Java Cookie记录商品浏览历史
2016-02-28 20:59
615 查看
最后显示效果如下:
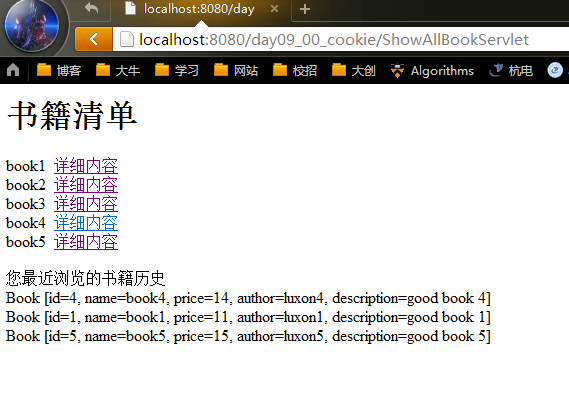
存储图书信息类Book.java
package com.app2;
public class Book {
private String id;
private String name;
private String price;
private String author;
private String description;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPrice() {
return price;
}
public void setPrice(String price) {
this.price = price;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
@Override
public String toString() {
return "Book [id=" + id + ", name=" + name + ", price=" + price + ", author=" + author + ", description="
+ description + "]";
}
public Book(String id, String name, String price, String author, String description) {
super();
this.id = id;
this.name = name;
this.price = price;
this.author = author;
this.description = description;
}
public Book() {}
}
模拟数据库类,存放图书信息
package com.app2;
import java.util.HashMap;
import java.util.Map;
/*
* 模拟数据
*/
public class BookDB {
// key:书的id value:id对应的书
private static Map<String, Book> books = new HashMap<String, Book>();
static {
books.put("1", new Book("1", "book1", "11", "luxon1", "good book 1"));
books.put("2", new Book("2", "book2", "12", "luxon2", "good book 2"));
books.put("3", new Book("3", "book3", "13", "luxon3", "good book 3"));
books.put("4", new Book("4", "book4", "14", "luxon4", "good book 4"));
books.put("5", new Book("5", "book5", "15", "luxon5", "good book 5"));
}
public static Map<String, Book> findAllBooks() {
return books;
}
public static Book findById(String id) {
return books.get(id);
}
}
显示所有图书信息主页的servlet
package com.app2;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Map;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/*
* 显示书的所有信息
*/
@WebServlet("/ShowAllBookServlet")
public class ShowAllBookServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
// 显示所有书籍
out.write("<h1>书籍清单</h1>");
Map<String, Book> books = BookDB.findAllBooks();
for (Map.Entry<String, Book> ele : books.entrySet()) {
out.write(ele.getValue().getName() + " " +
"<a href='"+request.getContextPath()+"/ShowDetailsSerblet?id="+ele.getKey()+"' target='_blank'>详细内容</a><br/>");
}
out.write("<br/>您最近浏览的书籍历史<br/>");
Cookie[] cs = request.getCookies();
for (int i = 0; cs != null && i < cs.length; i++) {
if ("bookHistory".equals(cs[i].getName())) {
String[] ids = cs[i].getValue().split("\\-");
for (String s : ids) {
out.write(BookDB.findById(s).toString() + "<br/>");
}
}
}
System.out.println(request.getRemoteAddr());
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
显示图书的详细信息,设置cookie的服务servlet
package com.app2;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/*
* 显示书的详细信息
* 组织数据,向客户端写入cookie
*/
@WebServlet("/ShowDetailsSerblet")
public class ShowDetailsSerblet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;UTF-8");
PrintWriter out = response.getWriter();
String id = request.getParameter("id");
Book book = BookDB.findById(id);
out.println(book.toString());
// 往客户端写入cookie
String bookId = makeId(id, request);
Cookie c = new Cookie("bookHistory", bookId);
c.setPath(request.getContextPath());
response.addCookie(c);
}
private String makeId(String id, HttpServletRequest request) {
// cookie为null
Cookie[] cs = request.getCookies();
if (cs == null) {
return id;
}
String result = "";
for (int i = 0; i < cs.length; i++) {
if ("bookHistory".equals(cs[i].getName())) {
// 浏览历史中不存在该id
if (cs[i].getValue().indexOf(id) == -1) {
result = id + "-" + cs[i].getValue();
break;
}
else if (cs[i].getValue().indexOf(id) == 0) {
result = cs[i].getValue();
break;
}
else {
result = id;
String[] ids = cs[i].getValue().split("\\-");
for (String str : ids) {
if (!str.equals(id)) {
result += "-" + str;
}
}
break;
}
}
}
if (result.length() <= 5) {
return result;
}
else {
return result.substring(0, 5);
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
存储图书信息类Book.java
package com.app2;
public class Book {
private String id;
private String name;
private String price;
private String author;
private String description;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPrice() {
