iOS ——NSFileManager(文件管理)
2016-01-28 00:00
561 查看
摘要: NSFileManager类是用于实现沙盒目录中文件的管理,包含目录\文件的创建、删除、获取文件的属性数据等操作。
##1、文件保存的目录结构
iOS的文件存储采用的是沙盒目录机制,没有SD卡保存的概念。一个APP安装成功后就会对应一个文件目录这个目录就叫沙盒。这个APP的所有文件都会保存在这个目录中,每个APP沙盒独立互相不能访问目录,沙盒目录中包含Documents、Library、Tmp三个子目录。结构如下:
####应用沙盒路径:
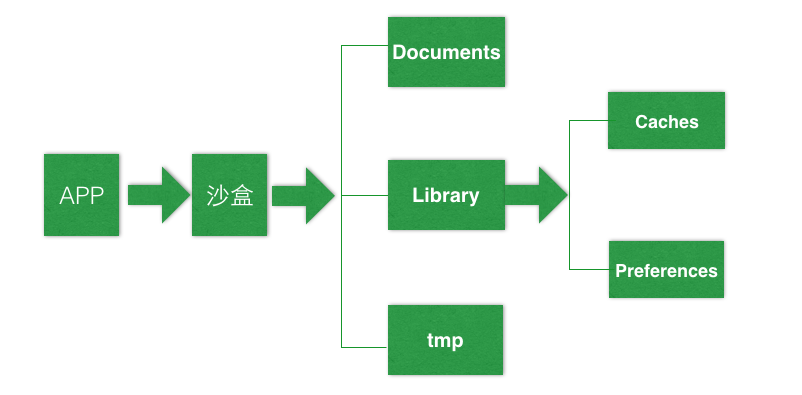

####Documents目录:
用于存储用户数据,iTunes备份和恢复的时候会包括此目录,程序创建产生的或在程序中浏览保存的文件数据放在该目录下,本地持久化的数据放在这个目录中。
####Library目录:
包含两个子目录:Caches 和 Preferences。Caches用来缓存的文件。Preferences保存通过NSUserDefaults创建的文件。
####Cache目录:
用于保存应用程序产生的缓存文件,程序需要手动缓存的数据也保存在此目录。做清理缓存的工作是需要清理这个目录的内容。
####Tmp目录:
用户保存应用程序参数的临时文件,程序关闭后这个目录中的文件被自动清理掉。
##2、NSFileManager管理文件目录
iOS中的文件管理由NSFileManager类实现,负责文件\目录的增删改查、获取数据属性等。
####创建NSFileManager对象
####创建目录
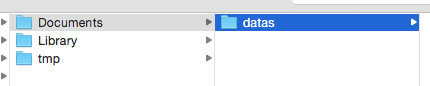
####创建文件

####删除文件
####写入数据

####读取数据
####获取文件属性
##1、文件保存的目录结构
iOS的文件存储采用的是沙盒目录机制,没有SD卡保存的概念。一个APP安装成功后就会对应一个文件目录这个目录就叫沙盒。这个APP的所有文件都会保存在这个目录中,每个APP沙盒独立互相不能访问目录,沙盒目录中包含Documents、Library、Tmp三个子目录。结构如下:
####应用沙盒路径:
// NSHomeDirectory() 获取沙盒目录 NSString *homePath = NSHomeDirectory(); NSLog(@"%@",homePath);
// command + shift + G 可以前往该文件夹 /Users/qinglinfu/Library/Developer/CoreSimulator/Devices/039371EC-EC36-40B3-9CB8-3065925EDB7D/data/Containers/Data/Application/55F7965F-0D92-4E88-B422-E423C9A732E1
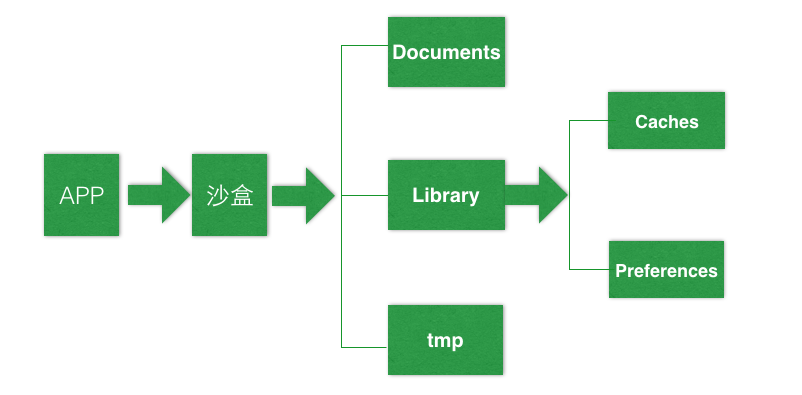

