(原创)c#学习笔记04--流程控制03--分支02--if语句
2015-10-20 21:29
936 查看
4.3.2 if语句
if 语句没有结果(所以不在赋值语句中使用它),使用该语句是为了有条件地执行其他语句。
if语句最简单的语法如下:
if (<test>)
<code executed if <test> is true>;
先执行<test>(其计算结果必须是一个布尔值,这样代码才能编译),如果<test>的计算结果是true,就执行该语句之后的代码。在这段代码执行完毕后,或者因为<test>的计算结果是false,而没有执行这段代码,将继续执行后面的代码行。
也可以将else语句和if 语句合并使用,指定其他代码。如果<test>的计算结果是false,就执行else语句:
if (<test>)
<code executed if <test> is true>;
else
<code executed if <test> is false>;
可以使用成对的花括号将这两段代码放在多个代码行上:
if (<test>)
{
<code executed if <test> is true>;
}
else
{
<code executed if <test> is false>;
}
例如, 重新编写上一节使用三元运算符的代码:
string resultString = (myInteger < 10) ? "Less than 10" : "Greater than or equal to 10";
因为if语句的结果不能赋给一个变量,所以要单独将值赋给变量:
string resultString;
if (myInteger < 10)
resultString = "Less than 10";
else
resultString = "Greater than or equal to 10";
这样的代码尽管比较冗长,但与三元运算符相比,更便于阅读和理解,也更加灵活。
下面代码演示下if用法,代码如下:
运行结果如下:
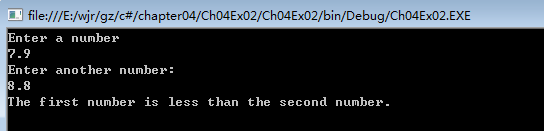
常见错误:常会错误地将诸如if (var1 == 3 ||var1 == 4)的条件写为if (var1 == 3 ||4)。由于运算符有优先级,因此先执行==运算符,接着用||运算符处理布尔和数值操作数,就会出现错误。
上面的代码可以使用else if语句,如下:
if 语句没有结果(所以不在赋值语句中使用它),使用该语句是为了有条件地执行其他语句。
if语句最简单的语法如下:
if (<test>)
<code executed if <test> is true>;
先执行<test>(其计算结果必须是一个布尔值,这样代码才能编译),如果<test>的计算结果是true,就执行该语句之后的代码。在这段代码执行完毕后,或者因为<test>的计算结果是false,而没有执行这段代码,将继续执行后面的代码行。
也可以将else语句和if 语句合并使用,指定其他代码。如果<test>的计算结果是false,就执行else语句:
if (<test>)
<code executed if <test> is true>;
else
<code executed if <test> is false>;
可以使用成对的花括号将这两段代码放在多个代码行上:
if (<test>)
{
<code executed if <test> is true>;
}
else
{
<code executed if <test> is false>;
}
例如, 重新编写上一节使用三元运算符的代码:
string resultString = (myInteger < 10) ? "Less than 10" : "Greater than or equal to 10";
因为if语句的结果不能赋给一个变量,所以要单独将值赋给变量:
string resultString;
if (myInteger < 10)
resultString = "Less than 10";
else
resultString = "Greater than or equal to 10";
这样的代码尽管比较冗长,但与三元运算符相比,更便于阅读和理解,也更加灵活。
下面代码演示下if用法,代码如下:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Ch04Ex02 { class Program { static void Main(string[] args) { string comparison; Console.WriteLine("Enter a number"); double var1 = Convert.ToDouble(Console.ReadLine()); Console.WriteLine("Enter another number:"); double var2 = Convert.ToDouble(Console.ReadLine()); if (var1 < var2) { comparison = "less than"; } else { if (var1 == var2) { comparison = "equal to"; } else { comparison = "greater than"; } } Console.WriteLine("The first number is {0} the second number.", comparison); Console.ReadLine(); } } }
运行结果如下:
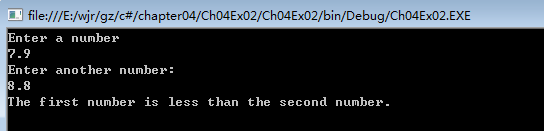
常见错误:常会错误地将诸如if (var1 == 3 ||var1 == 4)的条件写为if (var1 == 3 ||4)。由于运算符有优先级,因此先执行==运算符,接着用||运算符处理布尔和数值操作数,就会出现错误。
上面的代码可以使用else if语句,如下:
if (var1 < var2) { comparison = "less than"; } else if (var1 == var2) { comparison = "equal to"; } else { comparison = "greater than"; }
相关文章推荐
- .NET 应用程序中的内存
- (原创)c#学习笔记04--流程控制03--分支01--三元运算符
- (原创)c#学习笔记04--流程控制02--goto语句
- (原创)c#学习笔记04--流程控制01--布尔逻辑03--运算符优先级
- (原创)c#学习笔记04--流程控制01--布尔逻辑02--按位运算符
- c#调用cmd(可输入指令)
- c# 读取xml常用方法
- C#调用注册表,修改IE相关配置
- C#图片压缩
- C#中的异常捕获机制(try catch finally)
- 把C#程序(含多个Dll)合并成一个Exe的超简单方法
- C#的脚本编译
- VS中实时获取SVN的版本号并写入到AssemblyInfo.cs中(C#)
- 大白话系列之C#委托与事件讲解
- c# IEnumerable和IEnumerator & Lambda表达式
- C# WinForm 单例模式(例:同一个窗体只创建一次实例)
- C#学习日记27----属性
- 如何有效的使用C#读取文件
- C#中的隐藏和重写
- c# XML和实体类之间相互转换(序列化和反序列化)