异常@ExceptionHandler
1.自定义异常
2.抛出异常
suppose that SpittleRepository ’s save() method throws a DuplicateSpittleException if a user attempts to create a Spittle with text identical to one they’ve already created. That means the saveSpittle() method of SpittleController might need to deal with that exception. As shown in the following listing,saveSpittle() could directly handle the exception.
It works fine, but the method is a bit complex. Two paths can be taken, each with a different outcome. It’d be simpler if saveSpittle() could focus on the happy path and let some other method deal with the exception.First, let’s rip the exception-handling code out of saveSpittle() :很怀疑这里是不是会自己抛出异常DuplicateSpittleException,当保存的id重复时????
Now let’s add a new method to SpittleController that will handle the case where DuplicateSpittleException is thrown:
If @ExceptionHandler methods can handle exceptions thrown from any handler method in the same controller class, you might be wondering if there’s a way they can handle exceptions thrown from handler methods in any controller. As of Spring 3.2 they certainly can, but only if they’re defined in a controller advice class.
三、在application级别处理异常@ControllerAdvice
A controller advice is
any class that’s annotated with @ControllerAdvice and has one or more of the following kinds of methods:
@ExceptionHandler -annotated
@InitBinder -annotated
@ModelAttribute -annotated
Those methods in an @ControllerAdvice -annotated class are applied globally across all @RequestMapping -annotated methods on all controllers in an application.
The @ControllerAdvice annotation is itself annotated with @Component . Therefore,an @ControllerAdvice -annotated class will be picked up by component-scanning, just like an @Controller -annotated class.
处理应用中所有异常
Spring offers a handful of ways to translate exceptions to responses:
Certain Spring exceptions are automatically mapped to specific HTTP status codes.
An exception can be annotated with @ResponseStatus to map it to an HTTP status code.
A method can be annotated with @ExceptionHandler to handle the exception.
一、利用HTTP status codes的便利性
1.让Spring自动匹配Spring的自定义异常
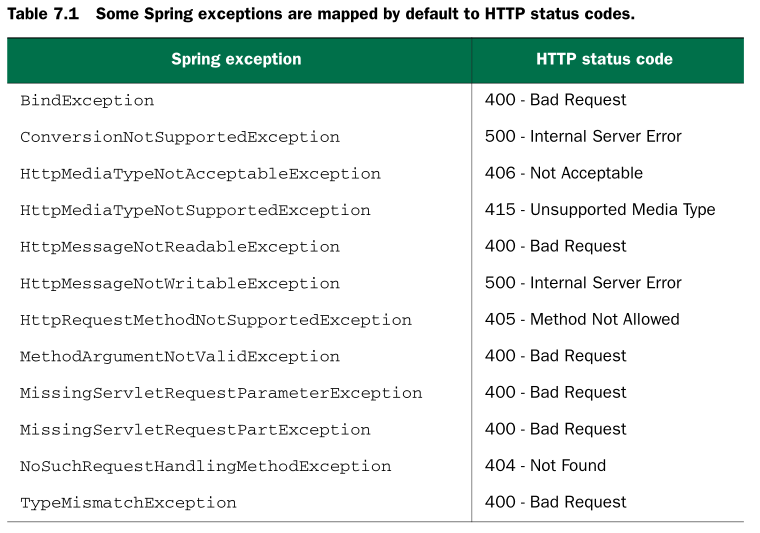
The exceptions in table 7.1 are usually thrown by Spring itself as the result of something going wrong in DispatcherServlet or while performing validation. For example, if DispatcherServlet can’t find a controller method suitable to handle a request,a NoSuchRequestHandlingMethodException will be thrown, resulting in a response
with a status code of 404 (Not Found).
2.自己来匹配
(1)假设consider the following request-handling method from SpittleController that could result in an HTTP 404 status (but doesn’t):
@RequestMapping(value="/{spittleId}", method=RequestMethod.GET) public String spittle( @PathVariable("spittleId") long spittleId, Model model) { Spittle spittle = spittleRepository.findOne(spittleId); if (spittle == null) { throw new SpittleNotFoundException(); } model.addAttribute(spittle); return "spittle"; }
if findOne() returns null , then a SpittleNotFoundException is thrown. For now, SpittleNotFoundException is a simple unchecked exception that looks like this:
package spittr.web; public class SpittleNotFoundException extends RuntimeException {}
If the spittle() method is called on to handle a request, and the given ID comes up empty, the SpittleNotFoundException will (by default) result in a response with a 500 (Internal Server Error) status code. In fact, in the event of any exception that isn’t otherwise mapped, the response will always have a 500 status code. But you can change that by mapping SpittleNotFoundException otherwise.
