C++中static关键字的使用总结
2015-11-28 14:41
344 查看
1、初始化
下代码中能够正确执行,在控制台中输出0,static int x语句做了两件事:定义了一个变量;将变量x初始化为0.
提示变量在使用之前没有初始化。
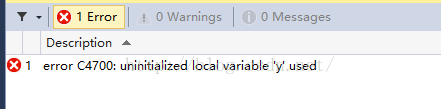
2、作用域
C++静态成员变量的作用域,使用static修饰的全局变量能够在各个函数内部被使用,但是不能再文件的外部使用。
这就是说,一般情况下同意工程中不同的cpp文件中的全局变量在编译时是彼此可见的,所以,在同一工程中,不能定义同名的全局变量,但是加入static关键字,在另一cpp文件赋值等是可以编译成功的。
如果在函数中声明了一个静态变量并初始化,然后该函数被main函数多次调用,变量会被定义初始化几次呢?看下例子:
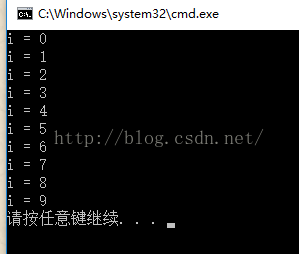
===================================================================
以下内容摘自MSDN:
The static keyword can be used to declare variables, functions, class data members and class functions.
By default, an object or variable that is defined outside all blocks has static duration and external linkage. Static duration means that the object or variable is allocated when the program starts and is deallocated when the program ends. External linkage
means that the name of the variable is visible from outside the file in which the variable is declared. Conversely, internal linkage means that the name is not visible outside the file in which the variable is declared.
The static keyword can be used in the following situations.
When you declare a variable or function at file scope (global and/or namespace scope), the static keyword specifies that the variable or function has internal linkage. When you declare a variable, the variable has static duration and the compiler initializes
it to 0 unless you specify another value.
When you declare a variable in a function, the static keyword specifies that the variable retains its state between calls to that function.
When you declare a data member in a class declaration, the static keyword specifies that one copy of the member is shared by all instances of the class. A static data member must be defined at file scope. An integral data member that you declare as const
static can have an initializer.
When you declare a member function in a class declaration, the static keyword specifies that the function is shared by all instances of the class. A static member function cannot access an instance member because the function does not have an implicit this
pointer. To access an instance member, declare the function with a parameter that is an instance pointer or reference.
You cannot declare the members of a union as static. However, a globally declared anonymous union must be explicitly declared static.
Examples:
1)The following example shows how a variable declared static in a function retains its state between calls to that function.
下代码中能够正确执行,在控制台中输出0,static int x语句做了两件事:定义了一个变量;将变量x初始化为0.
#include <stdio.h> #include <iostream> int main(void) { //int y; static int x; //printf("%d %d",x,y); printf("%d", x); }
</pre><p></p><p>下面代码发生错误</p><p></p><pre name="code" class="cpp">#include <stdio.h> #include <iostream> int main(void) { int y; static int x; printf("%d %d",x,y); //printf("%d", x); }
提示变量在使用之前没有初始化。
2、作用域
C++静态成员变量的作用域,使用static修饰的全局变量能够在各个函数内部被使用,但是不能再文件的外部使用。
这就是说,一般情况下同意工程中不同的cpp文件中的全局变量在编译时是彼此可见的,所以,在同一工程中,不能定义同名的全局变量,但是加入static关键字,在另一cpp文件赋值等是可以编译成功的。
如果在函数中声明了一个静态变量并初始化,然后该函数被main函数多次调用,变量会被定义初始化几次呢?看下例子:
#include <stdio.h> #include <iostream> using namespace std; static int x = 5;//定义一个静态全局变量x,在main函数内部可以访问 typedef void (FUNC)();//定义一个函数类型 FUNC fn;//声明一个函数fn int main(void) { for (int x = 0; x < 10; x++) { fn(); } } void fn() { static int i = 0; cout << "i = " << i++ << endl; }输出结果表示:静态变量仅仅被定义和初始化一次
===================================================================
以下内容摘自MSDN:
The static keyword can be used to declare variables, functions, class data members and class functions.
