java多态与异常处理——动手动脑
2015-11-14 13:47
459 查看
代码一:
class Mammal{}
class Dog extends Mammal {}
class Cat extends Mammal{}
public class TestCast {
public static void main(String args[]) {
Mammal m;
Dog d=new Dog();
Cat c=new Cat();
m=d;
d=m;
d=(Dog)m;
d=c;
c=(Cat)m;
}
}
结果截图:
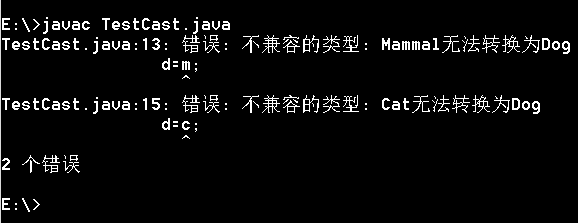
错误分析:
1、子类对象可以直接赋给基类变量。
2、基类对象要赋给子类对象变量,必须执行类型转换,其语法是:子类对象变量=(子类名)基类对象名;也不能乱转换。如果类型转换失败Java会抛出以下这种异常:ClassCastException
代码二:
import javax.swing.*;
class AboutException {
public static void main(String[] a) {
int i=1, j=0, k;
k=i/j;
try
{
k = i/j; // Causes division-by-zero exception
//throw new Exception("Hello.Exception!");
}
catch ( ArithmeticException e)
{
System.out.println("被0除. "+ e.getMessage());
}
catch (Exception e)
{
if (e instanceof ArithmeticException)
System.out.println("被0除");
else
{
System.out.println(e.getMessage());
}
}
finally
{
JOptionPane.showConfirmDialog(null,"OK");
}
}
}
结果截图:
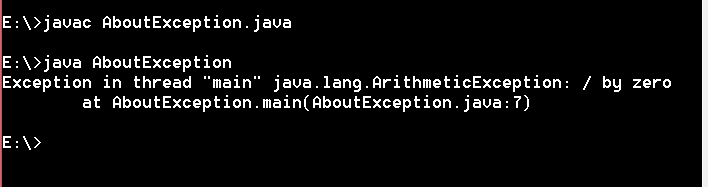
结果分析:
1、把可能会发生错误的代码放进try语句块中。
2、当程序检测到出现了一个错误时会抛出一个异常对象。异常处理代码会捕获并处理这个错误。
3、catch语句块中的代码用于处理错误。
4、当异常发生时,程序控制流程由try语句块跳转到catch语句块。
5、不管是否有异常发生,finally语句块中的语句始终保证被执行。
6、如果没有提供合适的异常处理代码,JVM将会结束掉整个应用程序。
代码三
public class CatchWho {
public static void main(String[] args) {
try {
try {
throw new ArrayIndexOutOfBoundsException();
}
catch(ArrayIndexOutOfBoundsException e) {
System.out.println( "ArrayIndexOutOfBoundsException" + "/内层try-catch");
}
throw new ArithmeticException();
}
catch(ArithmeticException e) {
System.out.println("发生ArithmeticException");
}
catch(ArrayIndexOutOfBoundsException e) {
System.out.println( "ArrayIndexOutOfBoundsException" + "/外层try-catch");
}
}
}
结果截图:

代码四:
public class CatchWho2 {
public static void main(String[] args) {
try {
try {
throw new ArrayIndexOutOfBoundsException();
}
catch(ArithmeticException e) {
System.out.println( "ArrayIndexOutOfBoundsException" + "/内层try-catch");
}
throw new ArithmeticException();
}
catch(ArithmeticException e) {
System.out.println("发生ArithmeticException");
}
catch(ArrayIndexOutOfBoundsException e) {
System.out.println( "ArrayIndexOutOfBoundsException" + "/外层try-catch");
}
}
}
结果截图:

结果分析:
1、可以有多个catch语句块,每个代码块捕获一种异常。在某个try块后有两个不同的catch 块捕获两个相同类型的异常是语法错误。
2、使用catch语句,只能捕获Exception类及其子类的对象。因此,一个捕获Exception对象的catch语句块可以捕获所有“可捕获”的异常。
3.将catch(Exception e)放在别的catch块前面会使这些catch块都不执行,因此Java不会编译这个程序。
代码五:
public class EmbededFinally {
public static void main(String args[]) {
int result;
try {
System.out.println("in Level 1");
try {
System.out.println("in Level 2"); // result=100/0; //Level 2
try {
System.out.println("in Level 3");
result=100/0; //Level 3
}
catch (Exception e) {
System.out.println("Level 3:" + e.getClass().toString());
}
finally {
System.out.println("In Level 3 finally");
} // result=100/0; //Level 2
}
catch (Exception e) {
System.out.println("Level 2:" + e.getClass().toString());
}
finally {
System.out.println("In Level 2 finally");
} // result = 100 / 0; //level 1
}
catch (Exception e) {
System.out.println("Level 1:" + e.getClass().toString());
}
finally {
System.out.println("In Level 1 finally");
}
}
}
结果截图:

结果分析:当有多层嵌套的finally时,异常在不同的层次抛出 ,在不同的位置抛出,可能会导致不同的finally语句块执行顺序。
class Mammal{}
class Dog extends Mammal {}
class Cat extends Mammal{}
public class TestCast {
public static void main(String args[]) {
Mammal m;
Dog d=new Dog();
Cat c=new Cat();
m=d;
d=m;
d=(Dog)m;
d=c;
c=(Cat)m;
}
}
结果截图:
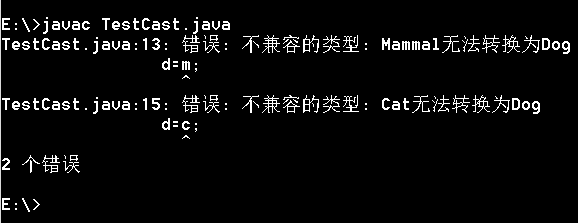
错误分析:
1、子类对象可以直接赋给基类变量。
2、基类对象要赋给子类对象变量,必须执行类型转换,其语法是:子类对象变量=(子类名)基类对象名;也不能乱转换。如果类型转换失败Java会抛出以下这种异常:ClassCastException
代码二:
import javax.swing.*;
class AboutException {
public static void main(String[] a) {
int i=1, j=0, k;
k=i/j;
try
{
k = i/j; // Causes division-by-zero exception
//throw new Exception("Hello.Exception!");
}
catch ( ArithmeticException e)
{
System.out.println("被0除. "+ e.getMessage());
}
catch (Exception e)
{
if (e instanceof ArithmeticException)
System.out.println("被0除");
else
{
System.out.println(e.getMessage());
}
}
finally
{
JOptionPane.showConfirmDialog(null,"OK");
}
}
}
结果截图:
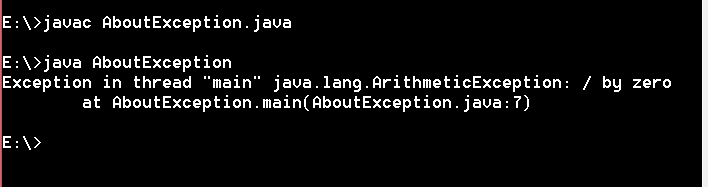
结果分析:
1、把可能会发生错误的代码放进try语句块中。
2、当程序检测到出现了一个错误时会抛出一个异常对象。异常处理代码会捕获并处理这个错误。
3、catch语句块中的代码用于处理错误。
4、当异常发生时,程序控制流程由try语句块跳转到catch语句块。
5、不管是否有异常发生,finally语句块中的语句始终保证被执行。
6、如果没有提供合适的异常处理代码,JVM将会结束掉整个应用程序。
代码三
public class CatchWho {
public static void main(String[] args) {
try {
try {
throw new ArrayIndexOutOfBoundsException();
}
catch(ArrayIndexOutOfBoundsException e) {
System.out.println( "ArrayIndexOutOfBoundsException" + "/内层try-catch");
}
throw new ArithmeticException();
}
catch(ArithmeticException e) {
System.out.println("发生ArithmeticException");
}
catch(ArrayIndexOutOfBoundsException e) {
System.out.println( "ArrayIndexOutOfBoundsException" + "/外层try-catch");
}
}
}
结果截图:

代码四:
public class CatchWho2 {
public static void main(String[] args) {
try {
try {
throw new ArrayIndexOutOfBoundsException();
}
catch(ArithmeticException e) {
System.out.println( "ArrayIndexOutOfBoundsException" + "/内层try-catch");
}
throw new ArithmeticException();
}
catch(ArithmeticException e) {
System.out.println("发生ArithmeticException");
}
catch(ArrayIndexOutOfBoundsException e) {
System.out.println( "ArrayIndexOutOfBoundsException" + "/外层try-catch");
}
}
}
结果截图:

结果分析:
1、可以有多个catch语句块,每个代码块捕获一种异常。在某个try块后有两个不同的catch 块捕获两个相同类型的异常是语法错误。
2、使用catch语句,只能捕获Exception类及其子类的对象。因此,一个捕获Exception对象的catch语句块可以捕获所有“可捕获”的异常。
3.将catch(Exception e)放在别的catch块前面会使这些catch块都不执行,因此Java不会编译这个程序。
代码五:
public class EmbededFinally {
public static void main(String args[]) {
int result;
try {
System.out.println("in Level 1");
try {
System.out.println("in Level 2"); // result=100/0; //Level 2
try {
System.out.println("in Level 3");
result=100/0; //Level 3
}
catch (Exception e) {
System.out.println("Level 3:" + e.getClass().toString());
}
finally {
System.out.println("In Level 3 finally");
} // result=100/0; //Level 2
}
catch (Exception e) {
System.out.println("Level 2:" + e.getClass().toString());
}
finally {
System.out.println("In Level 2 finally");
} // result = 100 / 0; //level 1
}
catch (Exception e) {
System.out.println("Level 1:" + e.getClass().toString());
}
finally {
System.out.println("In Level 1 finally");
}
}
}
结果截图:

结果分析:当有多层嵌套的finally时,异常在不同的层次抛出 ,在不同的位置抛出,可能会导致不同的finally语句块执行顺序。
相关文章推荐
- STS怎么创建一个springMVC的Maven项目
- Java学习笔记(面向对象1)
- Java中 对象克隆实例
- JDK和JVM
- 自己写的一个转换数字为中文大写形式的工具类
- Java基础之注解(annotation)
- 关于xml自定义颜色在xml布局的调用和java类中的调用
- Java泛型基础知识
- Java Web性能优化之一:减少DAO层的调用次数
- java按值传递理解
- [CareerCup] 14.4 Templates Java模板
- Java入门之数据类型以及变量的定义
- Java与Mysql开发中的特殊字符(包括Emoji)
- java.lang.IllegalArgumentException: Service Intent must be explicit异常说明
- java日志组件介绍(common-logging,log4j,slf4j,logback)
- java中Random()详解
- 解决spring、springMVC重复扫描导致事务失效的问题
- JavaFX透明窗口
- 如何给Spring MVC的action加上事务
- 利用java反射动态调整数组长度