Webpack初试
2015-10-08 23:43
525 查看
npm install -g webpack
老规矩,公司内网请设置代理:
npm config set proxy=http://127.0.0.1:8118
详细请参见官网 http://webpack.github.io/docs/tutorials/getting-started/
---------------------
1. 引用js
下面需要准备的文件的目录结构如下:
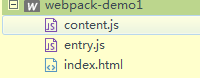
准备html页面 index.html
<html> <head> <meta charset="utf-8"> </head> <body> <script type="text/javascript" src="bundle.js" charset="utf-8"></script> </body> </html>
准备js
content.js
module.exports = "It works from content.js.";
entry.js
document.write(require("./content.js"));
用webpack命令编译,webpack命令的工作路径为entry.js所在路径:
webpack ./entry.js bundle.js
查看index.html便可以看到效果
2. 引用css
先安装loader,现在这个前端离开nodejs 还能玩么
用node安装需要的模块:
npm install css-loader style-loader
并在entry.js最上面加上如下代码:
require("!style!css!./style.css");
如果不想写前缀!style!css!,则对特定的文件扩展名指定特定的loader即可,下面是官网的详细介绍:
引用官网“
BINDING LOADERS
We don’t want to write such long requiresrequire("!style!css!./style.css");.
We can bind file extensions to loaders so we just need to write:
require("./style.css")
update
entry.js
- require("!style!css!./style.css"); + require("./style.css"); document.write(require("./content.js"));
Run the compilation with:
webpack ./entry.js bundle.js --module-bind "css=style!css"
”
编译后的bundles.js代码解读:
主要思路就是用数组(第一次读数组,第二次直接取缓存)存放了各个模块,一个require调用算一个模块,require css是通过加处理css的util的函数生成,然后用这些js函数向dom中加入css内容.
/******/ (function(modules) { // webpackBootstrap /******/ // The module cache /******/ var installedModules = {}; /******/ // The require function /******/ function __webpack_require__(moduleId) { /******/ // Check if module is in cache /******/ if(installedModules[moduleId]) /******/ return installedModules[moduleId].exports; /******/ // Create a new module (and put it into the cache) /******/ var module = installedModules[moduleId] = { /******/ exports: {}, /******/ id: moduleId, /******/ loaded: false /******/ }; /******/ // Execute the module function /******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__); /******/ // Flag the module as loaded /******/ module.loaded = true; /******/ // Return the exports of the module /******/ return module.exports; /******/ } /******/ // expose the modules object (__webpack_modules__) /******/ __webpack_require__.m = modules; /******/ // expose the module cache /******/ __webpack_require__.c = installedModules; /******/ // __webpack_public_path__ /******/ __webpack_require__.p = ""; /******/ // Load entry module and return exports /******/ return __webpack_require__(0); /******/ }) /************************************************************************/ /******/ ([ /* 0 */ /***/ function(module, exports, __webpack_require__) { __webpack_require__(1); document.write(__webpack_require__(5)); /***/ }, /* 1 */ /***/ function(module, exports, __webpack_require__) { // style-loader: Adds some css to the DOM by adding a <style> tag // load the styles var content = __webpack_require__(2); if(typeof content === 'string') content = [[module.id, content, '']]; // add the styles to the DOM var update = __webpack_require__(4)(content, {}); if(content.locals) module.exports = content.locals; // Hot Module Replacement if(false) { // When the styles change, update the <style> tags if(!content.locals) { module.hot.accept("!!./node_modules/css-loader/index.js!./style.css", function() { var newContent = require("!!./node_modules/css-loader/index.js!./style.css"); if(typeof newContent === 'string') newContent = [[module.id, newContent, '']]; update(newContent); }); } // When the module is disposed, remove the <style> tags module.hot.dispose(function() { update(); }); } /***/ }, /* 2 */ /***/ function(module, exports, __webpack_require__) { exports = module.exports = __webpack_require__(3)(); // imports // module exports.push([module.id, "body {\r\n background: yellow;\r\n}", ""]); // exports /***/ }, /* 3 */ /***/ function(module, exports) { /* MIT License