By default, an object or variable that is defined outside all blocks has static duration and external linkage. Static duration means that the object or variable is allocated when the program starts and is deallocated when the program ends. External linkage
means that the name of the variable is visible from outside the file in which the variable is declared. Conversely, internal linkage means that the name is not visible outside the file in which the variable is declared.
The static keyword can be used in the following situations.
When you declare a variable or function at file scope (global and/or namespace scope), the static keyword specifies that the variable or function has internal linkage. When you declare a variable, the variable has static duration and the compiler initializes
it to 0 unless you specify another value.
When you declare a variable in a function, the static keyword specifies that the variable retains its state between calls to that function.
When you declare a data member in a class declaration, the static keyword specifies that one copy of the member is shared by all instances of the class. A static data member must be defined at file scope. An integral data member that you declare as const
static can have an initializer.
When you declare a member function in a class declaration, the static keyword specifies that the function is shared by all instances of the class. A static member function cannot access an instance member because the function does not have an implicit this
pointer. To access an instance member, declare the function with a parameter that is an instance pointer or reference.
You cannot declare the members of a union as static. However, a globally declared anonymous union must be explicitly declared static.
Examples:
1)The following example shows how a variable declared static in a function retains its state between calls to that function.
// static1.cpp // compile with: /EHsc #include <iostream> using namespace std; void showstat( int curr ) { static int nStatic; // Value of nStatic is retained // between each function call nStatic += curr; cout << "nStatic is " << nStatic << endl; } int main() { for ( int i = 0; i < 5; i++ ) showstat( i ); }Output:
nStatic is 0 nStatic is 1 nStatic is 3 nStatic is 6 nStatic is 102)The following example shows the use of static in a class.
// static2.cpp // compile with: /EHsc #include <iostream> using namespace std; class CMyClass { public: static int m_i; }; int CMyClass::m_i = 0; CMyClass myObject1; CMyClass myObject2; int main() { cout << myObject1.m_i << endl; cout << myObject2.m_i << endl; myObject1.m_i = 1; cout << myObject1.m_i << endl; cout << myObject2.m_i << endl; myObject2.m_i = 2; cout << myObject1.m_i << endl; cout << myObject2.m_i << endl; CMyClass::m_i = 3; cout << myObject1.m_i << endl; cout << myObject2.m_i << endl; }Output:
0 0 1 1 2 2 3 33)The following example shows a local variable declared static in a member function. The static variable is available to the whole program; all instances of the type share the same copy of the static variable.
// static3.cpp // compile with: /EHsc #include <iostream> using namespace std; struct C { void Test(int value) { static int var = 0; if (var == value) cout << "var == value" << endl; else cout << "var != value" << endl; var = value; } }; int main() { C c1; C c2; c1.Test(100); c2.Test(100); }Output:
var != value var == value
相关文章推荐
- C++primer plus第六版课后编程练习答案8.2
- C++primer plus第六版课后编程练习答案8.1
- C语言字节对齐详解
- 一起talk C栗子吧(第六十五回:C语言实例--DIY字符串连接函数)
- C语言基础总结
- C语言中的特殊符号
- 【C++】前序线索化二叉树及其遍历
- C语言求最大公约数最小公倍数
- 【C++】后序线索化二叉树及其遍历
- c语言可变参数的两种用法
- C语言不定参数的函数
- c++ 深拷贝string类 简单实现
- 可变参数列表实现printf函数
- C/C++——程序实现过程之编译、链接和执行
- 数组传参,main传参(c++)
- 35.c/c++程序员面试宝典-容器
- C语言去掉字符串中的数字
- 在C++里类多一点好还是少一点好?
- 在C++里类多一点好还是少一点好?
- C++中char*与wchar_t*之间的转换