安卓访问网络,从数据库拿数据
2015-10-24 12:30
525 查看
摘要: 客户端访问请求,服务端从数据库取数据,响应给客户端,
先写服务端,首先正确导入用到的jar包,json的和MySQL连接的,
服务端就是一个负责处理数据的Servlet
客户端
客户端主布局就是一个ListView,Java代码是接受json并处理
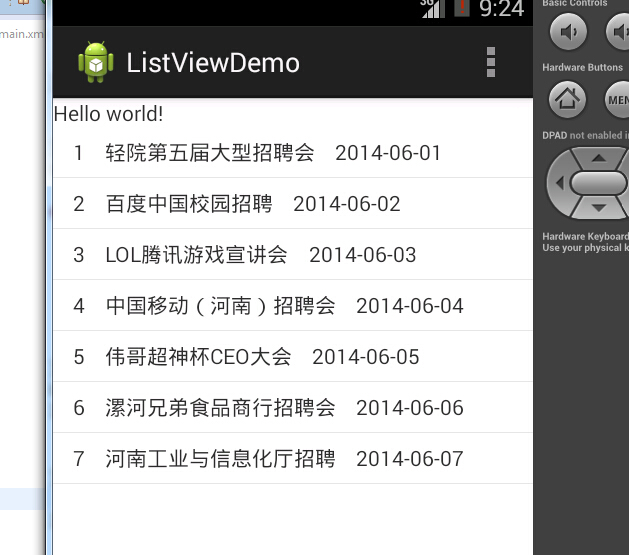

先写服务端,首先正确导入用到的jar包,json的和MySQL连接的,
服务端就是一个负责处理数据的Servlet
package com.ww.zphdemo.web; import java.io.IOException; import java.io.PrintWriter; import java.sql.Connection; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.json.JSONException; import org.json.JSONObject; import com.ww.zphdemo.util.JdbcMySQLUtil; /** * Servlet implementation class ZphInfoServlet */ @WebServlet("/ZphInfoServlet") public class ZphInfoServlet extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public ZphInfoServlet() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub response.setContentType("text/html;charset=utf-8"); response.setCharacterEncoding("utf-8"); PrintWriter out = response.getWriter(); //1.接收并判断请求 String req = request.getParameter("zphInfo"); System.out.println("客户端请求:"+req); //1.1如果请求不合法, if (!req.equals("zphInfo")) { out.write("{\"zphInfo\",\"请求不存在或网络故障!\"}"); }else{ //1.2如果请求合法,返回数据 Connection conn = null; Statement stmt = null; ResultSet rs = null; try { conn = JdbcMySQLUtil.getConn(); stmt = conn.createStatement(); String sql = "select *from zph_info"; rs = stmt.executeQuery(sql); List<Map<String, Object>> list = new ArrayList<>(); Map<String, Object> map ; while(rs.next()){ map = new HashMap<>(); //Map<String, Object> map = new LinkedHashMap<>(); map.put("zph_id", rs.getInt(1)); map.put("zph_name", rs.getString("name")); map.put("zph_date", rs.getDate("date")); list.add(map); } JSONObject json = new JSONObject(); json.put("zph_info", list); out.write(json.toString()); System.out.println("服务器反馈:"+json.toString()); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch(JSONException e){ e.printStackTrace(); } finally{ JdbcMySQLUtil.closeDB(rs, stmt, conn); } } } }
客户端
客户端主布局就是一个ListView,Java代码是接受json并处理
package com.ww.demo.listviewdemo; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; import java.util.concurrent.ExecutionException; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; import com.ww.demo.listviewdemo.util.HttpUtil; import android.os.Bundle; import android.app.Activity; import android.util.Log; import android.view.Menu; import android.view.View; import android.widget.AdapterView; import android.widget.AdapterView.OnItemClickListener; import android.widget.ListView; import android.widget.SimpleAdapter; import android.widget.Toast; public class MainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); int len = 0; //用于记录json数组的长度 String[] zph_name = null; String[] zph_date = null; //接收服务器反馈 try { JSONObject json = zphJson(); JSONArray jsonArr = (JSONArray) json.get("zph_info"); len = jsonArr.length(); zph_name = new String[len]; zph_date = new String[len]; for (int i = 0; i < len; i++) { JSONObject js = (JSONObject) jsonArr.get(i); zph_name[i] = (String) js.get("zph_name"); zph_date[i] = (String) js.get("zph_date"); } System.out.println("服务器反馈的json:"+json); } catch (JSONException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (ExecutionException e) { // TODO Auto-generated catch block e.printStackTrace(); } List<Map<String, Object>> list = new ArrayList<Map<String,Object>>(); for (int i = 0; i < len; i++) { Map<String, Object> map = new HashMap<String, Object>(); map.put("zph_id", i+1); map.put("zph_name", zph_name[i]); map.put("zph_date", zph_date[i]); list.add(map); } ListView lv = (ListView) findViewById(R.id.listView1); lv.setAdapter(new SimpleAdapter(this, list, R.layout.userinfo_content, new String[]{"zph_id","zph_name","zph_date"}, new int[]{R.id.zph_id, R.id.zph_name, R.id.zph_date} )); lv.setOnItemClickListener(new OnItemClickListener() { @Override public void onItemClick(AdapterView<?> arg0, View arg1, int arg2, long arg3) { // TODO Auto-generated method stub Log.d("arg2:", "arg2是什么: "+arg2); Log.d("arg3:", "arg3代表什么:"+arg3); Toast.makeText(MainActivity.this, arg3+"被点击了", Toast.LENGTH_SHORT).show(); } }); } /** * 定义发送请求的方法 * @throws ExecutionException * @throws InterruptedException * @throws JSONException * */ private JSONObject zphJson() throws JSONException, InterruptedException, ExecutionException{ //使用map封装请求体 Map<String, String> map = new HashMap<String, String>(); map.put("zphInfo", "zphInfo"); //定义发送请求的URL String url = HttpUtil.BASE_URL+"ZphInfoServlet"; //发送请求 return new JSONObject(HttpUtil.postRequest(url, map)); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu); return true; } }
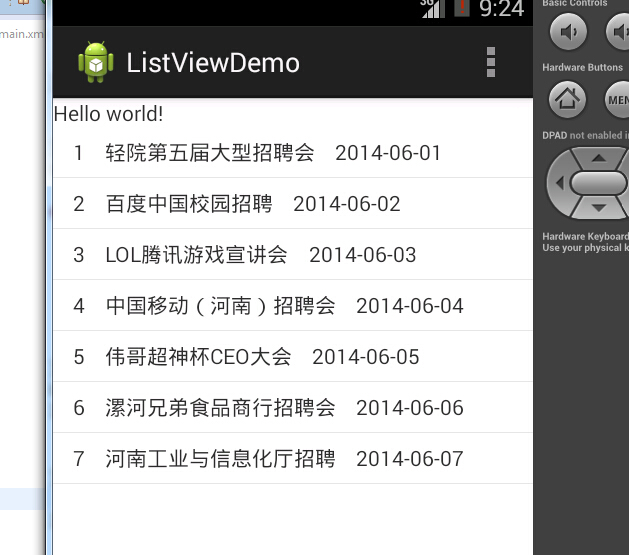


相关文章推荐
- 基于NanoHttpd的Android视频服务器开发
- 基于NanoHttpd的Android视频服务器开发
- 2015 ACM/ICPC Asia Regional Changchun Online
- HDU 5454 Excited Database (2015年沈阳赛区网络赛E题)
- TCP/IP基础知识
- 以太网OAM技术介绍 http://blog.chinaunix.net/uid-113269-id-267427.html
- hdoj 3549 Flow Problem【网络流最大流入门】
- Nodejs网络模块的选择
- android中图片的三级cache策略(内存、文件、网络)
- RHCE 系列(一):如何设置和测试静态网络路由
- 查看、关闭linux自启动网络服务
- android中图片的三级cache策略(内存、文件、网络)
- tcpdump+wireshark分析数据笔记(2)
- TCP/IP 协议栈及 OSI 参考模型详解
- 5.2的Dr.com客户端启动不了,3.7的客户端提示本机未安装TCPIP协议
- RHCE 7系列(一):如何设置和测试静态网络路由
- 10002---使用 XMLHttpRequest 实现 Ajax
- ASIHttpRequest的get和post应用
- Transport Security has blocked a cleartext HTTP
- Android网络通信框架LiteHttp:开篇简介和教程大纲