http://www.opensource.org/licenses/mit-license.php Author Tobias Koppers @sokra */ // css base code, injected by the css-loader module.exports = function() { var list = []; // return the list of modules as css string list.toString = function toString() { var result = []; for(var i = 0; i < this.length; i++) { var item = this[i]; if(item[2]) { result.push("@media " + item[2] + "{" + item[1] + "}"); } else { result.push(item[1]); } } return result.join(""); }; // import a list of modules into the list list.i = function(modules, mediaQuery) { if(typeof modules === "string") modules = [[null, modules, ""]]; var alreadyImportedModules = {}; for(var i = 0; i < this.length; i++) { var id = this[i][0]; if(typeof id === "number") alreadyImportedModules[id] = true; } for(i = 0; i < modules.length; i++) { var item = modules[i]; // skip already imported module // this implementation is not 100% perfect for weird media query combinations // when a module is imported multiple times with different media queries. // I hope this will never occur (Hey this way we have smaller bundles) if(typeof item[0] !== "number" || !alreadyImportedModules[item[0]]) { if(mediaQuery && !item[2]) { item[2] = mediaQuery; } else if(mediaQuery) { item[2] = "(" + item[2] + ") and (" + mediaQuery + ")"; } list.push(item); } } }; return list; }; /***/ }, /* 4 */ /***/ function(module, exports, __webpack_require__) { /* MIT License http://www.opensource.org/licenses/mit-license.php Author Tobias Koppers @sokra */ var stylesInDom = {}, memoize = function(fn) { var memo; return function () { if (typeof memo === "undefined") memo = fn.apply(this, arguments); return memo; }; }, isOldIE = memoize(function() { return /msie [6-9]\b/.test(window.navigator.userAgent.toLowerCase()); }), getHeadElement = memoize(function () { return document.head || document.getElementsByTagName("head")[0]; }), singletonElement = null, singletonCounter = 0; module.exports = function(list, options) { if(false) { if(typeof document !== "object") throw new Error("The style-loader cannot be used in a non-browser environment"); } options = options || {}; // Force single-tag solution on IE6-9, which has a hard limit on the # of <style> // tags it will allow on a page if (typeof options.singleton === "undefined") options.singleton = isOldIE(); var styles = listToStyles(list); addStylesToDom(styles, options); return function update(newList) { var mayRemove = []; for(var i = 0; i < styles.length; i++) { var item = styles[i]; var domStyle = stylesInDom[item.id]; domStyle.refs--; mayRemove.push(domStyle); } if(newList) { var newStyles = listToStyles(newList); addStylesToDom(newStyles, options); } for(var i = 0; i < mayRemove.length; i++) { var domStyle = mayRemove[i]; if(domStyle.refs === 0) { for(var j = 0; j < domStyle.parts.length; j++) domStyle.parts[j](); delete stylesInDom[domStyle.id]; } } }; } function addStylesToDom(styles, options) { for(var i = 0; i < styles.length; i++) { var item = styles[i]; var domStyle = stylesInDom[item.id]; if(domStyle) { domStyle.refs++; for(var j = 0; j < domStyle.parts.length; j++) { domStyle.parts[j](item.parts[j]); } for(; j < item.parts.length; j++) { domStyle.parts.push(addStyle(item.parts[j], options)); } } else { var parts = []; for(var j = 0; j < item.parts.length; j++) { parts.push(addStyle(item.parts[j], options)); } stylesInDom[item.id] = {id: item.id, refs: 1, parts: parts}; } } } function listToStyles(list) { var styles = []; var newStyles = {}; for(var i = 0; i < list.length; i++) { var item = list[i]; var id = item[0]; var css = item[1]; var media = item[2]; var sourceMap = item[3]; var part = {css: css, media: media, sourceMap: sourceMap}; if(!newStyles[id]) styles.push(newStyles[id] = {id: id, parts: [part]}); else newStyles[id].parts.push(part); } return styles; } function createStyleElement() { var styleElement = document.createElement("style"); var