使用自定义的item、Adapter和AsyncTask、第三方开源框架PullToRefresh联合使用实现自定义的下拉列表(从网络加载图片显示在item中的ImageView)
2015-12-23 19:08
1266 查看
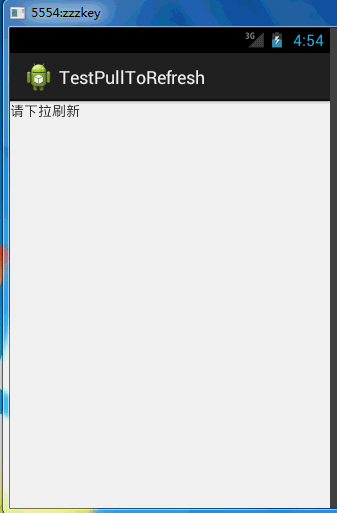
AsyncTask使用方法详情:/article/7168257.html
下拉开源框架PullToRefresh使用方法和下载详情:/article/7168293.html
具体实现的代码如下:
item.xml:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" > <ImageView android:id="@+id/imageView1" android:layout_width="50dp" android:layout_height="50dp" android:layout_alignParentLeft="true" android:src="@drawable/ic_launcher" /> <TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBottom="@+id/imageView1" android:layout_alignParentTop="true" android:layout_toLeftOf="@+id/imageView2" android:layout_toRightOf="@+id/imageView1" android:gravity="center" android:text="TextView" android:textColor="@android:color/holo_red_light" android:textSize="30sp" /> <ImageView android:id="@+id/imageView2" android:layout_width="50dp" android:layout_height="50dp" android:layout_alignParentRight="true" android:layout_alignParentTop="true" android:src="@drawable/ic_launcher" /> </RelativeLayout> item.xml
activity_main.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.zzw.testpulltorefresh.MainActivity" > <com.handmark.pulltorefresh.library.PullToRefreshListView android:id="@+id/listView" android:layout_width="match_parent" android:layout_height="match_parent" /> </RelativeLayout> activity_main.xml
MainActivity.java:
package com.zzw.testpulltorefresh; import java.io.BufferedInputStream; import java.io.ByteArrayOutputStream; import java.io.InputStream; import java.net.HttpURLConnection; import java.net.URL; import java.util.ArrayList; import java.util.Date; import java.util.HashMap; import com.handmark.pulltorefresh.library.PullToRefreshBase; import com.handmark.pulltorefresh.library.PullToRefreshBase.Mode; import com.handmark.pulltorefresh.library.PullToRefreshBase.OnRefreshListener; import com.handmark.pulltorefresh.library.PullToRefreshListView; import android.app.Activity; import android.content.Context; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.graphics.Color; import android.os.AsyncTask; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ArrayAdapter; import android.widget.ImageView; import android.widget.ListView; import android.widget.TextView; import android.widget.Toast; public class MainActivity extends Activity { private int COUNT = 0; private final String IMAGE_KEY1 = "IMAGE_KEY1"; private final String IMAGE_KEY2 = "IMAGE_KEY2"; private final String TEXT_KEY = "TEXT_KEY"; private PullToRefreshListView listView; private ArrayList<HashMap<String, Object>> data; private ArrayAdapter adapter; private String[] urls = { "http://p1.so.qhimg.com/bdr/_240_/t01829c584b50b68311.jpg", "http://p2.so.qhimg.com/bdr/_240_/t011c2cd4fe8723bcb2.jpg", "http://p4.so.qhimg.com/bdr/_240_/t01b11db3c0961b24a9.jpg", "http://p4.so.qhimg.com/bdr/_240_/t019786092c7b1688b9.jpg", "http://p4.so.qhimg.com/bdr/_240_/t015b226d64a10097ce.jpg", "http://p1.so.qhimg.com/bdr/_240_/t01f6c4382c907133ab.jpg" }; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); data = new ArrayList<HashMap<String, Object>>(); listView = (PullToRefreshListView) findViewById(R.id.listView); // 设置向下滑动时刷新 listView.setMode(Mode.PULL_FROM_START); // 支持下拉和上拉 listView.setMode(Mode.BOTH); // 设置监听 listView.setOnRefreshListener(new OnRefreshListener<ListView>() { @Override public void onRefresh(PullToRefreshBase<ListView> refreshView) { // 在这完成业务逻辑 new MyAsyncTask().execute(); } }); adapter = new MyAdapter(this, R.layout.item); listView.setAdapter(adapter); // 设置如果数据为空的时候显示什么 TextView textView = new TextView(this); textView.setText("请下拉刷新"); listView.setEmptyView(textView); } //自定义的Item显示参数设置在Adapter中操作 private class MyAdapter extends ArrayAdapter { private LayoutInflater inflater; private int item; public MyAdapter(Context context, int resource) { super(context, resource); inflater = LayoutInflater.from(context); this.item = resource; } @Override public int getCount() { return data.size(); } @Override public HashMap<String, Object> getItem(int position) { return data.get(position); } @Override public View getView(int position, View convertView, ViewGroup parent) { HashMap<String, Object> map = getItem(position); if (convertView == null) { convertView = inflater.inflate(item, null); } ImageView image1 = (ImageView) convertView.findViewById(R.id.imageView1); ImageView image2 = (ImageView) convertView.findViewById(R.id.imageView2); TextView textView = (TextView) convertView.findViewById(R.id.textView); image1.setImageBitmap((Bitmap) map.get(IMAGE_KEY1)); image2.setImageBitmap((Bitmap) map.get(IMAGE_KEY2)); textView.setText(map.get(TEXT_KEY) + ""); if (position % 2 == 1) { textView.setTextColor(Color.BLUE); } return convertView; } } //联网等一系列延时操作在AsyncTask中操作 private class MyAsyncTask extends AsyncTask { @Override protected void onPreExecute() { // 开始刷新 listView.setRefreshing(); } @Override protected Object doInBackground(Object... params) { HashMap<String, Object> map = new HashMap<String, Object>(); try { byte[] buf = loadRawDataFromURL(urls[COUNT]); BitmapFactory factory = new BitmapFactory(); Bitmap bitmap = factory.decodeByteArray(buf, 0, buf.length); map.put(TEXT_KEY, "数据->" + COUNT); map.put(IMAGE_KEY1, bitmap); map.put(IMAGE_KEY2, bitmap); } catch (Exception e) { e.printStackTrace(); } return map; } @Override protected void onPostExecute(Object result) { data.add(0,(HashMap<String, Object>) result); adapter.notifyDataSetChanged(); // 设置标签 listView.setLastUpdatedLabel("最后更新新的时间:" + new Date()); // 刷新完成 listView.onRefreshComplete(); COUNT++; if (COUNT >= urls.length) { COUNT = 0; } Toast.makeText(getApplicationContext(), "加载成功", 0).show(); } } // 通过URL读取字节数组 public static byte[] loadRawDataFromURL(String u) throws Exception { URL url = new URL(u); HttpURLConnection conn = (HttpURLConnection) url.openConnection(); InputStream is = conn.getInputStream(); BufferedInputStream bis = new BufferedInputStream(is); ByteArrayOutputStream baos = new ByteArrayOutputStream(); // 缓存2KB final int BUFFER_SIZE = 2 * 1024; final int EOF = -1; int c; byte[] buf = new byte[BUFFER_SIZE]; while (true) { c = bis.read(buf); if (c == EOF) break; baos.write(buf, 0, c); } conn.disconnect(); is.close(); byte[] b = baos.toByteArray(); baos.flush(); return b; } }
相关文章推荐
- ASIHTTPRequest简介
- ASIHTTPRequest运用
- IOS开发网络篇之──ASIHTTPRequest下载示例(支持断点续传)
- KJHttp的基础功能和工作原理
- 面试总结8--计算机网络相关问题Part2
- iOS 获取网络状态
- android4.0 HttpClient 以后不能在主线程发起网络请求
- java最简单的方式实现httpget和httppost请求
- 卷积神经网络基础
- 网络编程之IO复用:select or epoll
- jquery sortable的拖动方法内容说明和示例详解(转载http://www.jb51.net/article/45803.htm)
- jquery sortable的拖动方法内容说明和示例详解(转载http://www.jb51.net/article/45803.htm)
- TCP和UDP的区别有哪些?
- ASIHTTPRequest 上传文件无响应问题研究
- ASIHTTPRequest 上传文件无响应问题研究
- 批处理、分时、实时、网络、分布式操作系统的区别
- libnids中TCP/IP栈实现细节分析(上)——TCP会话重组
- Quartz实现动态定时任务--http://my.oschina.net/u/1177710/blog/284608
- Network Analysis]复杂网络分析总结
- TCP异常终止(reset报文)