数据结构之单向链表
2016-02-05 20:57
411 查看
[写文目的]
对于C语言初学者来说,链表可以说是一个难点(本人就深有体会),但是当你了解链表之后,你就会发现,其实链表也是很容易掌握的!
[链表介绍]
链表是一种基础的数据结构,主要是建立链表,增加元素,删除元素,查找元素
1.建立链表
首先需要定义一个链表的结构,一般包括存储在节点的值(该文默认为int)以及指向下一个节点的指针,一般如下:
2.增加元素
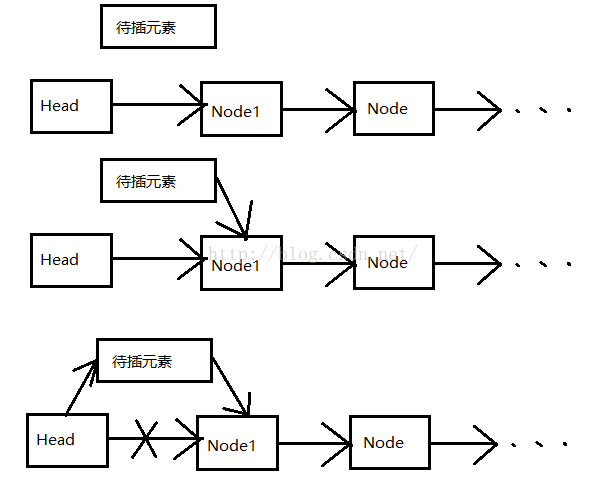
注意,插入的顺序一定是 (待插元素->next=Node1,Head->next=待插元素),对应的代码如下
通过便利找到要删除的元素,直接将该节点的前一个节点的next指针指向该节点的下一个节点,然后free掉该节点
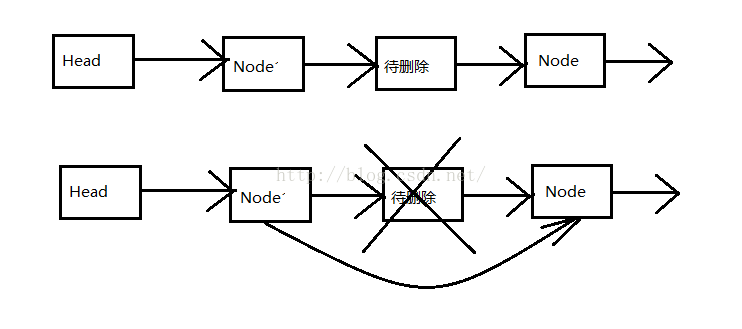
注意要标记当前节点的前一个节点,对应代码如下:
4,查找元素
查找元素只需要一次遍历即可,对应代码如下
[总结]
对于该题目我所使用的测试代码如下(仅供参考)
对于单向循环链表可以自己尝试去建立,下一篇将讲到双向循环链表!
对于C语言初学者来说,链表可以说是一个难点(本人就深有体会),但是当你了解链表之后,你就会发现,其实链表也是很容易掌握的!
[链表介绍]
链表是一种基础的数据结构,主要是建立链表,增加元素,删除元素,查找元素
1.建立链表
首先需要定义一个链表的结构,一般包括存储在节点的值(该文默认为int)以及指向下一个节点的指针,一般如下:
struct Node{ int data; Node* next; };但是为了后面使用的方便,我在这里做如下的定义
typedef struct Node* node; struct Node{ int data; node next; };创建一个链表代码如下,为了方便,一般设置一个头结点指向空
node creatList(){ node head=(node)malloc(sizeof(Node));//申请空间 head->next=NULL; return head; }
2.增加元素
注意,插入的顺序一定是 (待插元素->next=Node1,Head->next=待插元素),对应的代码如下
void insertItem(node head,int n){ node tempNode=(node)malloc(sizeof(Node)); tempNode->data=n; tempNode->next=head->next; head->next=tempNode; }3,删除元素
通过便利找到要删除的元素,直接将该节点的前一个节点的next指针指向该节点的下一个节点,然后free掉该节点
注意要标记当前节点的前一个节点,对应代码如下:
void deleteItem(node head,int n){ node last; for(node l=head->next;l!=NULL;last=l,l=l->next){ if(l->data==n){ last->next=l->next; free(l); return; } } }
4,查找元素
查找元素只需要一次遍历即可,对应代码如下
bool searchItem(node head,int n){ for(node l=head->next;l!=NULL;l=l->next){ if(l->data==n) return true; } return false; }
[总结]
对于该题目我所使用的测试代码如下(仅供参考)
#include<stdio.h>
#include<malloc.h>
typedef struct Node* node;
struct Node{
int data;
node next;
4000
};
node creatList(){
node L=(node)malloc(sizeof(Node));//申请空间
L->next=NULL;
return L;
}
void insertItem(node head,int n){ node tempNode=(node)malloc(sizeof(Node)); tempNode->data=n; tempNode->next=head->next; head->next=tempNode; }
void deleteItem(node head,int n){ node last; for(node l=head->next;l!=NULL;last=l,l=l->next){ if(l->data==n){ last->next=l->next; free(l); return; } } }
bool searchItem(node head,int n){ for(node l=head->next;l!=NULL;l=l->next){ if(l->data==n) return true; } return false; }
int main(){
node n;
n=creatList();
insertItem(n,4);
insertItem(n,8);
insertItem(n,6);
insertItem(n,9);
insertItem(n,3);
deleteItem(n,6);
if(searchItem(n,80)){
printf("找到!\n");
}else printf("未找到!\n");
for(node l=n->next;l!=NULL;l=l->next)
{
printf("%d ",l->data);
}
putchar('\n'); return 0;
}
对于单向循环链表可以自己尝试去建立,下一篇将讲到双向循环链表!
相关文章推荐
- 小蚂蚁学习数据结构(30)——图的其他知识点简介
- 数据结构(4)--循环链表的应用之约瑟夫环问题以及线性表总结之顺序表与链表的比较
- 【Redis笔记(六)】 Redis数据结构 - 有序集合zset
- 学习笔记------数据结构(C语言版)队列链式存储
- LinuxC常用数据结构及函数总结
- C++数据结构栈的实现
- Floodlight之 FloodlightContextStore 数据结构
- 单源最短路径之Bellman-Ford 算法
- 数据结构实验之二叉树六:哈夫曼编码
- Nginx源码分析 - 基础数据结构篇 - 单向链表结构 ngx_list.c
- 数据结构之红黑树与平衡二叉树
- 数据结构(3)--线性表实现一元多项式加法
- 【BZOJ4370】【IOI2015】horses 数据结构 平衡树+线段树
- 小蚂蚁学习数据结构(29)——图的存储表示
- 学习笔记------数据结构(C语言版)栈和递归 汉诺塔
- AC自动机入门
- 数据结构(2)--线性表单链表的主要操作的实现
- 数据结构之AVL树
- 微信涉及的重要数据结构-2
- Codevs_P2048 数据结构 2(SplayTree区间翻转)