head = getHeadElement(); styleElement.type = "text/css"; head.appendChild(styleElement); return styleElement; } function createLinkElement() { var linkElement = document.createElement("link"); var head = getHeadElement(); linkElement.rel = "stylesheet"; head.appendChild(linkElement); return linkElement; } function addStyle(obj, options) { var styleElement, update, remove; if (options.singleton) { var styleIndex = singletonCounter++; styleElement = singletonElement || (singletonElement = createStyleElement()); update = applyToSingletonTag.bind(null, styleElement, styleIndex, false); remove = applyToSingletonTag.bind(null, styleElement, styleIndex, true); } else if(obj.sourceMap && typeof URL === "function" && typeof URL.createObjectURL === "function" && typeof URL.revokeObjectURL === "function" && typeof Blob === "function" && typeof btoa === "function") { styleElement = createLinkElement(); update = updateLink.bind(null, styleElement); remove = function() { styleElement.parentNode.removeChild(styleElement); if(styleElement.href) URL.revokeObjectURL(styleElement.href); }; } else { styleElement = createStyleElement(); update = applyToTag.bind(null, styleElement); remove = function() { styleElement.parentNode.removeChild(styleElement); }; } update(obj); return function updateStyle(newObj) { if(newObj) { if(newObj.css === obj.css && newObj.media === obj.media && newObj.sourceMap === obj.sourceMap) return; update(obj = newObj); } else { remove(); } }; } var replaceText = (function () { var textStore = []; return function (index, replacement) { textStore[index] = replacement; return textStore.filter(Boolean).join('\n'); }; })(); function applyToSingletonTag(styleElement, index, remove, obj) { var css = remove ? "" : obj.css; if (styleElement.styleSheet) { styleElement.styleSheet.cssText = replaceText(index, css); } else { var cssNode = document.createTextNode(css); var childNodes = styleElement.childNodes; if (childNodes[index]) styleElement.removeChild(childNodes[index]); if (childNodes.length) { styleElement.insertBefore(cssNode, childNodes[index]); } else { styleElement.appendChild(cssNode); } } } function applyToTag(styleElement, obj) { var css = obj.css; var media = obj.media; var sourceMap = obj.sourceMap; if(media) { styleElement.setAttribute("media", media) } if(styleElement.styleSheet) { styleElement.styleSheet.cssText = css; } else { while(styleElement.firstChild) { styleElement.removeChild(styleElement.firstChild); } styleElement.appendChild(document.createTextNode(css)); } } function updateLink(linkElement, obj) { var css = obj.css; var media = obj.media; var sourceMap = obj.sourceMap; if(sourceMap) { // http://stackoverflow.com/a/26603875 css += "\n/*# sourceMappingURL=data:application/json;base64," + btoa(unescape(encodeURIComponent(JSON.stringify(sourceMap)))) + " */"; } var blob = new Blob([css], { type: "text/css" }); var oldSrc = linkElement.href; linkElement.href = URL.createObjectURL(blob); if(oldSrc) URL.revokeObjectURL(oldSrc); } /***/ }, /* 5 */ /***/ function(module, exports) { module.exports = "It works from content.js."; /***/ } /******/ ]);
真正让css插入到html dom对象中的是 346行
调用栈如下:
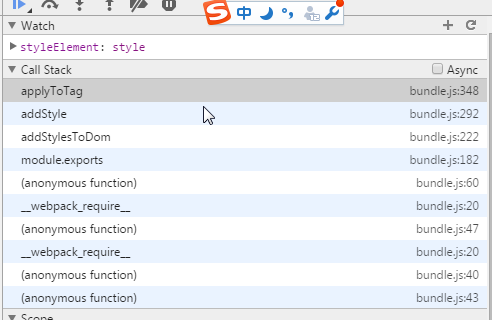
63-74行的代码压根不会起作用,不知道为什么会生成出来
整个代码的可读性不是很好
3. 编译进度条展示 加自动编译(auto build)
增加下面的选项即可:
webpack --progress --colors --watch
4.
部署到服务器…
5. 各种loader的支持
http://webpack.github.io/docs/list-of-loaders.html
如果是less的话 举例:
在js中加上
require("!style!css!less!./test.less");
end
相关文章推荐
- webpack速查
- webpack配置
- Grunt、webpack个人笔记
- webpack 学习笔记 03 Code Splitting
- webpack 学习笔记 02 快速入门
- webpack 学习笔记 01 使用webpack的原因
- webpack学习笔记
- 关于将Webpack,编译文件输出到不同的目录下
- 一小时包教会 —— webpack 入门指南
- webpack echarts配置实例
- webpack模块依赖管理介绍
- Webpack 入门指迷--转载(题叶)
- 用webpack来取代browserify
- webpack 使用加载器
- webpack 使用插件
- webpack 安装
- 一小时包教会 —— webpack 入门指南
- webpack学习 教程地址
- webpack 用法
- 学习笔记 一步步了解webpack