When SpittleNotFoundException is thrown, it’s a situation where a requested resource isn’t found. The HTTP status code of 404 is precisely the appropriate response status code when a resource isn’t found. So, let’s use @ResponseStatus to
map SpittleNotFoundException to HTTP status code 404.
package spittr.web; import org.springframework.http.HttpStatus; import org.springframework.web.bind.annotation.ResponseStatus; @ResponseStatus(value=HttpStatus.NOT_FOUND, reason="Spittle Not Found") public class SpittleNotFoundException extends RuntimeException { }
After introducing this @ResponseStatus annotation, if a SpittleNotFoundException were to be thrown from a controller method, the response would have a status code of 404 and a reason of Spittle Not Found.
运行结果:
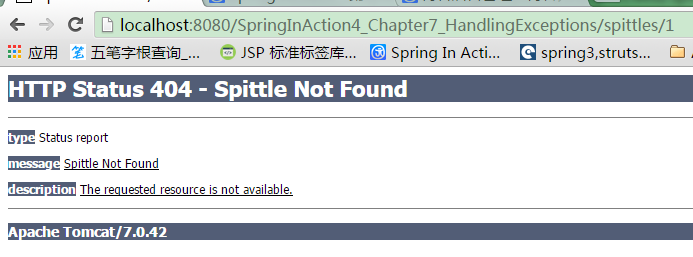
二、在controller级别处理异常@ExceptionHandler
1.自定义异常
package spittr.web; public class DuplicateSpittleException extends RuntimeException { }
2.抛出异常
suppose that SpittleRepository ’s save() method throws a DuplicateSpittleException if a user attempts to create a Spittle with text identical to one they’ve already created. That means the saveSpittle() method of SpittleController might need to deal with that exception. As shown in the following listing,saveSpittle() could directly handle the exception.
@RequestMapping(method = RequestMethod.POST) public String saveSpittle(SpittleForm form, Model model) { try { spittleRepository.save( new Spittle(null, form.getMessage(), new Date(), form.getLongitude(), form.getLatitude())); return "redirect:/spittles"; } catch (DuplicateSpittleException e) { return "error/duplicate"; } }
It works fine, but the method is a bit complex. Two paths can be taken, each with a different outcome. It’d be simpler if saveSpittle() could focus on the happy path and let some other method deal with the exception.First, let’s rip the exception-handling code out of saveSpittle() :很怀疑这里是不是会自己抛出异常DuplicateSpittleException,当保存的id重复时????
@RequestMapping(method = RequestMethod.POST) public String saveSpittle(SpittleForm form, Model model) { spittleRepository.save( new Spittle(null, form.getMessage(), new Date(), form.getLongitude(), form.getLatitude())); return "redirect:/spittles"; }
Now let’s add a new method to SpittleController that will handle the case where DuplicateSpittleException is thrown:
@ExceptionHandler(DuplicateSpittleException.class) public String handleDuplicateSpittle() { return "error/duplicate"; }
If @ExceptionHandler methods can handle exceptions thrown from any handler method in the same controller class, you might be wondering if there’s a way they can handle exceptions thrown from handler methods in any controller. As of Spring 3.2 they certainly can, but only if they’re defined in a controller advice class.
三、在application级别处理异常@ControllerAdvice
A controller advice is
any class that’s annotated with @ControllerAdvice and has one or more of the following kinds of methods:
@ExceptionHandler -annotated
@InitBinder -annotated
@ModelAttribute -annotated
Those methods in an @ControllerAdvice -annotated class are applied globally across all @RequestMapping -annotated methods on all controllers in an application.
The @ControllerAdvice annotation is itself annotated with @Component . Therefore,an @ControllerAdvice -annotated class will be picked up by component-scanning, just like an @Controller -annotated class.
处理应用中所有异常
package spittr.web; import org.springframework.web.bind.annotation.ControllerAdvice; import org.springframework.web.bind.annotation.ExceptionHandler; @ControllerAdvice public class AppWideExceptionHandler { @ExceptionHandler(DuplicateSpittleException.class) public String handleNotFound() { return "error/duplicate"; } }
相关文章推荐
- Java 回顾笔记_包
- 用Eclipse编写Java时调用algs4.jar和stdlib.jar解决StdOut和StdIn无法被识别的问题
- Java学习笔记
- 20145324 《Java程序设计》第1周学习总结
- Java的native方法
- java计划任务调度框架quartz结合spring实现调度的配置实例代码分享
- struts2 中redirectAction如何传递参数!
- 简单的springmvc的maven项目创建
- Java设计模式--观察者模式
- 20145214 《Java程序设计》第1周学习总结
- 第20课 :SPARK Top N彻底解秘 TOPN 排序(Scala)SPARK分组TOPN 算法(JAVA) 必须掌握!
- Java复习笔记(四)——Java基本类型的类型转换
- HDOJ——2002计算两点间的距离(java版)
- Spring MVC 数据绑定多个对象
- Java 类加载器
- HDOJ——2001 ASCII码排序(java 版)
- Java异常
- Java类加载器的工作原理
- Spring框架中IoC(控制反转)的原理(转)
- java中关键字volatile的作用