return price;
}
public void setPrice(String price) {
this.price = price;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
@Override
public String toString() {
return "Book [id=" + id + ", name=" + name + ", price=" + price + ", author=" + author + ", description="
+ description + "]";
}
public Book(String id, String name, String price, String author, String description) {
super();
this.id = id;
this.name = name;
this.price = price;
this.author = author;
this.description = description;
}
public Book() {}
}
模拟数据库类,存放图书信息
package com.app2;
import java.util.HashMap;
import java.util.Map;
/*
* 模拟数据
*/
public class BookDB {
// key:书的id value:id对应的书
private static Map<String, Book> books = new HashMap<String, Book>();
static {
books.put("1", new Book("1", "book1", "11", "luxon1", "good book 1"));
books.put("2", new Book("2", "book2", "12", "luxon2", "good book 2"));
books.put("3", new Book("3", "book3", "13", "luxon3", "good book 3"));
books.put("4", new Book("4", "book4", "14", "luxon4", "good book 4"));
books.put("5", new Book("5", "book5", "15", "luxon5", "good book 5"));
}
public static Map<String, Book> findAllBooks() {
return books;
}
public static Book findById(String id) {
return books.get(id);
}
}
显示所有图书信息主页的servlet
package com.app2;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Map;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/*
* 显示书的所有信息
*/
@WebServlet("/ShowAllBookServlet")
public class ShowAllBookServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
// 显示所有书籍
out.write("<h1>书籍清单</h1>");
Map<String, Book> books = BookDB.findAllBooks();
for (Map.Entry<String, Book> ele : books.entrySet()) {
out.write(ele.getValue().getName() + " " +
"<a href='"+request.getContextPath()+"/ShowDetailsSerblet?id="+ele.getKey()+"' target='_blank'>详细内容</a><br/>");
}
out.write("<br/>您最近浏览的书籍历史<br/>");
Cookie[] cs = request.getCookies();
for (int i = 0; cs != null && i < cs.length; i++) {
if ("bookHistory".equals(cs[i].getName())) {
String[] ids = cs[i].getValue().split("\\-");
for (String s : ids) {
out.write(BookDB.findById(s).toString() + "<br/>");
}
}
}
System.out.println(request.getRemoteAddr());
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
显示图书的详细信息,设置cookie的服务servlet
package com.app2;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/*
* 显示书的详细信息
* 组织数据,向客户端写入cookie
*/
@WebServlet("/ShowDetailsSerblet")
public class ShowDetailsSerblet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;UTF-8");
PrintWriter out = response.getWriter();
String id = request.getParameter("id");
Book book = BookDB.findById(id);
out.println(book.toString());
// 往客户端写入cookie
String bookId = makeId(id, request);
Cookie c = new Cookie("bookHistory", bookId);
c.setPath(request.getContextPath());
response.addCookie(c);
}
private String makeId(String id, HttpServletRequest request) {
// cookie为null
Cookie[] cs = request.getCookies();
if (cs == null) {
return id;
}
String result = "";
for (int i = 0; i < cs.length; i++) {
if ("bookHistory".equals(cs[i].getName())) {
// 浏览历史中不存在该id
if (cs[i].getValue().indexOf(id) == -1) {
result = id + "-" + cs[i].getValue();
break;
}
else if (cs[i].getValue().indexOf(id) == 0) {
result = cs[i].getValue();
break;
}
else {
result = id;
String[] ids = cs[i].getValue().split("\\-");
for (String str : ids) {
if (!str.equals(id)) {
result += "-" + str;
}
}
break;
}
}
}
if (result.length() <= 5) {
return result;
}
else {
return result.substring(0, 5);
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
相关文章推荐
- cookie的secure属性详解
- 浏览器 cookie 限制
- 浅谈COOKIE和SESSION区别
- 深入解析Session是否必须依赖Cookie
- 对比分析php中Cookie与Session的异同
- 新手菜鸟必读:session与cookie的区别
- php实现通过cookie换肤的方法
- C#中Cookie之存储对象
- C#基于WebBrowser获取cookie的实现方法
- ASP.NET Cookie 操作实现
- php中cookie的作用域
- 写入cookie的JavaScript代码库 cookieLibrary.js
- ie7下利用ajax跨域盗取cookie的解决办法
- JS使用cookie实现DIV提示框只显示一次的方法
- js 通过cookie实现刷新不变化树形菜单
- 详谈javascript中的cookie
- 不要在cookie中使用特殊字符的原因分析
- cookie在javascript中的使用技巧以及隐私在服务器端的设置
- 二级域名Cookie问题的解决方法