####Documents目录:
用于存储用户数据,iTunes备份和恢复的时候会包括此目录,程序创建产生的或在程序中浏览保存的文件数据放在该目录下,本地持久化的数据放在这个目录中。
directory:要查找的目录
domainMask:从哪个主目录中获取(通常是沙盒目录)
expandTilde: 是否返回完整的路径
// 获取目录路径的方法 NSArray<NSString *> *NSSearchPathForDirectoriesInDomains(NSSearchPathDirectory directory, NSSearchPathDomainMask domainMask, BOOL expandTilde);
// NSDocumentDirectory: documents目录 // NSUserDomainMask: 当前沙盒目录 NSString *path = [NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES) lastObject];
####Library目录:
包含两个子目录:Caches 和 Preferences。Caches用来缓存的文件。Preferences保存通过NSUserDefaults创建的文件。
NSString *path = [NSSearchPathForDirectoriesInDomains(NSLibraryDirectory, NSUserDomainMask, YES) lastObject];
####Cache目录:
用于保存应用程序产生的缓存文件,程序需要手动缓存的数据也保存在此目录。做清理缓存的工作是需要清理这个目录的内容。
NSString *path = [NSSearchPathForDirectoriesInDomains(NSCachesDirectory, NSUserDomainMask, YES) lastObject];
####Tmp目录:
用户保存应用程序参数的临时文件,程序关闭后这个目录中的文件被自动清理掉。
NSString *path = NSTemporaryDirectory();
##2、NSFileManager管理文件目录
iOS中的文件管理由NSFileManager类实现,负责文件\目录的增删改查、获取数据属性等。
####创建NSFileManager对象
// NSFileManager是一个单例对象,只实例化一次。 NSFileManager *manager = [NSFileManager defaultManager];
####创建目录
// 获取documents目录,将创建的新目录保存在该目录下 NSString *docPath = [NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES) lastObject]; // 目录的保存路径 NSString *directoryPath = [docPath stringByAppendingPathComponent:@"datas"]; // 使用NSFileManager 创建目录 BOOL isSuccess = [manager createDirectoryAtPath:directoryPath withIntermediateDirectories:YES attributes:nil error:nil]; if (isSuccess) { NSLog(@"创建成功!"); } else { NSLog(@"创建失败!"); }
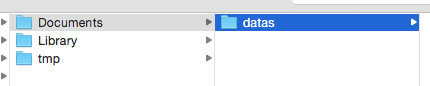
####创建文件
// 创建文件的保存路径 NSString *filePath = [directoryPath stringByAppendingPathComponent:@"userInfo.plist"]; //使用 NSFileManager 创建文件 BOOL isSuccess = [manager createFileAtPath:filePath contents:nil attributes:nil]; if (isSuccess) { NSLog(@"创建成功!"); } else { NSLog(@"创建失败!"); }

####删除文件
// 使用NSFileManager 移除指定路径的文件 BOOL isSuccess = [manager removeItemAtPath:directoryPath error:nil]; if (isSuccess) { NSLog(@"删除文件成功!"); } else { NSLog(@"删除文件失败!"); }
####写入数据
// 将字典持久保存到创建的plist文件中 NSMutableDictionary *info = [NSMutableDictionary dictionary]; [info setObject:@"2015年10月26日13:05:46" forKey:@"date"]; [info setObject:@"这个是持久化保存的数据内容" forKey:@"content"]; // 写入数据 BOOL isSuccess = [info writeToFile:filePath atomically:YES]; if (isSuccess) { NSLog(@"数据写入成功!"); } else { NSLog(@"数据写入失败!"); }

####读取数据
// 从保存的路径中读取对应数据类型的数据 NSDictionary *userInfo = [NSDictionary dictionaryWithContentsOfFile:filePath]; if (userInfo) { NSLog(@"%@",userInfo); } else { NSLog(@"数据读取失败!"); }
####获取文件属性
// 获取指定单个文件的属性 //NSDictionary *infoDic = [manager attributesOfItemAtPath:filePath error:nil]; // 获取指定目录下所有子文件路径 NSArray *subPaths = [manager subpathsAtPath:directoryPath]; // 获取每个子文件的属性 for (NSString *subPath in subPaths) { NSString *subPathString = [directoryPath stringByAppendingPathComponent:subPath]; NSDictionary *fileAttribute = [manager attributesOfItemAtPath:subPathString error:nil]; NSLog(@"%@",fileAttribute); }
// 文件属性列表 NSFileCreationDate = "2015-10-28 02:48:44 +0000"; NSFileExtensionHidden = 0; NSFileGroupOwnerAccountID = 80; NSFileGroupOwnerAccountName = admin; NSFileModificationDate = "2015-10-28 02:48:44 +0000"; NSFileOwnerAccountID = 501; NSFilePosixPermissions = 420; NSFileReferenceCount = 1; NSFileSize = 327; NSFileSystemFileNumber = 14750886; NSFileSystemNumber = 16777224; NSFileType = NSFileTypeRegular;
相关文章推荐
- 峰回路转,Firefox 浏览器即将重返 iOS 平台
- 峰回路转,Firefox 浏览器即将重返 iOS 平台
- 不可修补的 iOS 漏洞可能导致 iPhone 4s 到 iPhone X 永久越狱
- iOS 12.4 系统遭黑客破解,漏洞危及数百万用户
- 每日安全资讯:NSO,一家专业入侵 iPhone 的神秘公司
- [转][源代码]Comex公布JailbreakMe 3.0源代码
- 使用ShellClass获取文件属性详细信息的实现方法
- 讲解iOS开发中基本的定位功能实现
- js判断客户端是iOS还是Android等移动终端的方法
- IOS开发环境windows化攻略
- 浅析iOS应用开发中线程间的通信与线程安全问题
- 检测iOS设备是否越狱的方法
- .net平台推送ios消息的实现方法
- Windows批处理中获取文件属性的一些方法
- 探讨Android与iOS,我们将何去何从?
- Android、iOS和Windows Phone中的推送技术详解
- JAVA读取属性文件的几种方法总结
- IOS 改变键盘颜色代码
- 举例详解iOS开发过程中的沙盒机制与文件